The `fliplr` function in MATLAB flips a matrix from left to right, effectively reversing the order of its columns.
Here's a code snippet demonstrating its use:
A = [1 2 3; 4 5 6; 7 8 9];
B = fliplr(A);
disp(B);
In this example, the matrix `A` is flipped horizontally to produce `B`, which will be:
3 2 1
6 5 4
9 8 7
Understanding the `fliplr` Function
What is `fliplr`?
`fliplr` is a built-in MATLAB function that stands for flip left to right. This function is particularly useful when you need to reverse the columns of a matrix or an array. Flipping matrices is a common operation in data manipulation, often utilized in image processing or numerical analysis, where the orientation of data may need to be adjusted for better clarity or alignment with other datasets.
The Syntax of `fliplr`
The basic syntax of the `fliplr` function is straightforward:
B = fliplr(A)
In this syntax:
- A is the input matrix or array that you want to flip.
- B is the output matrix, which will contain the columns of A reversed.
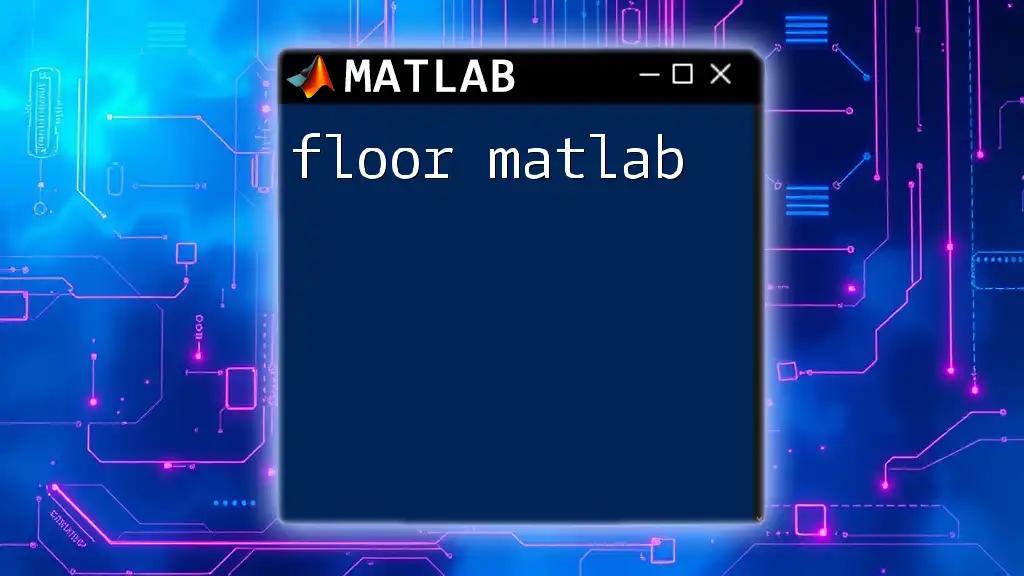
How `fliplr` Works
Flipping Left to Right
When you apply the `fliplr` function to a matrix, each row of the matrix is flipped horizontally. This means that the first column becomes the last column, the second column becomes the second-to-last column, and so on.
For example, if you have a matrix defined as:
A = [1 2 3; 4 5 6; 7 8 9];
Using the `fliplr` function:
B = fliplr(A);
disp(B);
The output will be:
3 2 1
6 5 4
9 8 7
This result clearly shows how the columns have been reversed.
Example: Flipping a Matrix
Consider the earlier defined matrix A:
A = [1 2 3; 4 5 6; 7 8 9];
B = fliplr(A);
disp(B);
This code snippet illustrates the use of `fliplr` effectively. The function operates row-wise, maintaining the integrity of individual rows while reorganizing the order of columns. The flipped output allows for a different perspective when analyzing the data.
Visual Representation
Visualizing how `fliplr` flips your data can significantly enhance understanding. Imagine each row as a series of columns that represents values. When you flip them, you reverse their positions, providing a new orientation that may reveal different insights or relationships between data points.
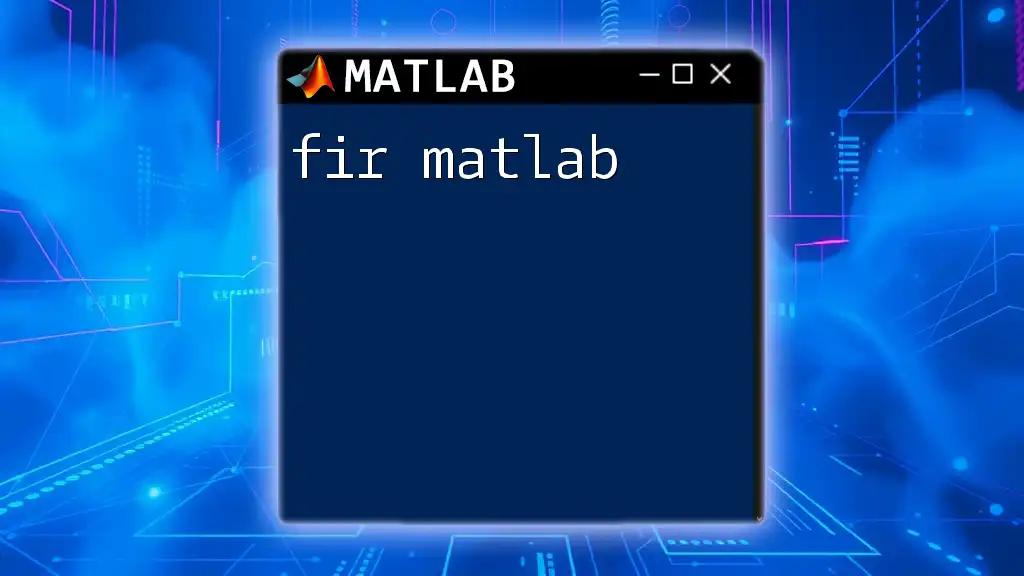
Common Use Cases for `fliplr`
Data Preprocessing
In data preprocessing, it is often necessary to adjust the arrangement of your data. For instance, when working with images, flipping them left to right can provide insights into patterns and anomalies that are not immediately visible. This is especially true in machine learning applications where dataset orientation can impact training results.
Data Analysis
Flipping arrays can also benefit statistical analyses. When comparing datasets, `fliplr` may allow practitioners to align similar patterns for thorough comparison. Consider the following example:
data = [5 10 15; 20 25 30];
flipped_data = fliplr(data);
Here, the output will be:
15 10 5
30 25 20
In this scenario, flipping the data can facilitate better insights in comparative analysis, revealing trends and relationships obscured by orientation.
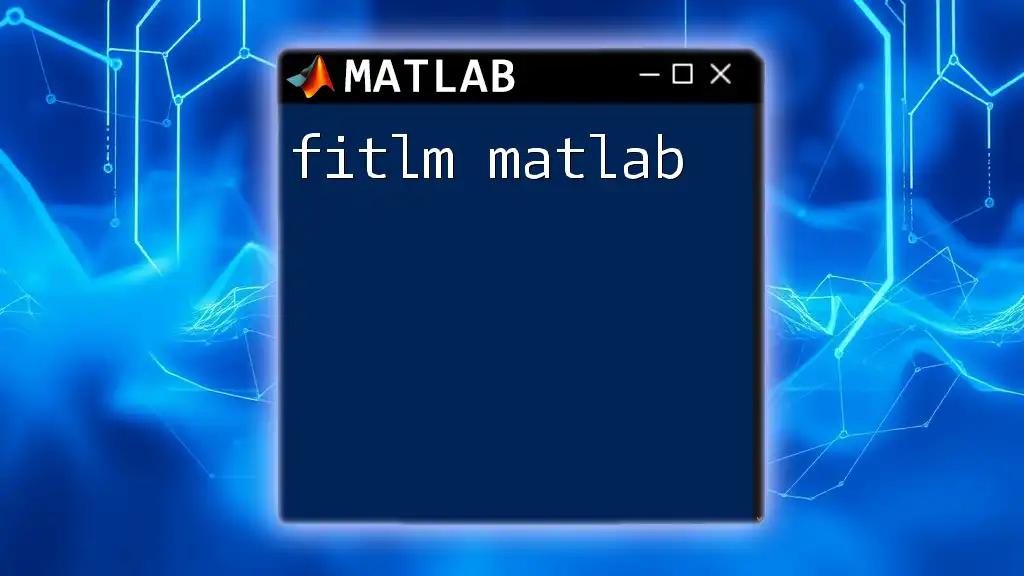
Best Practices for Using `fliplr`
When to Use `fliplr`
Understanding when to apply `fliplr` is crucial. Use this function:
- When working with images that require manipulation along the horizontal axis.
- In scenarios needing realignment of datasets to facilitate comparison.
- During preprocessing stages to prepare your dataset for further analysis.
Performance Considerations
Always consider the size of your array or matrix when using `fliplr`. While it efficiently handles traditional-sized matrices, performance may decline with extraordinarily large datasets. Always conduct performance checks to ensure that flipping operations do not bottleneck your workflow.
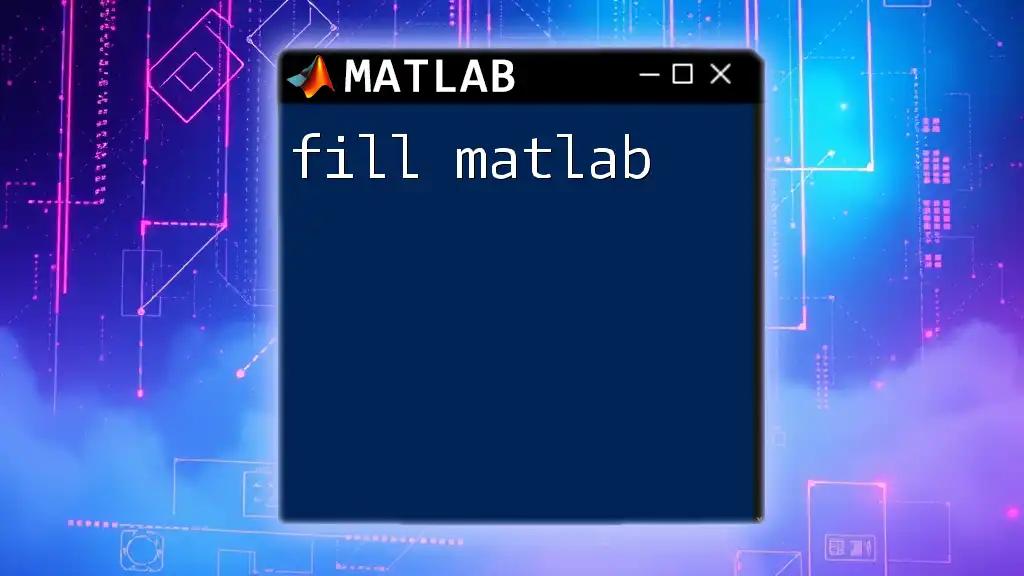
Common Mistakes to Avoid
Understanding Dimensions
A common mistake is not being cognizant of a matrix's dimensions. The `fliplr` function can only operate on 2D matrices. If you mistakenly apply it to a 1D vector, MATLAB will return an unexpected result. Understanding the shape of your data is paramount.
Misinterpretation of Results
Misinterpretation of the flipped data can lead to incorrect conclusions. It is essential to reassess how data orientation influences your analysis and methodologies. Always visualize or print output to ensure the flipping has performed as intended.
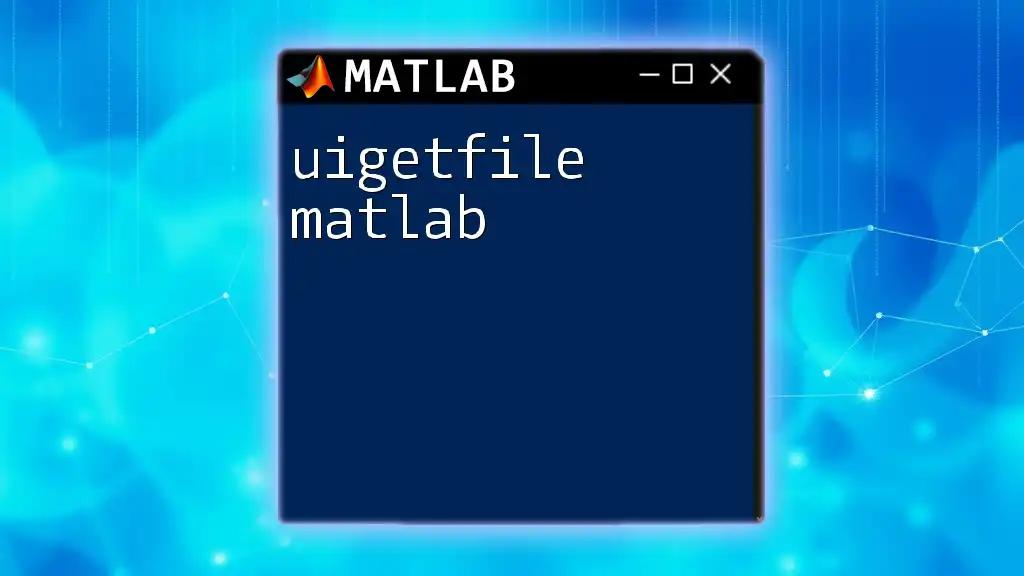
Enhancing Your Skills with MATLAB
Further Reading
To deepen your knowledge of data manipulation in MATLAB, consider exploring related functions like `flipud`, which flips matrices up and down. Combine this with `fliplr` for comprehensive data manipulation techniques.
Additional Resources
The [official MATLAB documentation](https://www.mathworks.com/help/matlab/ref/fliplr.html) offers a wealth of information on `fliplr` and related operations. Community forums are also a great resource for solving specific problems and learning from experienced MATLAB users.
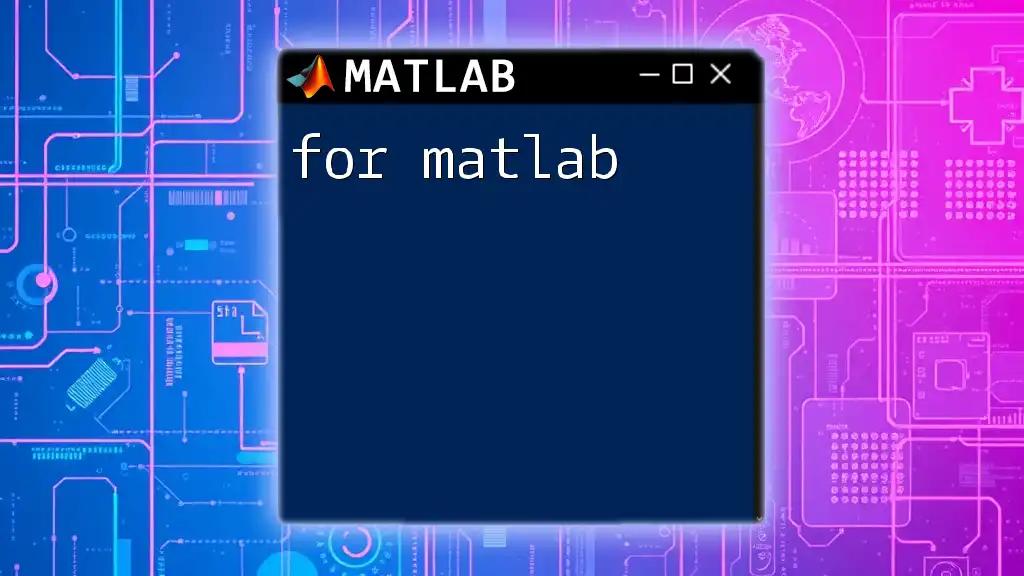
Conclusion
In summary, the `fliplr` function is a powerful tool in MATLAB for rearranging your data. By flipping matrices horizontally, you can gain new perspectives and insights, particularly in data analysis and image processing. Regular practice of techniques discussed here will enhance your MATLAB skill set.
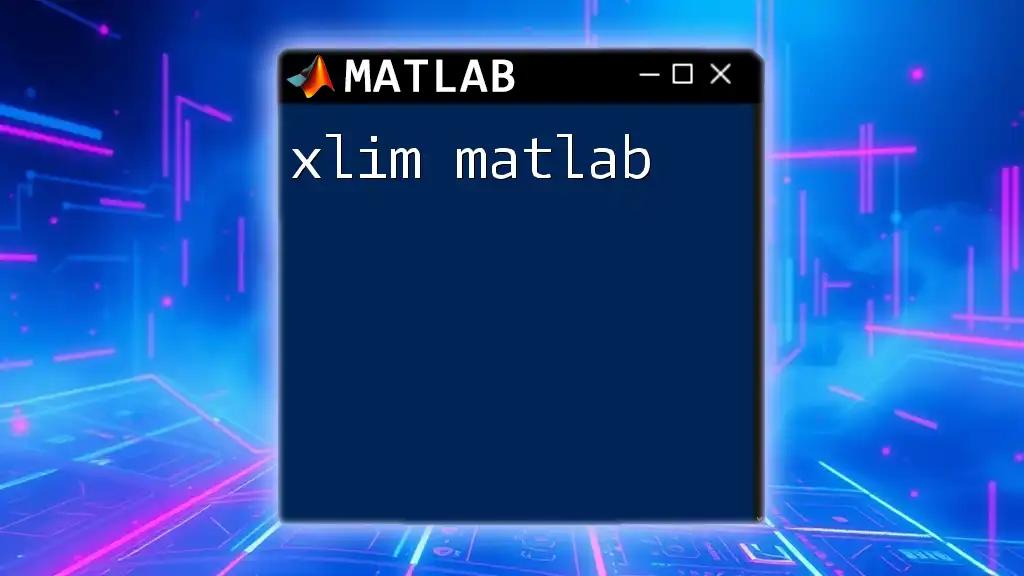
Call to Action
If you are looking to improve your MATLAB skills further, consider joining a dedicated learning community. Sharing your experiences and questions in forums will not only help you but also contribute to the collective growth of knowledge among MATLAB users.