The `ode45` function in MATLAB is used to solve ordinary differential equations (ODEs) using a variable-step, variable-order Runge-Kutta method, ideal for non-stiff problems.
Here’s a code snippet that demonstrates how to use `ode45` to solve a simple ODE:
% Define the ODE as a function
function dydt = myODE(t, y)
dydt = -2 * y; % Example ODE: dy/dt = -2y
end
% Initial conditions
y0 = 1; % Initial value
tspan = [0 5]; % Time interval
% Solve the ODE using ode45
[t, y] = ode45(@myODE, tspan, y0);
% Plot the results
plot(t, y);
xlabel('Time');
ylabel('y');
title('Solution of ODE using ode45');
What is ODE45?
`ode45` is a powerful function in MATLAB designed to solve Ordinary Differential Equations (ODEs) using an adaptive step size method based on the Dormand-Prince algorithm. This function is particularly useful for solving non-stiff ODEs, which makes it a go-to choice for many engineers and researchers.
When dealing with ODEs, you might wonder when to use `ode45` versus other solvers such as `ode23` or `ode15s`. While `ode23` uses a lower-order method and may be faster for simple problems, `ode45` generally offers greater accuracy and efficiency for a broader range of applications, particularly when precise solutions are required over complex intervals.

Getting Started with ODE45
Setting Up Your MATLAB Environment
Before diving into coding, ensure you have a proper setup:
- Requirements: Make sure you have MATLAB installed, along with the necessary toolboxes (most standard installations include these).
- Installation Guide: Follow the installation procedure provided by MathWorks to set up the environment effectively.
Once set up, you can open MATLAB's command window, where you'll perform the coding and debugging needed for your ODE solutions.
Basic Syntax of ODE45
The basic syntax for using `ode45` is structured as follows:
[t, y] = ode45(@(t, y) odeFunc(t, y), tspan, y0);
- `@` refers to the function handle, allowing you to specify the ODE function to be solved.
- `odeFunc` represents the user-defined function containing the ODE definition.
- `tspan` defines the interval of integration, while `y0` specifies the initial conditions.
Understanding this structure is crucial as it sets the stage for effective ODE solving.

Step-by-Step Guide to Using ODE45
Defining the ODE
In MATLAB, defining your ODE is straightforward. Let's start with a common first-order ODE:
\[ y' = -2y \]
To implement this in MATLAB, you would define a function like so:
function dydt = odeFunc(t, y)
dydt = -2 * y;
end
Here, `dydt` represents the derivative of `y` with respect to `t`. This function reflects how `y` changes over time.
Specifying the Time Span and Initial Conditions
Next, you need to specify the interval over which to solve the ODE (the `tspan`) and the initial conditions (`y0`). For our example, let's assume we want to solve the equation from \( t = 0 \) to \( t = 5 \) with the initial condition \( y(0) = 1 \):
tspan = [0 5];
y0 = 1;
This will guide `ode45` in determining how to approach the solution during its calculations.
Running the ODE Solver
Once you have your function set up and your parameters defined, it's time to call the `ode45` function:
[t, y] = ode45(@odeFunc, tspan, y0);
This line executes the solving process, with the output variables `t` and `y` storing the time points and corresponding solutions, respectively.
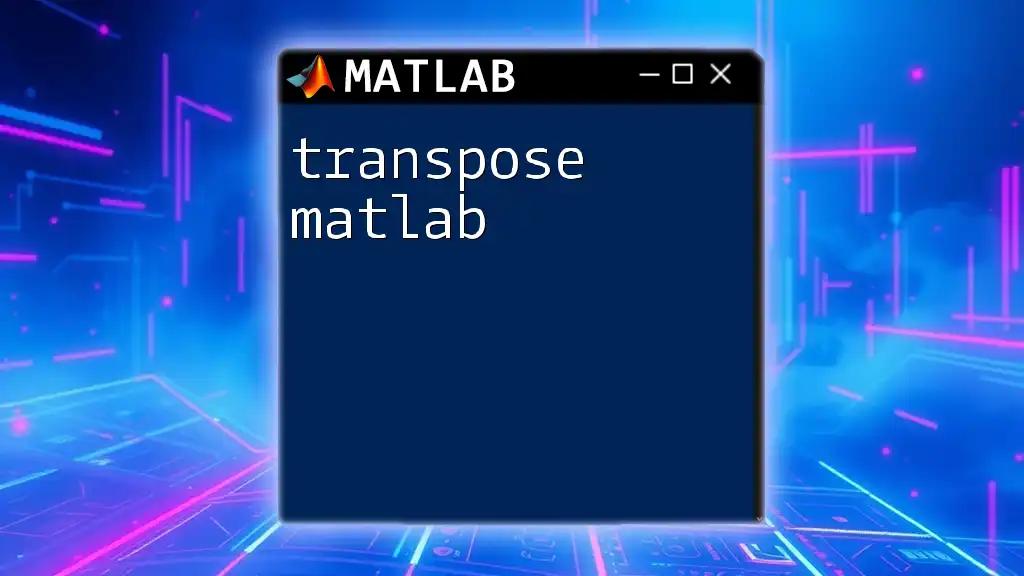
Analyzing the Results
Plotting the Results
Visualizing the results is crucial to understanding the solution's behavior. Using MATLAB's plotting capabilities, you can easily create a graph of your results:
plot(t, y)
xlabel('Time')
ylabel('Solution y(t)')
title('Solution of the ODE using ode45')
The graph will show how the variable `y` changes over time, providing visual insights into the dynamics of your differential equation.
Interpreting the Solution
When interpreting the graph, look for key behaviors such as:
- The general trend of the solution (ascending, descending, constant).
- The impact of initial conditions; for instance, changing `y0` to a larger or smaller value will shift the curve vertically.
Understanding these dynamics is essential, as they can have significant implications in real-world applications.

Advanced Usage of ODE45
Handling Systems of ODEs
`ode45` can also be used to solve systems of ODEs. For example, consider a system defined by two first-order equations:
\[ y_1' = y_2 \] \[ y_2' = -y_1 - 0.1y_2 \]
To implement this in MATLAB, you would define a function that returns both derivatives:
function dydt = odeSystem(t, y)
dydt = [y(2); -y(1) - 0.1 * y(2)];
end
You would then define your initial conditions and time span similarly to the previous example, enabling `ode45` to work through both equations simultaneously.
Adding Parameters to the ODE Function
Introducing parameters into your ODE function can increase flexibility and applicability. For instance, if you wish to include a variable parameter `param`, you could structure your function as follows:
function dydt = odeWithParams(t, y, param)
dydt = param * y;
end
Subsequently, when calling `ode45`, you would have to utilize an anonymous function to pass this parameter:
param = 2;
[t, y] = ode45(@(t, y) odeWithParams(t, y, param), tspan, y0);
This feature allows for greater control and versatility in modeling complex systems.
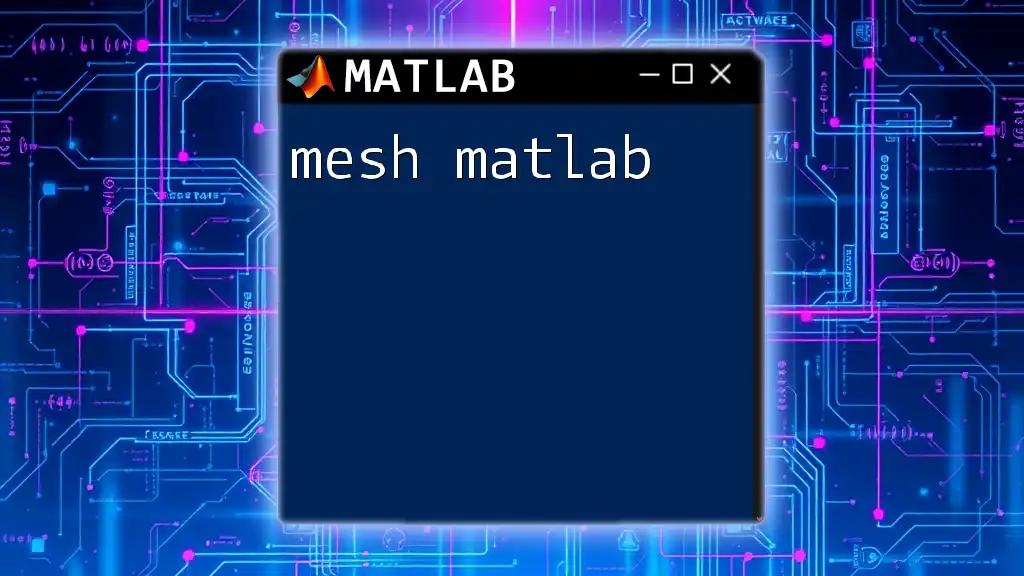
Common Issues and Troubleshooting
Common Error Messages
As with any programming task, you may encounter errors when using `ode45`. Common issues include:
- Incompatible dimensions: Ensure that your input vectors/parameters match expected formats.
- Singularity or complex solution: Verify that your function doesn't lead to undefined values or complex numbers.
Performance Considerations
When dealing with larger systems or more complex equations, you might find that `ode45` can become slow. In such cases, consider alternative ODE solvers like `ode23`, which can be computationally less intensive for simpler problems, or `ode15s` for stiff problems where solutions change rapidly.

Conclusion
In summary, mastering `ode45` in MATLAB unlocks the potential to solve a wide array of ODEs effectively. By grasping its syntax, exploring basic and advanced usage, and understanding how to analyze results, you will be well-equipped to tackle various challenges involving differential equations.
Feel free to enhance the content further by incorporating examples specific to your audience's interests, providing additional insights into real-world applications, or exploring complex scenarios with unique boundary conditions!