The `rem` function in MATLAB computes the remainder after division of two numbers and is commonly used for modular arithmetic in mathematical operations.
% Example of using the rem function
result = rem(10, 3); % This will return 1, since 10 divided by 3 leaves a remainder of 1
What is the `rem` Function?
The `rem` function in MATLAB is utilized to calculate the remainder after division of two numbers, making it a crucial tool for various mathematical operations. Operating within the realm of numerical computing, this function is widely used in algorithms where finding the remainder is necessary, such as in cyclic processes, modular arithmetic, and optimizing code efficiency.

Understanding the `rem` Function
Syntax of the `rem` Function
The basic syntax for the `rem` function is as follows:
R = rem(A, B)
In this syntax:
- A is the dividend, the number you are dividing.
- B is the divisor, the number by which you are dividing.
- R will hold the remainder resulting from the division.
Key Characteristics
-
Return Value: The `rem` function returns the remainder, which retains the same data type as the input. This means, whether you're dividing integers or floating-point numbers, the result will align accordingly.
-
Behavior with Different Data Types: The `rem` function can accept both scalar values and arrays, allowing for versatile applications in complex mathematical computations.
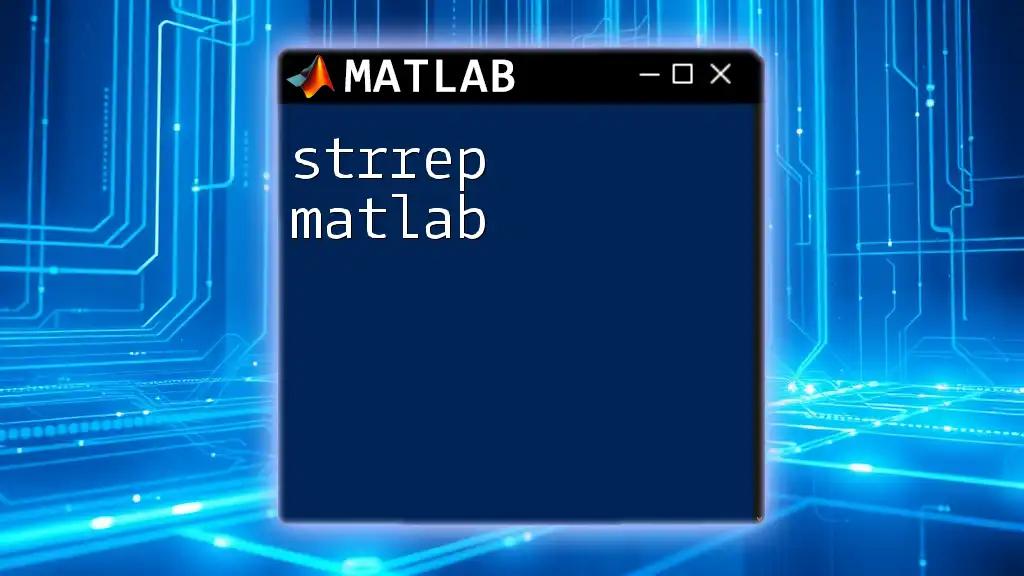
Examples and Practical Use Cases
Simple Examples
Example 1: Basic Usage
To understand how `rem` works, consider the following simple expression:
result = rem(10, 3);
disp(result); % Output: 1
In this code, 10 divided by 3 leaves a remainder of 1. Therefore, when we call `rem(10, 3)`, MATLAB returns 1, which highlights the succinctness of the function for basic arithmetic operations.
Example 2: Working with Negative Numbers
The handling of negative numbers in the `rem` function can lead to intriguing results:
result = rem(-10, 3);
disp(result); % Output: 2
Here, the dividend is negative. The result is 2, which is the remainder when -10 is divided by 3. Notably, the remainder takes the sign of the dividend, which differentiates `rem` from some other functions.
Advanced Examples
Example 3: Arrays
The versatility of the `rem` function shines especially when dealing with arrays:
A = [10, 20, 30];
B = 7;
results = rem(A, B);
disp(results); % Output: [3, 6, 2]
In this case, applying `rem` to the array A allows us to compute the remainder of each element when divided by B all at once. This demonstrates the efficiency of MATLAB for batch processing data.
Example 4: Remainder in Cyclic Applications
The `rem` function becomes particularly useful in cyclic scenarios. For instance, consider a case where we want to assign numbers cyclically:
% Cyclically assigning numbers within a range
N = 15; % Total numbers
M = 7; % Cycle length
result = rem(0:N-1, M);
disp(result); % Output: 0 1 2 3 4 5 6 0 1 2 3 4 5 6 0
This example shows how we can efficiently manage data in cycles, where the function helps track positions in a repeating structure.
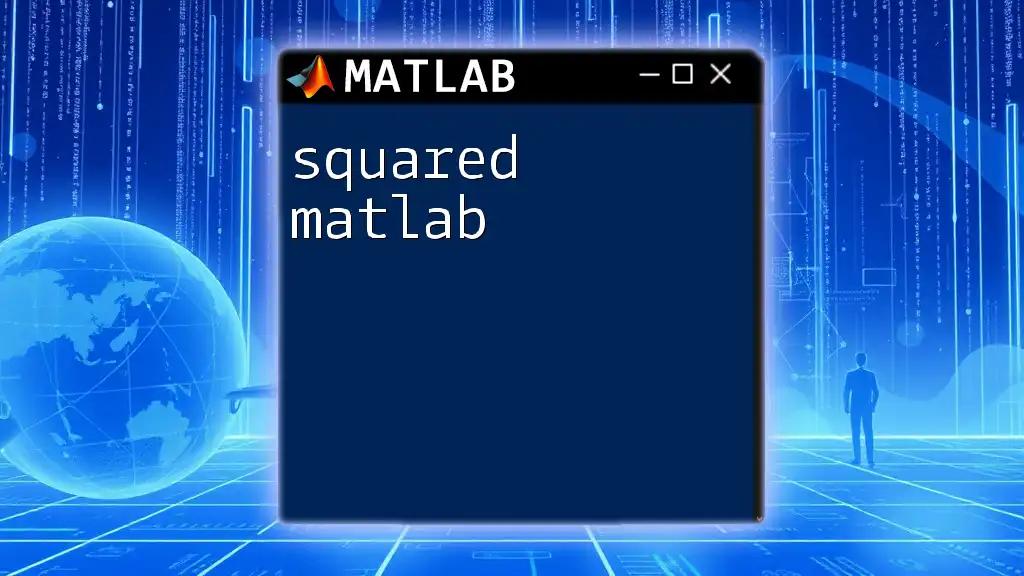
Comparison with Similar Functions
`mod` vs `rem`
When discussing remainders, it is essential to distinguish between the `mod` and `rem` functions.
- Key Differences: The principal distinction between `rem` and `mod` lies in how they treat negative dividends. The `rem` function returns a remainder that shares the sign of the dividend, whereas the `mod` function always yields a non-negative remainder.
Examples for Differentiation
Here’s a practical illustration of the difference:
disp(rem(-10, 3)); % Output: 2
disp(mod(-10, 3)); % Output: 1
The output here clarifies that `rem` keeps the sign of the dividend, providing users with insight that may be crucial for specific mathematical applications.
When to Use `rem` Over `mod`
Choose `rem` when the sign of the result is relevant to your calculations, especially in applications involving modular arithmetic or functions where negative values are common.

Common Errors and Troubleshooting
Common Mistakes with the `rem` Function
Users often encounter pitfalls when using `rem`, such as trying to apply it to non-scalar inputs incorrectly or overlooking data type mismatches.
To avoid these errors, ensure that:
- You are working with compatible numeric types.
- Your input values adhere to expected vector or array formats when dealing with multiple computations.
Error Handling
A prudent approach to debugging involves:
- Inspecting input data types with `class()` or `size()`.
- Checking for unexpected values or matrix dimensions that could lead to errors.
- Using MATLAB's documentation for further clarification on function behavior and expected input types.
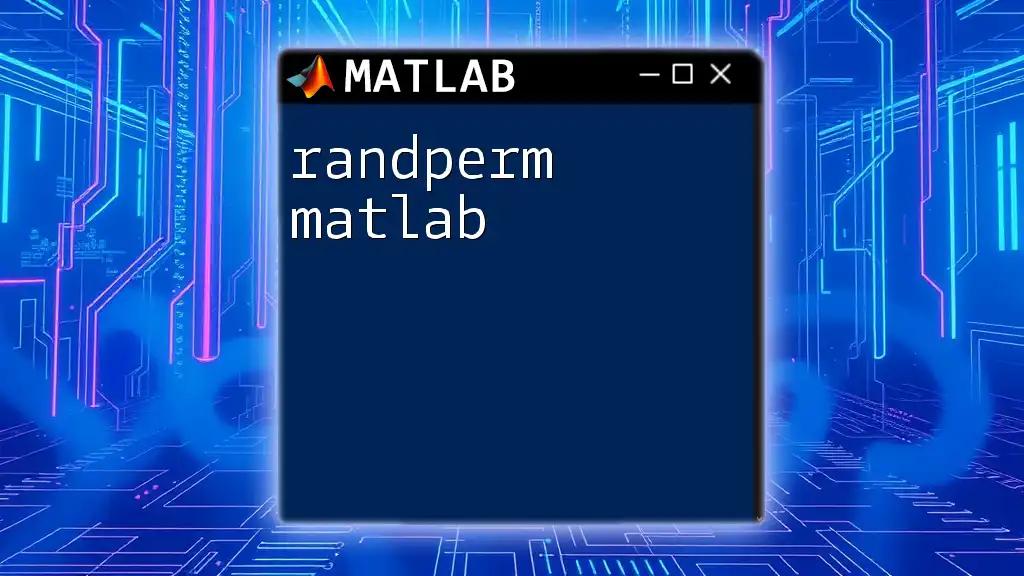
Conclusion
In conclusion, the `rem` function in MATLAB serves as a powerful tool for performing remainder calculations with a multitude of applications. From basic arithmetic to advanced programming concepts, understanding and mastering `rem` can significantly enhance your MATLAB coding experience.
I encourage you to practice using the `rem` function within your own projects, as hands-on experience will solidify your understanding and proficiency.
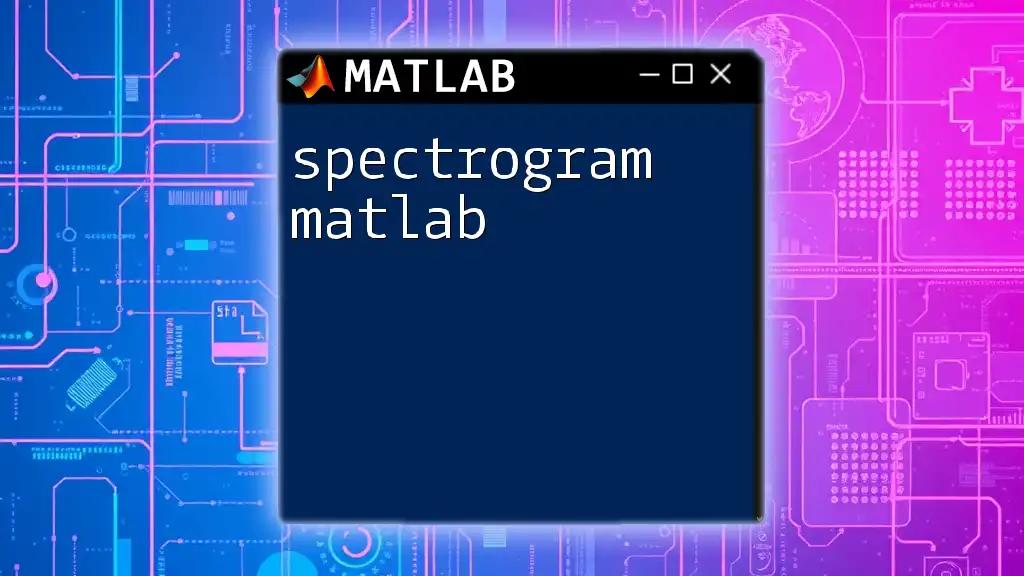
Additional Resources
For those looking to dive deeper into MATLAB programming and the `rem` function:
- MATLAB Documentation on `rem`: A thorough resource for understanding all function nuances.
- Online MATLAB Tutorials and Courses: Providing structured learning paths for continued growth.
By regularly practicing and experimenting with the `rem` function, you'll find it becoming an integral part of your MATLAB toolkit, empowering you to write more efficient and clear code.