In MATLAB, the `color` property is often used to set the color of various graphical elements, allowing users to enhance visual representations of their data.
Here’s a simple example of how to change the color of a plot:
x = 0:0.1:10;
y = sin(x);
plot(x, y, 'Color', [0, 0.5, 0.8]); % Sets the color of the sine wave to a custom RGB value
Understanding Color in MATLAB
The Color Model
In MATLAB, color representation is primarily based on the RGB color model (Red, Green, Blue). This model is widely adopted in digital graphics and visualizations because it aligns closely with how colors are created on screens. Each color is generated by combining various intensities of red, green, and blue, which range from 0 to 1 in MATLAB.
In addition to RGB, MATLAB supports other color models, including HSV (Hue, Saturation, Value) which can often be more intuitive for manipulating colors based on human perception, and CMYK (Cyan, Magenta, Yellow, Black), generally used in printing contexts.
Understanding the fundamentals of these color representations is crucial for effective data visualization, ensuring that the colors you choose convey the right information effectively.
Color Specification in MATLAB
MATLAB provides multiple ways to specify colors, allowing for flexibility in how you apply them in your visualizations.
-
RGB Triples: An RGB triple is defined as a three-element vector, where each element corresponds to the intensity of red, green, and blue, respectively. The values should be in the range of 0 to 1. For instance, to set a custom color:
% Setting color using RGB Triples myColor = [0.5, 0.3, 0.2]; % RGB values plot(x, y, 'Color', myColor);
-
Color Names: MATLAB also includes a selection of predefined color names. Some common names include 'red', 'green', 'blue', and 'cyan'. This can simplify your code when you want to use standard colors:
% Using color name plot(x, y, 'Color', 'blue');
-
Hexadecimal Color Codes: For more precise control over colors, MATLAB allows you to define colors using hexadecimal codes, much like in web development. This method helps achieve the exact shade you desire:
% Using hexadecimal code plot(x, y, 'Color', '#FF6347'); % Tomato color
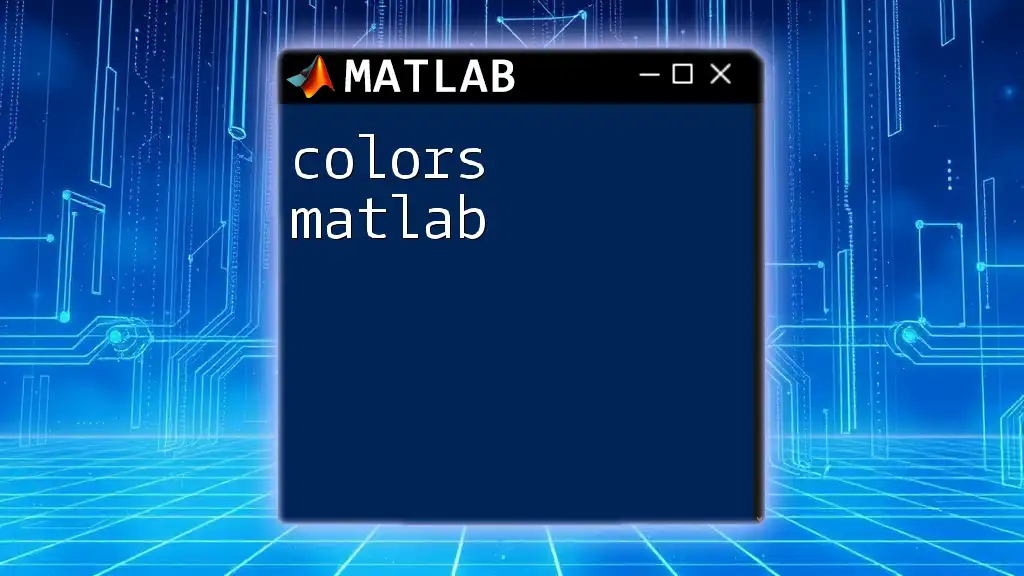
Customizing Plots with Color
Basic Plotting Functions
Color customization is readily available with MATLAB's basic plotting functions. You can specify color attributes to enhance your visual clarity. For example, if you want to visualize a sine wave in blue and make it stand out, you can do the following:
x = 0:0.1:10;
y = sin(x);
plot(x, y, 'LineWidth', 2, 'Color', [0, 0, 1]); % Custom blue line
In this example, the sine wave is rendered in blue with an increased line width, making it bolder and easier to examine.
Filling Areas with Color
Creating filled area plots can further enhance the visual impact of your data. This technique is useful for illustrating information like the area under a curve. For instance, you can use the `fill()` function as follows:
x = [1, 2, 3, 4, 5];
y = [2, 3, 4, 3, 2];
fill(x, y, 'cyan'); % Filling the area under the curve
This code applies a cyan fill to the specified polygon, visually emphasizing the area between the points, which can provide additional insight into the data being represented.
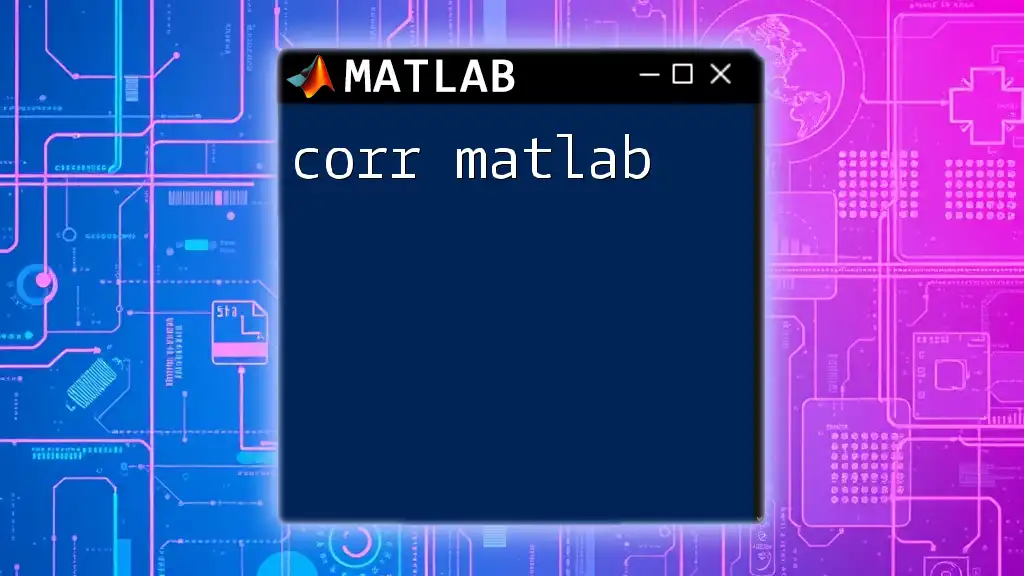
Advanced Color Customization
Color Maps
Color maps in MATLAB are essential for enhancing visual data representation, particularly when displaying matrices or 3D data. These maps define how data values correspond to colors, making it easier to interpret trends or patterns.
You can utilize predefined color maps or create a custom one to tailor your visualizations. For example, using a built-in color map such as 'jet' is straightforward:
surf(peaks); % Example surface plot
colormap(jet); % Apply the 'jet' colormap
This code snippet applies the 'jet' colormap to the surface plot of the peaks function, enhancing the visualization with a color gradient.
To create a custom color map, you might define specific RGB values like this:
myColors = [1, 0, 0; 0, 1, 0; 0, 0, 1]; % Red, Green, and Blue
colormap(myColors);
This example sets up a simple custom colormap with three colors, which could be utilized in conjunction with scatter or surface plots.
Using Color for Data Representation
Color can be a powerful tool for representing different data values effectively. In a scatter plot, for example, you can encode additional information with colors. Consider the following:
n = 100; % Number of points
x = rand(n, 1);
y = rand(n, 1);
c = x .* y; % Color data based on product of x and y
scatter(x, y, 50, c, 'filled'); % Scatter plot with color
In this example, the colors of the points in the scatter plot are determined by the product of their x and y coordinates. This not only visualizes the distribution of points but also highlights variations in their relationships based on color.
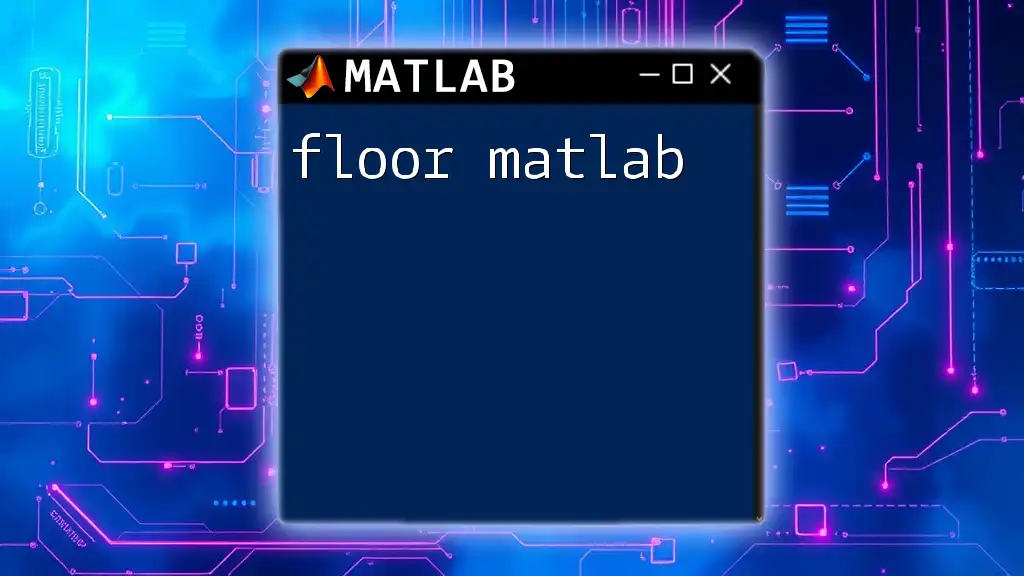
Color Theory Concepts Relevant to MATLAB Visualization
Contrast and Color Harmony
When designing visualizations, it is vital to consider contrast and color harmony. High contrast between foreground and background colors ensures that your plots are easily readable. Choose a color palette that is visually appealing and accurate in representation to your data.
Using color harmony—the pleasing arrangement of colors—can enhance viewers' understanding and retention of information presented in your visualizations.
Accessibility and Colorblindness Considerations
Lastly, it is crucial to consider accessibility in your color choices, especially given that a significant portion of the population experiences some form of color vision deficiency. To create inclusive visuals, leverage color palettes specifically designed for colorblind viewers. MATLAB has several recommendations and functions to help you choose colors that remain distinguishable for those with different types of color blindness.
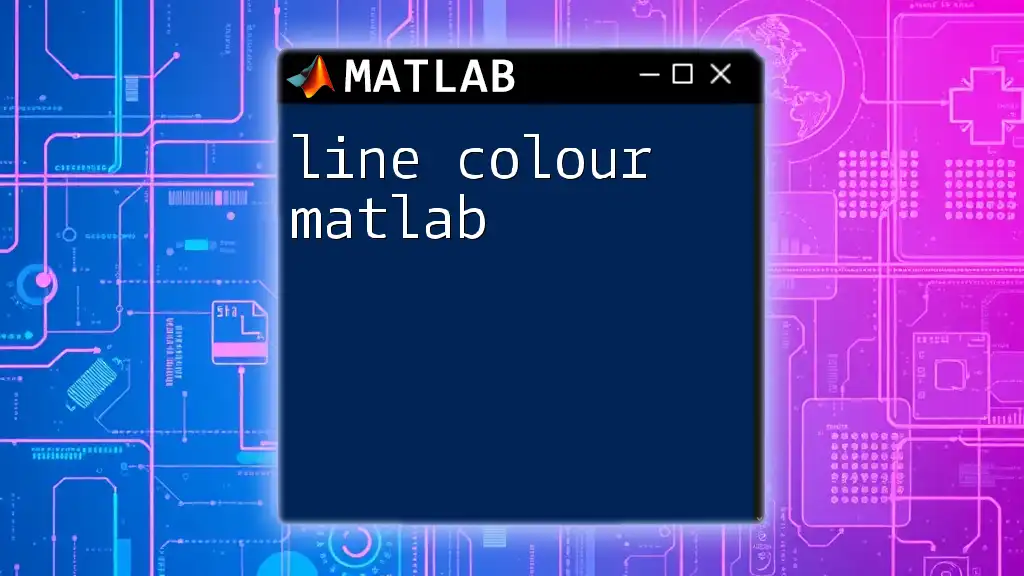
Conclusion
In conclusion, understanding and effectively implementing color in your MATLAB visualizations can vastly improve the clarity and appeal of your data presentations. By specifying colors accurately, customizing plots, and adhering to best practices in color theory, you will be well-equipped to create visually captivating and informative visualizations.
Through experimenting with various color commands and combinations in your own MATLAB projects, you can enhance the interpretability and engagement of your data visualizations, ensuring that they communicate the intended messages clearly and effectively.