The `dict` in MATLAB refers to the creation of a dictionary or map object that stores key-value pairs, enabling efficient data retrieval.
% Create a containers.Map object as a dictionary
dict = containers.Map({'key1', 'key2', 'key3'}, {100, 200, 300});
% Access value associated with 'key2'
value = dict('key2'); % Returns 200
Understanding Dictionaries in MATLAB
What Makes Up a Dictionary?
A dictionary in MATLAB is fundamentally comprised of key-value pairs. This structure allows you to store data in a way that associates unique keys with specific values. Each key in a MATLAB dictionary must be unique, enabling efficient data retrieval and management.
Types of Dictionaries in MATLAB
MATLAB provides a built-in class, `containers.Map`, which serves as the primary means for creating dictionary-like structures. Unlike standard arrays, `containers.Map` offers flexibility in data storage and retrieval, allowing you to utilize various data types as keys and values.

Creating a Dictionary in MATLAB
Syntax and Basic Structure
Creating a dictionary in MATLAB is straightforward. The basic syntax revolves around the `containers.Map` class. For instance, to initiate a simple dictionary, you can use the following code:
myDict = containers.Map({'One', 'Two', 'Three'}, {1, 2, 3});
In this example, the dictionary `myDict` is initialized with three key-value pairs: 'One' is associated with 1, 'Two' with 2, and 'Three' with 3. This structure allows you to store and quickly access data using the keys.
Adding and Removing Items
Adding elements to a dictionary is as simple as assigning a value to a new key. Here’s how you can add a new key-value pair:
myDict('Four') = 4; % Adds a new key-value pair
Conversely, removing an item is equally straightforward. Use the `remove` function to delete a specific key:
remove(myDict, 'Two'); % Removes the key 'Two'
It’s crucial to ensure that the key you want to remove exists in the dictionary; otherwise, MATLAB will return an error.
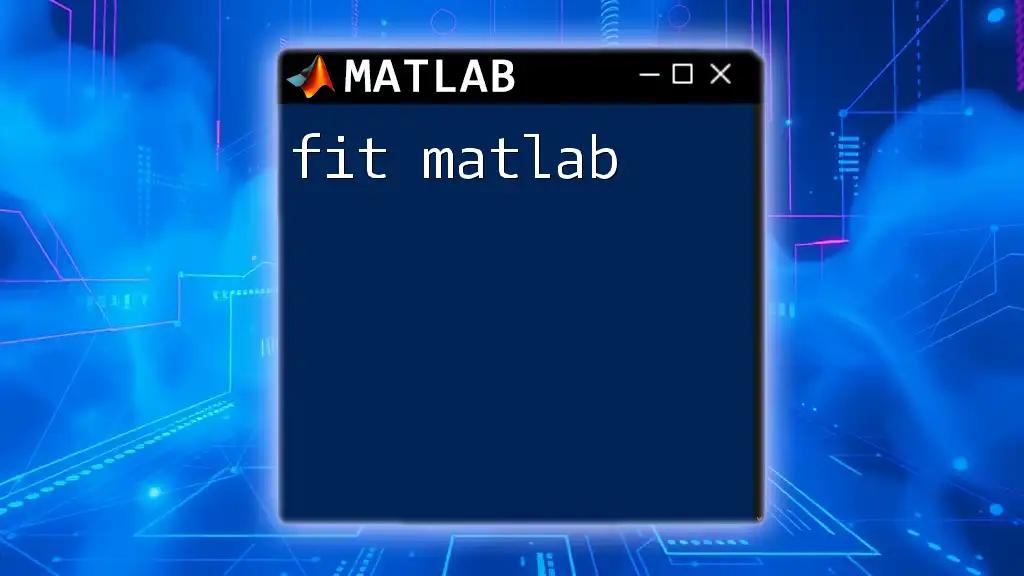
Accessing Dictionary Elements
Retrieving Values
One of the significant advantages of using dictionaries is the ease of accessing values via their keys. To retrieve a value, you can use the following syntax:
value = myDict('One'); % Output: 1
This command will fetch the value associated with the key 'One', in this case, returning 1.
Checking for Keys
You may often need to check whether a particular key exists in the dictionary before attempting to retrieve its value. This can be efficiently done using the `isKey` function:
exists = isKey(myDict, 'Three'); % Checks if 'Three' exists
By using this function, you can prevent potential errors that arise from trying to access a non-existent key.

Modifying Dictionary Elements
Updating Values
If you need to update a value associated with an existing key, simply assign a new value to that key:
myDict('One') = 10; % Updates the value for key 'One'
This code snippet changes the value associated with 'One' from 1 to 10.

Practical Applications of Dictionaries in MATLAB
Use Case Scenarios
Dictionaries can be exceptionally useful in various scenarios. One common application is storing configurations for scripts or functions. By storing options in a dictionary, you can easily access and modify settings without needing to change the core logic of your code.
Another scenario involves mapping input data to output results. For example, you can efficiently associate user inputs with the corresponding outputs by leveraging keyed access.
Real-world Example
To illustrate how dictionaries can be used in a practical context, let’s build a simple lookup tool. This function will allow users to retrieve values based on their input keys:
function value = lookup(dict, key)
if isKey(dict, key)
value = dict(key);
else
value = 'Key not found';
end
end
This function first checks if the specified key exists in the dictionary. If it does, it returns the associated value; otherwise, it alerts the user that the key was not found.
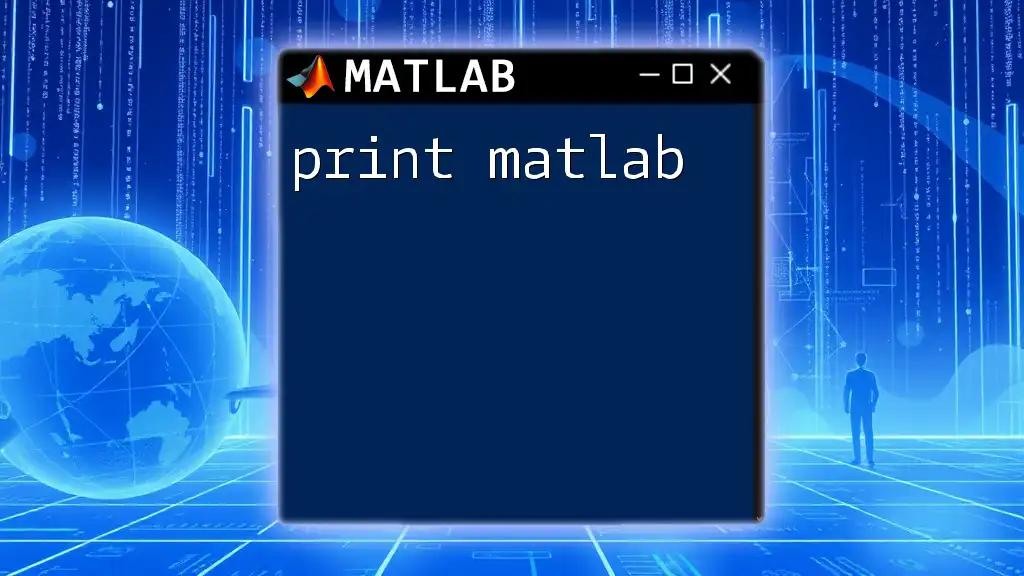
Best Practices for Using Dictionaries in MATLAB
Performance Considerations
When using dictionaries, it's essential to consider performance, especially when dealing with large datasets. `containers.Map` provides efficient access times for large collections of data compared to traditional arrays or structures.
When to Use and When Not to Use
To decide if a dictionary is the right choice, consider the structure of your data. If you need to frequently access and modify data using unique identifiers, dictionaries shine. However, if your tasks involve simple numerical computations or matrix operations, arrays may suffice.
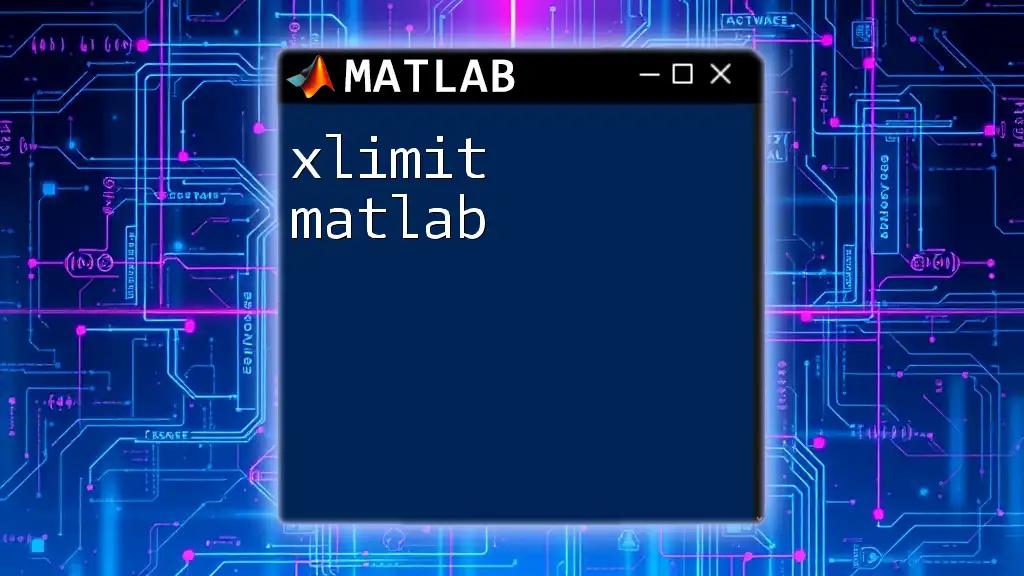
Common Issues and Troubleshooting
Error Handling
One common error arises when attempting to access a key that does not exist in the dictionary. To avoid this, always check for key existence with the `isKey` function before trying to retrieve the value.
Performance Optimization
To enhance the efficiency of dictionary access, keep the number of elements manageable and leverage the uniqueness of keys effectively to minimize look-up times.

Conclusion
Dictionaries in MATLAB, particularly through `containers.Map`, offer a powerful way to manage and manipulate data using key-value pairs. Their flexibility and efficiency make them invaluable for specific tasks in MATLAB programming. By understanding the fundamentals of using dictionaries, you can improve your coding practices and streamline your workflows.
Further Reading and Resources
For additional insights and advanced use cases, consult the [official MATLAB documentation](https://www.mathworks.com/help/matlab/ref/containers.map-class.html) and explore further resources on optimized data structures.
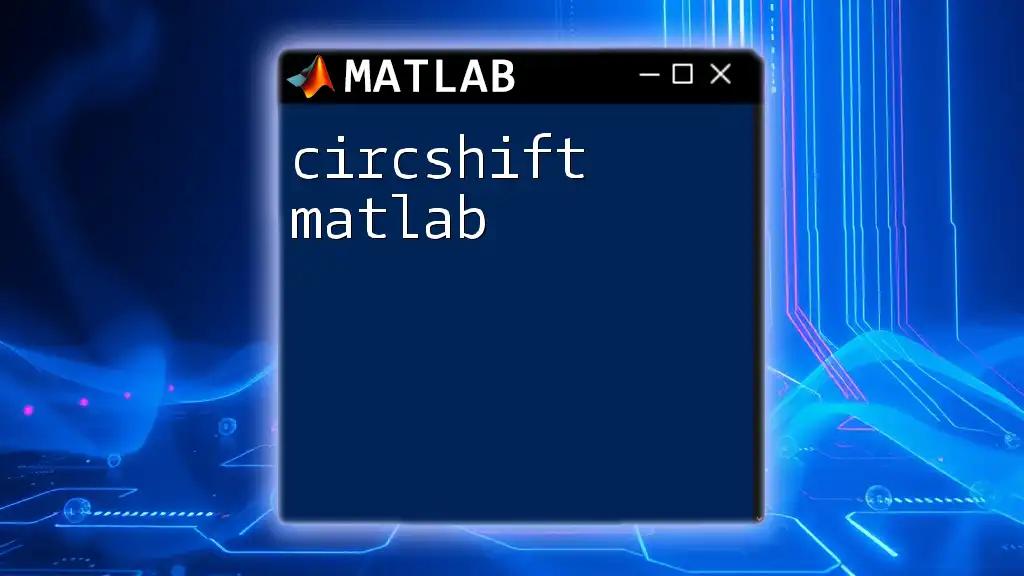
Call to Action
Ready to master MATLAB? Join our learning community and subscribe for more tips and lessons on using MATLAB commands effectively!