The `uigetfile` function in MATLAB opens a dialog box for users to select one or multiple files, returning the file name and path for further processing.
[filename, pathname] = uigetfile({'*.m';'*.mat';'*.*'}, 'Select a file');
Understanding `uigetfile`
What is `uigetfile`?
The `uigetfile` function in MATLAB is an essential command that facilitates user interaction by allowing users to choose files interactively from their system directories. This command is particularly useful in scenarios where a MATLAB script or function requires input files that may vary depending on user preferences or use cases. By incorporating `uigetfile`, you can prompt users to select the appropriate file needed for analysis, visualization, or manipulation within the MATLAB environment.
Syntax of `uigetfile`
The basic syntax of `uigetfile` is as follows:
[file, path] = uigetfile(filter, dialogTitle);
- file: This variable will hold the name of the file selected by the user.
- path: This variable will contain the directory path where the selected file is located.
- filter: This optional string argument specifies the types of files that will be displayed in the dialog box.
- dialogTitle: This optional string argument sets the title of the file selection dialog.
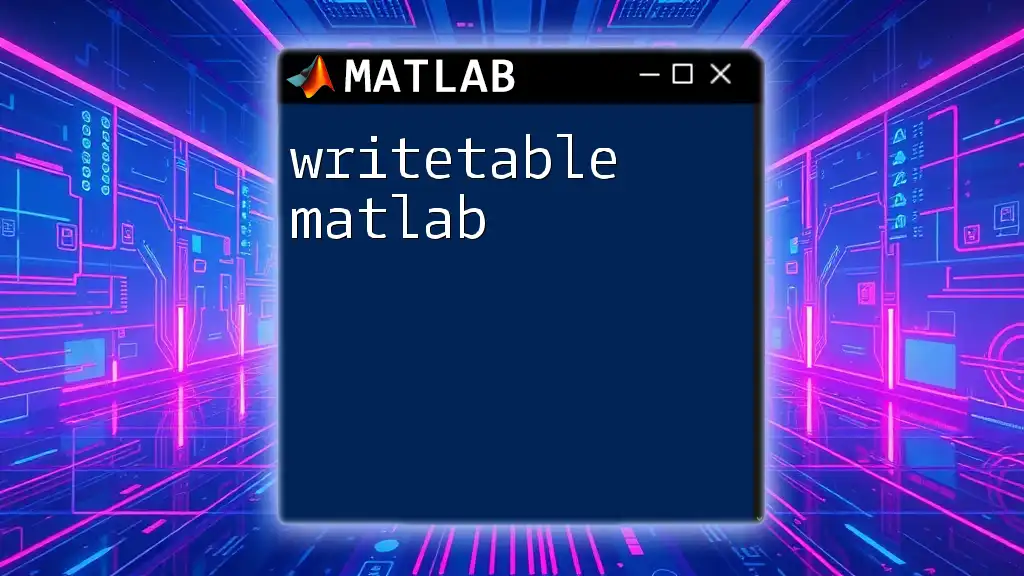
How to Use `uigetfile`
Basic Usage Example
To get started with `uigetfile`, here is a simple example that demonstrates how to prompt the user to select a .mat file:
[file, path] = uigetfile('*.mat', 'Select a MAT-file');
In this command, a dialog box will open, allowing the user to navigate through their file system to select a .mat file. The title of the dialog is set to "Select a MAT-file". Once the user selects a file, its name will be stored in the variable file, and the directory path will be stored in path.
Setting File Filters
Importance of File Filters
Setting file filters when using `uigetfile` significantly improves the user experience. The filters limit the choices to relevant file types, making it easier for users to find what they need without sifting through numerous irrelevant files.
Examples of File Filters
You can configure the filter parameter to present different file types within a single dialog. For instance:
[file, path] = uigetfile({'*.jpg;*.png;*.gif', 'Image Files (*.jpg, *.png, *.gif)'; ...
'*.txt', 'Text Files (*.txt)'; ...
'*.*', 'All Files (*.*)'}, 'Select a File');
In this example, users can choose from Image Files or Text Files. The filter is defined to show specific formats, simplifying user interaction and reducing the chance of file selection errors.
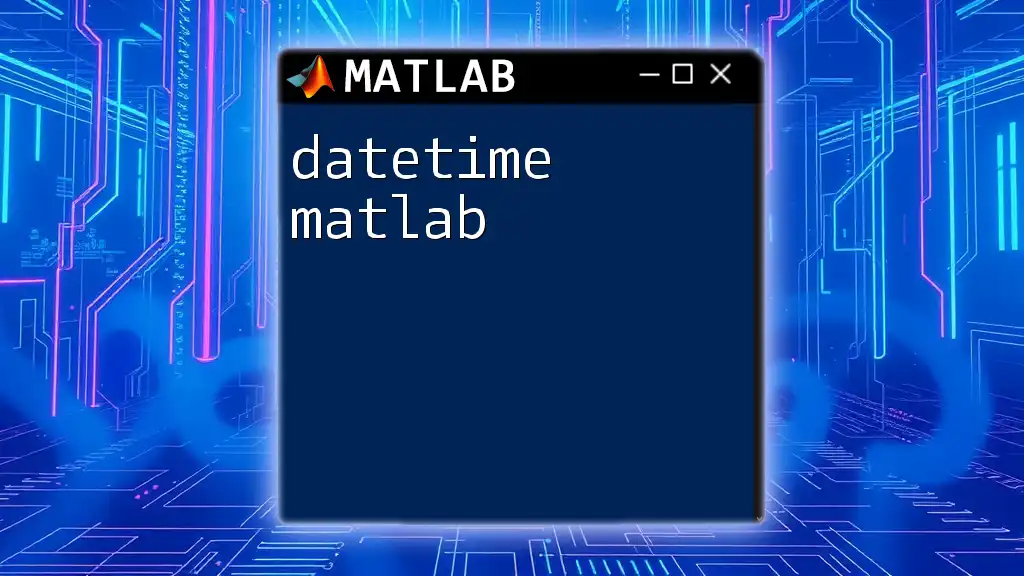
Advanced Options with `uigetfile`
Specifying Initial Directory
To enhance the user experience further, you can specify an initial directory that the dialog box opens to. This is particularly useful if users frequently select files from a particular location. Here’s how to do it:
initialFolder = 'C:\Users\YourUsername\Documents';
[file, path] = uigetfile('*.txt', 'Select a Text File', initialFolder);
In this code snippet, the dialog will open to the specified folder, allowing users to locate their files more quickly.
Handling Cancelled Selections
Why It's Important to Handle Cancellations
It's crucial to anticipate user cancellations to ensure the robustness of your application. This improves user experience and reliability by allowing for graceful exits rather than unexpected errors.
Code Example for Handling Cancellations
You can easily check if a user has clicked Cancel in the dialog by evaluating the file variable:
if isequal(file, 0)
disp('User selected Cancel');
else
disp(['User selected ', fullfile(path, file)]);
end
This code snippet checks if the user pressed Cancel; if so, it outputs a message to the console. Otherwise, it displays the path and name of the selected file.
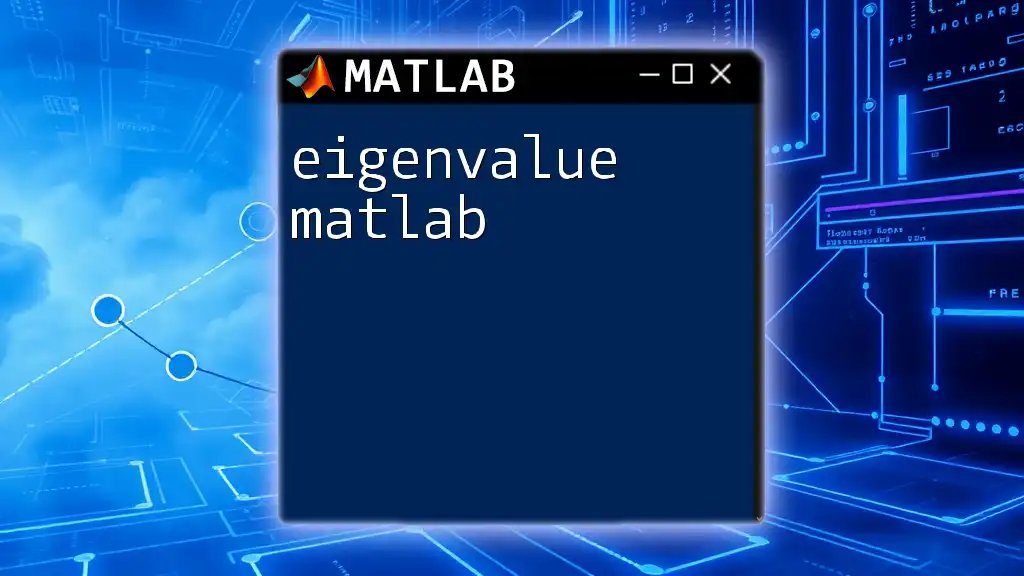
Practical Applications of `uigetfile`
Using `uigetfile` with Other Functions
One of the direct applications of `uigetfile` is in loading data files. After obtaining the file name and path, you can easily load data into MATLAB using the returned variables:
data = load(fullfile(path, file));
This command loads the contents of the selected .mat file, making it readily available for further manipulation or analysis.
Integration with GUI Development
Creating a Simple GUI
Integrating `uigetfile` into a graphical user interface (GUI) can streamline user interaction. Here’s a simple example of how you might structure a GUI that includes a file selection button:
hFig = figure('Position', [100, 100, 300, 200]);
uicontrol('Style', 'pushbutton', 'String', 'Select File', ...
'Position', [100, 80, 100, 40], ...
'Callback', @selectFile);
function selectFile(~, ~)
[file, path] = uigetfile('*.csv', 'Select a CSV File');
if isequal(file, 0)
disp('User selected Cancel');
else
disp(['User selected ', fullfile(path, file)]);
end
end
In this example, a button labeled "Select File" is created in the GUI. When clicked, it triggers the `selectFile` function, allowing users to choose a CSV file and handles potential cancellations effectively.
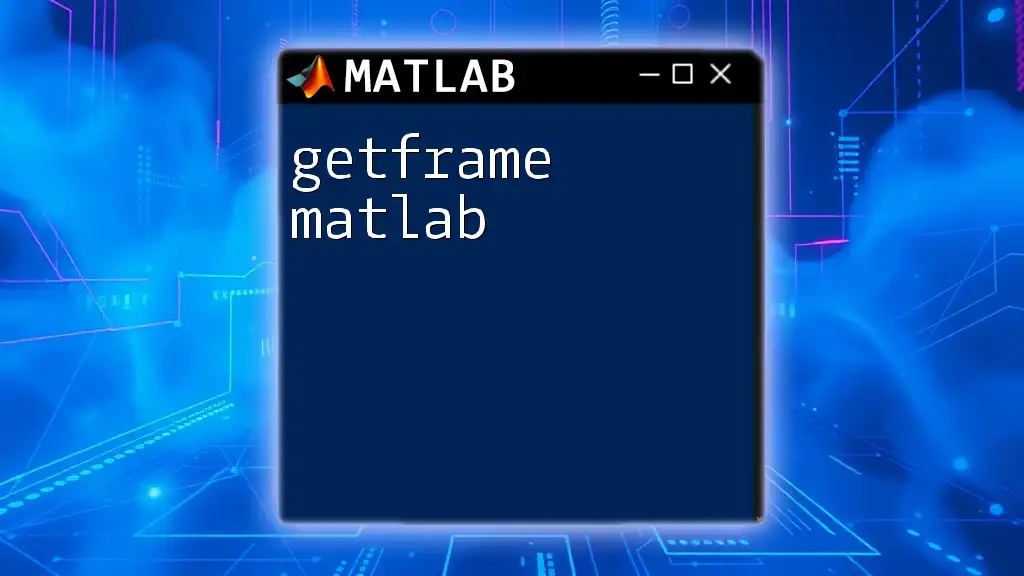
Common Issues and Troubleshooting
Potential Errors with `uigetfile`
Despite its simplicity, users can encounter issues when using `uigetfile`. Common problems include dialog boxes not opening, users selecting files of incompatible formats, or program crashes when Cancel is pressed without appropriate handling.
Tips for Resolving Errors
To mitigate these issues, ensure that your code checks for user cancellations. Additionally, thoroughly document and test your file filters to prevent inaccessible file types from being mistakenly presented to users.
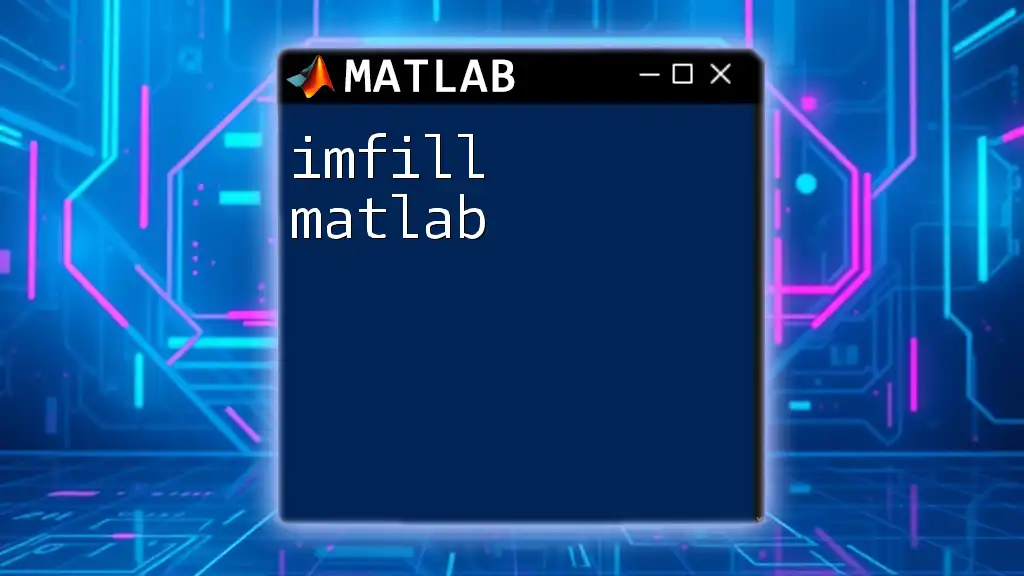
Conclusion
Incorporating `uigetfile` in your MATLAB projects enhances user interaction and simplifies file management, particularly for scripts that require user-supplied input files. This function is invaluable in various applications, particularly data loading, GUI design, and improving overall user experience. Practice using `uigetfile` alongside other MATLAB commands to deepen your understanding and mastery of MATLAB programming.
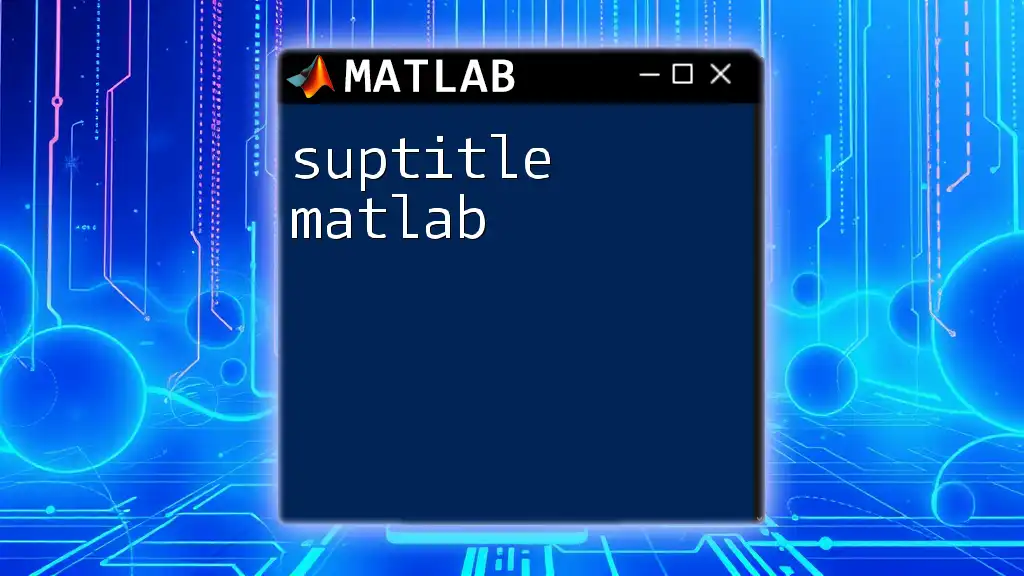
Additional Resources
For further insight, consider exploring the official MATLAB documentation on `uigetfile`, which provides in-depth explanations and examples. Engaging with tutorials and example projects can also enrich your practical knowledge and application of this versatile command.