In MATLAB, you can specify the color of a line in plots using RGB triplets, predefined color strings, or hexadecimal color codes, allowing for customized visual representations of your data.
Here’s an example code snippet:
x = 0:0.1:10;
y = sin(x);
plot(x, y, 'Color', [0 0.5 1]); % RGB triplet for a light blue line
Understanding Line Colors in MATLAB
What is Line Color?
Line color in MATLAB plays a critical role in data visualization. It is not just a matter of aesthetic preferences; the choice of line color can significantly affect how viewers interpret the data presented in a plot. Different colors can highlight trends, differentiate data series, and draw attention to specific data points. Effective use of color can improve readability and clarity in visual representations of data.
The RGB Color Model
MATLAB utilizes the RGB color model to define colors for lines in plots. This model represents colors as combinations of three primary colors: Red, Green, and Blue. Each color component can have a value ranging from 0 to 1, where 0 means no contribution of that color and 1 means full intensity. By mixing different intensities of red, green, and blue, you can create a wide spectrum of colors. For example, the RGB triplet `[1, 0, 0]` corresponds to pure red, while `[0, 1, 0]` represents pure green.
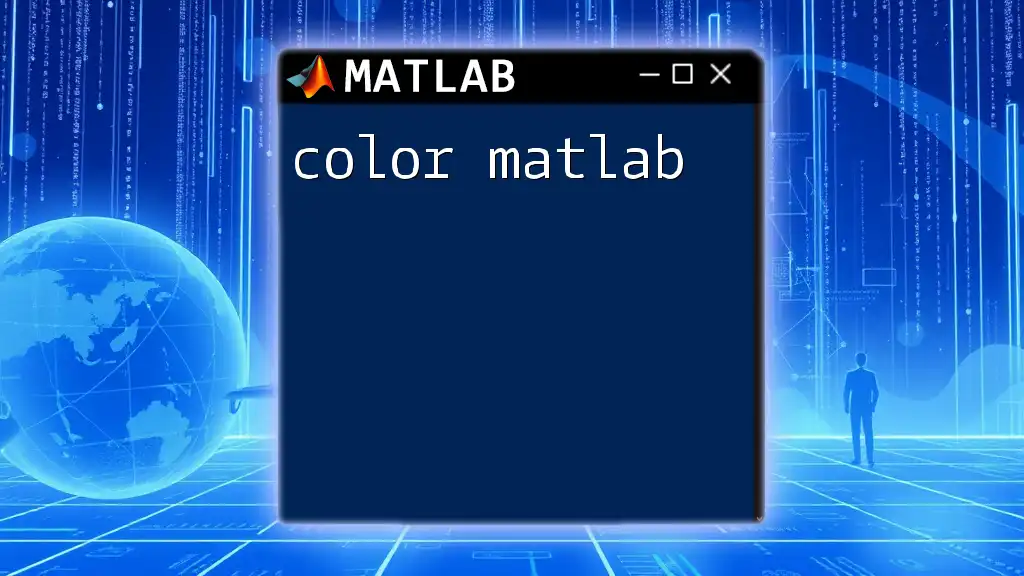
Setting Line Colors in MATLAB Plots
Default Line Colors in MATLAB
By default, MATLAB has a predefined set of colors used for plotting data. When you create a new plot, MATLAB automatically cycles through these default colors for each line. This can be seen in a simple example where two lines are plotted without specifying colors:
x = 1:10;
y1 = rand(1, 10);
y2 = rand(1, 10);
plot(x, y1, x, y2);
title('Default Line Colors Example');
In this example, you will see two lines in different colors, which MATLAB chooses from its default color order.
Customizing Line Colors
Using Color Names
MATLAB also allows you to use named colors for customization. Some common colors include 'r' for red, 'g' for green, 'b' for blue, and 'k' for black. To set the color of a line using name:
plot(x, y1, 'r', 'LineWidth', 2); % Red line
title('Plot with Named Color');
This is a straightforward way to apply color to your lines, making it easy for anyone familiar with basic color terminology to create visually distinct plots.
Using RGB Triplets
For more granular control over your plot's appearance, you can define custom colors using RGB triplets. This method allows you to specify any color by providing the three primary color components. Here's how you can do it:
plot(x, y1, 'Color', [0.2, 0.6, 0.8]); % Custom RGB Color
title('Plot with RGB Triplet');
In this example, the specified RGB triplet creates a unique shade of blue.
Hexadecimal Color Codes
In addition to RGB triplets, you can also use hexadecimal color codes, which are popular in web design and provide a convenient way to specify colors as well. For example, using a hex code in MATLAB can be done as follows:
plot(x, y1, 'Color', '#FF5733'); % Hex Color Example
title('Plot with Hexadecimal Color');
Here, `#FF5733` represents a vibrant shade of orange. The flexibility of using hex codes expands your ability to customize visual elements in your plots even further.
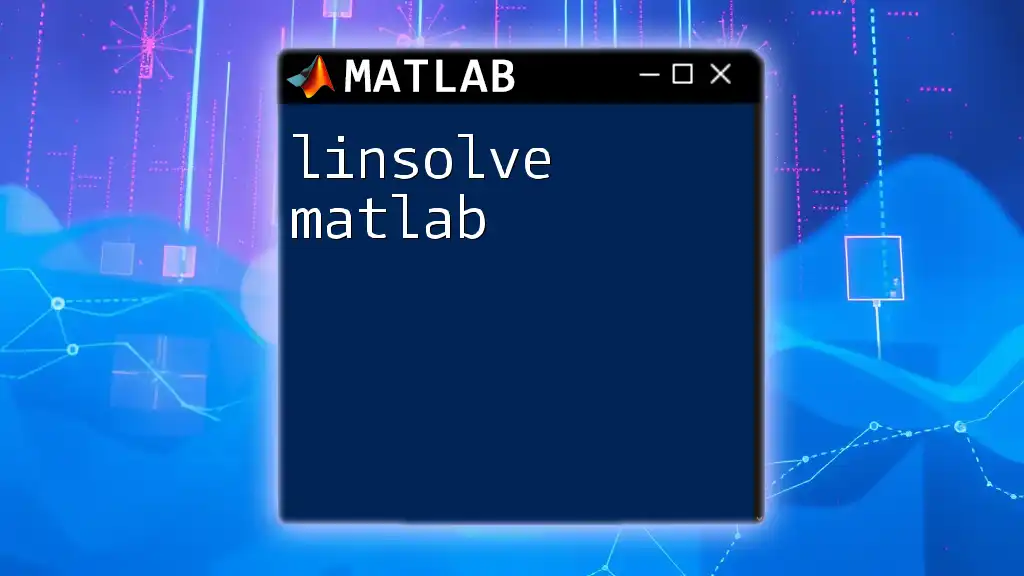
Advanced Techniques for Line Color Customization
Using Color Data to Represent Values
One of the more advanced techniques involves using color data to represent specific values in your dataset. This can be particularly useful when you want to visualize a trend or highlight ranges within a dataset. You can achieve this by using colormaps in MATLAB. Here’s an example where we use the jet colormap to create a set of colors based on data values:
c = colormap(jet(10)); % Creating a colormap
for i = 1:10
plot(x, y1*i, 'Color', c(i,:), 'LineWidth', 2);
hold on;
end
title('Dynamic Line Color Based on Values');
In this code, the `jet` function generates a range of colors, and each line in the loop receives a distinct color based on its index.
Creating Multiple Lines with Different Colors
When plotting multiple lines, you may want to assign different colors to each line systematically. Leveraging the built-in `lines` function can help you generate a distinct set of colors easily. Here’s an example:
colors = lines(5); % MATLAB built-in color set
for i = 1:5
plot(x, rand(1, 10)*i, 'Color', colors(i,:), 'LineWidth', 2);
hold on;
end
title('Multiple Lines with Different Colors');
This example demonstrates how to efficiently use loops for plotting multiple lines, allowing for clean differentiation among them while maintaining visual harmony.
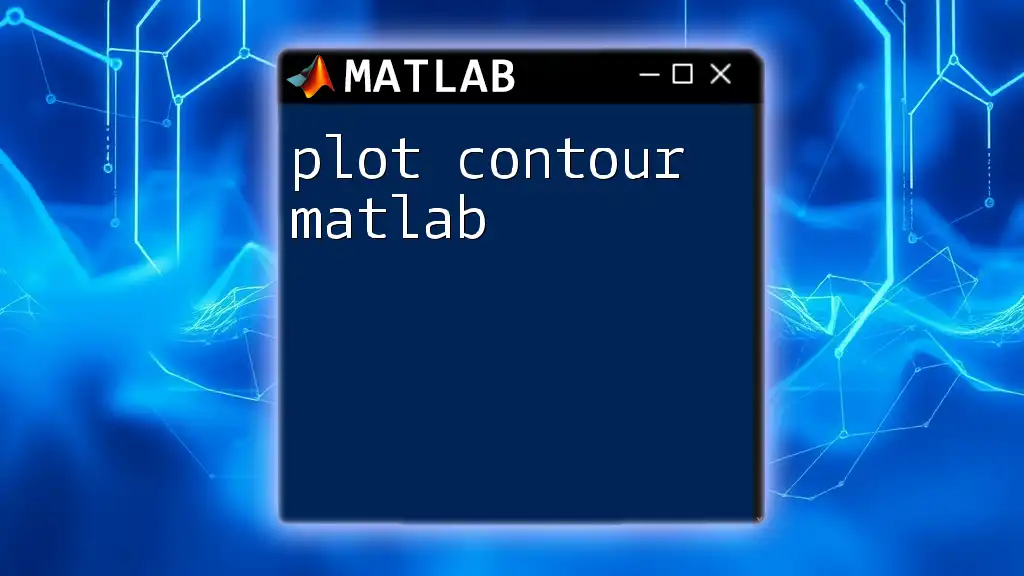
Conclusion
In this article, we've explored the versatility of line colour in MATLAB. By understanding default color settings, customizing colors with named options, RGB triplets, and hexadecimal codes, you are equipped to improve your data visualizations. Advanced techniques such as using color data and systematically assigning colors allow you to present your data clearly and effectively. Experimentation is key, so feel free to explore different color combinations and enhance your MATLAB plotting skills!
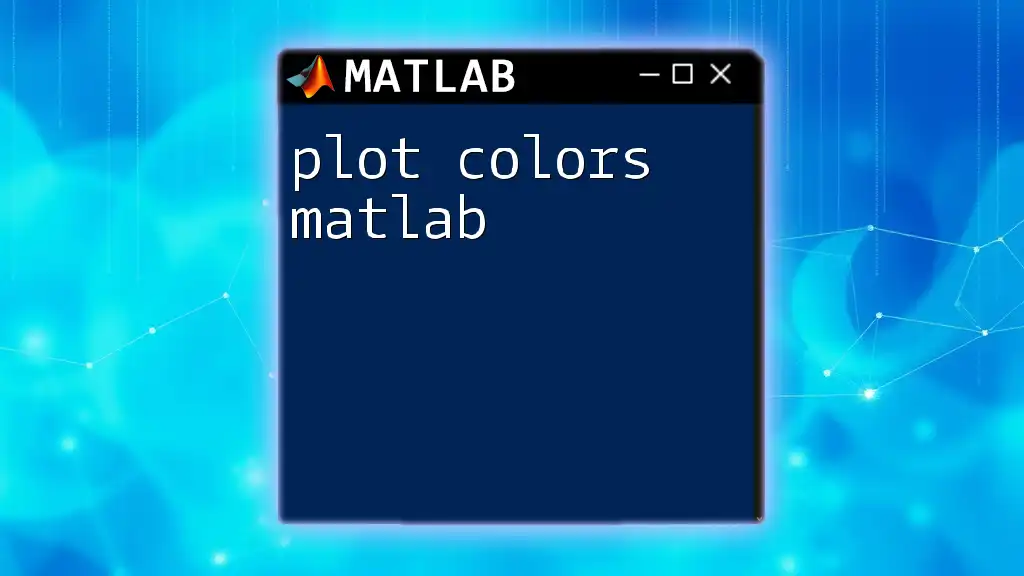
Additional Resources
Further Reading
For those looking to deepen their knowledge of MATLAB, consider exploring books or online courses specifically tailored to visual data representation.
Useful MATLAB Commands
A quick reference for commands discussed:
- `plot()`: Basic plotting function for creating graphs.
- `colormap()`: Function to define a range of colors for visualization.
- `lines()`: Generates a distinct set of colors for multiple plots.
Join Our Learning Community
Interested in mastering more MATLAB commands and data visualization techniques? Join us for comprehensive tutorials and resources tailored just for you!