The `int` function in MATLAB is used to compute the symbolic integral of a given function with respect to a specified variable.
Here's a simple example of using the `int` command in MATLAB:
syms x
f = x^2; % Define the function
integral_result = int(f, x); % Compute the integral
disp(integral_result); % Display the result
Understanding the `int` Command
The `int matlab` command is integral for anyone dealing with symbolic mathematics in the MATLAB environment. In essence, `int` stands for 'integrate', and it's commonly used to compute both definite and indefinite integrals of functions. Understanding when and how to use `int` can significantly improve your problem-solving capabilities.
Definition and Purpose
The `int` function aims to facilitate symbolic integration, allowing you to work with algebraic expressions instead of numerical values. This can be particularly powerful for deriving equations and simplifying complex problems prevalent in various disciplines, such as engineering, physics, and economics.
When to Use `int`
Employ the `int` command when you need to:
- Determine exact integral values,
- Work with symbolic variables or expressions,
- Analyze functions over specific intervals.
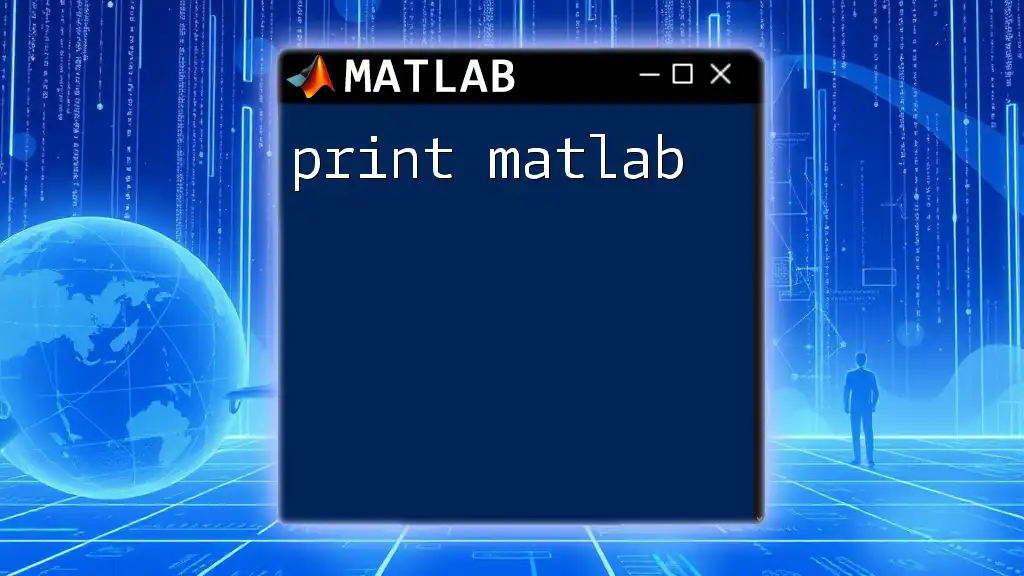
Syntax of the `int` Command
Basic Syntax
The primary syntax for invoking the `int` command is as follows:
int(f, v)
Here, `f` is the function you wish to integrate, and `v` represents the variable of integration.
Extended Syntax Options
For cases requiring definite integrals, the syntax expands to:
int(f, v, a, b)
- `a` and `b` indicate the lower and upper limits for integration, respectively.
Example:
syms x
result = int(x^2, x, 0, 1)
In this example, MATLAB calculates the area under the curve of \(x^2\) from 0 to 1.
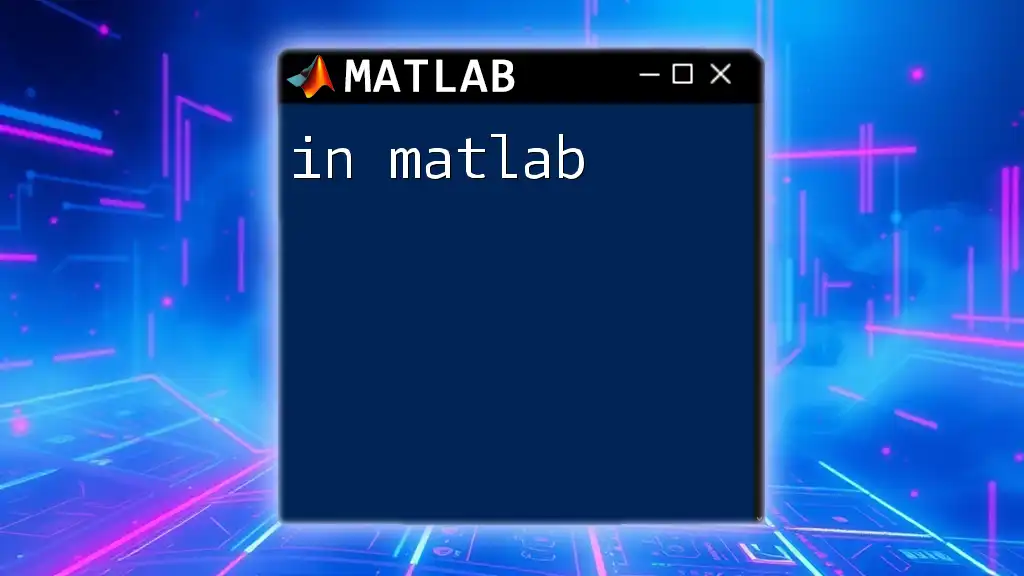
Applications of the `int` Command
Definite vs. Indefinite Integration
It's crucial to distinguish between definite and indefinite integration:
-
Indefinite Integration does not consider limits and generally results in a family of functions (antiderivatives).
- Example:
syms x result = int(x^3, x)
-
Definite Integration computes the integral over specified limits, giving a numerical value.
- Example:
result = int(cos(x), x, 0, pi)
Understanding these types of integration lays the foundation for successfully applying the `int` function in more complex scenarios.
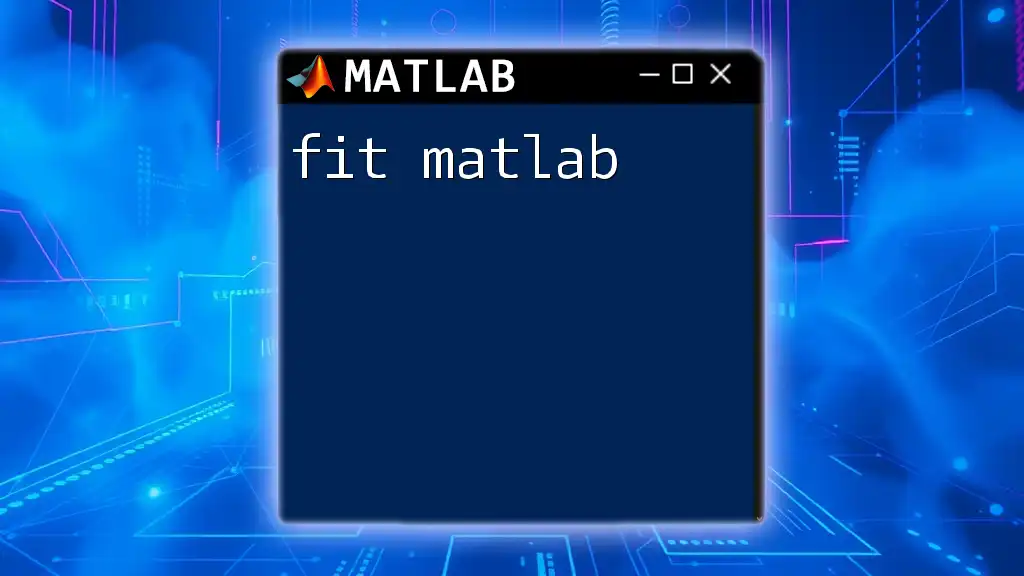
Step-by-Step Examples
Basic Usage Example
To initiate your understanding of the `int` command in MATLAB, let’s start with a straightforward example. Consider the integral of a polynomial.
syms x
result = int(x^3)
This command will output \(\frac{x^4}{4} + C\), where \(C\) is the constant of integration.
Definite Integration Example
For definite integrals, use clear limits to evaluate the function over a specific range.
syms x
result = int(x^2, x, 0, 1)
The result will provide the area under the curve of \(x^2\) from 0 to 1, resulting in \(\frac{1}{3}\).
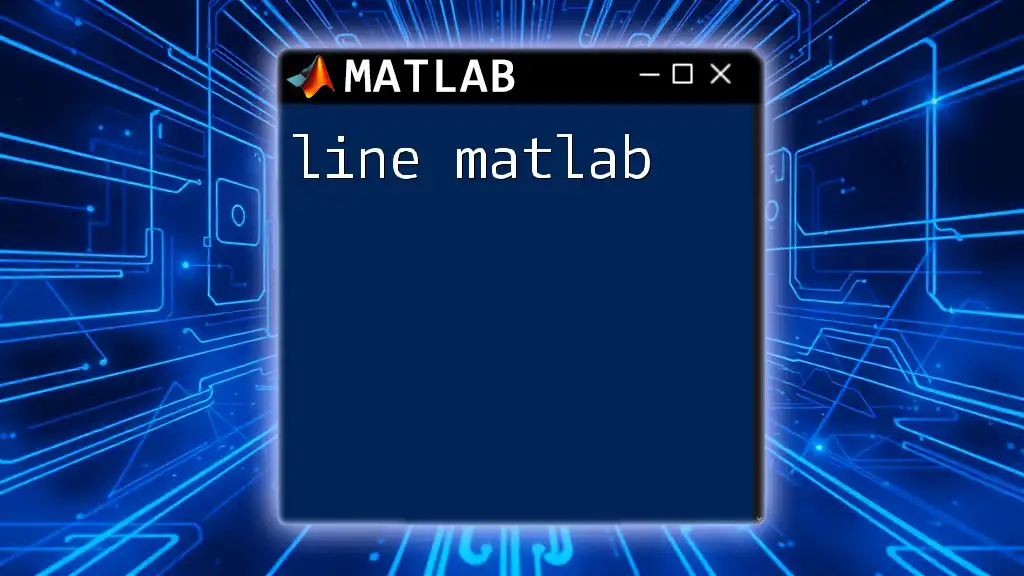
Advanced Features of `int`
Integration with Multiple Variables
The `int` command is versatile and can handle functions involving more than one variable. This expands its utility to multi-dimensional problems.
syms x y
result = int(x*y, x, 0, 1)
Here, the command evaluates the integral over the variable \(x\), treating \(y\) as a constant.
Integrating Functions with Conditions
Integration doesn’t always involve straightforward functions. Piecewise functions can also be integrated using `int`.
syms x
f = piecewise(x < 0, 0, x >= 0, x^2);
result = int(f, x)
In this example, the function defines one behavior for \(x < 0\) and another for \(x \geq 0\), effectively enabling nuanced problem-solving with conditional functions.
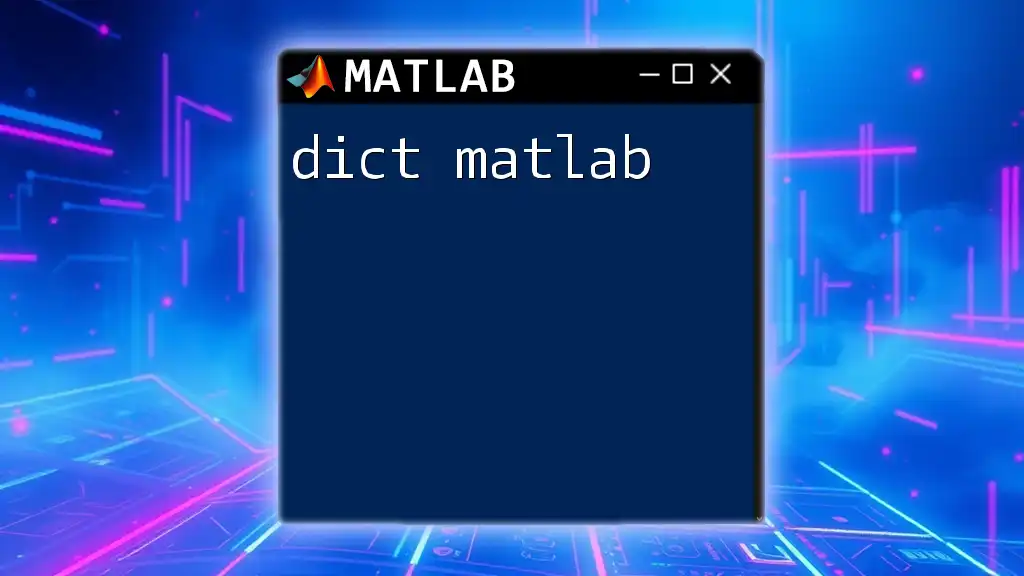
Common Errors and Troubleshooting
Common Errors when Using `int`
It’s not uncommon to encounter errors when utilizing the `int` command. Here are some frequent pitfalls to watch out for:
- Incorrect Limits: If the limits of integration are ordered incorrectly, MATLAB might either return an error or give unexpected results.
- Uninitialized Variables: Failing to define symbolic variables before using them can lead to confusion and errors.
Debugging Tips
When you face integration errors, consider these troubleshooting steps:
- Verify that all variables used are declared as symbolic by using `syms`.
- Ensure that the function you are attempting to integrate is well-defined and does not lead to complications, such as discontinuities within the integration limits.
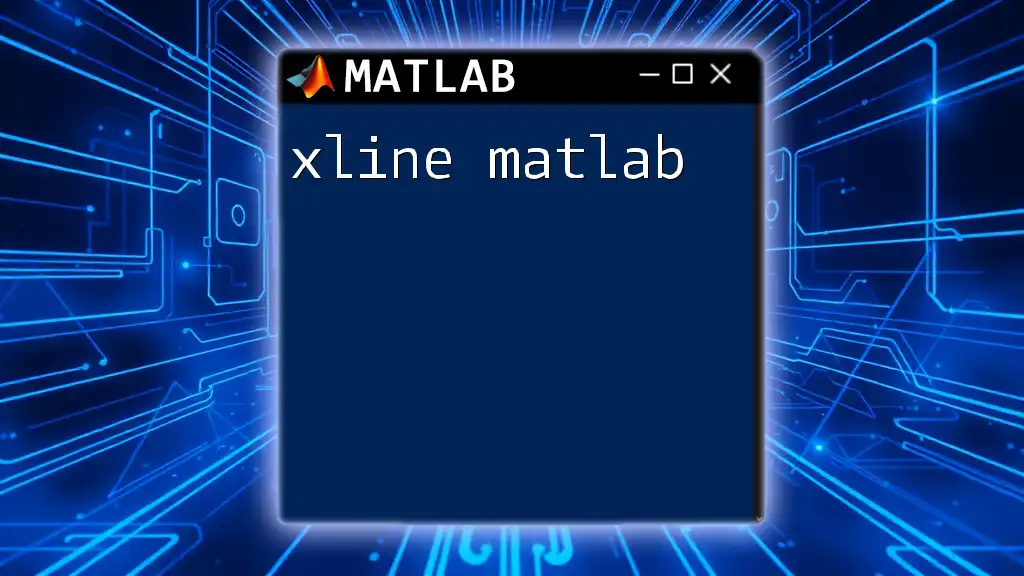
Conclusion
In summary, the `int matlab` command serves as a powerful tool for symbolic integration. By mastering its syntax, understanding its applications, and knowing how to troubleshoot common issues, you can enhance your mathematical modeling and problem-solving aptitude in MATLAB.
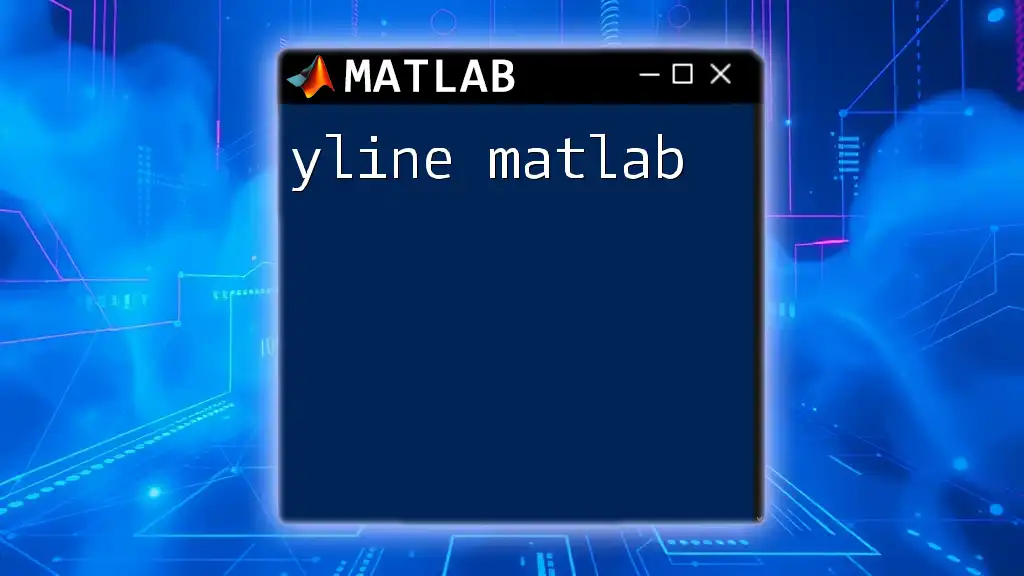
Call to Action
We encourage you to explore the `int` command in your MATLAB projects and share your experiences. Whether you encounter challenges or have success stories to tell, we would love to hear your thoughts and questions!
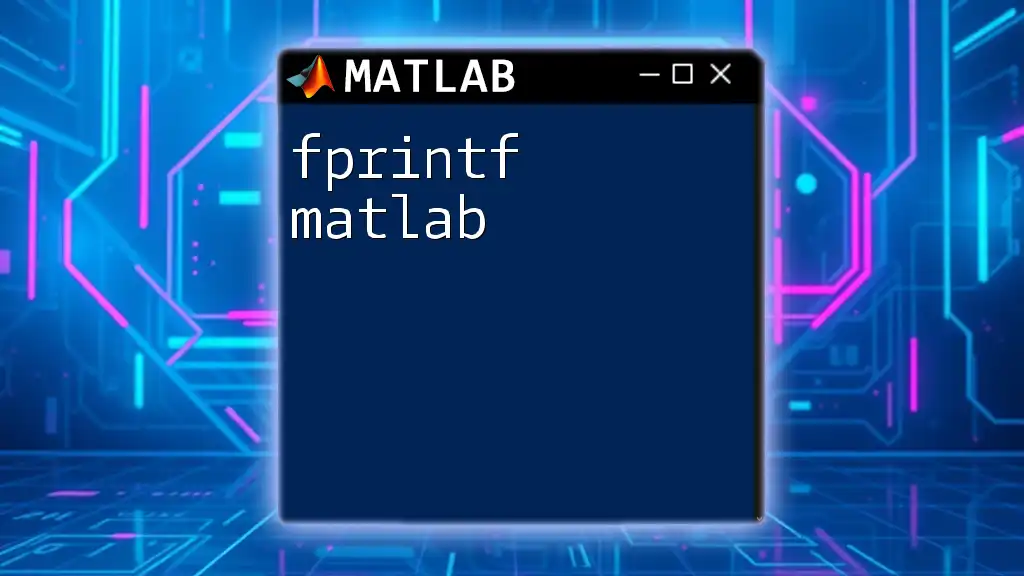
FAQs
What is the difference between `int` and `integrate` in MATLAB?
While both `int` and `integrate` often yield similar results in MATLAB, `int` is more commonly used in symbolic integration. For numerical integration, you might come across functions like `integral`.
Can `int` handle numerical integration?
The `int` command is primarily for symbolic integration. For numerical integration, MATLAB offers other commands like `integral` or `trapz`. However, once a symbolic expression has been integrated, you can convert it to numeric form for further calculations.