"To solve equations in MATLAB, you can use the `solve` function, which allows you to find the roots of algebraic equations symbolically."
syms x; % Define the variable
eq = x^2 - 4 == 0; % Define the equation
solution = solve(eq, x); % Solve the equation
disp(solution); % Display the solution
Understanding the `solve` Function
What is the `solve` Function?
The `solve` function in MATLAB is a powerful tool designed for solving equations analytically. Whether you are faced with simple algebraic equations or complex systems, `solve` provides the means to find exact symbolic solutions. This makes it invaluable for engineers, mathematicians, and scientists who often need quick resolutions to mathematical problems.
Syntax and Parameters
The general syntax of the `solve` function is straightforward:
solution = solve(equation, variable)
In this syntax:
- equation: The equation you wish to solve.
- variable: The variable for which you want to find the solution.
Both parameters can be varied according to the complexity of the equations involved.
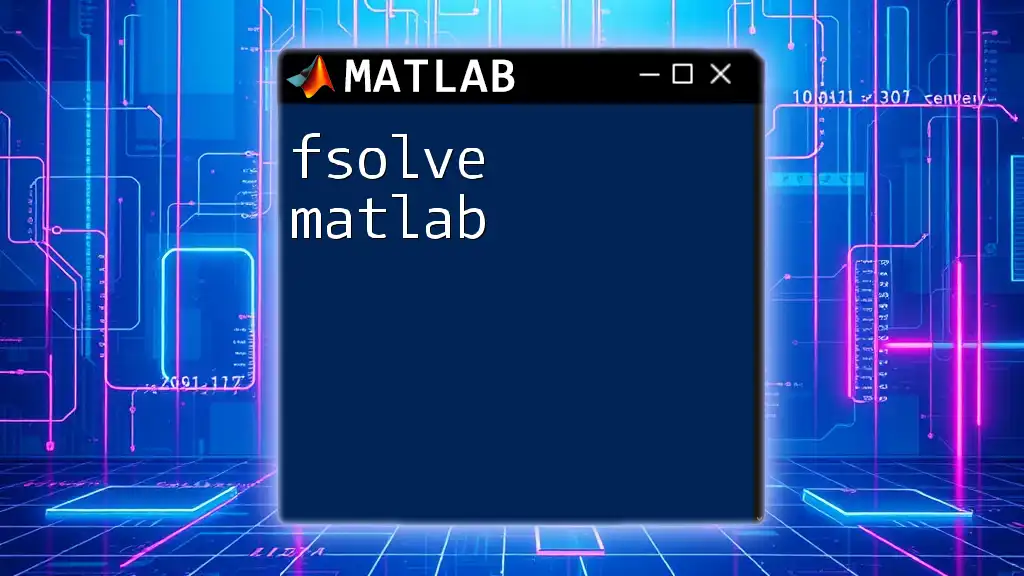
Types of Equations Solved by `solve`
Algebraic Equations
Algebraic equations can range from linear to polynomial forms. The `solve` function efficiently finds the roots of these equations.
Example: Solving a simple polynomial equation
Consider the polynomial equation x² - 4 = 0. To solve this using `solve`, you would write:
syms x
eq = x^2 - 4 == 0;
solution = solve(eq, x)
When executed, MATLAB will return the solutions x = 2 and x = -2, showcasing its ability to handle polynomial equations effectively.
Systems of Equations
Another application of the `solve` function is for systems of equations, where multiple equations share the same set of variables.
Example: Solving a system of linear equations
Let’s say we have the following two equations:
- 2x + 3y = 8
- 5x - y = 1
To solve this system, you would use:
syms x y
eq1 = 2*x + 3*y == 8;
eq2 = 5*x - y == 1;
solutions = solve([eq1, eq2], [x, y])
This will return the values of x and y that satisfy both equations, demonstrating how `solve` can efficiently handle multi-variable scenarios.
Differential Equations
The `solve` function can also be applied to ordinary differential equations (ODEs), making it versatile for various mathematical applications.
Example: Solving a simple ODE
To solve the first-order ODE dy/dt + y = 0, the following code can be employed:
syms y(t)
Dy = diff(y, t);
ode = Dy + y == 0;
sol = dsolve(ode)
Running this snippet will yield the general solution to the differential equation, elaborating the capabilities of `solve` in the context of calculus.
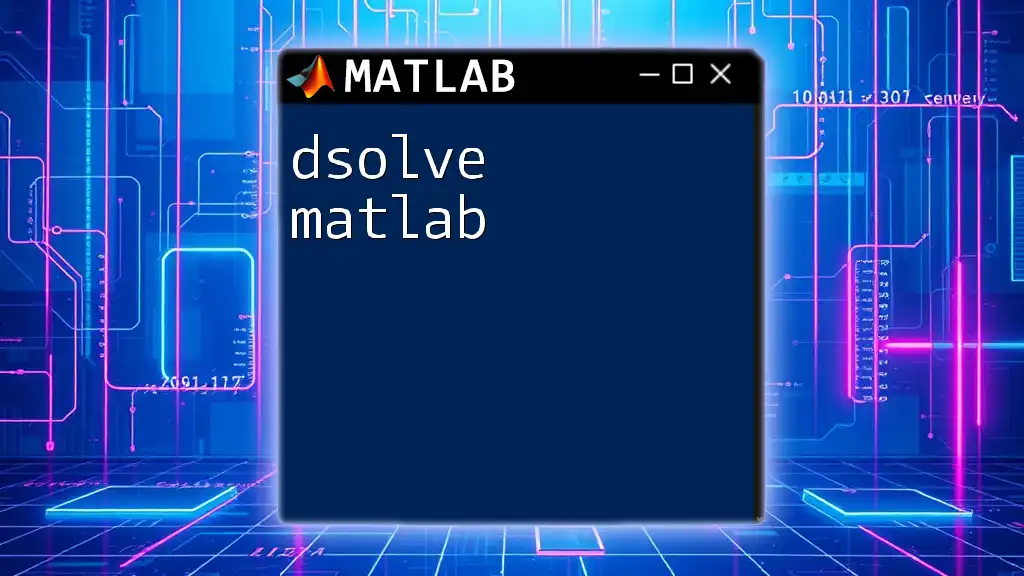
Using `vpasolve` for Numerical Solutions
When to Use `vpasolve`
While `solve` provides symbolic solutions, there are scenarios where numerical solutions are preferred or necessary—especially with non-linear or complicated equations. Here, the `vpasolve` function comes into play.
Syntax and Parameters of `vpasolve`
The syntax for using `vpasolve` is similar to `solve` but specifically geared towards obtaining a numeric answer:
numerical_solution = vpasolve(equation, variable)
This makes `vpasolve` an excellent choice for scenarios where an analytical solution may be difficult or impossible to derive.
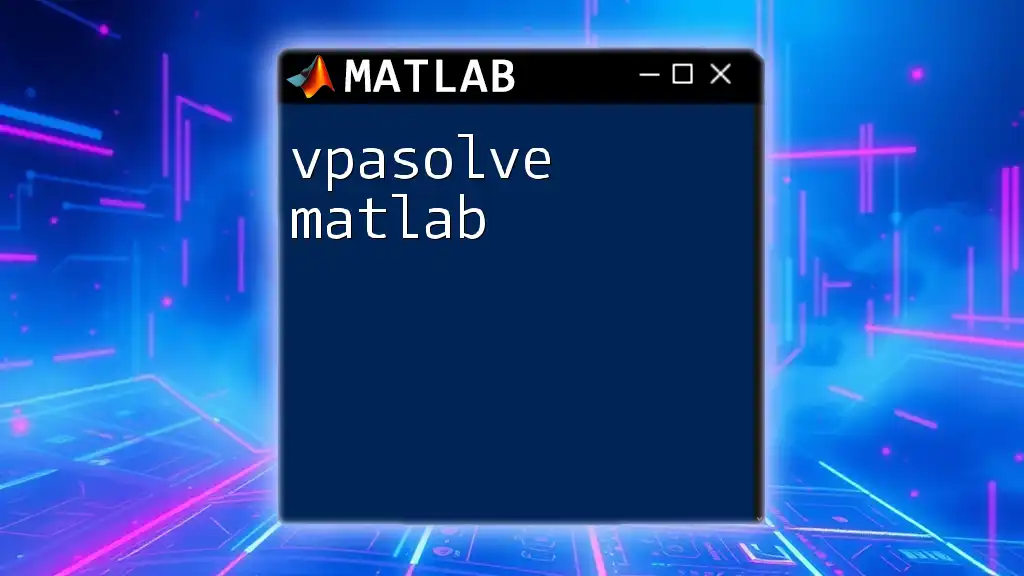
Additional Tips for Using `solve`
Working with Symbolic Variables
When using the `solve` function, it is imperative to declare your variables symbolically. This is accomplished using the `syms` command.
Example:
syms x
Using symbolic variables is crucial in enabling MATLAB to interpret and manipulate the equations correctly.
Handling Multiple Solutions
Sometimes, equations yield multiple solutions. The `solve` function is adept at managing this by returning all relevant roots.
Example: Solving a cubic equation
For the cubic equation x³ - 1 = 0:
eq = x^3 - 1 == 0;
solutions = solve(eq, x)
MATLAB will return three solutions—one real and two complex—demonstrating the function's robustness in addressing various equation types.
Plotting Solutions
To gain deeper insight into solutions, visualizing them with MATLAB's plotting functionalities can be invaluable. It allows for a graphical representation, enhancing comprehension of the solutions in context.
Example:
fplot(@(x) x^3 - 1, [-2, 2])
grid on
This command plots the function x³ - 1, intersecting the x-axis at the root x = 1.
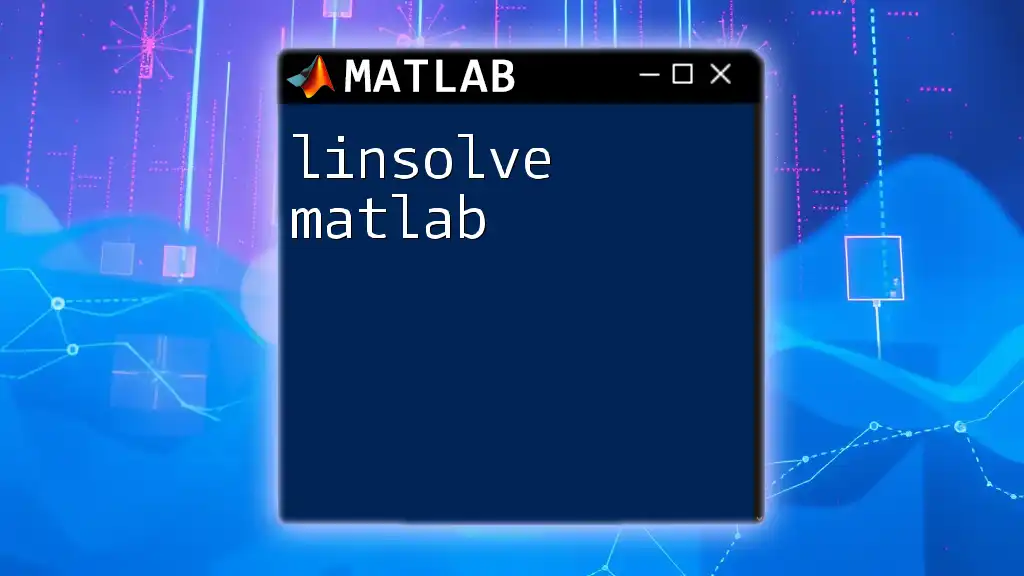
Advanced Applications
Using `solve` with Higher-Dimensional Systems
Advanced users can leverage `solve` to address higher-dimensional systems involving multiple variables and equations.
Example: Solving a system in three variables
For the following equations:
- x + y + z = 6
- x - 2y + z = 4
- 2x + y - z = 3
You can solve as follows:
syms x y z
eq1 = x + y + z == 6;
eq2 = x - 2*y + z == 4;
eq3 = 2*x + y - z == 3;
sol = solve([eq1, eq2, eq3], [x, y, z])
This will yield the solutions for x, y, and z that satisfy all three equations simultaneously, indicating how `solve` can tackle complex scenarios found in real-world applications.
Implementation in Real-World Problems
MATLAB’s `solve` function is not just an academic tool; it has real-world applications, such as resolving equilibrium conditions in engineering, optimizing models in economics, and modeling physical systems in physics. For example, solving equilibrium equations of structures can ensure safety and stability, making it a vital resource for professionals.
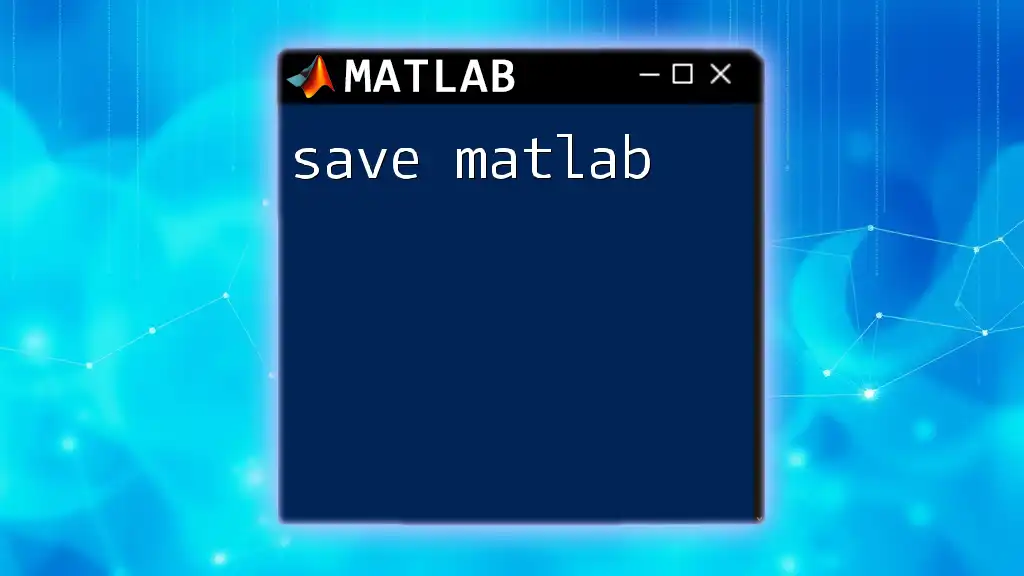
Conclusion
The `solve` function in MATLAB is an essential element for anyone looking to delve into numerical computing and mathematical problem solving. Its versatility in handling various types of equations—from simple polynomials to complex systems—makes it indispensable in both academic and practical contexts. By mastering the `solve matlab` functionality, you can significantly enhance your problem-solving capabilities. Embrace the journey of learning MATLAB, apply these concepts, and explore the extensive potential of this powerful computational tool.
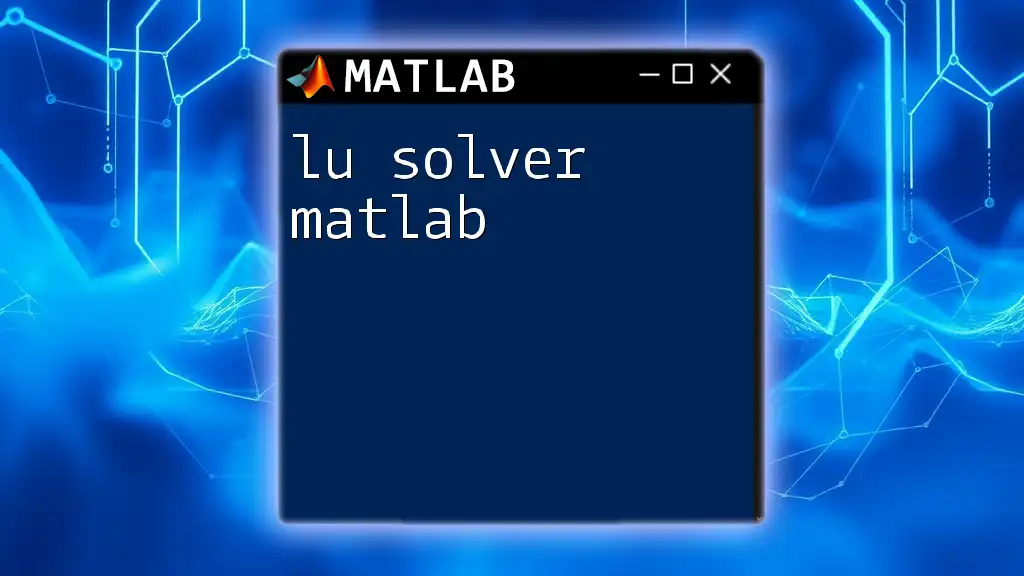
Call to Action
We encourage you to engage with MATLAB’s `solve` function and put the theory into practice with real problems. Share your experiences and challenges, whether in a classroom, at work, or if you’re coding for fun. For more in-depth workshops and personalized guidance on MATLAB commands, consider joining our upcoming sessions tailored exactly to meet your needs.
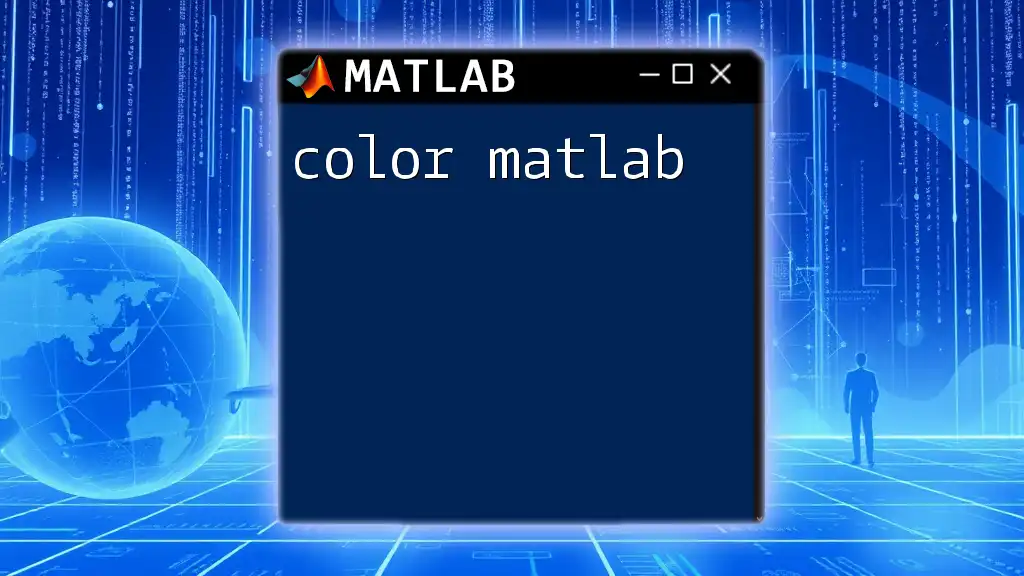
References
To further improve your understanding of the `solve` function and its applications, we suggest reviewing the MATLAB official documentation. Dive deep into the rich array of resources available to elevate your MATLAB proficiency.