The `parfor` command in MATLAB enables parallel processing by allowing the execution of loop iterations simultaneously, enhancing performance for computationally intensive tasks.
Here’s a simple code snippet demonstrating the usage of `parfor`:
% Example of using parfor to compute squares in parallel
n = 1:10;
results = zeros(size(n));
parfor i = 1:length(n)
results(i) = n(i)^2; % Compute the square of each element in parallel
end
disp(results);
Understanding Parallel Computing
What is Parallel Computing?
Parallel computing is a method in which multiple calculations or processes are carried out simultaneously to improve performance and efficiency. While traditional serial computing executes tasks one after another, parallel computing distributes tasks across multiple processors or cores, allowing for simultaneous execution. This difference can lead to significant reductions in computation time, particularly for large-scale problems or datasets.
Benefits of Parallel Computing in MATLAB
The Parallel Computing Toolbox in MATLAB is designed to harness the power of parallel computing, enabling users to speed up the execution of their code significantly. By efficiently utilizing available computational resources, MATLAB’s parallel computing features allow for:
- Faster Computations: The ability to perform tasks concurrently means that operations can be completed in a fraction of the time.
- Efficient Resource Use: Multi-core processors and clusters can be fully utilized, minimizing idle computational power.
- Handling Large Datasets: Advanced analysis techniques and simulations that were previously infeasible due to time constraints can now be efficiently tackled with parallel computing.
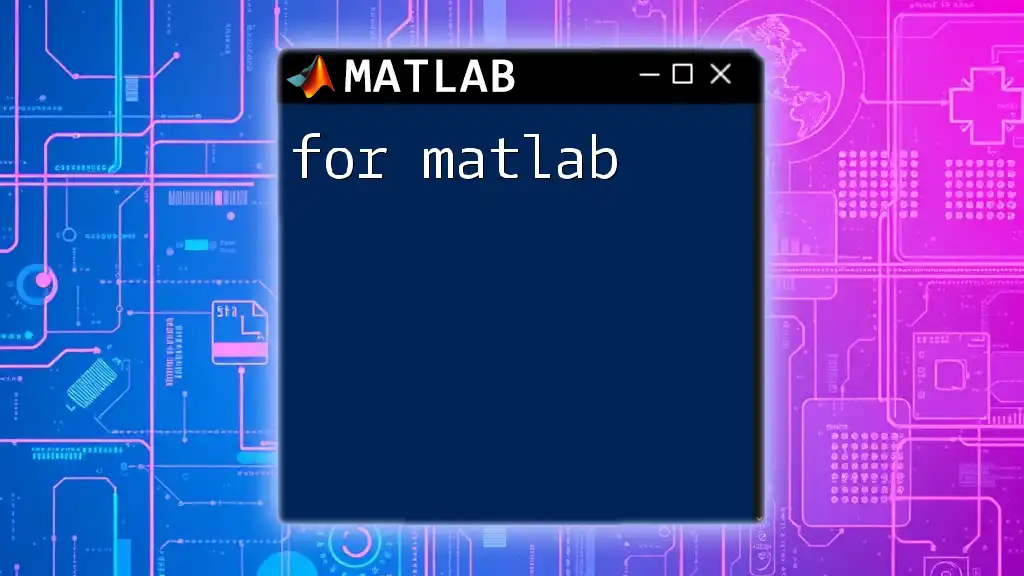
Getting Started with `parfor`
What is `parfor`?
`parfor`, short for parallel for, is a variant of the traditional `for` loop in MATLAB that enables the parallel execution of loop iterations. Unlike a regular `for` loop, which executes each iteration in sequence, `parfor` distributes iterations to different workers in the MATLAB pool, allowing them to run concurrently. This structure can lead to substantial improvements in performance.
Setting Up the MATLAB Environment
Before utilizing `parfor`, you must ensure that you have the Parallel Computing Toolbox installed. You can check if it is available by using the command:
ver
Review the output to see if "Parallel Computing Toolbox" is among the listed products. If applicable, verify that your system also supports GPU calculations by checking your hardware and software configuration.
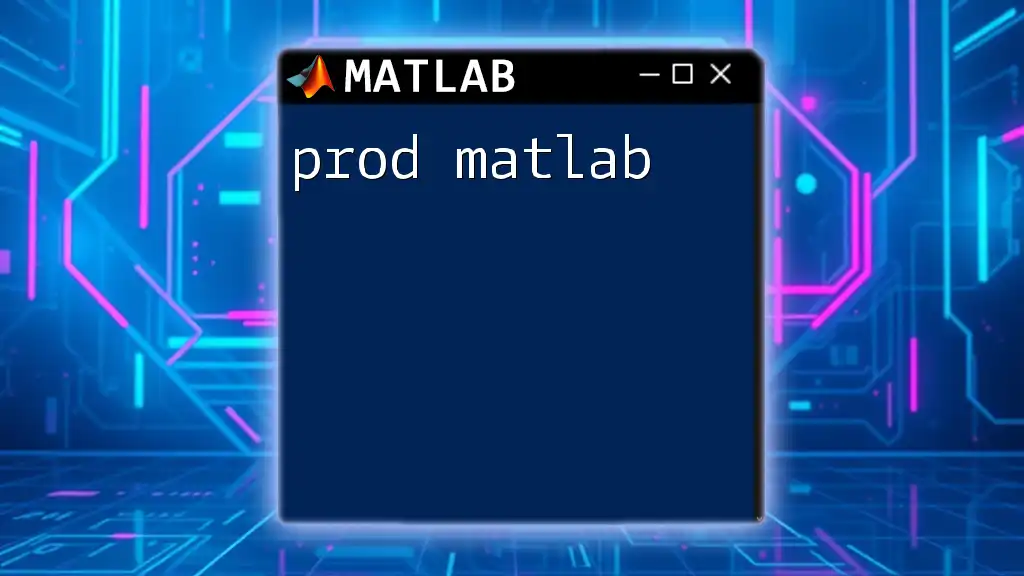
Syntax of `parfor`
Basic Syntax Structure
The basic syntax of a `parfor` loop is straightforward:
parfor idx = 1:N
% Your code here
end
In this structure, `idx` is the index variable that iterates from 1 to N. Each loop iteration is assigned to different workers, enabling concurrent execution.
Important Syntax Characteristics
When utilizing `parfor`, several rules must be followed regarding variable usage:
- Sliced Variables: For variables that can be sliced into segments, each worker gets its portion for processing.
- Reduction Variables: These variables accumulate values, such as sums, across all iterations.
- Temporary Variables: These are used solely within the loop, ensuring that they do not affect other iterations.
Understanding how to manage these variable types is crucial for the effective use of `parfor`.
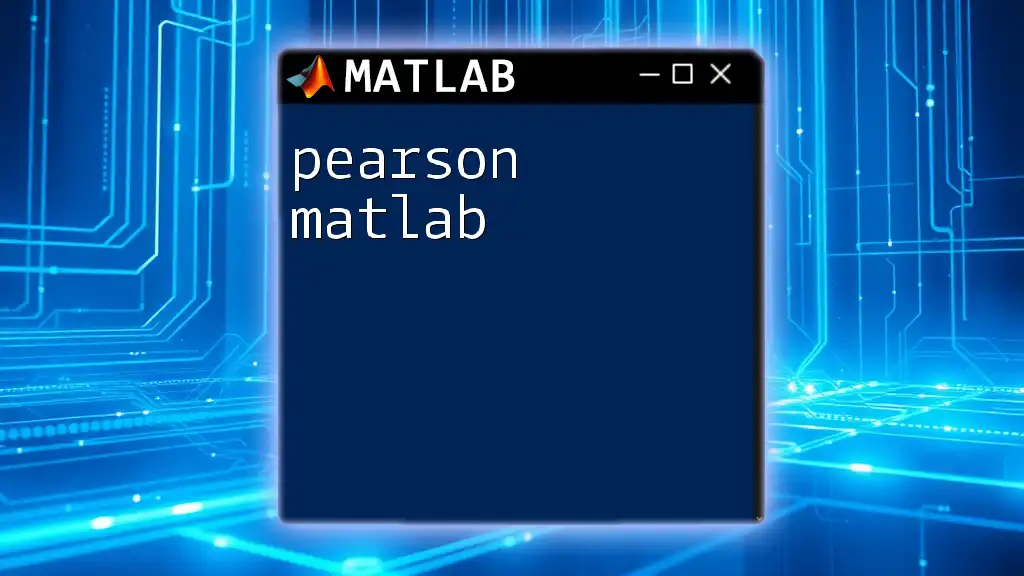
Key Features of `parfor`
Automatic Load Balancing
One significant advantage of `parfor` is its ability to automatically balance the workload among available workers. MATLAB distributes iterations based on each worker's current load, thus optimizing the overall execution time. In scenarios where iterations require different amounts of time, this feature improves performance by ensuring that no worker remains idle.
Reduction Variables
Reduction variables are a special type of variable that aggregates values from all iterations of the loop. When using them, you need to preallocate these variables before the loop begins. For example:
totalSum = 0;
parfor idx = 1:N
totalSum = totalSum + data(idx);
end
In this example, `totalSum` will hold the total of all elements in `data`. Being mindful of how reduction variables work is essential to avoid incorrect results.
Sliced Variables
Sliced variables allow for each worker to process distinct segments of an array or matrix. This means that if an array `A` is divided across iterations, each worker can manage its respective slice independently.
Temporary Variables
Temporary variables are entirely scoped within the `parfor` loop. They help compute values without affecting the global scope of other iterations. This feature is crucial to maintain data integrity and prevent conflicts.
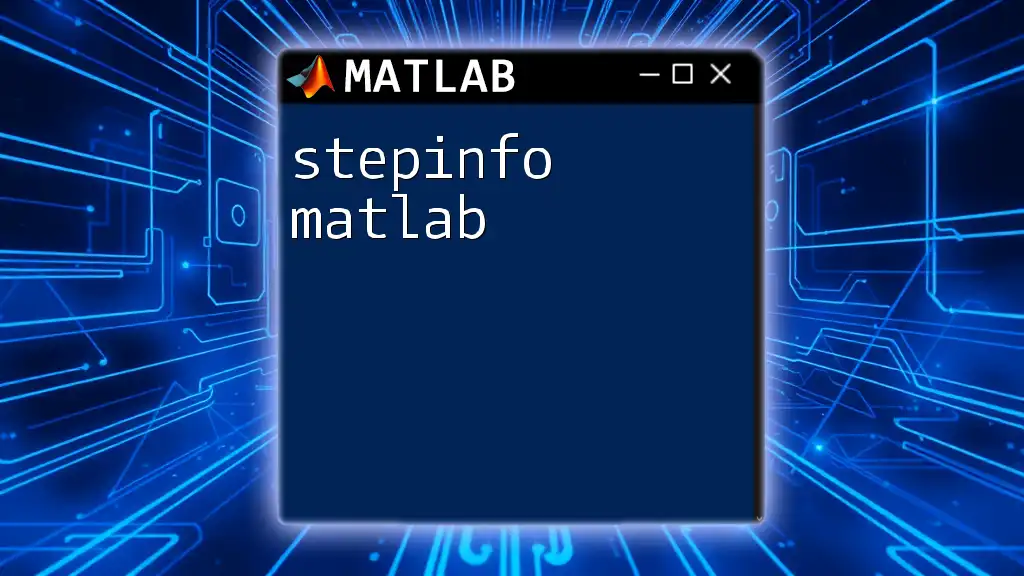
How to Use `parfor` Effectively
Best Practices
To utilize `parfor` effectively, consider these best practices:
- Identify Suitable Loops: Not all loops should be parallelized. Focus on loops that consume significant computation time and can run independently.
- Balance the Workload: Achieve an even distribution of iterations across workers to optimize performance.
Common Pitfalls
When using `parfor`, be cautious of common issues such as variable dependencies. If loop iterations depend on the same variable changes, it may cause race conditions, leading to incorrect outputs. Understanding how to manage variable usage effectively can mitigate these risks.
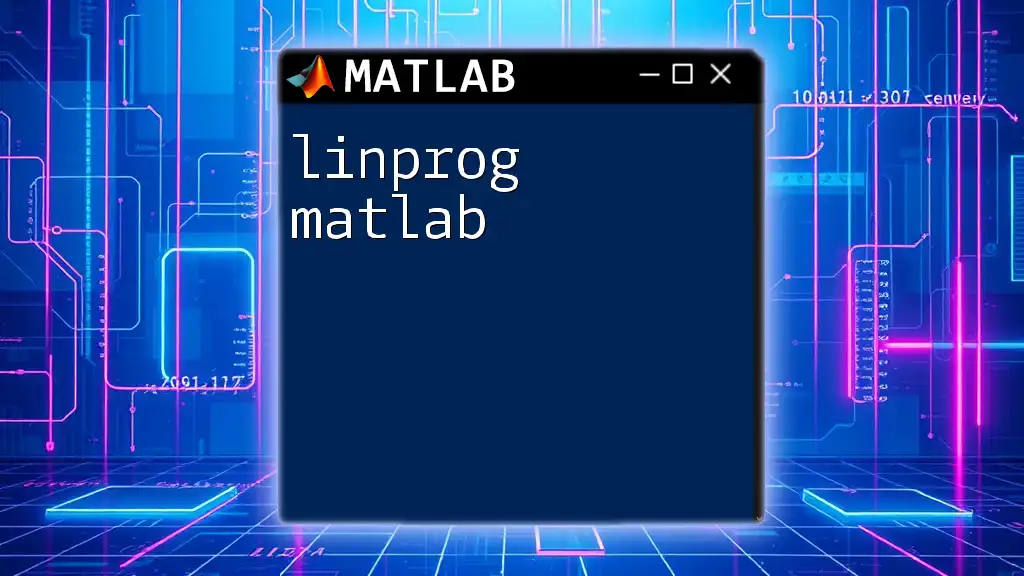
Advanced Techniques with `parfor`
Nested `parfor` Loops
Using nested `parfor` loops can increase complexity and is generally discouraged. However, in specific situations where each loop can be executed independently, it may be appropriate. For example:
parfor i = 1:M
parfor j = 1:N
% Your code for processing
end
end
Ensure that each nested loop also adheres to the rules governing variable use.
Using `parfor` with Functions
You can define and call functions within a `parfor` loop. This modular approach enhances code readability and organization. For instance:
function result = compute(data)
result = data * 2; % Example computation
end
parfor idx = 1:N
output(idx) = compute(data(idx));
end
This example demonstrates how `parfor` can call a separate function while parallelizing execution.
Parallel Pool Management
Managing your parallel pool is crucial for optimal performance. You can initiate and adjust the parallel pool size with the following commands:
myPool = parpool(numWorkers);
This code snippet allows you to set the number of workers based on your computational needs.
Troubleshooting `parfor` Issues
When encountering issues with `parfor`, pay attention to error messages related to variable usage. Often, these errors arise from improper variable management within the loop. Familiarizing yourself with MATLAB's debugging tools will aid in resolving issues promptly.
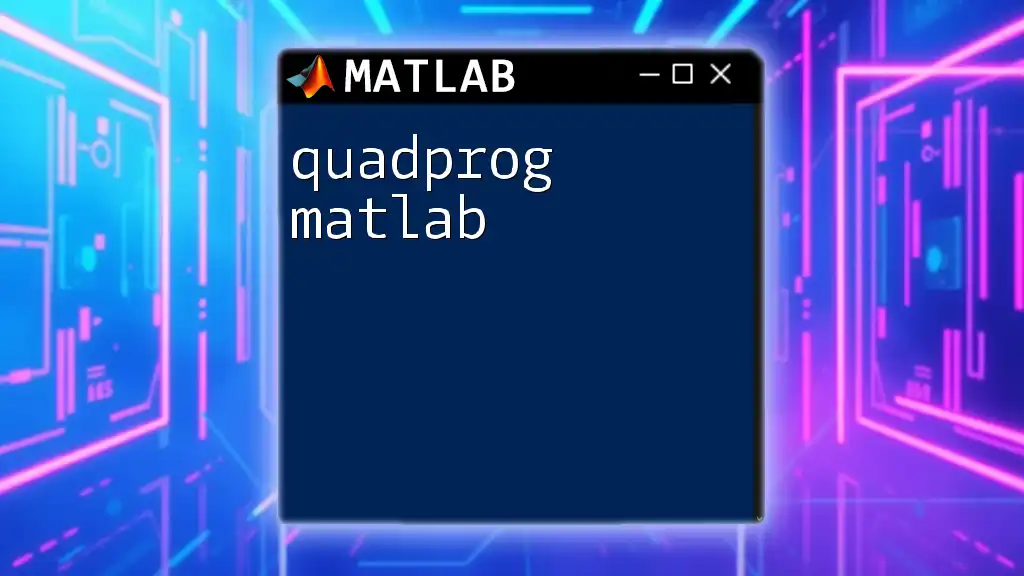
Performance Considerations
Benchmarking `parfor` Performance
To measure performance improvements with `parfor`, you can compare execution times against regular `for` loops. For example:
tic;
for idx = 1:N
% Your code
end
elapsedTimeFor = toc;
tic;
parfor idx = 1:N
% Your code
end
elapsedTimeParfor = toc;
This setup provides a clear picture of how much time you save by using `parfor`.
Factors Affecting Performance
Several factors can influence the effectiveness of `parfor`:
- Overhead Costs: There is an initial overhead associated with setting up parallelism. For small datasets or quick computations, this overhead may negate any performance benefits.
- Problem Scale: The larger the dataset or more complex the operations in each iteration, the more likely you are to see substantial benefits from parallelization.
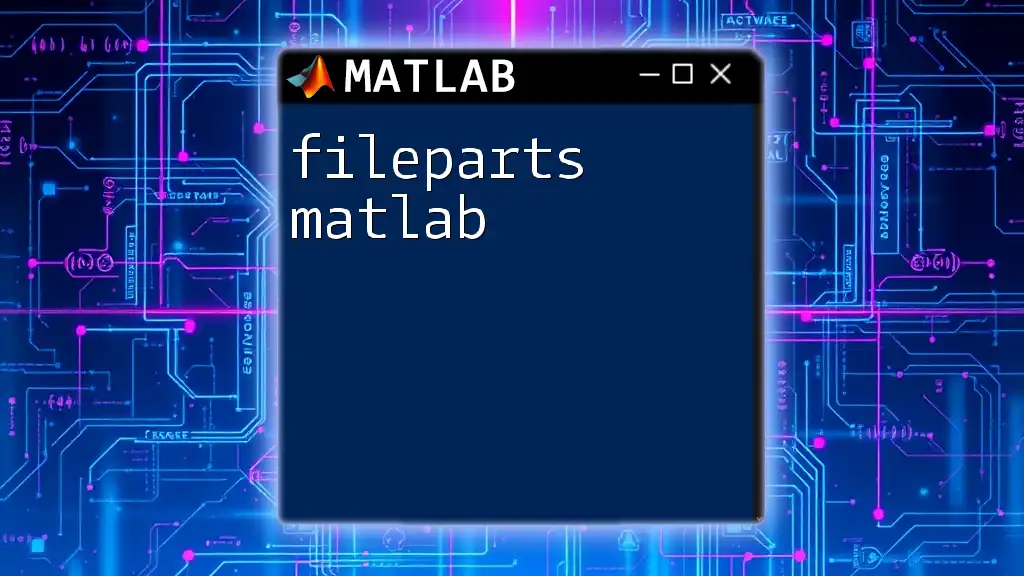
Real-world Applications of `parfor`
Use Cases in Various Fields
`parfor` has proven valuable across various industries, including:
- Data Analysis: Speed up large-scale data analytics tasks.
- Simulations: Perform Monte Carlo simulations more efficiently.
- Image Processing: Handle substantial image datasets for processing and analysis.
Case Study
Consider a case study where a data scientist is analyzing a large dataset for machine learning model training. By using `parfor`, they can effectively preprocess the data in parallel, reducing the overall time from several hours to mere minutes. This approach allowed the data scientist to iterate more quickly on model tuning, ultimately accelerating the project’s success.
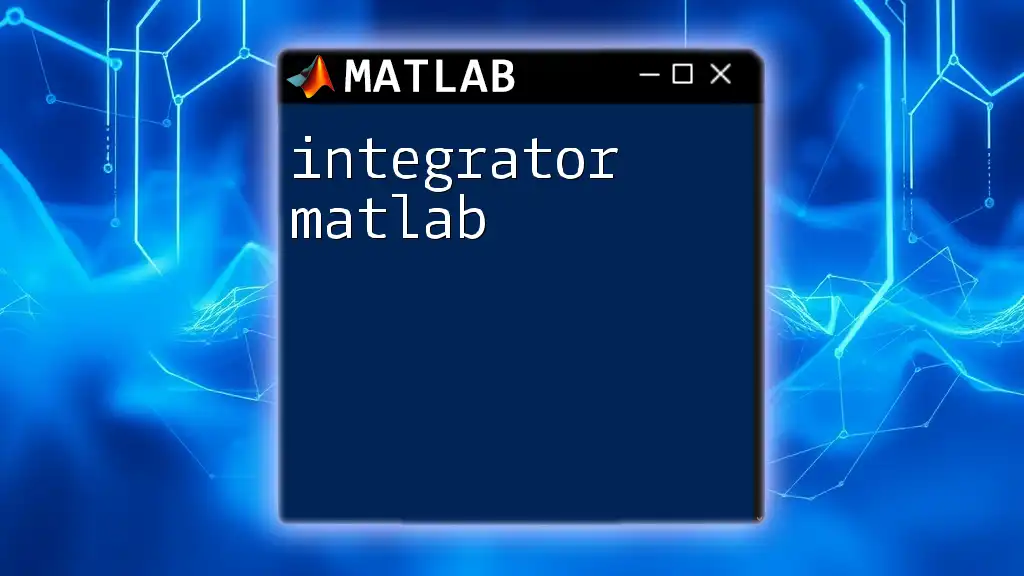
Conclusion
The `parfor matlab` function is a powerful tool for anyone looking to enhance the performance of their MATLAB code. It empowers users to parallelize their loops easily and efficiently, significantly cutting down computation times for large-scale data processing and simulations. By adopting best practices and understanding its nuances, you can take full advantage of parallel computing in MATLAB to drive your projects to success.
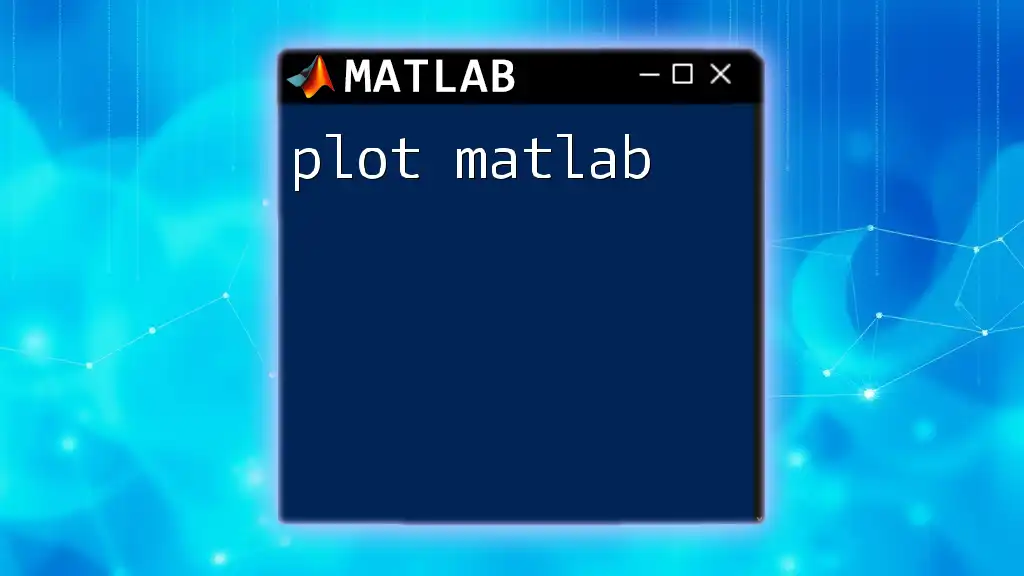
Additional Resources
Suggested Reading
Exploring MATLAB's official documentation and specialized literature on parallel computing can deepen your understanding of advanced concepts.
Online Communities and Forums
Participating in forums such as MATLAB Central allows you to connect with other professionals and learn from their experiences.
MATLAB Documentation and Tutorials
Refer to the official MATLAB documentation for comprehensive tutorials on implementing `parfor` and other advanced parallel computing techniques to continue your learning journey.