The `corrcoef` function in MATLAB computes the correlation coefficients between pairs of variables in a dataset, providing insights into their linear relationships.
Here's a simple code snippet to demonstrate its usage:
% Sample data
data = [1 2 3; 4 5 6; 7 8 9];
% Calculate correlation coefficients
R = corrcoef(data);
Understanding the `corrcoef` Function
Definition and Purpose
The `corrcoef` function in MATLAB plays a vital role in statistical analysis by calculating correlation coefficients between two or more variables. Correlation coefficients measure the strength and direction of the relationship between paired data points. A value of 1 indicates a perfect positive correlation, -1 indicates a perfect negative correlation, and 0 indicates no correlation at all.
Syntax of `corrcoef`
The syntax for using the `corrcoef` function is straightforward:
R = corrcoef(A)
In this command, `A` represents the input data matrix where each column corresponds to a different variable. The output `R` is a correlation matrix that summarizes the correlation coefficients for all pairs of variables present in the input matrix.
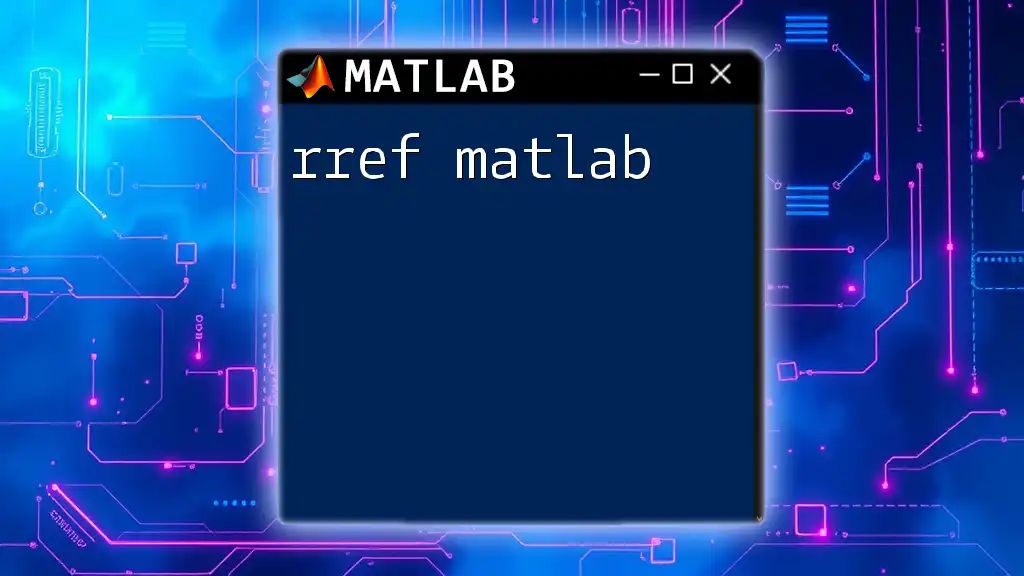
Preparing Data for Correlation Analysis
Types of Data Suitable for `corrcoef`
Only numerical data types are appropriate for the `corrcoef` function. Data can come from various sources, such as experiments, surveys, or databases, but it should always represent continuous variables. Categorical data must first be converted into numerical formats, such as dummy variables, before analysis.
Data Importing and Formatting
To analyze data effectively, you need to import it into MATLAB. The most common formats are CSV and Excel files. You can use the following methods to import your data:
data = readtable('data.csv');
data = rmmissing(data); % Remove missing values
In this example, you are reading in a CSV file called `data.csv` and cleaning it by removing rows with missing values.
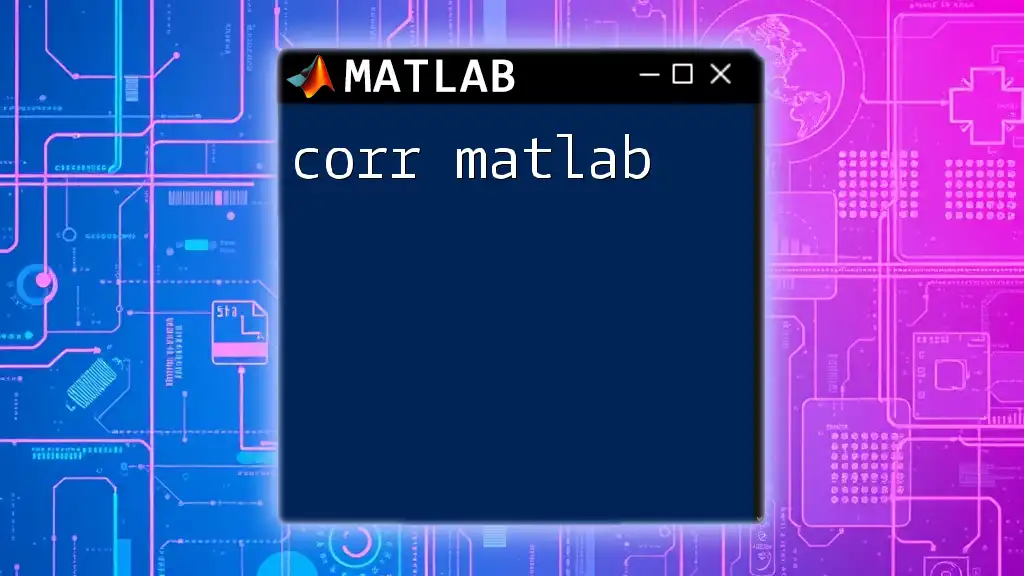
Using the `corrcoef` Function
Basic Usage
To demonstrate the basic functionality of `corrcoef`, consider the following simple example:
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
R = corrcoef(x, y);
disp(R);
In this code snippet, the vectors `x` and `y` are defined, and then their correlation coefficients are calculated. The output is a matrix where the correlation coefficient appears in both the (1,1) and (2,2) positions, while the cross-correlation appears in the (1,2) and (2,1) positions.
Interpreting the Output
When you run the `corrcoef` function, the output is a correlation matrix. For two variables, the output will be a 2x2 matrix. The diagonal entries represent the correlation of the variables with themselves (always 1), while the off-diagonal entries represent the actual correlation between the two variables. Thus, a value close to 1 reveals a strong positive relationship, while a value close to -1 indicates a strong negative relationship.
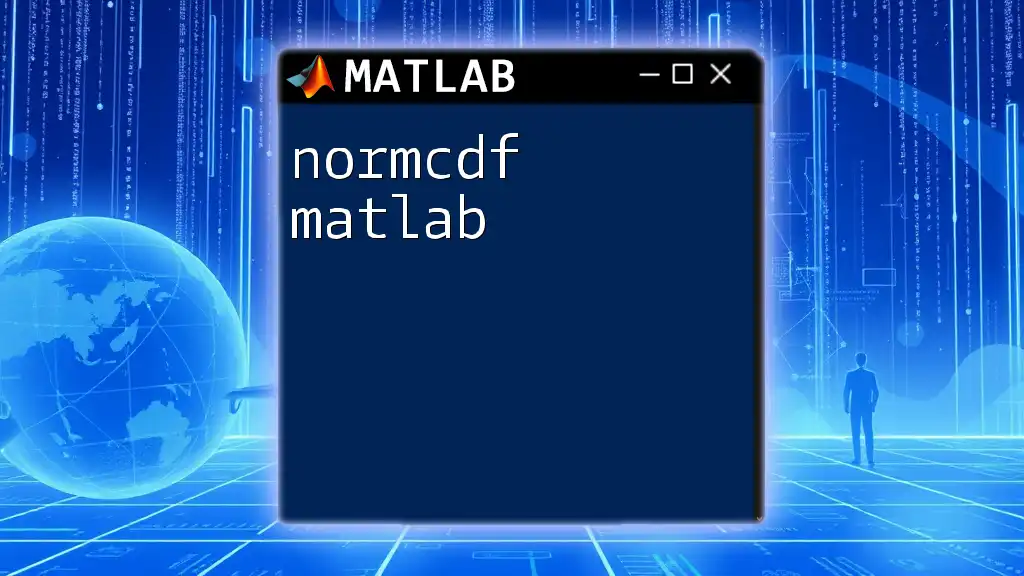
Advanced Usage of `corrcoef`
Multiple Variables
To compute the correlation coefficients for multiple datasets, you may pass a matrix where each column represents a different variable:
A = rand(100, 3); % Generating random data
R = corrcoef(A);
disp(R);
In this example, random data is generated for three variables, yielding a 3x3 correlation matrix showing how each variable correlates with the others.
Optional Outputs
The `corrcoef` function also facilitates optional outputs, such as p-values and confidence intervals. To obtain both the correlation matrix and the p-values, you can use:
[R, P] = corrcoef(x, y);
In this command, `R` contains the correlation coefficients, while `P` includes the corresponding p-values, which are essential for determining the statistical significance of the correlations.
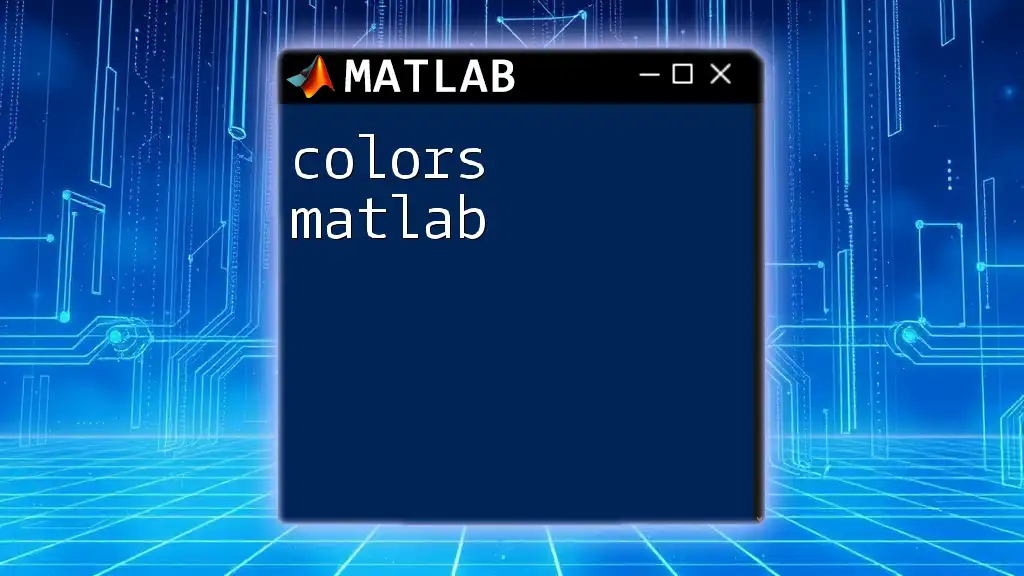
Practical Applications of `corrcoef`
Statistical Analysis
The `corrcoef` function has several real-world applications in fields like finance, biology, and social sciences. In finance, for example, it’s used to assess the relationship between different stocks to inform investment strategies. In social science research, understanding the correlation between survey responses can reveal critical insights into societal trends and behaviors.
Visualizing Correlation
Scatter Plots
Creating scatter plots provides a visual representation of correlations. Here’s how to create one in MATLAB:
scatter(x, y);
xlabel('X-axis label');
ylabel('Y-axis label');
title('Scatter Plot of X vs Y');
grid on;
This visualization can help you detect the presence and nature of relationships between variables.
Heat Maps
For a more comprehensive view, you can generate a heat map from the correlation matrix like this:
heatmap(R);
Heat maps visually represent correlation coefficients, allowing for quick identification of strong or weak relationships among multiple variables.
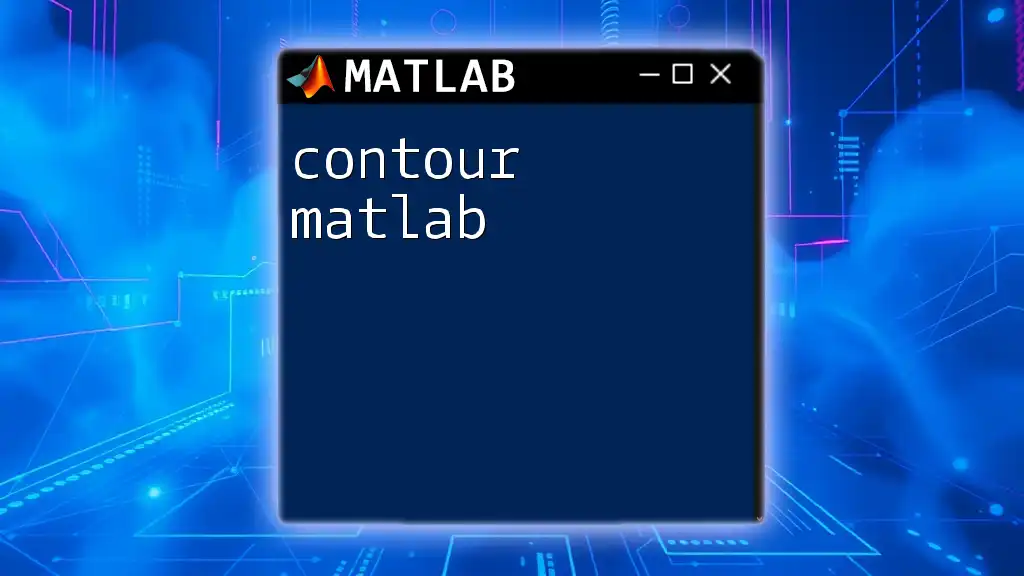
Common Mistakes and Troubleshooting
Common Errors with `corrcoef`
While using `corrcoef`, you might encounter several common pitfalls. One frequent mistake is using non-numeric data types, which will lead to an error. Always ensure your data is numeric before applying the function.
Verifying Results
After acquiring results, it's essential to verify them against previously known correlations or established research findings. If your correlation values appear unusual, review your data for potential discrepancies or outliers.
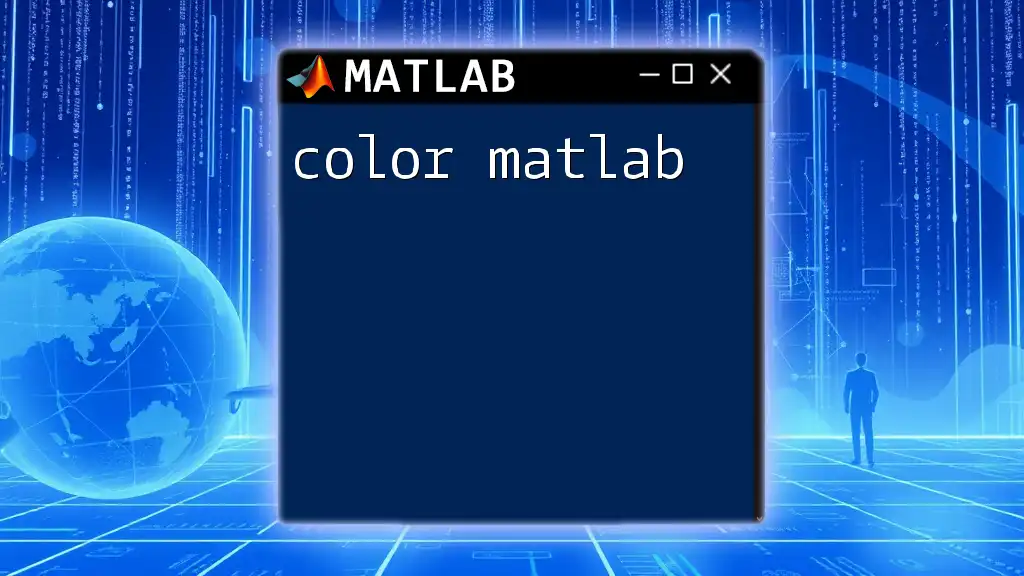
Conclusion
Understanding and utilizing the `corrcoef` function in MATLAB is crucial for effective data analysis. By mastering this function, you can conduct meaningful statistical analyses that can greatly enhance your understanding of the relationships among variables.
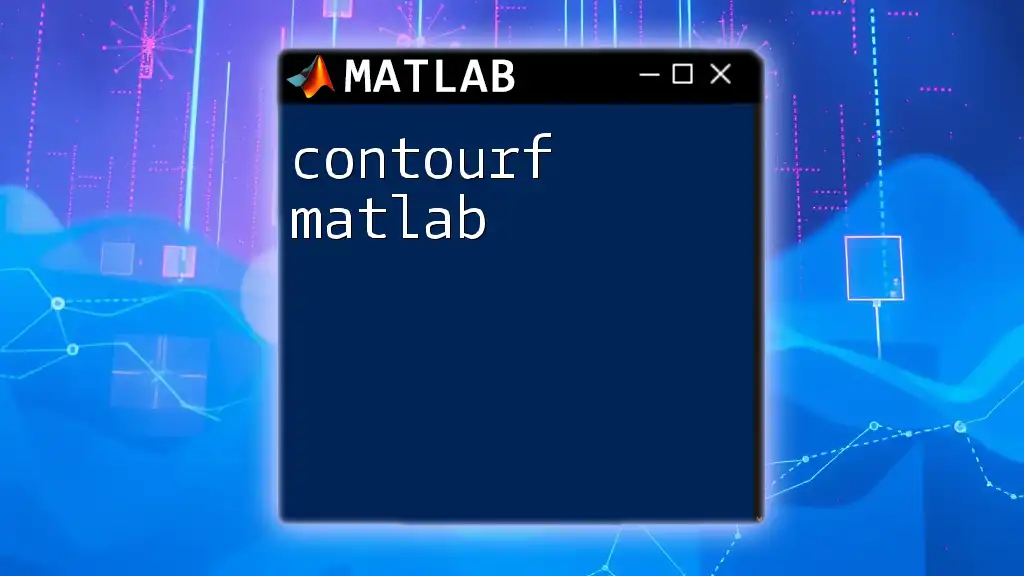
Further Reading and Resources
For a deeper understanding, refer to the official [MATLAB documentation](https://www.mathworks.com/help/matlab/ref/corrcoef.html) on `corrcoef`. Additionally, exploring textbooks and online courses focused on statistics and MATLAB can further your knowledge and application of these essential concepts.
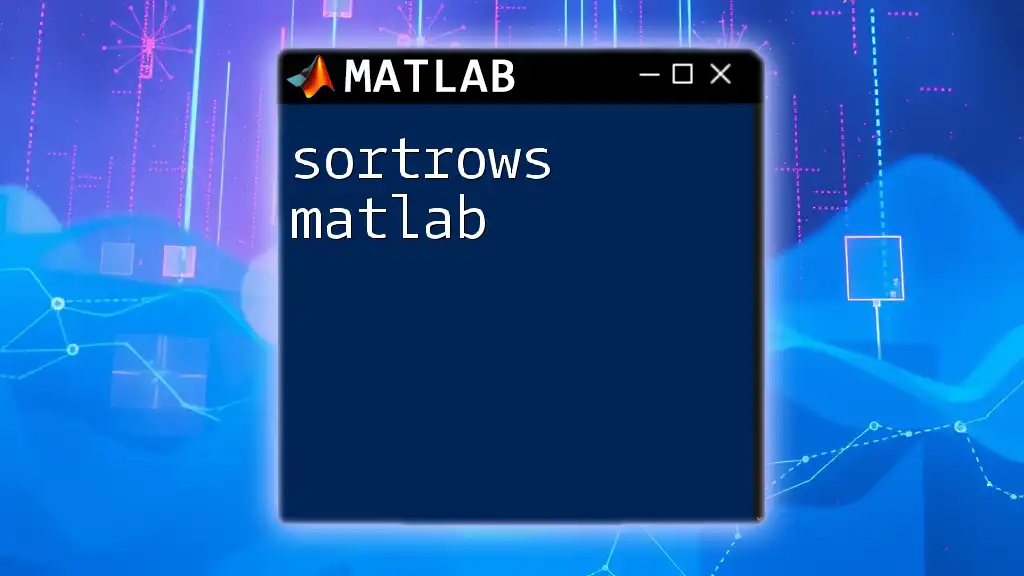
Call to Action
Join the MATLAB learning community today to deepen your understanding and improve your skills. Participate in our upcoming workshops or tutorials to explore this subject and more in greater detail!