"Fitness MATLAB refers to the use of MATLAB programming to create and analyze fitness-related models, simulations, or data manipulations that enhance understanding of physical training or health metrics."
Here's a simple code snippet that demonstrates how to calculate the Body Mass Index (BMI) using MATLAB:
% Calculate BMI
weight_kg = 70; % weight in kilograms
height_m = 1.75; % height in meters
bmi = weight_kg / (height_m^2); % BMI formula
fprintf('Your BMI is: %.2f\n', bmi);
Fitness Applications of MATLAB
Overview
Fitness MATLAB has become increasingly popular among fitness enthusiasts and professionals alike. With its powerful computational capabilities, MATLAB can analyze data, visualize results, and optimize training routines. This section explores how MATLAB is being used to revolutionize fitness training, health monitoring, and performance analysis.
Case Studies
Many athletes and sports scientists have utilized fitness MATLAB for performance enhancement. Various case studies highlight the effectiveness of MATLAB in tracking progress and tailoring workouts to individual needs. For instance, elite athletes have successfully applied data analysis techniques to identify strengths, weaknesses, and areas for improvement. Studies have also shown that the integration of MATLAB in sports science research has led to advancements in training methodologies.
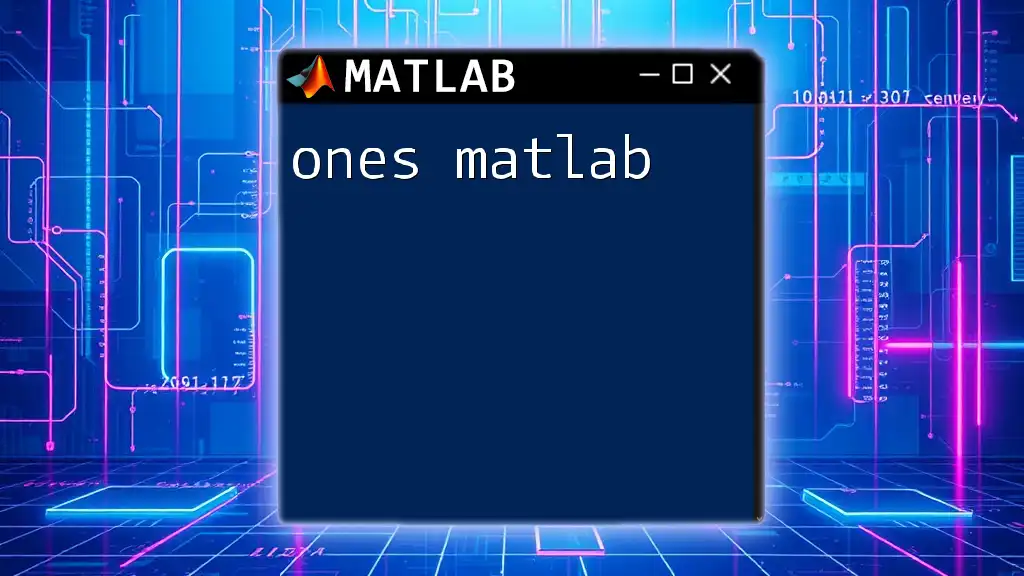
Getting Started with MATLAB for Fitness
Installation and Setup
Before diving into fitness MATLAB applications, it's essential to have the proper setup. Follow these steps to install MATLAB:
- Download MATLAB from MathWorks' website.
- Follow the installation instructions, ensuring that you include necessary toolboxes, such as the Statistics and Machine Learning Toolbox and the Optimization Toolbox.
Basic Commands
Familiarizing yourself with essential MATLAB commands will enhance your effectiveness. Some key commands to start with include:
- `clc`: Clears the command window.
- `clear`: Removes variables from the workspace, keeping your environment tidy.
- `close all`: Closes all open figure windows.
Here's a simple command to show that you can perform basic arithmetic:
a = 5;
b = 10;
c = a + b;
disp(c);
This small code snippet adds two numbers together and displays the result.
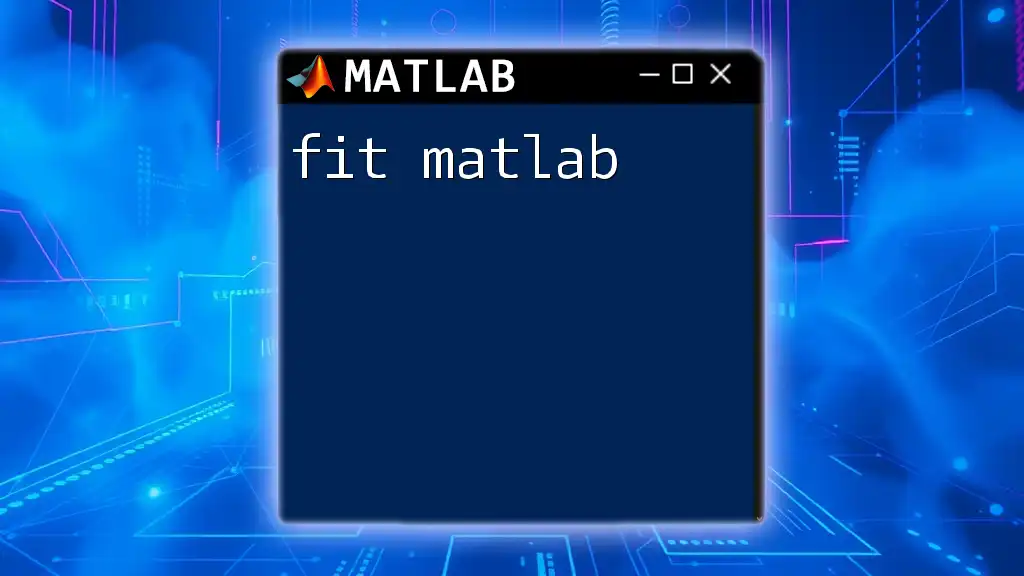
Analyzing Fitness Data in MATLAB
Types of Fitness Data
When it comes to fitness analysis, understanding the types of available data is critical. Common types include:
- Heart rate data: To monitor cardiovascular performance.
- Workout logs: To track exercise volume, intensity, and duration.
- Nutritional intake data: To correlate diet with fitness outcomes.
Ensuring the quality and collection methods of this data is essential for reliable analysis.
Importing Data into MATLAB
Once you have your fitness data, importing it into MATLAB is a straightforward process. You can utilize `readtable` to load common file formats such as CSV or Excel files.
For example, consider a CSV file named `fitness_data.csv`. You can import it as follows:
data = readtable('fitness_data.csv');
This script reads the data and stores it in a variable called `data`, allowing for further manipulation and analysis.
Data Visualization
Visual representation of fitness data can significantly enhance insights. MATLAB offers various plotting functions to visualize progress effectively.
To track your workout progress over time, a simple line plot can be created using the `plot` function:
time = [1, 2, 3, 4, 5]; % Days
performance = [50, 55, 60, 65, 70]; % Performance metric
plot(time, performance);
xlabel('Days');
ylabel('Performance');
title('Workout Performance Over Time');
grid on;
This visual aids in understanding how performance develops during a training regimen.
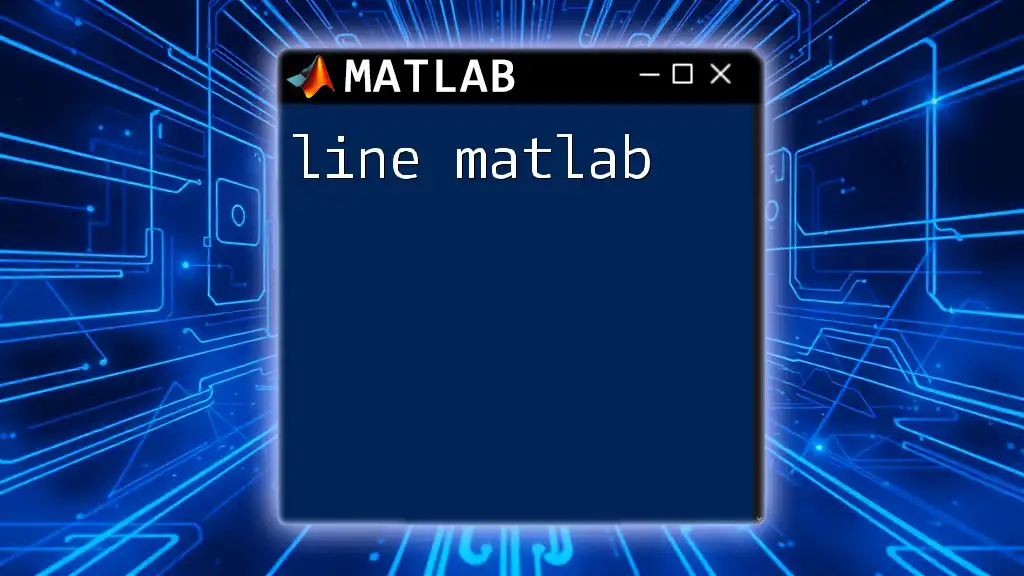
Fitness Modeling in MATLAB
Creating Fitness Models
Modeling is a powerful tool in fitness analysis. Statistical and computational models can provide insights into predicting outcomes based on various inputs. An essential approach is linear regression, which can be used to assess relationships between factors, such as exercise volume and weight loss.
Code Snippet Example
Here’s an example of building a linear regression model to predict weight loss based on workout frequency.
% Sample data
workouts_per_week = [1, 2, 3, 4, 5];
weight_loss = [2, 4, 6, 8, 10];
% Fitting a linear model
mdl = fitlm(workouts_per_week, weight_loss);
disp(mdl);
This code fits a linear model to the data, allowing you to interpret how workout frequency influences weight loss.
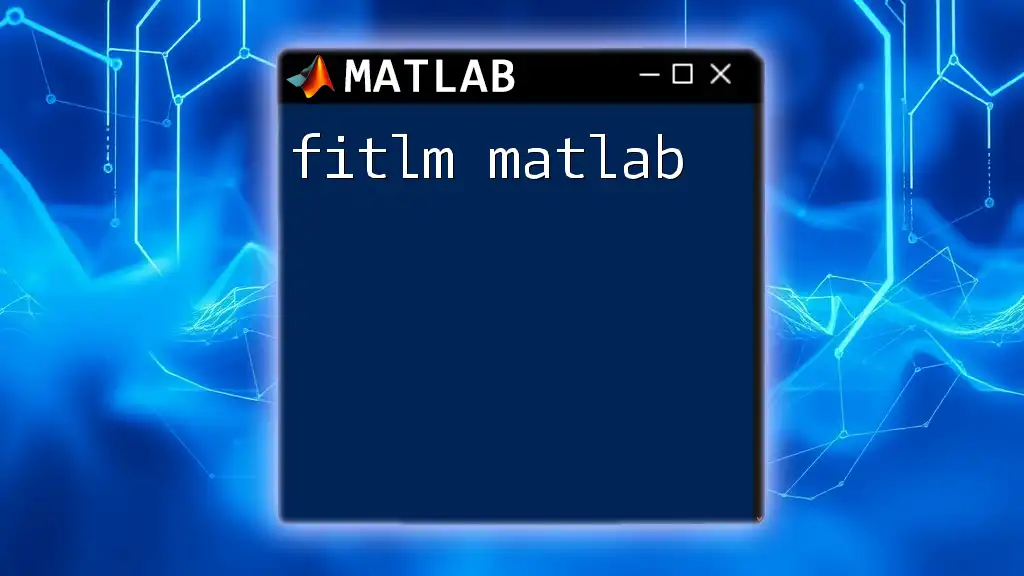
Optimization Techniques in Fitness
The Role of Optimization
Optimizing fitness goals is crucial for effective training. By employing optimization techniques, individuals can maximize their workout efficiency, thereby achieving desired outcomes more expediently.
Genetic Algorithms and MATLAB
Genetic algorithms are one way to personalize fitness plans. These algorithms mimic natural selection to explore a wide solution space for determining optimal workout schedules or nutritional plans.
Here’s an example of implementing a genetic algorithm in MATLAB:
% Objective function for optimization
objectiveFunc = @(x) (10 - x(1))^2 + (5 - x(2))^2;
% Genetic algorithm parameters
options = optimoptions('ga', 'Display', 'iter');
[x, fval] = ga(objectiveFunc, 2, [], [], [], [], [0, 0], [10, 10], [], options);
disp([x, fval]);
This script sets up a simple optimization scenario with two variables to find the best combination that minimizes the given objective function.
Sample Optimization Code
When optimizing workout schedules, you can set specific constraints based on personal fitness goals. Below is an example code to optimize training duration and intensity:
% Example function
fitnessFunc = @(x) -1 * (x(1) * x(2)); % Minimize product of duration and intensity
% Constraints
A = [1, 1; 2, 3];
b = [8; 18]; % hours
lb = [0, 0]; % lower bounds
ub = [10, 10]; % upper bounds
% Optimization
options = optimoptions('fmincon', 'Display', 'iter');
[x_opt, fval] = fmincon(fitnessFunc, [1, 1], A, b, [], [], lb, ub, [], options);
disp([x_opt, fval]);
This example minimizes the product of training duration and intensity under specified constraints.
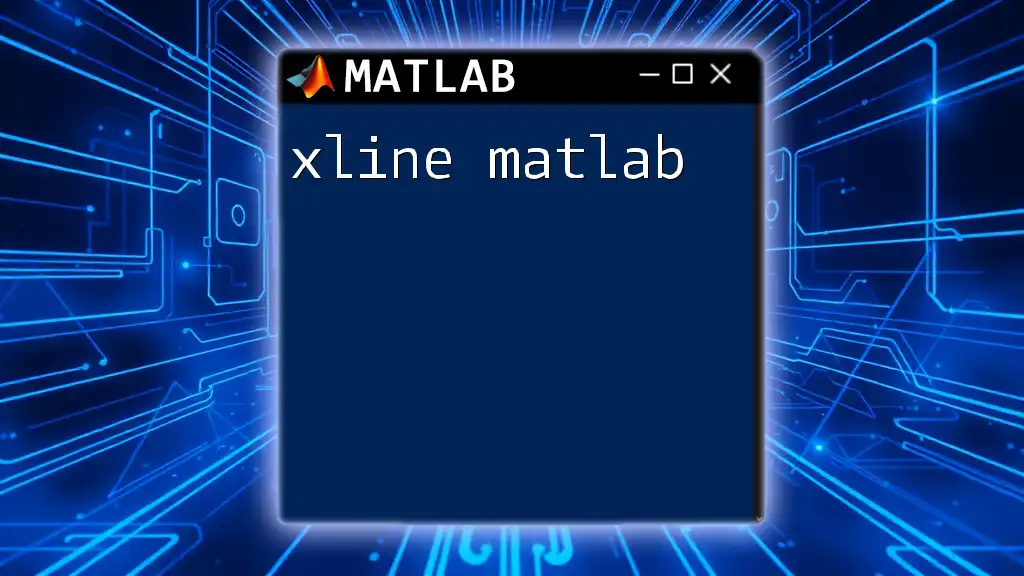
Case Study: Personalized Fitness Plans
Developing a Personal Plan using MATLAB
Creating a personalized fitness plan can be achieved through systematic analysis. Here’s how you can do it using MATLAB:
- Gather user input regarding fitness goals (e.g., weight loss, muscle gain) and current fitness levels.
- Use MATLAB scripts to analyze data and generate a customized workout plan accordingly.
Here’s a sample code snippet for generating a personalized workout plan based on user input:
% User input
user_goal = 'weight loss'; % Example goal
current_level = 5; % Scale of 1 to 10
% Generate plan based on goals
if strcmp(user_goal, 'weight loss')
plan = sprintf('Increase cardio by %d minutes each day', current_level * 10);
else
plan = 'Focus on strength training workouts';
end
disp(plan);
This script outputs a generalized workout plan based on user-defined goals.
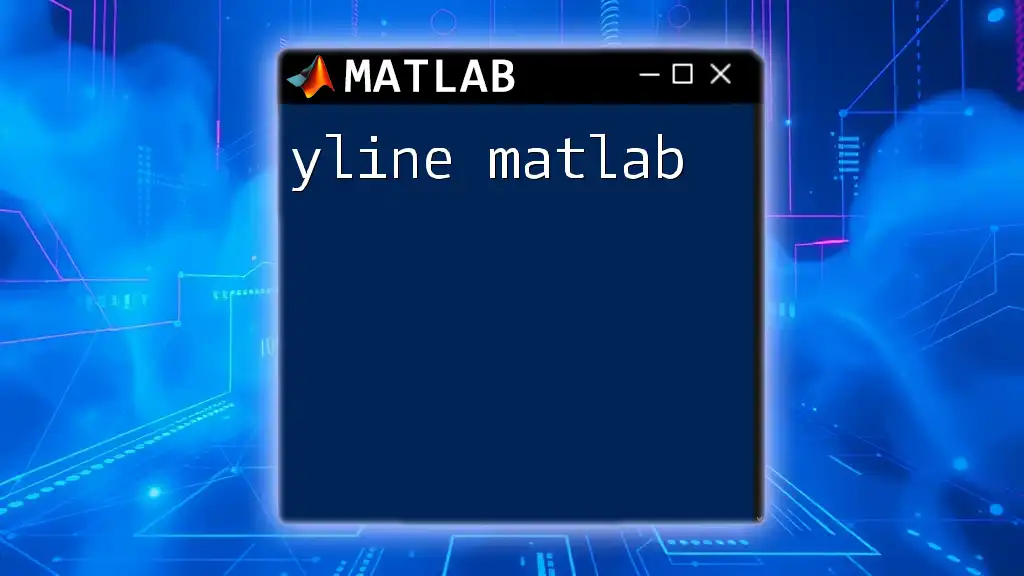
Advanced Features of MATLAB in Fitness Training
Simulations and Predictive Analytics
Utilizing simulations to predict fitness outcomes can be invaluable. MATLAB provides tools for simulating different training scenarios to assess potential results without physical trials.
For example, simulating workout effects might involve using random variables to model fluctuations in performance metrics. Here’s a simple simulation code:
% Simulation parameters
days = 30;
initial_performance = 100; % Initial performance metric
performance_loss = randn(days, 1); % Random variations
% Simulate daily performance
daily_performance = initial_performance - cumsum(performance_loss);
plot(1:days, daily_performance);
xlabel('Days');
ylabel('Daily Performance');
title('Simulated Daily Performance');
grid on;
Machine Learning Applications
Integrating machine learning techniques offers further advancements in fitness tracking. You can leverage algorithms to detect patterns in historical fitness data, thus predicting future performance.
An example might involve using a fitting algorithm to assess a user’s workout type and expected gains based on historical data.
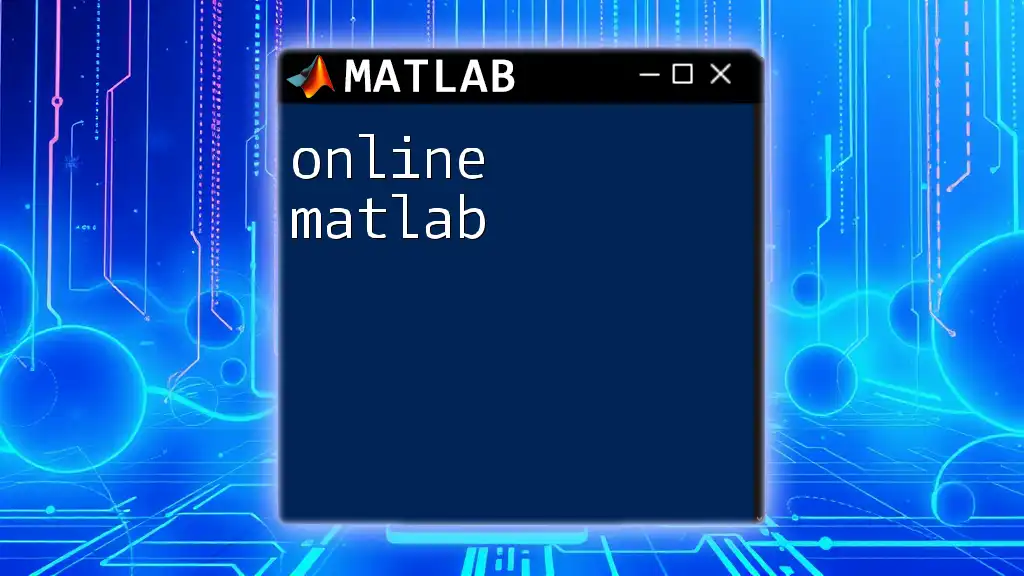
Community and Resources
Learning and Support
When beginning your journey with fitness MATLAB, it's vital to know where to find help. Several online forums, communities, and the official MATLAB support system offer guidance and tips for both beginners and advanced users.
Recommended Resources
To deepen your understanding, consider exploring books, online courses, or websites that focus on MATLAB programming in the context of fitness. This will not only augment your skills but also keep you updated on the latest advancements in the field.
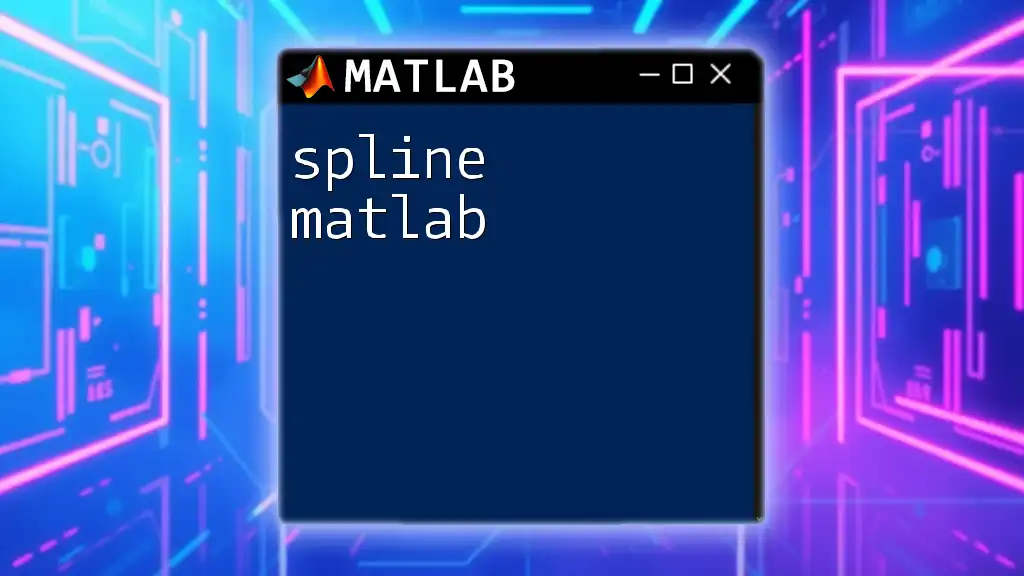
Conclusion
Utilizing fitness MATLAB can dramatically enhance your training regimen and analysis. By applying the tools and techniques discussed in this guide, you can better understand your fitness data, optimize your workout plans, and ultimately achieve your fitness goals more effectively.
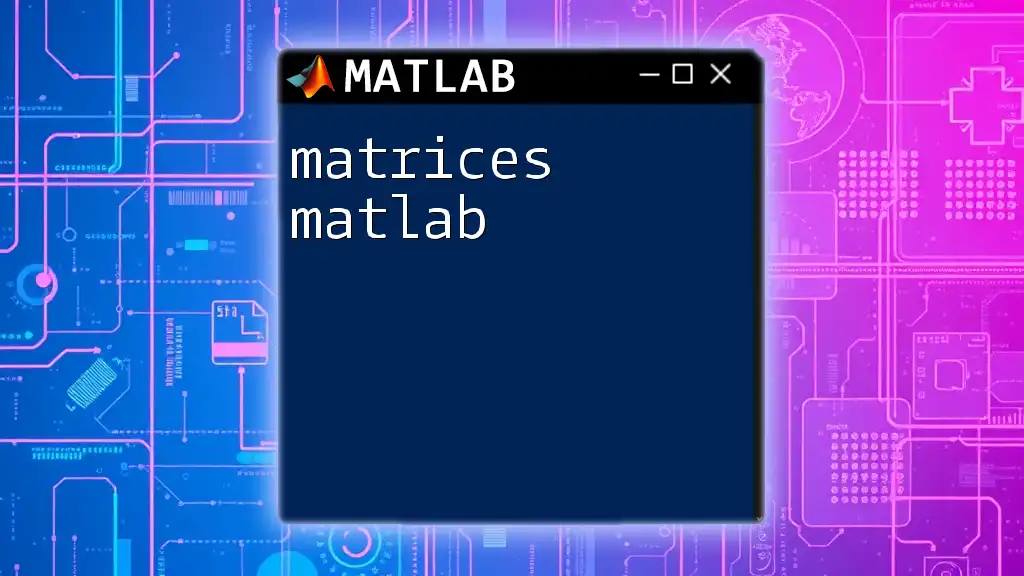
Call to Action
Ready to get started with fitness MATLAB? Sign up for our courses today, and take the first step toward mastering MATLAB for your fitness journey. The road to achieving your fitness goals has never been more accessible!