The `det` function in MATLAB calculates the determinant of a matrix, which is a valuable tool in linear algebra to assess the properties of matrices.
A = [1, 2; 3, 4];
det_A = det(A);
disp(det_A);
Understanding the `det` Function in MATLAB
What is the `det` Function?
The `det` function in MATLAB computes the determinant of a square matrix. The determinant is a scalar value that provides important information about the matrix, such as whether it is invertible and the volume scaling factor associated with the linear transformation represented by the matrix. In essence, if you have a matrix \( A \), the determinant can give insights into the nature of systems of linear equations represented by this matrix.
Applications of the Determinant
Determinants have numerous applications across various fields:
- Engineering: In structural engineering, determinants are used to analyze stresses and strains in materials, as well as in stability calculations.
- Physics: In mechanics and physics simulations, determinants help determine vectors' orientation and scaling properties in transformations.
- Statistics: They play a role in multivariate statistics, such as in the computation of covariance matrices, affecting correlations among variables.
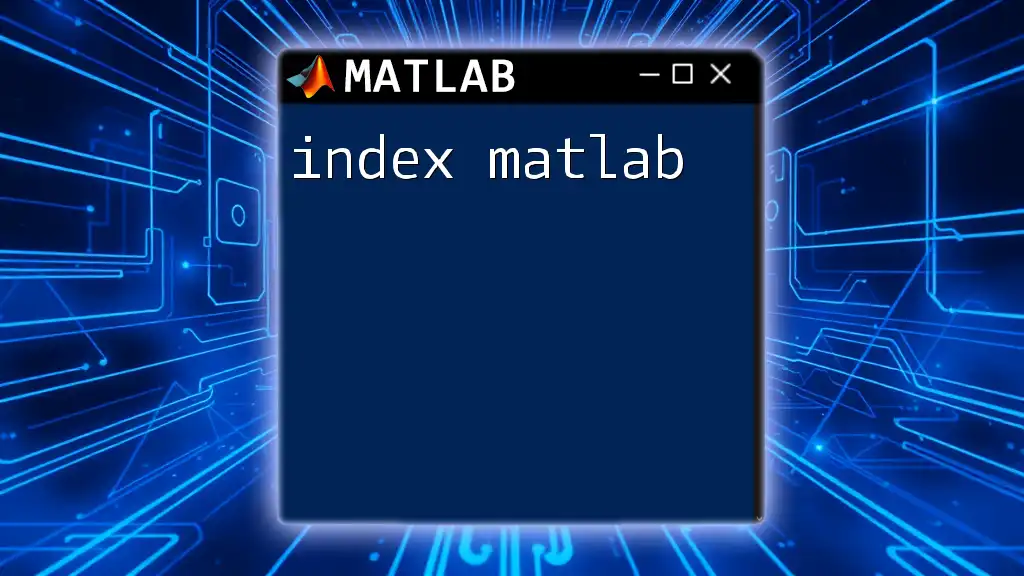
Syntax of the `det` Function
Basic Syntax
The usage of the `det` function is straightforward. The general syntax is as follows:
d = det(A)
Here, `A` is the input square matrix of which you want to compute the determinant. The returned value `d` is the determinant of `A`.
Input Requirements
It is crucial to ensure that the input matrix meets specific requirements for the `det` function to work correctly:
- Square Matrix Input: The matrix `A` must be square (having the same number of rows and columns). If `A` is not square, MATLAB will throw an error.
- Data Types: The `det` function supports numeric matrices, such as double or single precision. It can also handle symbolic matrices when using MATLAB's Symbolic Math Toolbox.
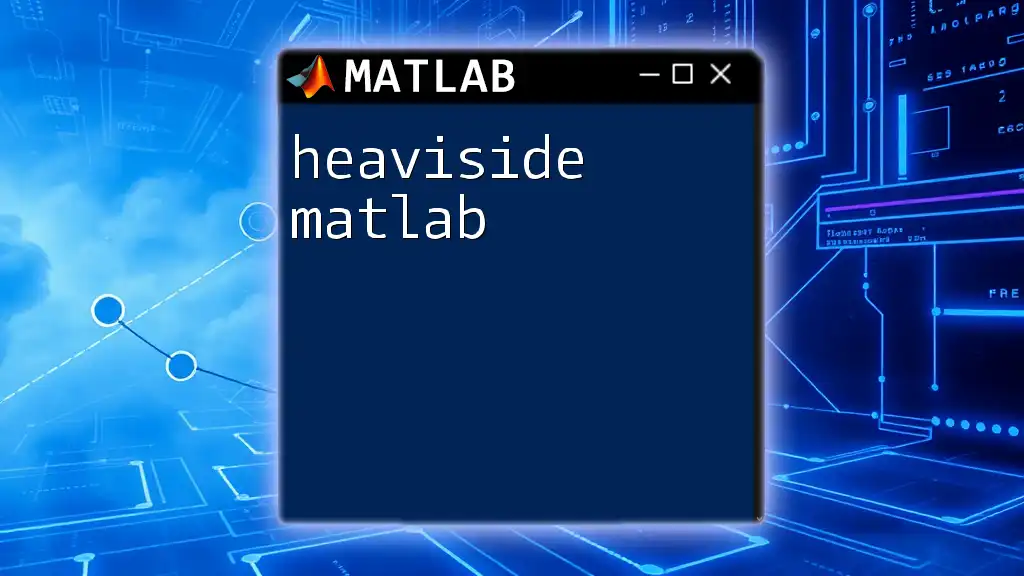
Step-by-Step Guide to Using `det`
Creating a Matrix
To begin using the `det` function, you need a square matrix. Here’s an example of how to create a simple 2x2 square matrix:
A = [1, 2; 3, 4];
This snippet creates a matrix `A`:
\[ A = \begin{pmatrix} 1 & 2 \\ 3 & 4 \end{pmatrix} \]
Calculating the Determinant
Once you have your square matrix, you can easily compute its determinant by calling the `det` function:
d = det(A);
This will assign the determinant of matrix `A` to the variable `d`. In this case, for matrix `A`, the determinant will be calculated as follows:
\[ \text{det}(A) = 1 * 4 - 2 * 3 = 4 - 6 = -2 \]
Example Scenario
Let’s take a more complex example by calculating the determinant of a 3x3 matrix:
B = [1, 2, 3; 0, 1, 4; 5, 6, 0];
det_B = det(B);
For matrix `B`, the determinant is computed, and the value of `det_B` will show insights about the volume scaling and whether the transformation is invertible. The calculated value here would be -24, indicating flatness towards the origin in a geometric interpretation.
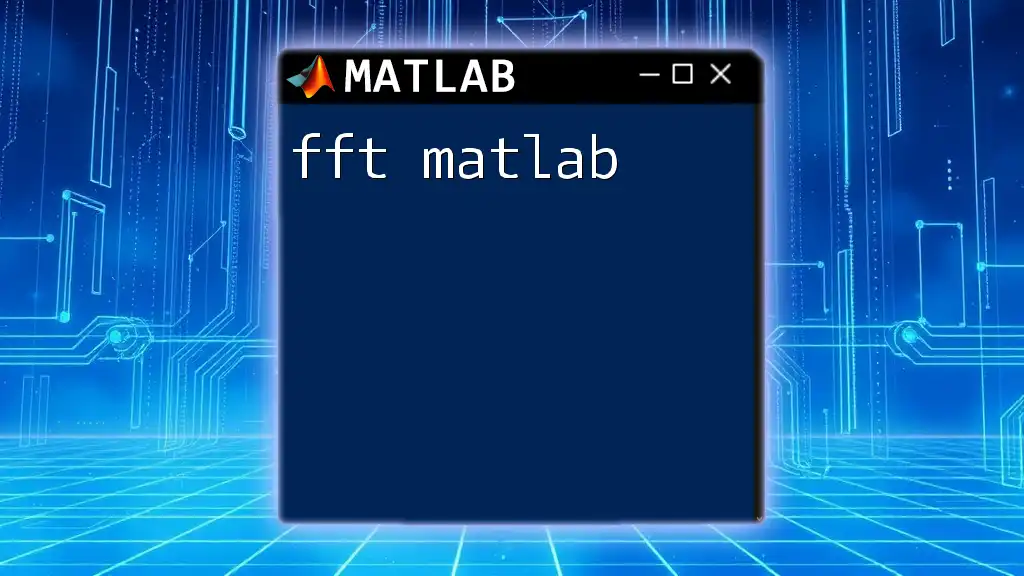
Key Properties of Determinants
Linearity
Determinants exhibit a property known as linearity, which states that if any row of a determinant is expressed as a linear combination of other rows, the determinant becomes a linear function of that row. For instance, if you scale one row of the matrix by a factor of \( k \), the determinant is also scaled by that same factor.
Multiplicative Property
Another essential property is that the determinant of the product of two matrices equals the product of their determinants:
C = A * B;
det_C = det(A) * det(B);
This property becomes particularly useful when working with linear transformations—helping confirm relationships between different transformations represented by matrices.
Effects of Row Operations
Certain row operations have distinct effects on the determinant:
- Row Switching: Switch two rows of a matrix will negate the determinant.
- Row Scaling: Multiplying a row by a constant will multiply the determinant by that constant.
- Row Addition: Adding a multiple of one row to another does not affect the determinant.
Determinant of Inverse and Transpose
The determinant behaves predictably for matrix inverses and transposes:
det_invA = det(inv(A));
det_transposeA = det(A');
For a matrix \( A \), the determinant of its inverse is given by:
\[ \text{det}(\text{inv}(A)) = \frac{1}{\text{det}(A)} \]
And importantly, the determinant of a transpose is the same as the original:
\[ \text{det}(A) = \text{det}(A') \]
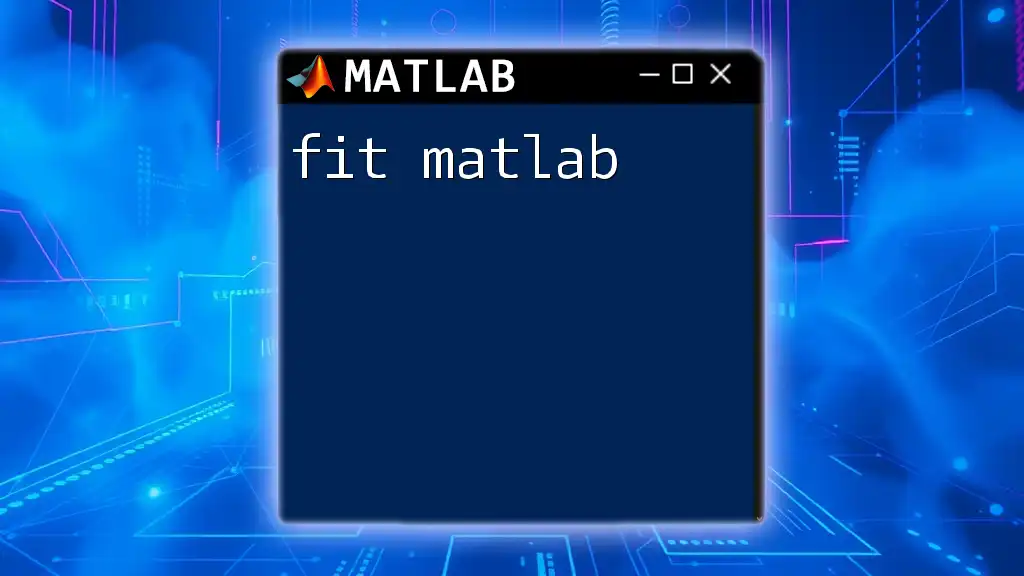
Common Errors and Troubleshooting
Non-Square Matrices
One common mistake users might encounter is trying to compute the determinant of a non-square matrix. MATLAB will produce an error message indicating that the input must be a square matrix. Be vigilant to create matrices of the appropriate dimensions.
NaN Outputs
If you receive a NaN (Not a Number) result, this often signals singularity in the matrix. It may also occur due to numerical instability or overflow. To resolve this, examine your matrix for potential issues or reduce the complexity of your calculations.
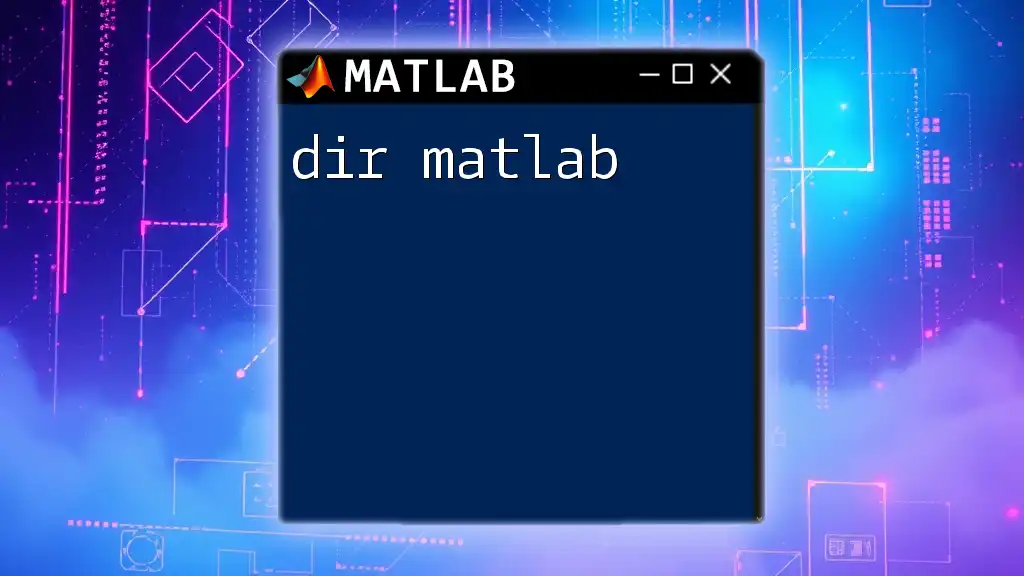
Conclusion
Understanding the `det` function is crucial for anyone looking to deepen their MATLAB capabilities, especially in disciplines like mathematics and engineering. The insights gained from determinants extend far beyond simple calculations, impacting how we analyze and interpret linear transformations.
Further Resources
To further enhance your understanding of the `det` function and its applications, explore MATLAB's official documentation or consider enrolling in online courses that dive deeper into linear algebra and MATLAB programming.
Call to Action
Now that you have a comprehensive understanding of the `det` function, take a moment to experiment with different matrices and observe how the determinants behave under various operations. Join us on this journey at our company where we aim to provide quick, concise, and effective training in MATLAB commands!