`fplot` in MATLAB is a powerful function that allows you to plot the graph of a function specified by a function handle, equation, or expression over a defined interval.
Here's a simple example using `fplot`:
fplot(@sin, [0, 2*pi])
What is `fplot`?
`fplot` is a powerful function in MATLAB that allows users to visualize mathematical functions easily and intuitively over a specified interval. By simplifying the process of plotting functions, `fplot` becomes an invaluable tool for both beginners and experienced users who need quick insights into mathematical expressions.
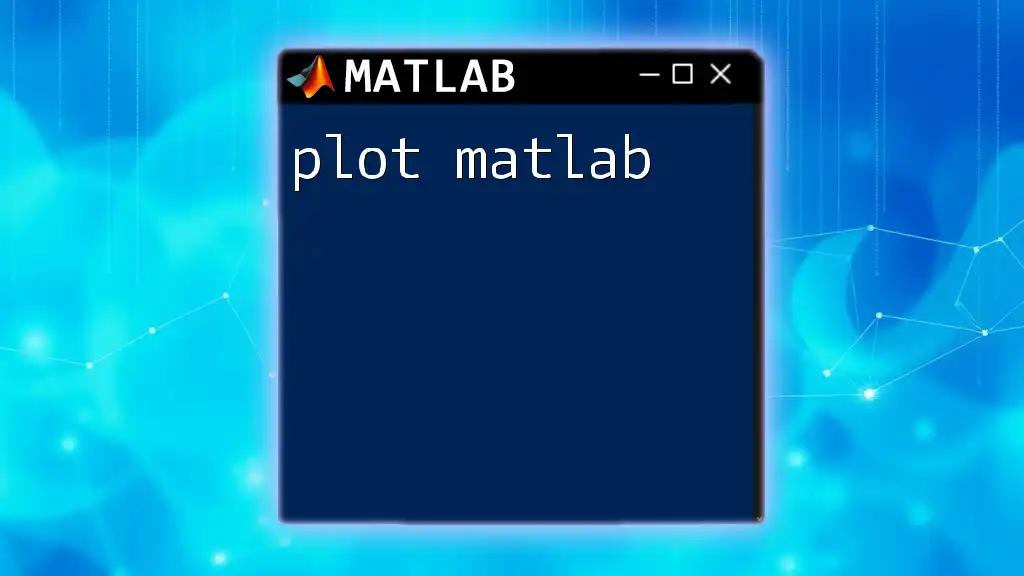
Setting Up Your MATLAB Environment
Installing MATLAB
Before you can delve into using `fplot` in MATLAB, you need to ensure you have the software installed. Visit the official MATLAB website and follow the step-by-step instructions to download and install it on your computer.
Opening MATLAB
Once installed, open MATLAB by clicking its icon. You'll be greeted by the MATLAB desktop interface, which includes the Command Window, Workspace, and Editor. Familiarizing yourself with this layout will enhance your coding efficiency.
Creating a New Script
To create a new script, navigate to the top-left corner of the MATLAB desktop and click on New Script. This action opens an editor where you can enter and save your MATLAB commands.
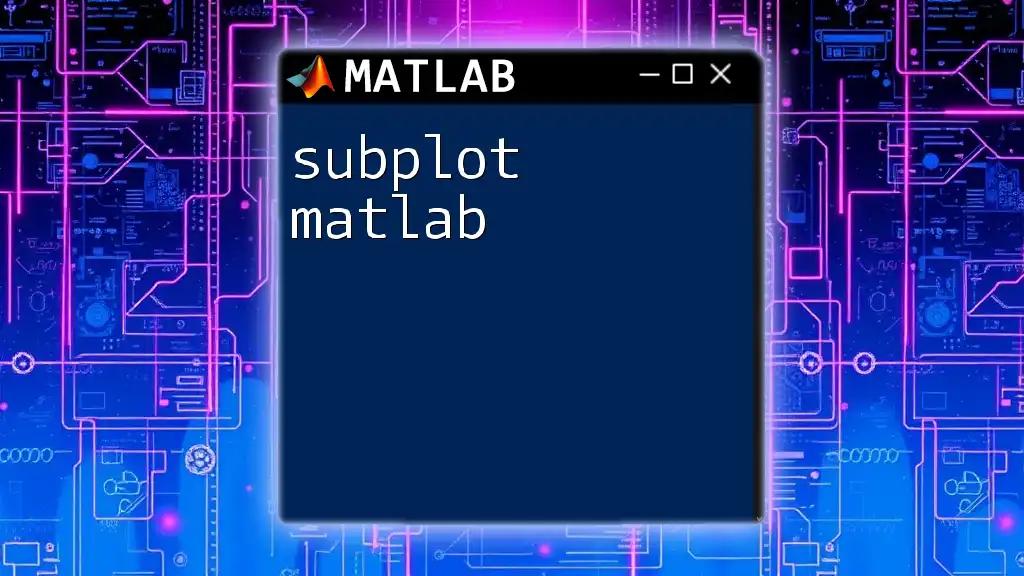
Understanding Function Plotting
Why Use Function Plotting?
Visual representation plays a crucial role in understanding complex mathematical functions. Plots help identify trends, intersections, and behaviors that may not be apparent in a purely numerical format.
Types of Functions You Can Plot
`fplot` can handle a wide range of functions, including:
- Algebraic functions (e.g., linear, quadratic)
- Trigonometric functions (e.g., sine, cosine)
- Exponential functions (e.g., \(e^x\))
- User-defined functions
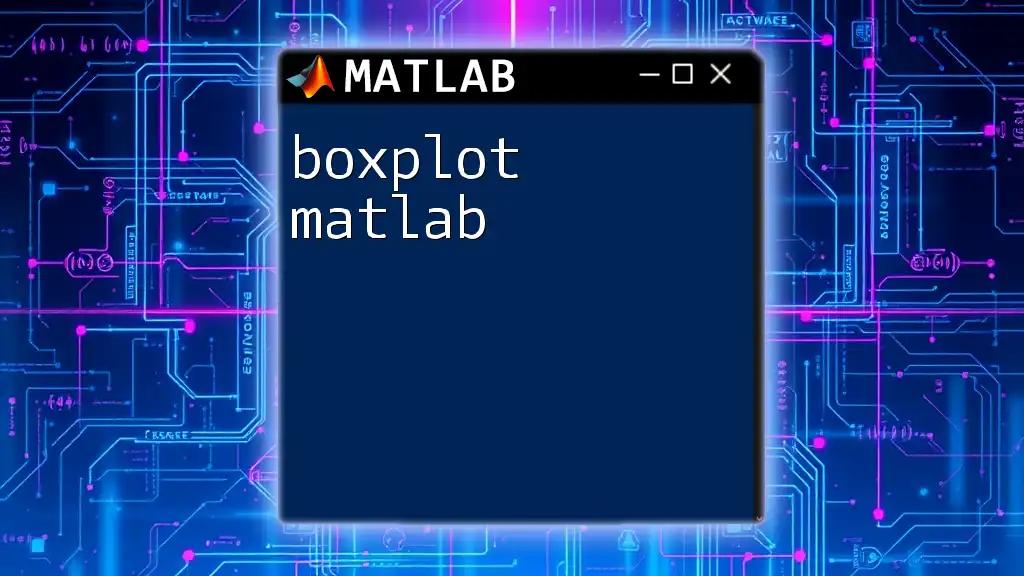
Basic Syntax of `fplot`
The general syntax of the `fplot` function is as follows:
fplot(fun, limits)
Breakdown of the Syntax
- `fun`: This parameter is the function you want to plot. You can use built-in functions or define your custom function using a function handle.
- `limits`: This parameter specifies the interval over which your function will be plotted. It accepts two values defining the lower and upper bounds.
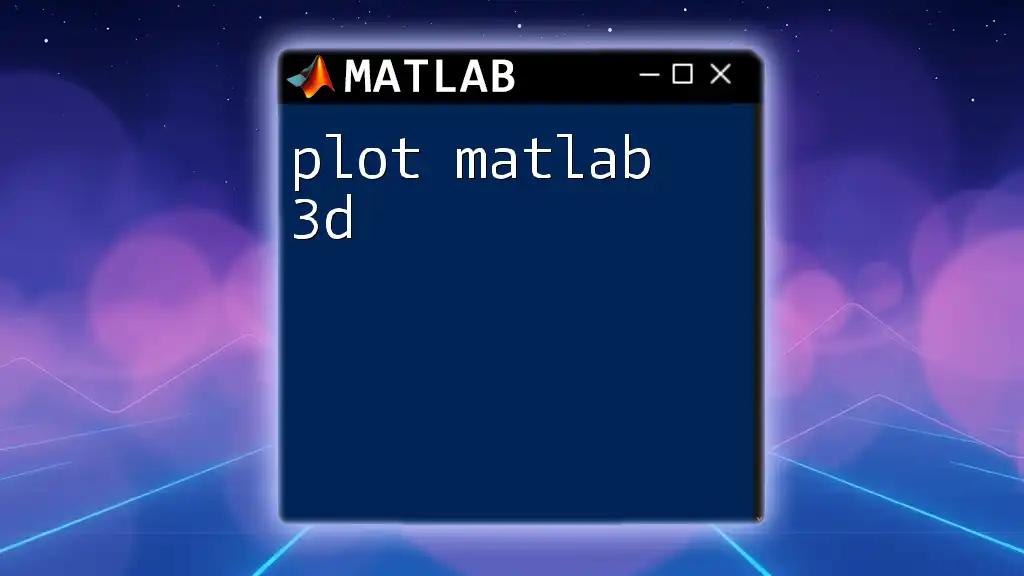
Creating Simple Plots with `fplot`
Plotting a Simple Function
To get started, let’s plot a simple linear function. The following MATLAB command can be used:
fplot(@(x) 2*x + 3, [-10, 10])
This command plots the function \(y = 2x + 3\) over the interval from -10 to 10.
Understanding the Output
Once you run the command, MATLAB generates the plot in a new figure window. The output will provide a visual representation of the linear equation, illustrating how the output \(y\) changes with varying \(x\) values.
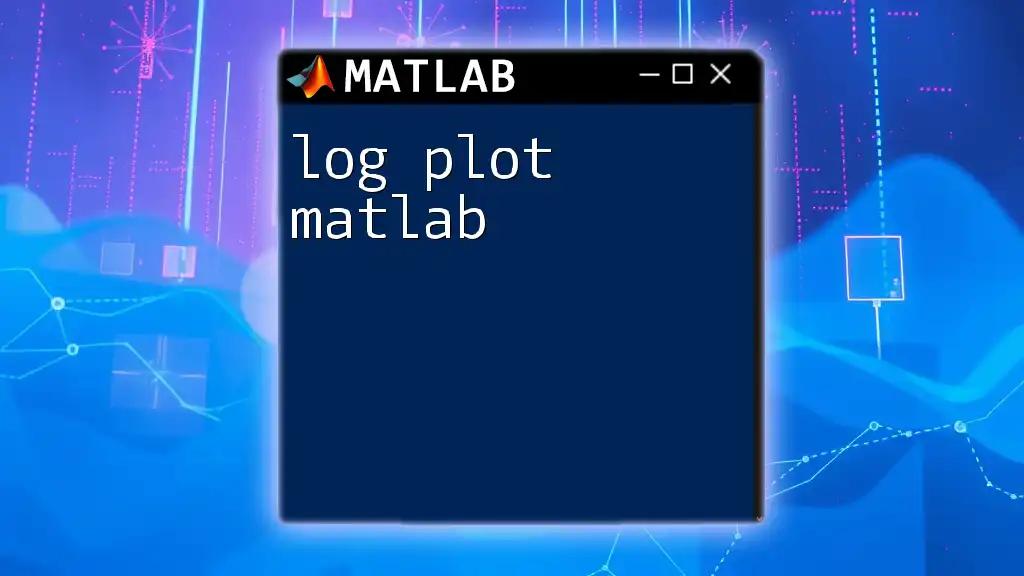
Advanced Plotting Options
Customizing the Plot
You can enhance your plots with various customization options.
Changing Line Styles and Colors
To change the appearance of the plot, utilize properties like `LineStyle` and `Color`. For example:
fplot(@(x) sin(x), [-2*pi, 2*pi], 'LineWidth', 2, 'Color', 'r')
This command plots the sine function with a thick red line.
Adding Titles and Labels
To make your plot informative, add titles and axis labels. Here’s an example:
title('Sine Function');
xlabel('x values');
ylabel('sin(x)');
Adding these elements provides context and clarity for anyone viewing the graph.
Plotting Multiple Functions
`fplot` allows you to display multiple functions in a single graph, which is helpful for comparison. Here’s how you can plot both the sine and cosine functions together:
fplot(@(x) sin(x), [-2*pi, 2*pi]);
hold on;
fplot(@(x) cos(x), [-2*pi, 2*pi]);
legend('sin(x)', 'cos(x)');
By utilizing the `hold on` command, you can overlay multiple plots on the same figure. The legend helps distinguish between the different functions.
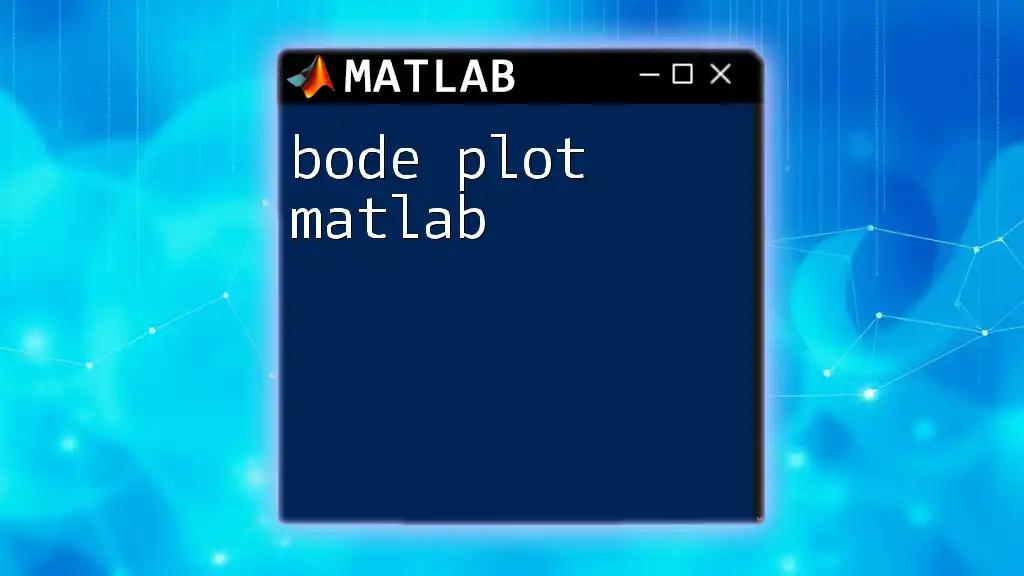
Working with Function Handles
What are Function Handles?
Function handles in MATLAB serve as a reference to functions, allowing them to be passed as arguments to other functions, such as `fplot`. This flexibility facilitates the plotting of both built-in and user-defined functions.
Creating Custom Functions
You can define and plot your functions with ease. For example, consider the following quadratic function:
myFunc = @(x) x.^2 - x;
fplot(myFunc, [-2, 3]);
This command defines a custom function and plots it over the specified range, showcasing your ability to visualize more complex mathematical expressions.
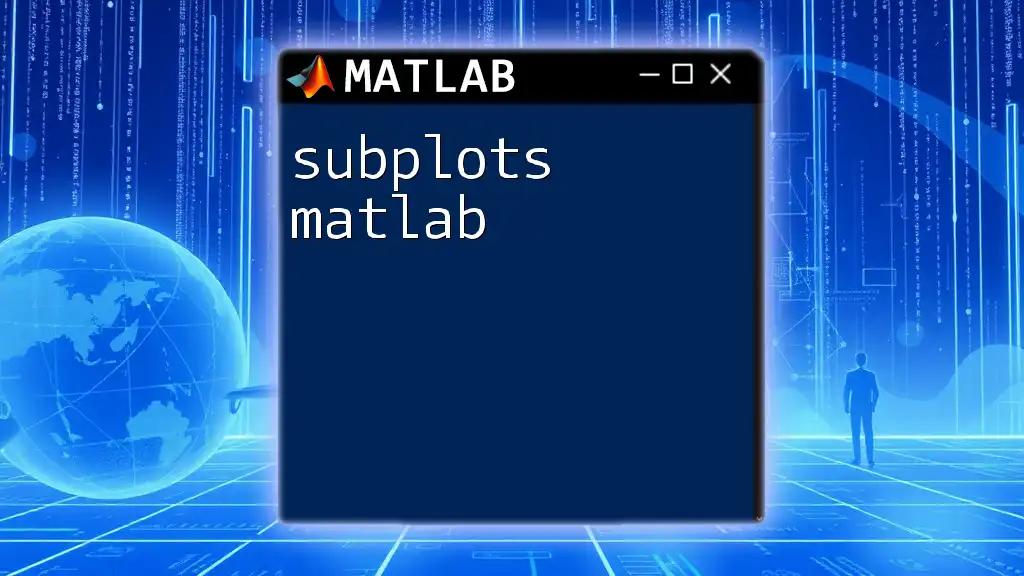
Advanced Customization Techniques
Setting Axes Limits
Controlling the visual output of your plots is important for clarity. You can set the limits of your axes using the `axis` command. For example:
axis([-2, 2, -1, 1]);
This instruction confines the x-axis from -2 to 2 and the y-axis from -1 to 1.
Adding Gridlines
To improve the readability of your plots, you can enable gridlines by using the command:
grid on;
Gridlines help in estimating values and identifying trends within the plot quickly.
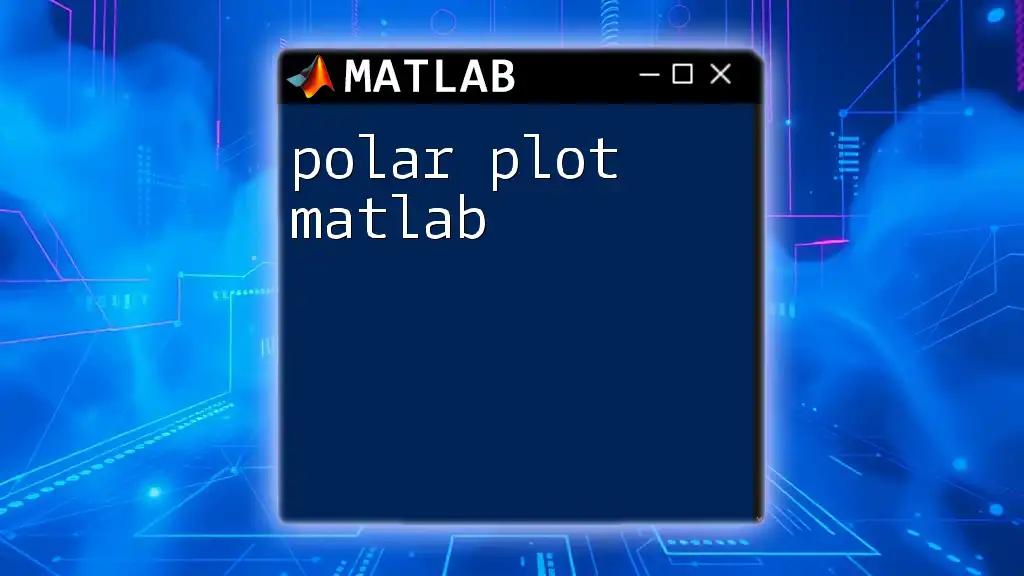
Tips and Tricks for Effective Plotting
Avoiding Common Mistakes
While working with `fplot`, some common pitfalls to avoid include:
- Forgetting to define the limits, which may cause unexpected plots.
- Not using function handles correctly, resulting in errors.
Best Practices
To present functions effectively:
- Ensure your axis labels and titles are descriptive.
- Use contrasting colors for different functions to enhance visibility.
- Customize line styles appropriately to increase clarity.
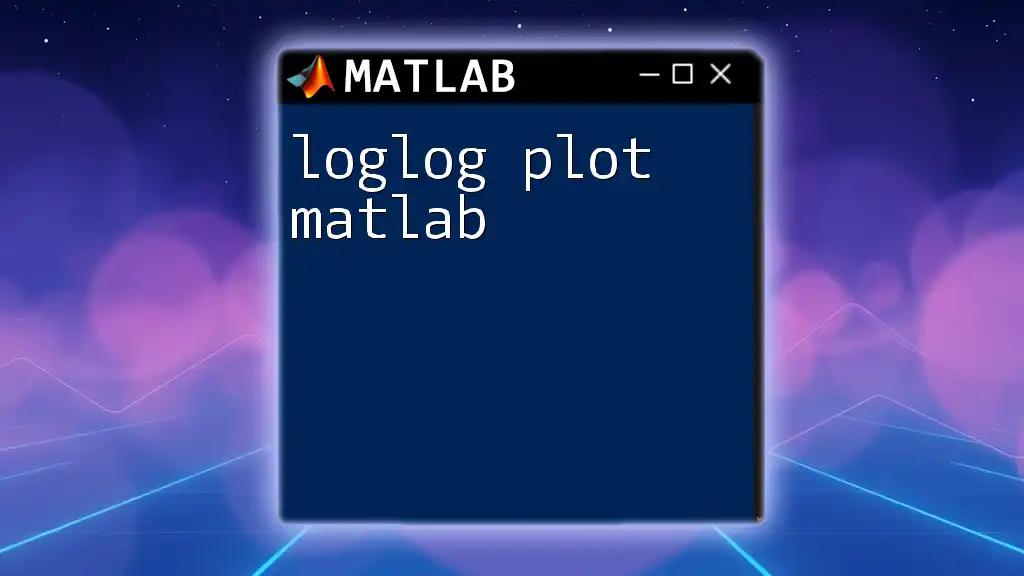
Real-World Applications of `fplot`
`fplot` is not just a tool for academic purposes; it has practical applications in various fields. For instance, engineers often use function plotting to visualize stress-strain relationships, while physicists might plot trajectories of projectiles. These real-world scenarios highlight the utility of `fplot` in transforming abstract mathematical concepts into comprehensible visual forms.

Conclusion
In this guide, we explored the ins and outs of using `fplot` in MATLAB. From basic syntax to advanced customizations, the `fplot` function offers you powerful tools for visualizing functions effectively. Don't hesitate to experiment with different functions and plotting styles to broaden your understanding and skills in MATLAB.
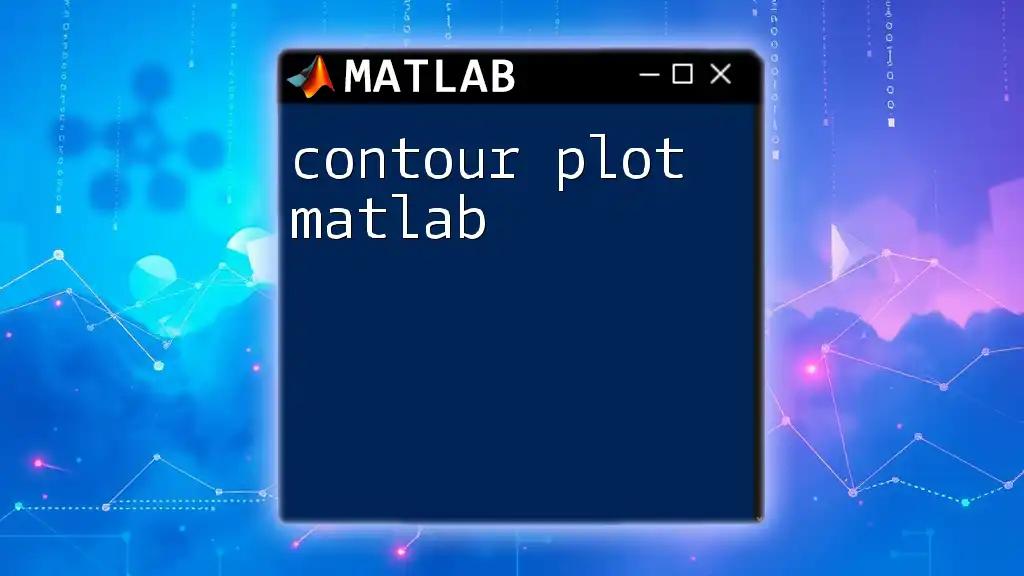
Additional Resources
For those looking to deepen their knowledge, consider exploring MATLAB documentation, online tutorials, and forums such as MATLAB Central. Engaging with the community can provide valuable insights, tips, and support as you continue your journey with MATLAB and `fplot`.