The `dir` command in MATLAB lists the files and folders in the current directory or a specified directory.
files = dir; % Lists all files and folders in the current directory
Understanding the `dir` Command
What is the `dir` Command?
The `dir` function in MATLAB serves as a powerful tool for accessing file and folder information from the current directory. This command not only allows users to view what files are available but also provides details such as file size, modification date, and whether the item is a directory. Understanding how to use `dir` effectively is essential for anyone managing multiple files or datasets.
Syntax of `dir`
The basic syntax for the `dir` command is fairly straightforward:
dir('filename');
In this command, you can specify a filename or use wildcards to match multiple files. This flexibility makes `dir` a versatile command for file management tasks.
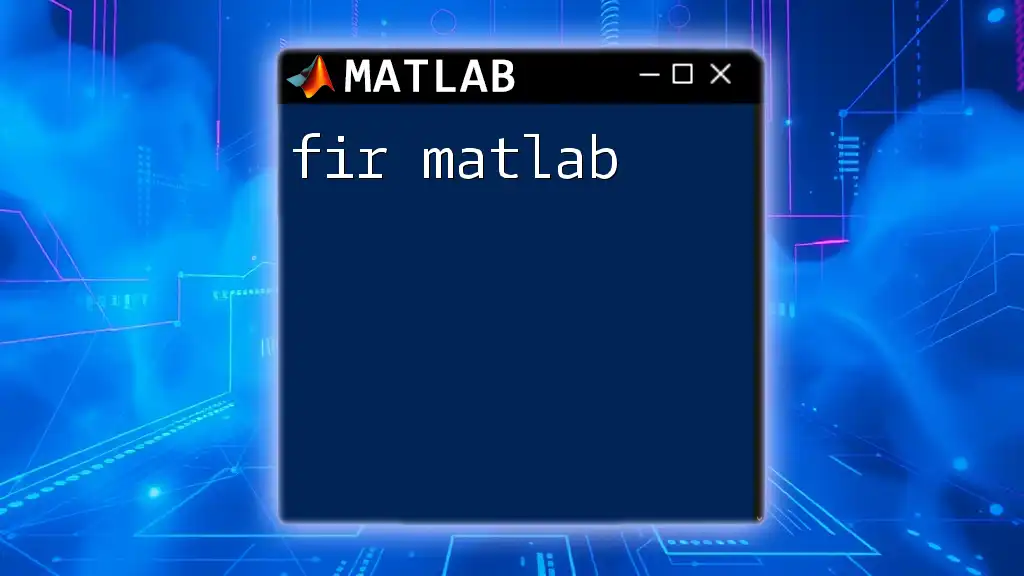
Basic Usage of `dir`
Listing Files and Folders
When you call `dir` without any arguments, it lists all the files and folders in the current working directory:
files = dir;
disp(files);
The output is a structured array that includes fields such as `name`, `date`, `bytes`, and `isdir`. Each entry corresponds to a file or folder, providing a snapshot of your directory’s content.
Specifying File Types
Using Wildcards
Wildcards allow for more specific queries. For instance, if you want to list all text files in your directory, you can use:
txtFiles = dir('*.txt');
This command will return only files that match the pattern (in this case, all `.txt` files). After executing, you can examine the output to see the names and attributes of the captured files, allowing for focused data handling.
Filtering Specific Files
The ability to filter files by type can streamline your workflow. To retrieve all JPEG files, simply use the following code:
jpgFiles = dir('*.jpg');
fprintf('JPEG files:\n');
for k = 1:length(jpgFiles)
disp(jpgFiles(k).name);
end
This approach not only extracts relevant files but also displays them conveniently.
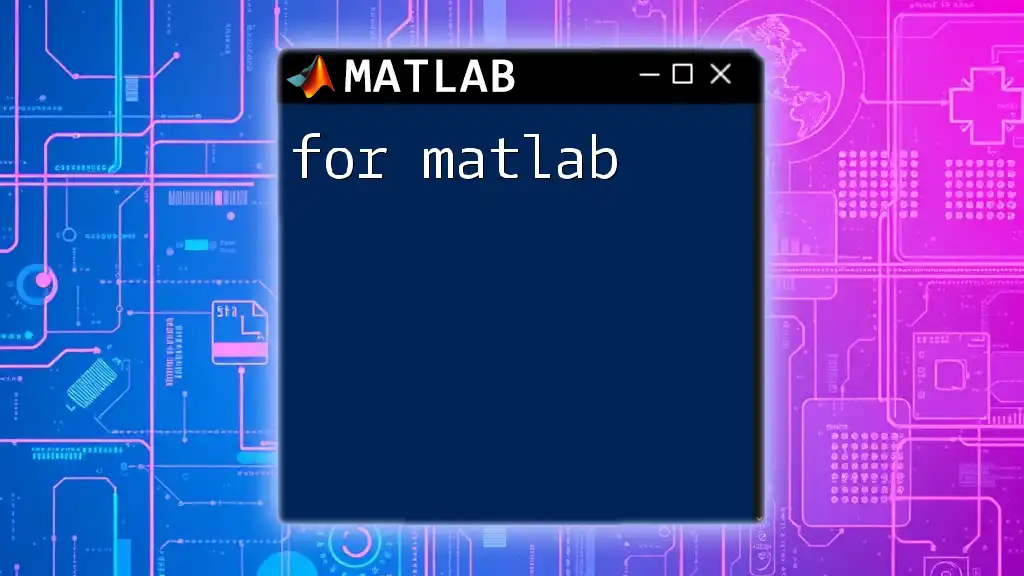
Advanced Usage of `dir`
Getting Directory Information
The `dir` command can also provide detailed information about a specific folder. For example:
folderInfo = dir('myFolder');
This command will generate a structured output of all files and subdirectories within myFolder, offering insights into its contents.
Retrieving Files Recursively
If your project involves subdirectories, retrieving files recursively can be crucial. You can achieve this by using:
allFiles = dir(fullfile('myFolder', '**', '*.*'));
This command will list all files within `myFolder` and any of its subdirectories. This capability is invaluable for complex file structures where data might be distributed across multiple locations.
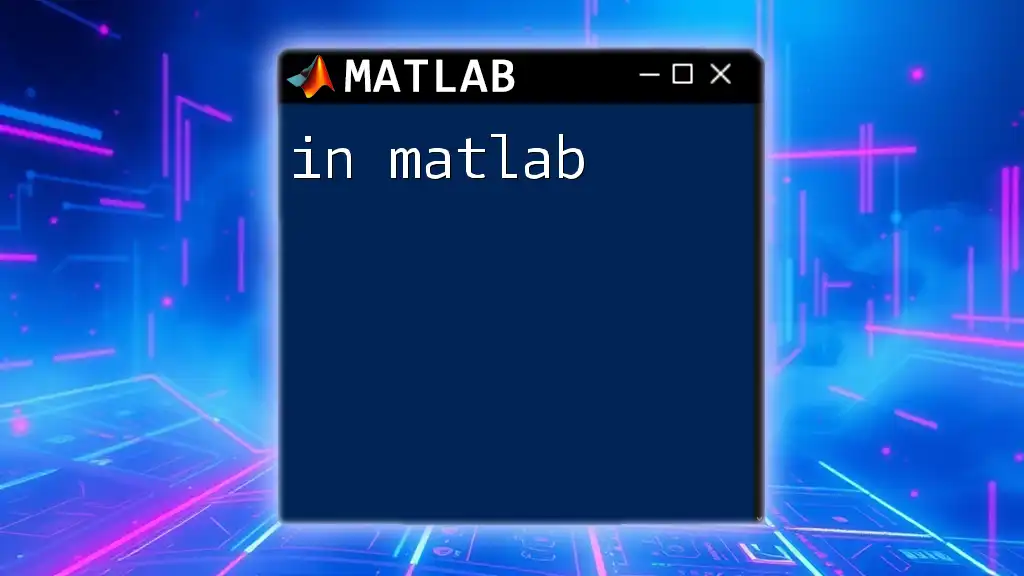
Common Use Cases of `dir`
Organizing Data Files
In research and engineering, keeping track of various data files is essential. Using `dir`, you can easily check for the presence of crucial data files, thus enhancing your workflow. For instance:
if isempty(dir('*.mat'))
warning('No MAT files found in the current directory.');
end
This snippet helps ensure that the expected files are available before proceeding with any analyses.
File Manipulation
The `dir` command often acts as a foundational tool in scripts that involve file manipulation. By checking for specific file types, you can conditionally proceed with data processing tasks:
if ~isempty(dir('*.csv'))
data = readtable('data.csv');
else
error('Expected CSV file not found.');
end
This allows for more robust and error-free scripts.
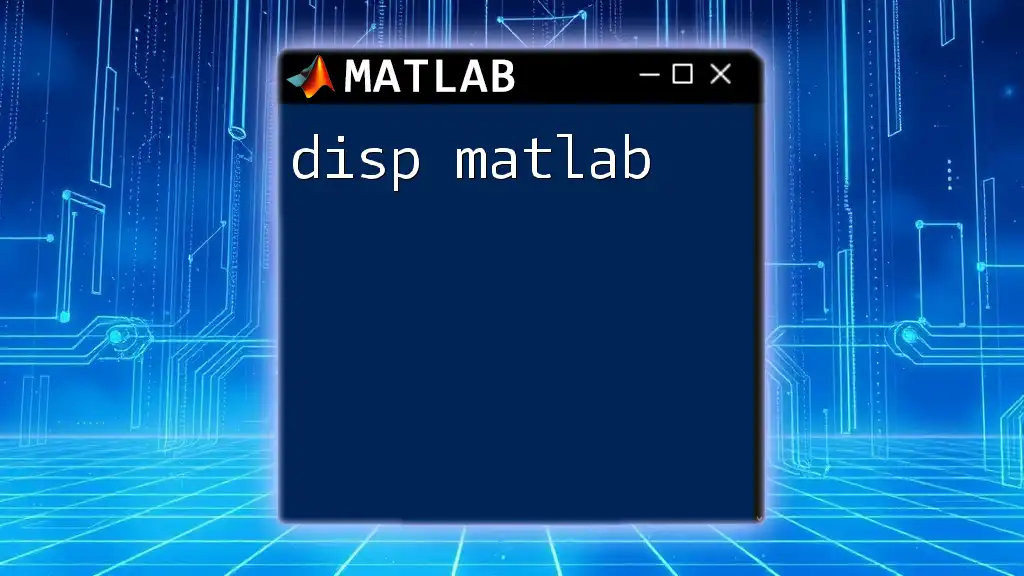
Working with `dir` Output
Understanding the Output Structure
The output of the `dir` command is a structure containing essential fields. Each entry includes:
- name: The name of the file or directory.
- date: The last modified date of the file or directory.
- bytes: The size of the file in bytes.
- isdir: A logical value indicating if the entry is a directory.
To illustrate how to navigate through this data, consider the following example:
d = dir;
for k = 1:length(d)
fprintf('File: %s, Size: %d bytes, Is Directory: %d\n', ...
d(k).name, d(k).bytes, d(k).isdir);
end
This code will print out a user-friendly list of files and directories within your current path.
Filtering and Sorting Data
Sorting by Date
Sorting files by their modification date can help you easily access the most relevant data. The following code snippet demonstrates how to accomplish this:
[~, idx] = sort([d.date]);
sortedFiles = d(idx);
After sorting, you can print out the files in chronological order, making it simple to find the latest entries.
Filtering Based on File Size
In some cases, you may want to filter files based on their size. For example, to find files larger than 1KB, you can use:
largeFiles = d([d.bytes] > 1000); % files larger than 1KB
This command effectively narrows down the list to those files that meet your criteria.
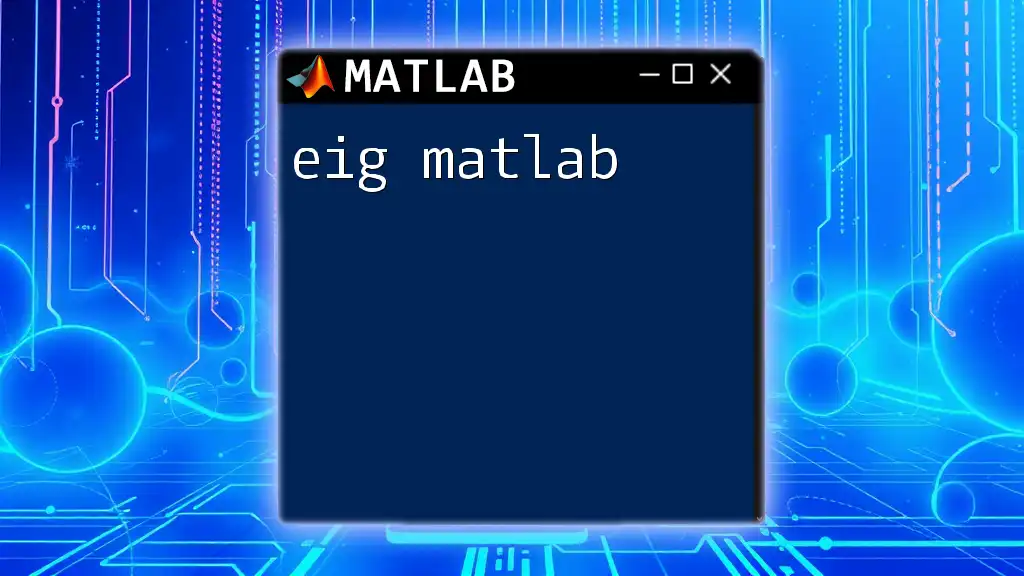
Common Errors and Troubleshooting
Handling Empty Outputs
It’s important to gracefully handle the cases when the `dir` command returns an empty array. For instance:
if isempty(dir('*.xyz'))
disp('No files match the pattern.');
end
This ensures users are informed if their search yields no results.
Accessing Non-existent Directories
Before using `dir` on a specified directory, it's good practice to check whether the directory exists. This can prevent runtime errors:
if exist('myFolder', 'dir') == 7
files = dir('myFolder');
else
disp('Directory does not exist.');
end
This safeguard contributes to more robust and user-friendly scripts.
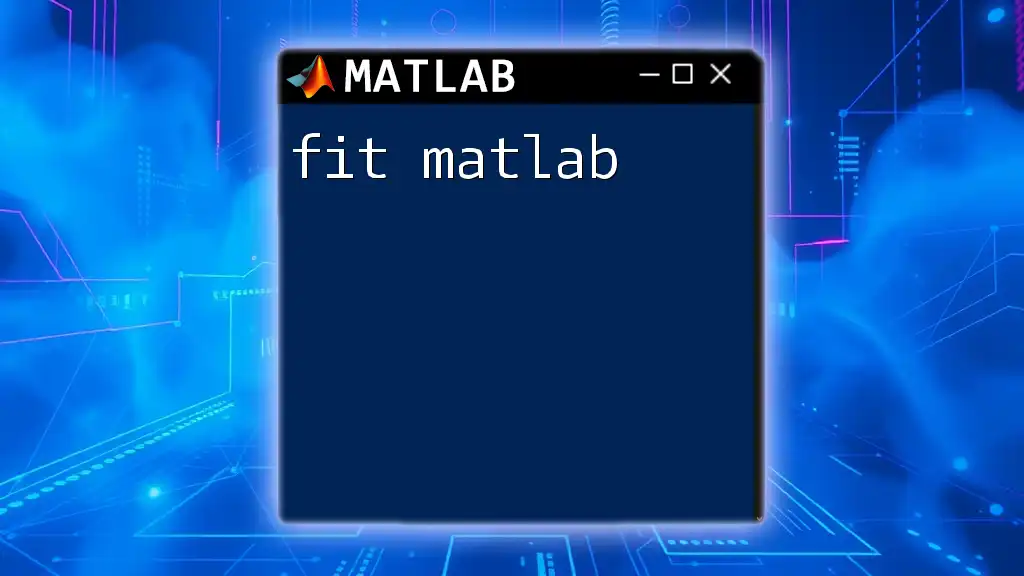
Conclusion
Understanding the capabilities of the `dir` command in MATLAB is essential for efficient file management. From listing files to filtering and sorting them, `dir` provides invaluable functionalities that enhance your workflow. By mastering this command, you can simplify your coding projects and improve your productivity in navigating through directories.