The "fir" command in MATLAB is used to design finite impulse response (FIR) filters based on specified parameters such as the filter order and cutoff frequency.
Here's a simple code snippet that demonstrates how to design a low-pass FIR filter using the `fir1` function:
% Design a low-pass FIR filter with a cutoff frequency of 0.5 times the Nyquist frequency
order = 20; % Filter order
cutoff = 0.5; % Cutoff frequency (normalized)
b = fir1(order, cutoff); % Coefficients of the FIR filter
Understanding FIR Filters
What is an FIR Filter?
An FIR (Finite Impulse Response) filter is a fundamental component in digital signal processing. It is characterized by its finite duration response. Unlike IIR (Infinite Impulse Response) filters, FIR filters do not have feedback, which grants them inherent stability and linearity.
Key Properties:
- Stability: FIR filters are stable since they do not involve feedback loops. This is crucial for real-time applications where unpredictable responses can lead to system instability.
- Linearity: FIR filters maintain the linearity of signal processing. This characteristic ensures that the output is directly proportional to the input, preserving the integrity of the signal.
Applications of FIR Filters
FIR filters are used in various fields, enabling numerous applications:
- Signal Processing: They play a vital role in audio processing, data smoothing, and multi-rate processing, dealing with both analog and digital signals.
- Image Processing: FIR filters are employed for tasks like noise reduction and edge detection, enhancing image clarity and detail.
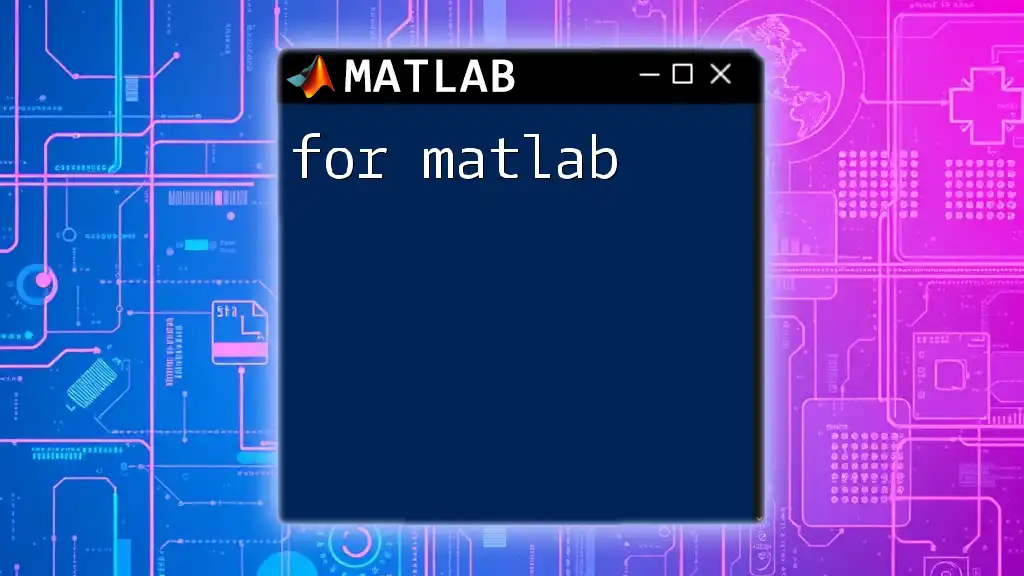
Basic Concepts of FIR Filtering in MATLAB
FIR Filter Design
There are several types of FIR filters, each tailored for specific signal processing needs:
- Low-Pass Filters: These filters allow signals with frequencies lower than a specified cutoff frequency to pass through while attenuating higher frequencies. This application is common in audio processing to eliminate unwanted high-frequency noise.
- High-Pass Filters: High-pass filters do the opposite, allowing high-frequency signals to pass while suppressing lower frequencies. They can be crucial for applications where low-frequency noise needs to be ignored.
- Band-Pass Filters: Band-pass filters permit a specific range of frequencies to pass through, often used in telecommunications and audio technology.
Key Functions in MATLAB
MATLAB offers excellent tools for designing FIR filters. Two primary functions are:
- `fir1`: This function creates a FIR filter using the window method, making it suitable for general-purpose filter design.
- `fir2`: With `fir2`, you can design FIR filters based on a specified frequency response, offering greater control over the filter's characteristics.
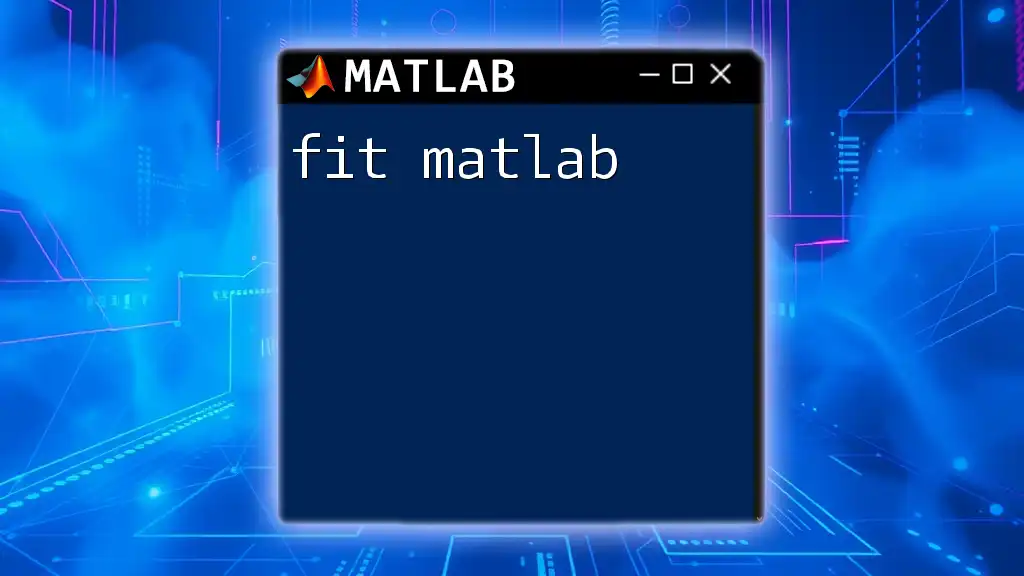
Implementing FIR Filters in MATLAB
Step-by-Step Implementation
Step 1: Define Filter Specifications
To start designing an FIR filter in MATLAB, first, you need to define the order of the filter and its cutoff frequency. For example:
order = 20;
cutoff = 0.4; % Normalized frequency (0 to 1, where 1 is half the sampling rate)
Here, the `order` determines the number of taps in the filter, while the `cutoff` frequency specifies the endpoint of the filter's response.
Step 2: Create the FIR Filter
After defining the specifications, you can create the FIR filter using the `fir1` function:
b = fir1(order, cutoff);
This line generates a low-pass FIR filter characterized by the specified order and cutoff frequency. The coefficients of the filter are stored in variable `b`.
Step 3: Analyze the Filter
Visualizing the filter's frequency response is crucial for validating its design. MATLAB provides the `fvtool` function for analyzing filters:
fvtool(b, 1); % 1 is the denominator for FIR filters
By executing this code, you will invoke a visualization tool that allows you to explore the amplitude and phase characteristics of the designed filter.
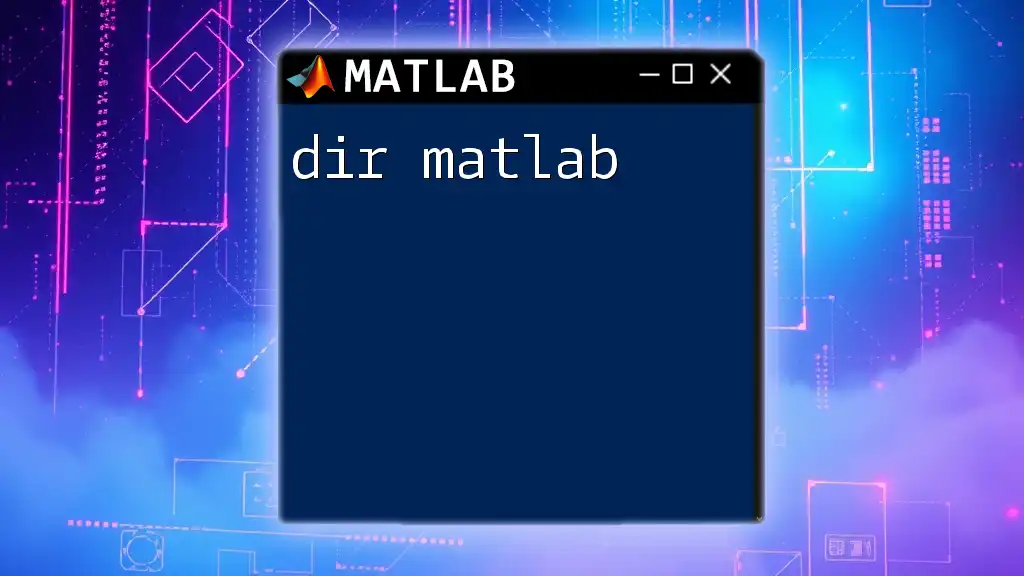
Filtering a Signal with FIR Filter
Signal Creation
To filter a signal, you first need to create a sample signal. Here’s how to generate a noisy sinusoidal signal:
fs = 1000; % Sampling frequency
t = 0:1/fs:1; % Time vector
signal = sin(2 * pi * 50 * t) + 0.5 * randn(size(t)); % Signal with noise
In this script, a sine wave mixed with random noise is generated to simulate a realistic signal for filtering.
Applying the FIR Filter
To apply the FIR filter to the noisy signal, use the `filter` function:
filtered_signal = filter(b, 1, signal);
The `filter` function takes the filter coefficients `b` and applies them to the noisy signal, resulting in a cleaner output stored in `filtered_signal`.
Visualizing the Results
To see the impact of FIR filtering, you can plot both the original and the filtered signals:
figure;
plot(t, signal);
hold on;
plot(t, filtered_signal, 'r');
legend('Original Signal', 'Filtered Signal');
title('Signal Before and After FIR Filtering');
xlabel('Time (s)');
ylabel('Amplitude');
This code generates a plot that helps you visually assess how effectively the FIR filter has attenuated the noise in the signal.
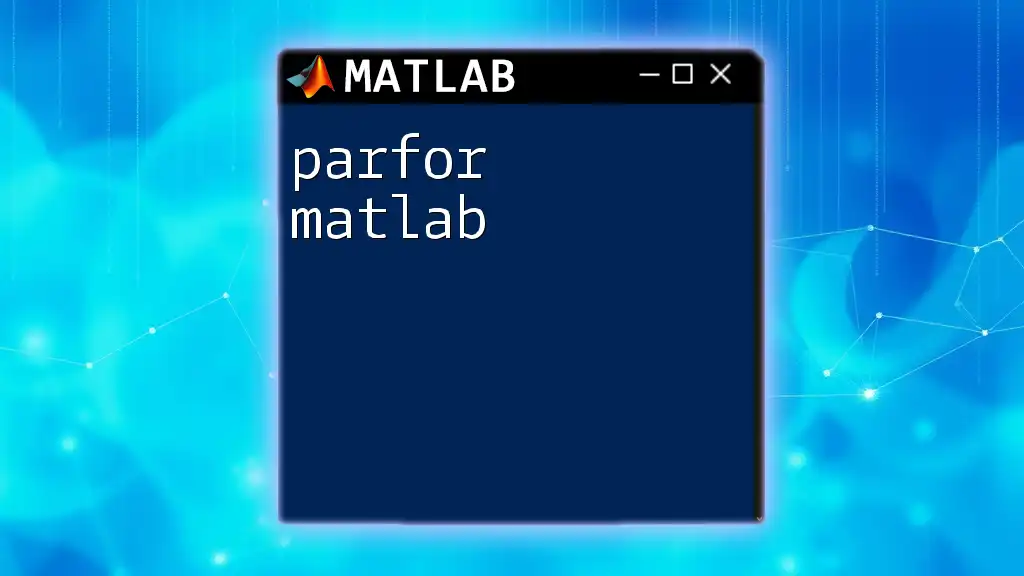
Advanced Topics in FIR Filtering
Designing Custom FIR Filters
While the `fir1` function is quite powerful, you can also design custom FIR filters using various windowing techniques. Common windows include Hamming, Hanning, and Blackman. For example, you can design a windowed FIR filter using:
b_windowed = fir1(order, cutoff, hamming(order + 1));
Using a window function can help reduce ripples in the frequency response, resulting in a more precise filter design.
Performance Optimization
Sometimes, reducing the filter order can be beneficial for computational efficiency. However, you need to balance the performance with the complexity of the filter. One powerful function for creating FIR filters with specific frequency characteristics is `firls`, which employs least squares optimization:
b_lsq = firls(order, [0 cutoff 1], [1 1 0]);
This code snippet illustrates how to design a filter whose frequency response behaves as desired across different bands.
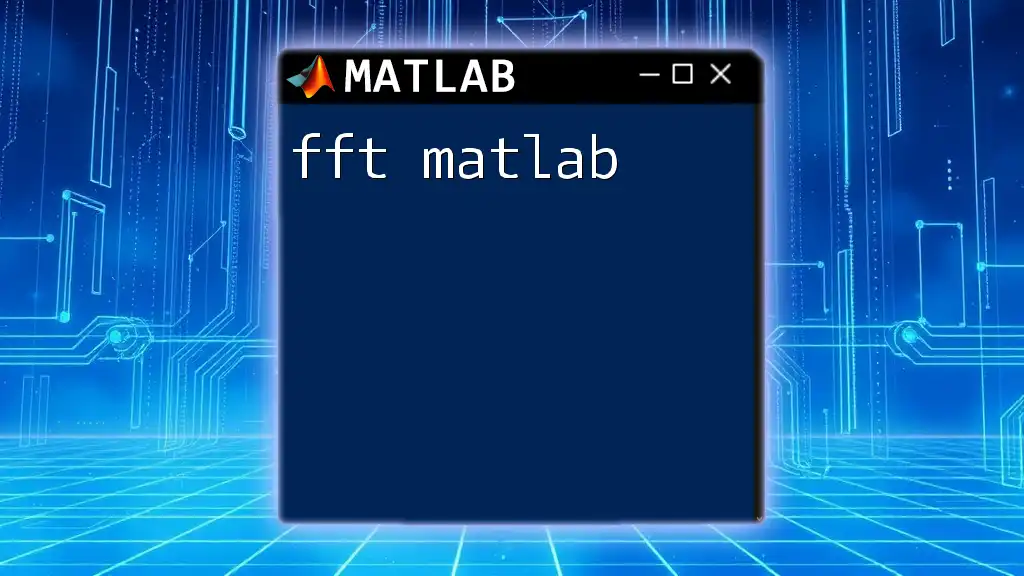
Best Practices for FIR Filter Design
Choosing Filter Specifications
When designing FIR filters, consider the necessary specifications carefully. Proper balance between performance and complexity is crucial to avoid over-designing or under-designing the filter, which can lead to inefficient processing or inadequate filtering.
Testing and Validation
Testing your FIR filter design is equally important. Always validate the performance with various types of signals to ensure that it meets the necessary design criteria. A simple assertion can help check the filter's stability:
assert(isstable(b), 'Filter is not stable');
This line of code acts as a safeguard against instability issues, assuring the reliability of your signal processing chain.
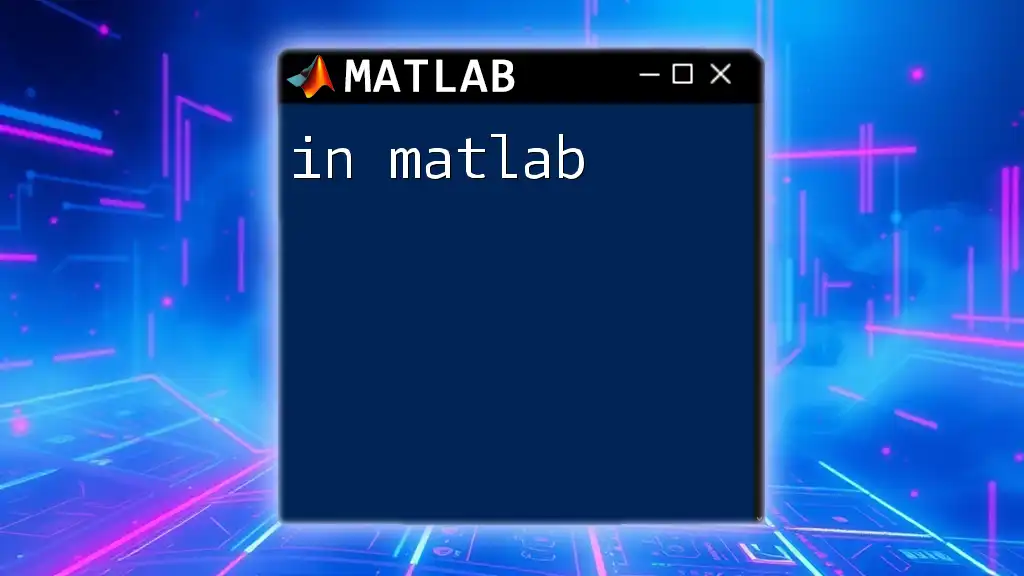
Conclusion
This guide provides an in-depth understanding of FIR filtering in MATLAB, from basic concepts to practical implementation. By mastering these techniques, you can significantly improve your signal processing capabilities, allowing for effective manipulation and enhancement of both audio and visual data.
Now that you are equipped with this knowledge, it is time to experiment with FIR filter designs and explore more sophisticated features in MATLAB to deepen your understanding and proficiency in digital signal processing.