"Fit MATLAB" refers to the process of creating a mathematical model that best describes a set of data points using the `fit` function, which provides robust fitting methods in MATLAB.
Here's a simple example of fitting a linear model to data:
% Sample data points
x = [1 2 3 4 5];
y = [2.2 2.8 3.6 4.5 5.1];
% Fit a linear model
f = fit(x', y', 'poly1');
% Plot the data and the fit
plot(f, x, y);
Understanding Curve Fitting
What is Curve Fitting?
Curve fitting is a technique used to create a curve or mathematical function that best describes a set of data points. Its primary purpose is to model real phenomena represented by observational data, often helping in predictions or understanding relationships. Curve fitting can be performed using different types of models, including linear, polynomial, and nonlinear equations.
When to Use Curve Fitting
There are various scenarios where curve fitting is beneficial. For example, scientists might want to analyze experimental data to understand the relationship between temperature and reaction rates, or engineers may wish to model stress-strain relationships in materials. Using curve fitting, one can derive useful insights from data, enabling better decision-making based on close approximations of real-world behaviors.
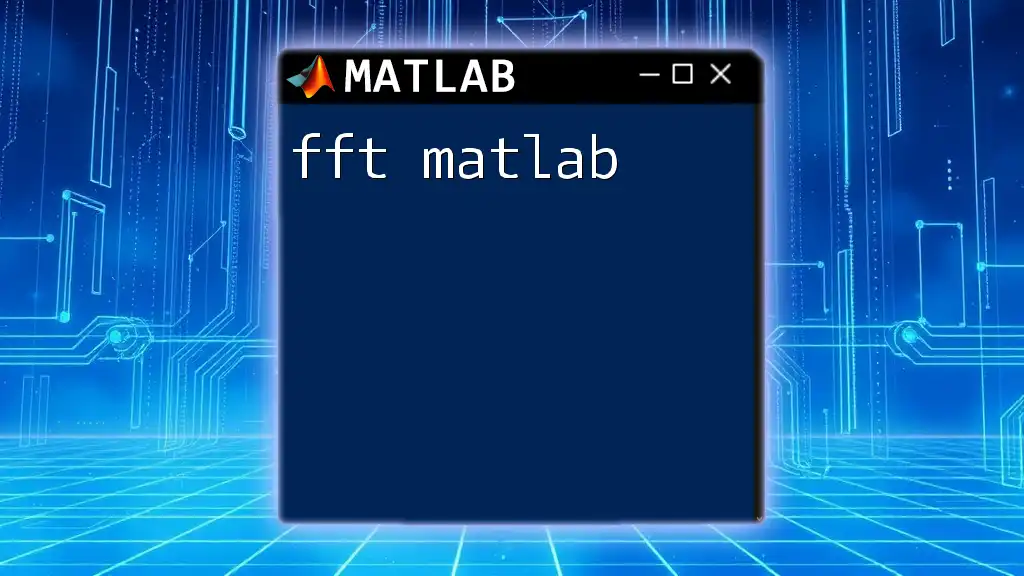
The fit Function in MATLAB
Syntax and Basic Usage
MATLAB provides a powerful built-in function called `fit`, which facilitates the process of fitting curves to data. The syntax for using the `fit` function typically looks like this:
ft = fit(x, y, 'model');
- Here, `x` represents the independent variable data, `y` is the dependent variable data, and `'model'` specifies the type of fit you want to perform.
For instance, if you have a simple dataset, you can fit a linear model as follows:
% Fitting a linear model
x = [1, 2, 3, 4, 5];
y = [2.1, 2.9, 3.6, 4.5, 5.2];
ft = fit(x', y', 'linear');
plot(ft, x, y);
In this example, `fit` creates a linear fit for the given `x` and `y` data, which can be further visualized using the `plot` function.
Key Options and Parameters
The `fit` function in MATLAB supports various options that allow users to tailor their fitting models to their datasets. Some common options include:
- `'polyN'`: For polynomial fitting, where `N` specifies the polynomial degree.
- `'exp1'`: For single exponential fitting.
For example, if you want to fit a polynomial of degree 2 (quadratic fit), you can write:
% Polynomial fitting of degree 2
ft = fit(x', y', 'poly2');
plot(ft, x, y);
This command will generate a quadratic polynomial that best fits the data.
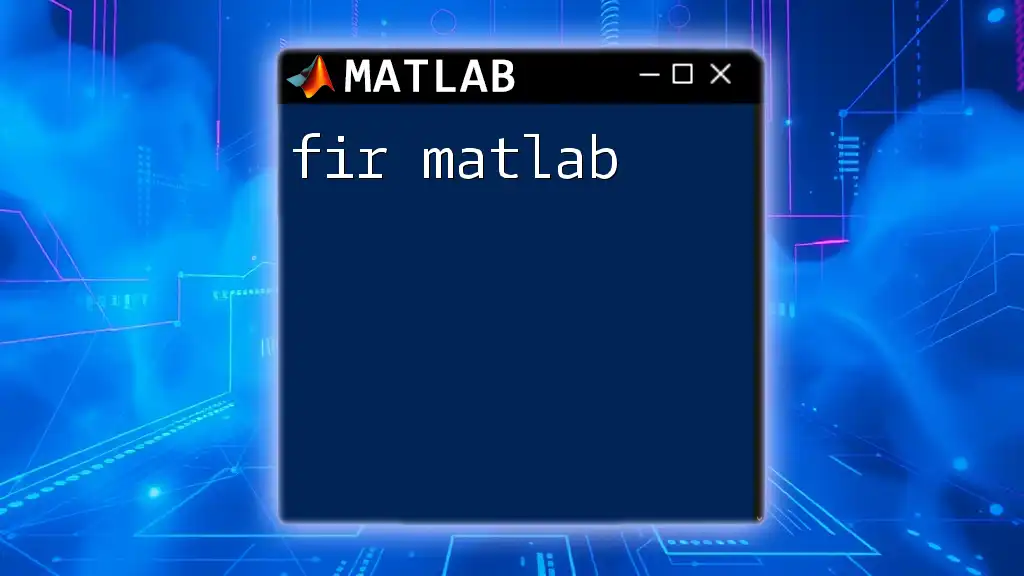
Types of Fit Models
Linear Fit
A linear fit is the simplest form of curve fitting where a straight line is used to model the data. It is expressed as `y = mx + b`, where `m` is the slope and `b` is the y-intercept. This type of fit can effectively approximate data that displays a linear relationship. For example:
% Linear fit example
ft = fit(x', y', 'poly1'); % Poly1 denotes linear model
plot(ft, x, y);
This code snippet will produce a linear fit to the provided data points.
Polynomial Fit
Polynomial fitting involves fitting data with an equation that is a polynomial. This model is useful when the data reveals a nonlinear relationship. As you increase the polynomial degree, the curve can take on a more complex shape, complementing the underlying data better but also risking overfitting.
% Fitting a cubic polynomial
ft = fit(x', y', 'poly3');
plot(ft, x, y);
Using this example, you will fit a cubic polynomial (degree 3) to your dataset.
Nonlinear Model Fit
Nonlinear fitting applies when the data does not follow a straight line or polynomial curve. This fitting is more complex and requires specifying a functional form that models the data appropriately, such as an exponential or logarithmic function.
% Nonlinear fit example with a custom equation
ft = fit(x', y', 'a*exp(b*x)', 'independent', 'x');
plot(ft, x, y);
In this case, a custom nonlinear model is set up to fit the data.
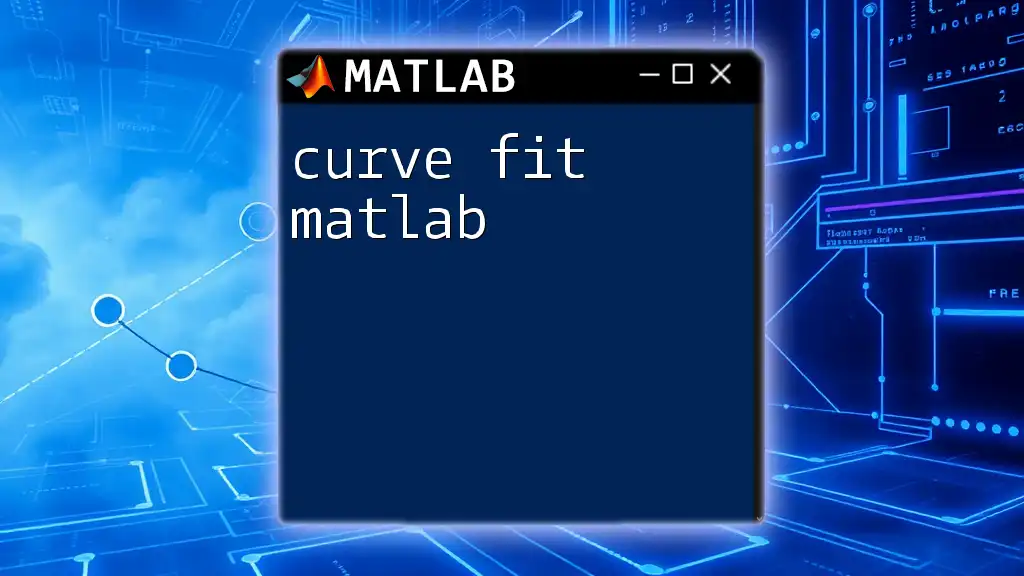
Evaluating the Fit Quality
Goodness of Fit Statistics
Evaluating how well the fitted curve captures the data is crucial. Common statistics used include R-squared, adjusted R-squared, and root mean square error (RMSE). R-squared indicates the proportion of variance in the dependent variable that's predictable from the independent variable.
You can easily extract goodness-of-fit statistics using:
% Getting goodness of fit
gof = ft;
disp(gof);
Inspecting these statistics helps identify the quality of the fit and its predictive power.
Visualizing Fit Quality
Visual inspection remains a powerful method for evaluating fit quality. By plotting the fitted model against the actual data, you can quickly ascertain how well the model conforms to the data points.
% Plotting the fit and data points
plot(ft, x, y);
legend('Data', 'Fitted curve');
This visualization will help you confirm if your model is appropriately representing the variability in your data.
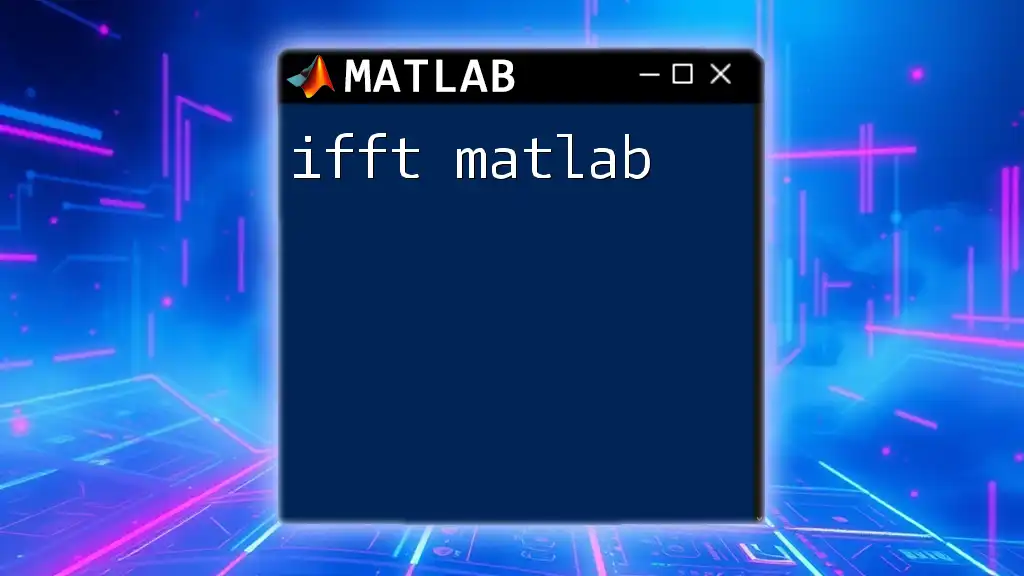
Advanced Topics in Fitting
Using Custom Fitting Models
In many cases, standard fit types may not suffice, necessitating custom fit functions. This allows you to define a model that fits your data's unique behavior.
% Custom fitting function
ft = fit(x', y', 'myCustomFunction');
In this example, `myCustomFunction` could be any function defined in MATLAB that you believe best represents the relationship between your variables.
Fitting Multiple Data Sets
When working with multiple datasets, you can simultaneously fit them by calling the `fit` function for each dataset. This allows for comparative analysis.
% Fitting multiple sets
[ft1, gof1] = fit(x1', y1', 'poly2');
[ft2, gof2] = fit(x2', y2', 'poly2');
By running this code segment, you generate polynomial fits for two independent datasets, facilitating a more comprehensive analysis of how they relate.
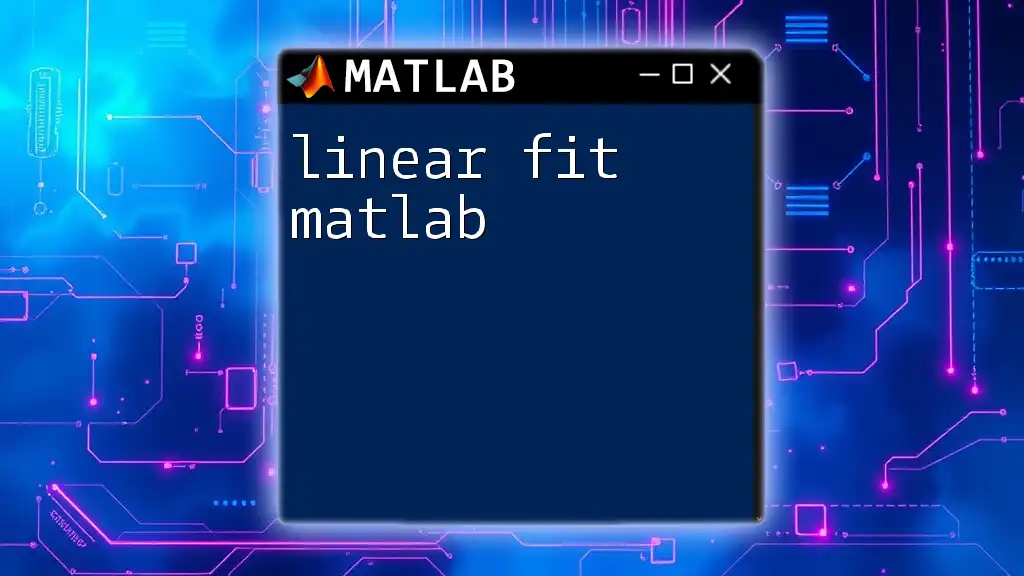
Common Issues and Troubleshooting
Convergence Problems
One common challenge during curve fitting is convergence issues, where the model fails to converge to a solution. This may stem from poor initial parameter values or improper model choices. Increasing the data points for clarity or refining your model can help alleviate these problems.
Overfitting vs. Underfitting
Striking a balance between model complexity and performance is crucial. An overfitted model may capture the noise in the data rather than its underlying trend, leading to poor predictions on new data. Conversely, an underfitted model fails to capture important trends, resulting in biased conclusions. Techniques such as cross-validation can help find this balance.
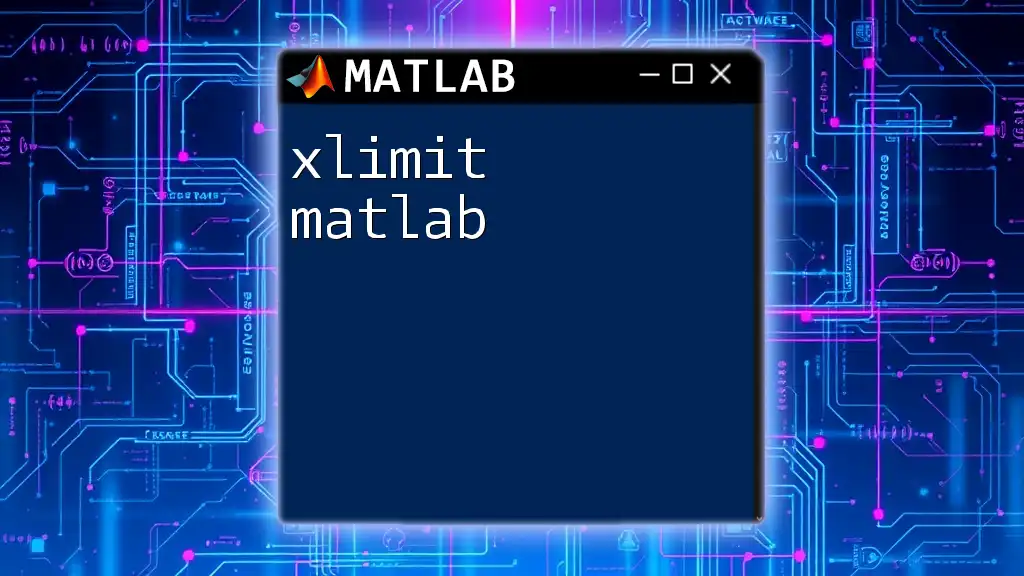
Conclusion
In summary, the `fit` function in MATLAB is a powerful tool for data analysis, enabling users to model relationships in data through various fitting techniques. Whether employing linear, polynomial, or nonlinear fits, the ability to evaluate fit quality and customize models enhances the utility of this function. If you're looking to deepen your skills in MATLAB and curve fitting, practice with real datasets to fully understand and apply these concepts effectively.
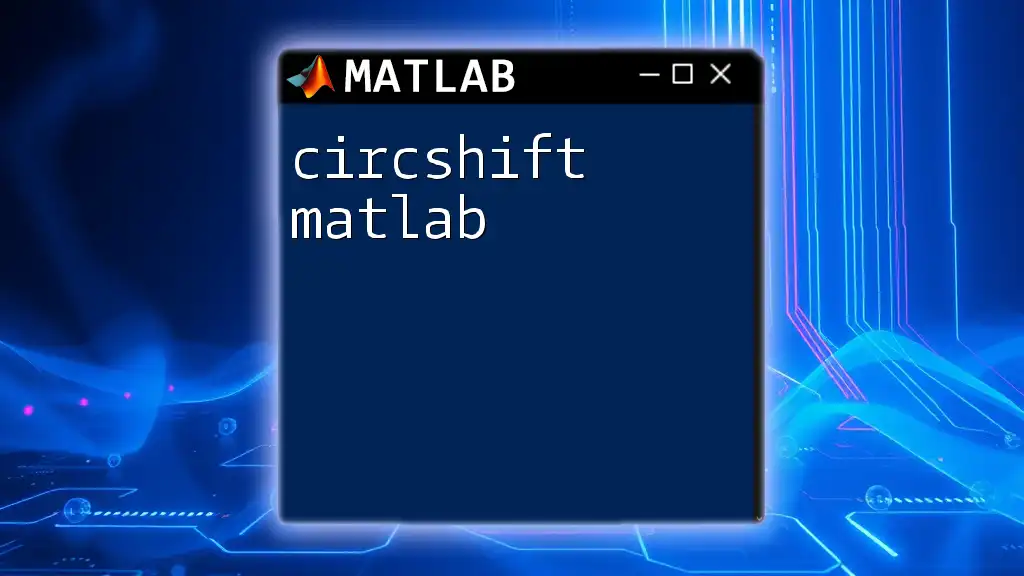
Additional Resources
While the above guide provides a substantial overview of the `fit` function in MATLAB, I encourage you to explore official MATLAB documentation and additional online resources for more advanced topics and practical exercises. This further reading will enhance your understanding and competency in using MATLAB for effective data fitting.