The Fast Fourier Transform (FFT) in MATLAB is a powerful algorithm for converting a signal from its original domain (often time or space) into the frequency domain, enabling efficient analysis of frequency components.
Here’s a simple example of how to use the FFT command in MATLAB:
% Define a sample signal
t = 0:0.001:1; % Time vector
signal = sin(2*pi*50*t) + sin(2*pi*120*t); % Signal with two frequency components
% Compute the FFT
Y = fft(signal);
% Calculate the corresponding frequencies
Fs = 1000; % Sampling frequency
L = length(signal); % Length of the signal
f = Fs*(0:(L/2))/L; % Frequency range
% Compute the two-sided spectrum P2 and single-sided spectrum P1
P2 = abs(Y/L);
P1 = P2(1:L/2+1);
P1(2:end-1) = 2*P1(2:end-1);
% Plot the single-sided amplitude spectrum
figure;
plot(f, P1);
title('Single-Sided Amplitude Spectrum of Signal');
xlabel('Frequency (f) [Hz]');
ylabel('|P1(f)|');
Understanding the Basics of FFT
What is FFT?
FFT, or Fast Fourier Transform, is a pivotal mathematical algorithm used to convert signals from the time domain to the frequency domain. Its primary purpose is to reveal the frequency components of a signal, which is essential in fields such as signal processing, communications, and audio analysis.
The Fourier Transform essentially analyzes the underlying frequency content of signals, and FFT is an optimized version of this process, making it exceptionally faster and more efficient. The distinction between FFT and DFT (Discrete Fourier Transform) lies in speed: while DFT computes the same result as FFT, it does so at a significantly higher computational cost.
Why Use FFT?
The advantages of employing FFT in computations cannot be overstated. Firstly, speed and efficiency are paramount; FFT reduces the time complexity of transforming a signal from O(N^2) with traditional DFT to O(N log N). This efficiency is crucial when working with large datasets or in real-time signal processing scenarios. Furthermore, FFT is particularly adept at reducing computational complexity, allowing researchers and engineers to analyze frequencies quickly and accurately.
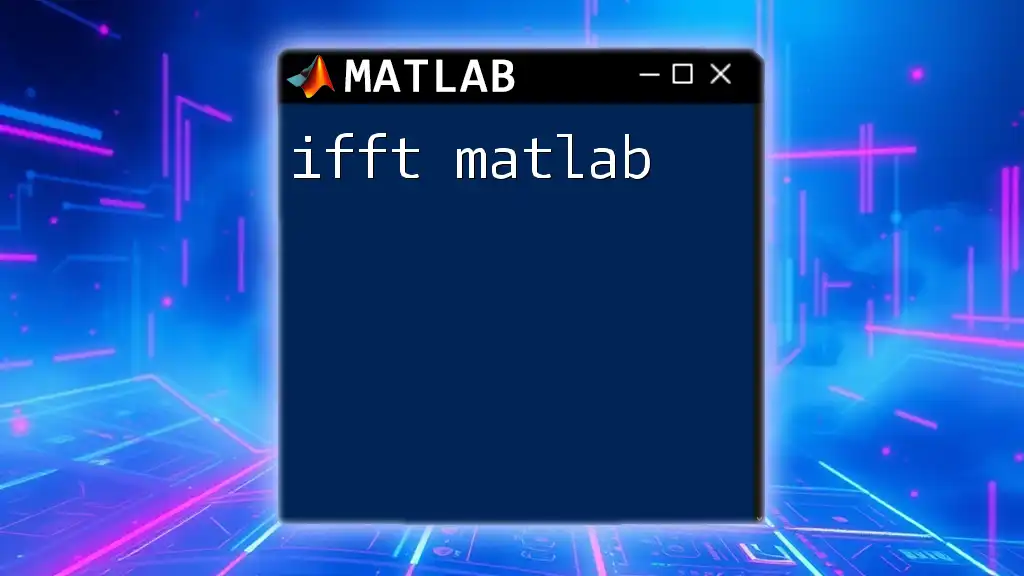
Setting Up Your MATLAB Environment
Installing MATLAB
To perform FFT operations, you need MATLAB installed on your system. The installation process typically includes downloading the installer from the MathWorks website and following the on-screen instructions. Ensure you also install the Signal Processing Toolbox, which enhances your capabilities with additional functions specifically designed for signal analysis.
Basic MATLAB Commands for FFT
Familiarizing yourself with fundamental MATLAB commands is essential. The most relevant commands for FFT include:
- `fft`: This command computes the discrete Fourier Transform.
- `ifft`: The inverse function to transform data back from the frequency domain to the time domain.
- `fftfreq`: Useful for generating frequency vectors relevant to your FFT outputs.
Understanding the syntax of these commands and their parameters is crucial for effective utilization. For instance, the `fft` command takes in a vector as an input, which represents data samples in the time domain.
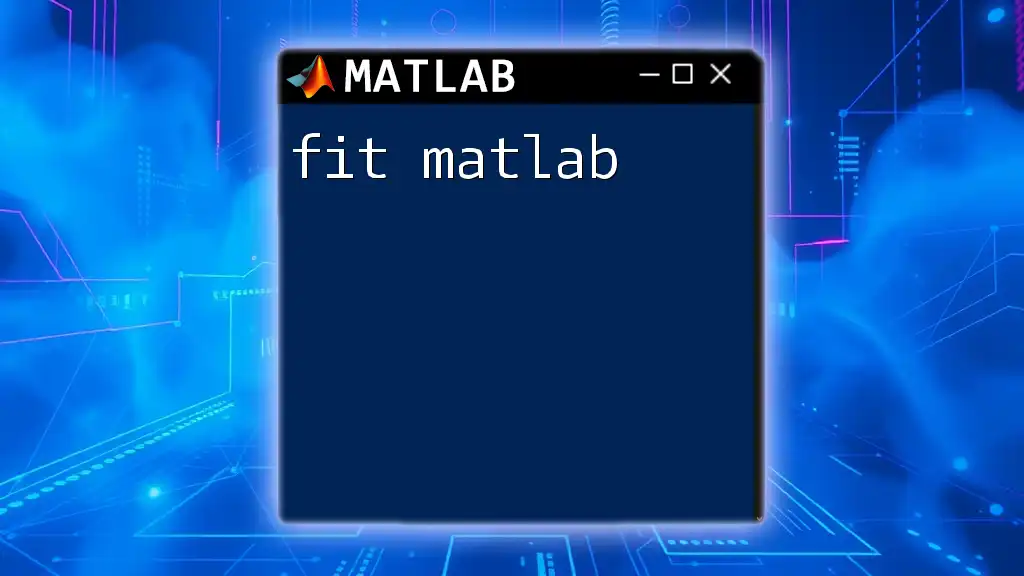
Performing FFT in MATLAB
Steps to Perform FFT
Before applying FFT, your data needs to be properly loaded and prepared. Here’s how you can load a signal from a CSV file in MATLAB:
data = readtable('signal_data.csv');
signal = data.value; % Assume 'value' is the column with your signal
Applying FFT to a Signal
Once the signal is loaded, you can easily apply the FFT. Use the following command:
Y = fft(signal);
The output `Y` is a complex vector representing the frequency components of the input signal. Each element in `Y` corresponds to a specific frequency component, enabling analysis of the signal's frequency content directly.
Plotting the FFT Result
Visualizing Frequency Spectrum
Visualizing the results of your FFT will help you understand the frequency components better. To plot the frequency spectrum, you can use the following code:
fs = 1000; % Sampling frequency
L = length(signal); % Length of signal
f = fs*(0:(L/2))/L; % Frequency range
P2 = abs(Y/L); % Compute amplitude
P1 = P2(1:L/2+1);
P1(2:end-1) = 2*P1(2:end-1);
% Plot
plot(f, P1);
title('Single-Sided Amplitude Spectrum');
xlabel('Frequency (f)');
ylabel('|P1(f)|');
This code snippet computes the amplitude of the frequencies and produces a plot that reveals the dominant frequency components present in your original signal.
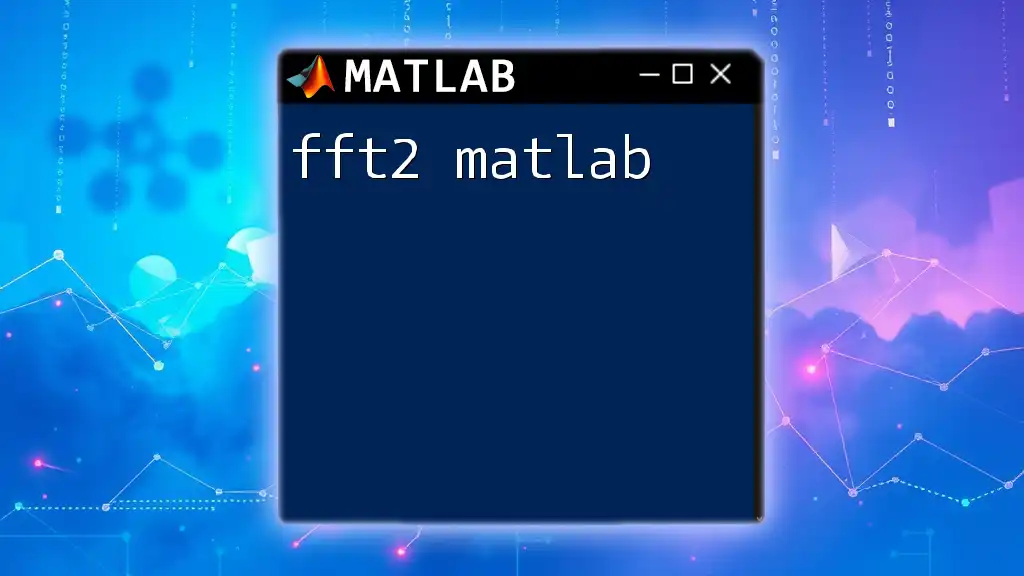
Real-World Examples of FFT in MATLAB
Example 1: Analyzing a Simple Sine Wave
Sine waves are fundamental signals in signal processing. Here’s how to generate and analyze a simple sine wave using FFT:
fs = 1000; % Sampling frequency
t = 0:1/fs:1-1/fs; % Time vector
f = 5; % Frequency of sine wave
signal = sin(2*pi*f*t); % Generate sine wave
Y = fft(signal);
This will yield the frequency components of a 5 Hz sine wave, allowing for analysis of its fundamental frequency.
Example 2: Identifying Frequency Components in a Noisy Signal
In many real-world applications, signals are not clean. Here’s an example of generating a noisy signal and using FFT to identify the frequency components:
signal = sin(2*pi*50*t) + 0.5*randn(size(t)); % Sine wave with noise
Y = fft(signal);
By analyzing `Y`, we can discern the underlying frequency despite the noise, showcasing the robustness of FFT in noisy data analysis.
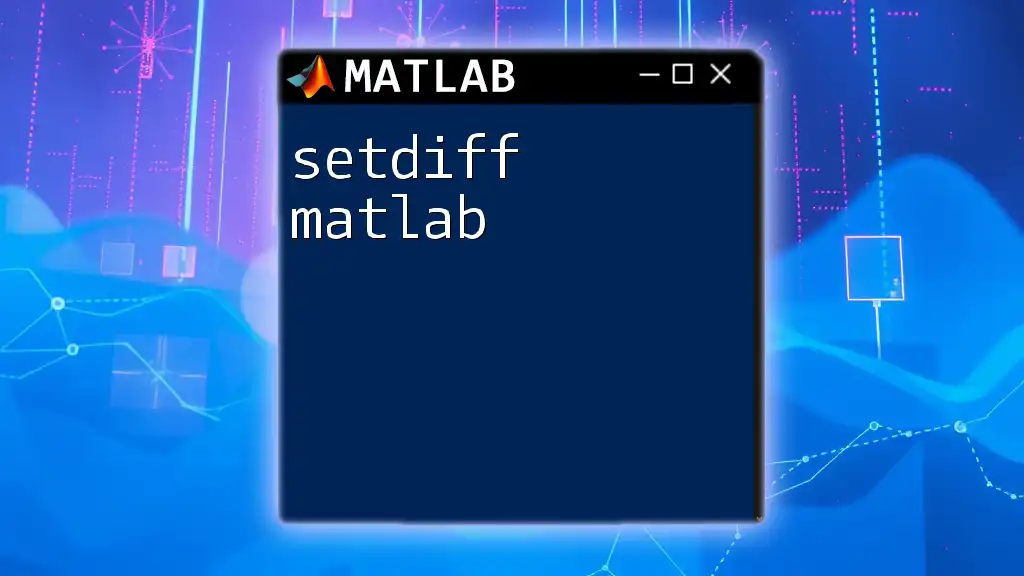
Common Pitfalls and Solutions
Handling Signal Lengths
When applying FFT, it’s crucial to manage the length of your signal. Zero-padding may become necessary to improve frequency resolution. Zero-padding refers to adding zeros to your signal to increase its length, enabling better frequency estimation.
N = 2^nextpow2(L); % Next power of 2
Y = fft(signal, N); % Zero padding
Using this technique allows you to upscale the resolution of your FFT analysis.
Understanding Frequency Resolution
Frequency resolution is influenced by the sampling rate and the length of the signal. A higher sampling rate or a longer signal leads to better frequency resolution. Keep in mind the trade-off between increasing resolution and computational efficiency, especially in real-time applications.
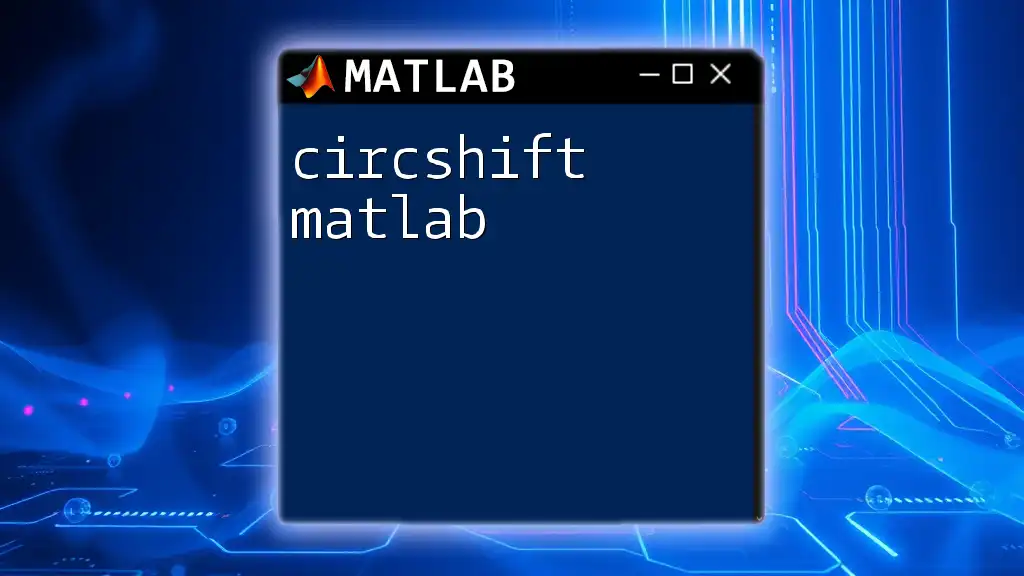
Advanced FFT Techniques in MATLAB
Using Window Functions
Windowing is crucial when analyzing signals, especially in preventing spectral leakage. MATLAB offers built-in functions for various window types, such as Hamming and Hanning windows. Apply a window to your signal as follows:
windowed_signal = signal .* hamming(length(signal))';
Using a window function smoothens the edges of the time-domain signal, enhancing the FFT output's accuracy.
Multi-dimensional FFT
For complex datasets, MATLAB provides functionality for multi-dimensional FFT. This is especially useful in image processing or when dealing with 2D or 3D datasets. To perform a 2D FFT, you can use:
Z = fft2(matrix); % Perform 2D FFT
This command transforms images or matrices into their frequency components, enabling a range of applications from image compression to feature extraction in data analysis.
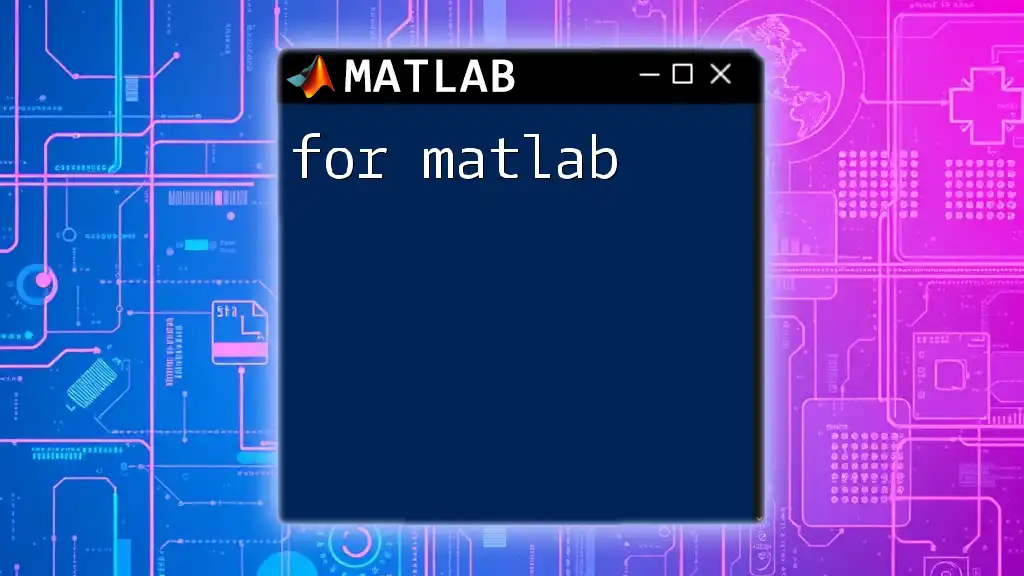
Conclusion
Exploring the `fft matlab` functionality provides invaluable tools for signal analysis. FFT's efficiency and ability to reveal frequency components empower users to dive deeper into data dynamics, be it for industrial, research, or entertainment applications. MATLAB serves as an exceptional platform for mastering FFT techniques, enabling users to parse, analyze, and understand their signals with clarity. By practicing with the provided examples and refining your skills with advanced techniques, you will harness the full potential of FFT in your MATLAB projects.
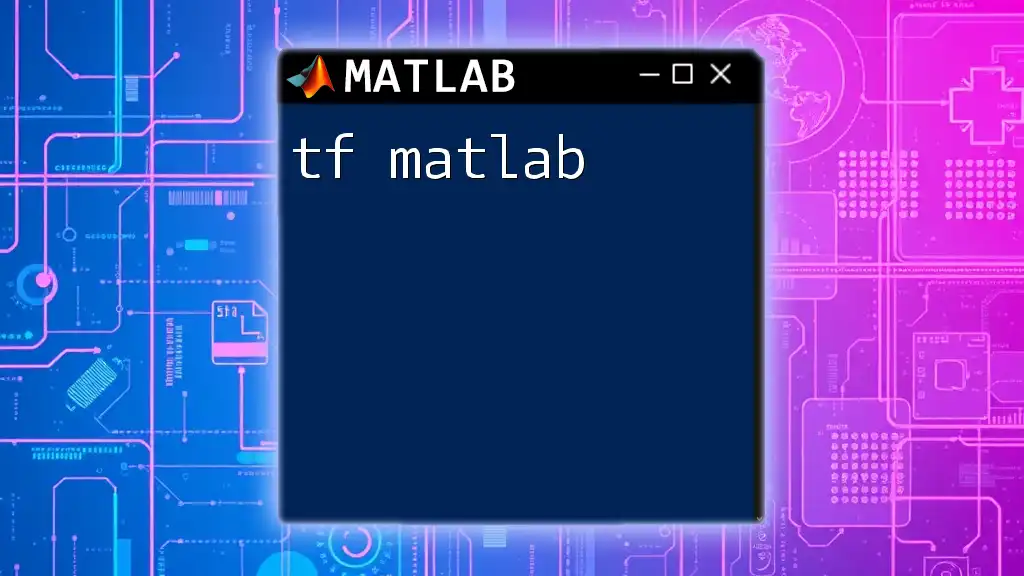
Additional Resources
For those looking to deepen their knowledge and expertise, consider exploring MATLAB’s official documentation on FFT, as well as recommended books and online courses dedicated to this essential topic in signal processing.