The `fzero` function in MATLAB is used to find the roots of a nonlinear function, allowing you to determine where the function equals zero.
Here's a simple example of using `fzero` to find the root of the function \( f(x) = x^2 - 4 \):
f = @(x) x^2 - 4; % Define the function
root = fzero(f, 1); % Find the root starting from an initial guess of 1
disp(root); % Display the root
What is `fzero`?
The `fzero` function in MATLAB is designed to find the roots of nonlinear equations. In mathematical terms, a root of a function is a value of \(x\) for which the function \(f(x) = 0\). This tool is essential for solving equations that cannot be expressed in a simple algebraic form and arises frequently in various fields including engineering, physics, and finance. For instance, determining the break-even point in cost functions or calculating zeros in waveform analysis both rely on root-finding techniques.
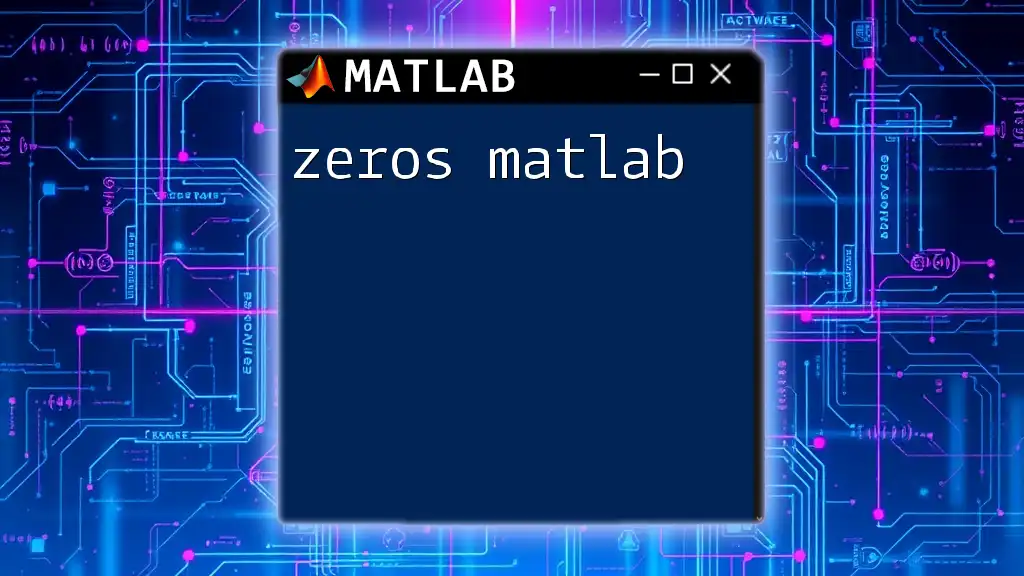
Understanding the Basics of `fzero`
Syntax of `fzero`
The basic syntax of the `fzero` function is as follows:
x = fzero(fun, x0)
x = fzero(fun, x0, options)
Here, fun is the handle to the function for which you want to find a root, x0 is your initial guess, and options allows you to customize the root-finding process.
Parameters Explained
- fun: This can either be a function handle for a MATLAB function or an inline function defined in your script.
- x0: Represents an initial guess or a starting interval for the root. The closer this guess is to the actual root, the more likely `fzero` will converge quickly and accurately.
- options: This parameter allows you to adjust various settings for the root-finding method, including tolerances for stopping criteria.
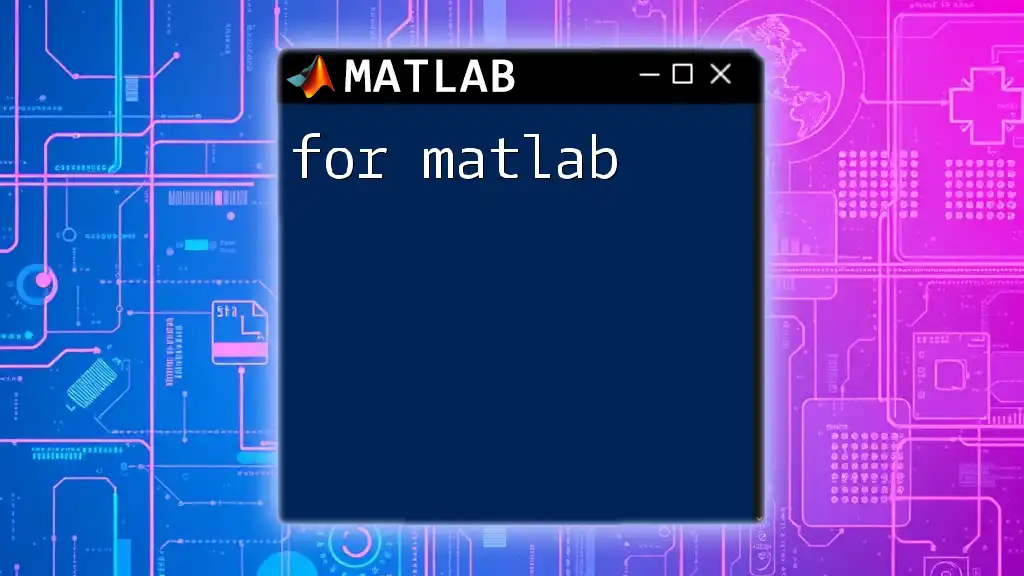
How to Use `fzero` in MATLAB
Step-by-Step Guide to Using `fzero`
Step 1: Define the Function to Find Roots
To begin using `fzero`, first, you need to define the function whose roots you're interested in finding. For example, if you want to find the roots of the function \(f(x) = x^2 - 4\), you can define it as follows:
fun = @(x) x.^2 - 4; % Find x such that x^2 - 4 = 0
Step 2: Choose an Initial Guess
Next, you must select an initial guess. In this case, let’s choose \(x0 = 1\):
x0 = 1; % Initial guess close to the expected root
Step 3: Call `fzero` with Example
With the function and initial guess defined, you can now call the `fzero` function:
root = fzero(fun, x0);
disp(['Root found: ' num2str(root)]);
This snippet will output the found root, which should be \(2\), since \(f(2) = 0\).
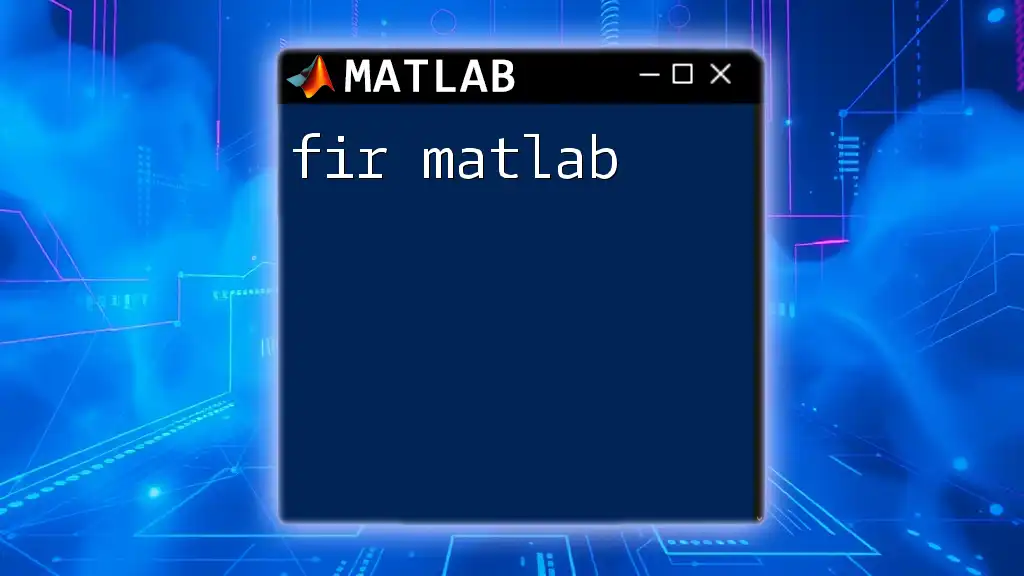
Practical Examples of `fzero`
Example 1: Finding Roots of a Quadratic Function
Let's take a closer look at the quadratic function we defined earlier:
f = @(x) x.^2 - 4;
root = fzero(f, 1); % Searching from initial guess 1
In this example, `fzero` starts searching based on our initial guess \(x0 = 1\). Upon execution, it will find that the root at \(x = 2\) satisfies the condition \(f(2) = 0\). The output will confirm this, demonstrating the effectiveness of `fzero` in locating roots efficiently.
Example 2: Root Finding with Multiple Roots
Consider a function with multiple roots:
g = @(x) (x-1).*(x+1).*(x-2);
If we apply `fzero` with different initial guesses, we can find each root.
root1 = fzero(g, 0); % Near root at -1
root2 = fzero(g, 2); % Near root at 2
The first call will yield a root close to `-1`, while the second will yield `2`. This illustrates how initial guesses significantly influence the results returned by `fzero`.
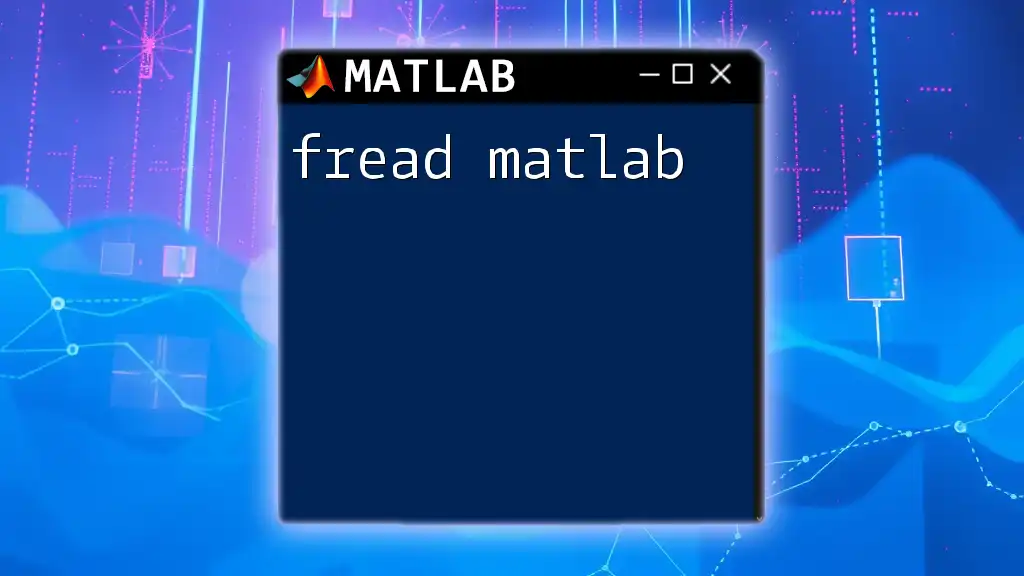
Advanced Features of `fzero`
Using Options to Refine Results
To enhance the performance and accuracy of `fzero`, you can customize settings through the `optimset` function. This allows you to define specific parameters such as tolerances and verbosity.
Here's a quick example of how to set options:
options = optimset('TolFun', 1e-6, 'Display', 'iter');
root = fzero(f, 1, options);
In this case, `TolFun` sets the function tolerance, while `Display` option allows you to see iterative output during the root-finding process. This feature can greatly assist you in diagnosing convergence issues.
Visualizing Function Behavior
Visualizing your function and its roots can greatly aid in understanding. Here’s how you can plot the function along with the roots found:
f = @(x) x.^2 - 4;
fplot(f, [-3, 3]);
hold on;
plot(root, f(root), 'ro'); % Marking the root on the graph
legend('f(x)', 'Root');
hold off;
This code snippet plots \(f(x) = x^2 - 4\) and marks the found root on the curve. Such visual representations are invaluable in validating results and gaining deeper insights.
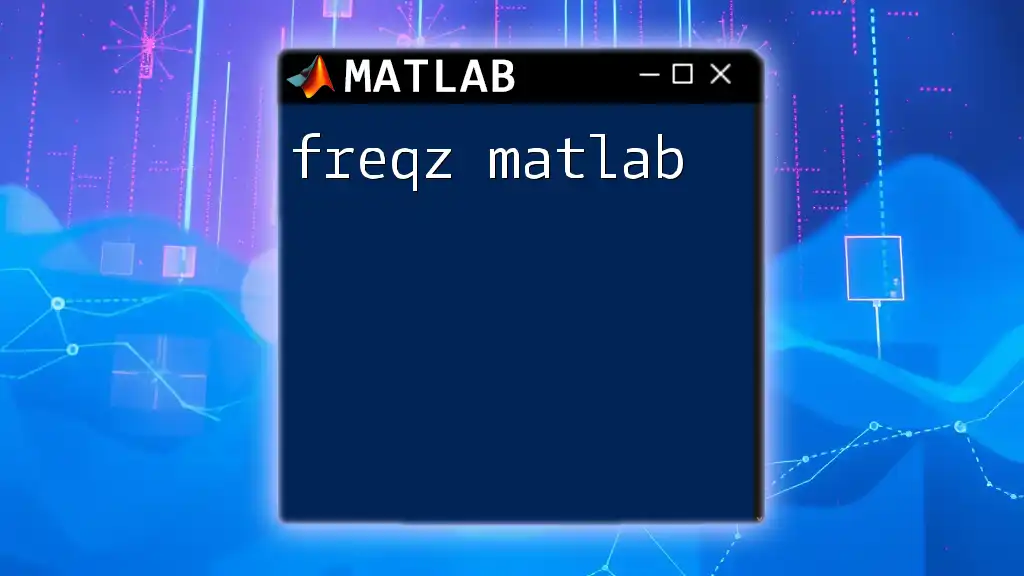
Common Issues and Troubleshooting
While using `fzero`, you may encounter challenges such as convergence issues, especially if your initial guess is too far from the actual root. If `fzero` fails to find a root, consider employing the following strategies:
- Refine your initial guess: Choose a guess closer to the expected root based on your function's behavior.
- Check the function values: Ensure that the function actually crosses zero within the interval defined by your guesses.
Utilizing these tips can lead to more reliable outcomes, reducing frustration in the root-finding process.
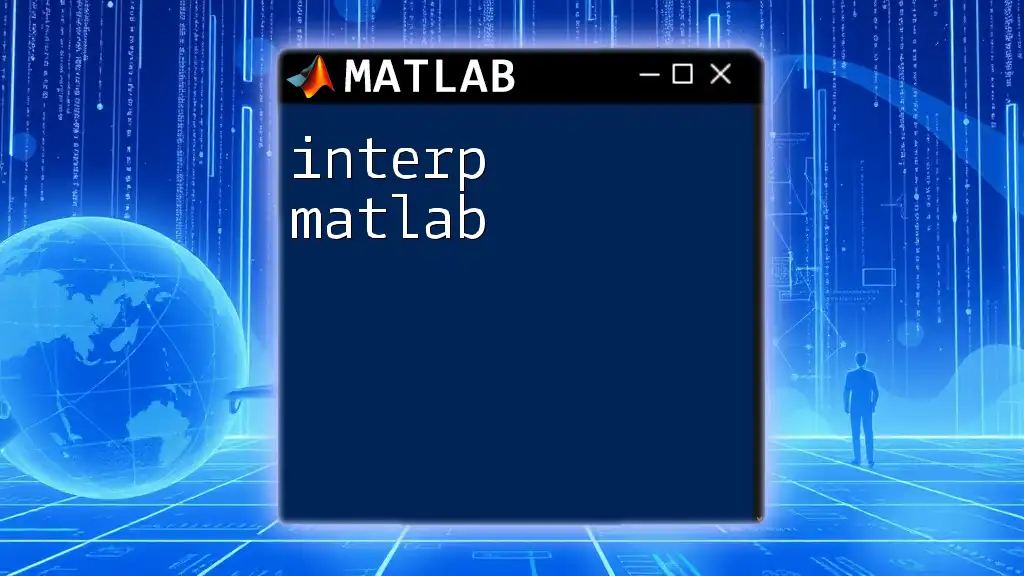
Conclusion
In summary, `fzero matlab` is an essential function for efficiently finding the roots of nonlinear equations. Its utility spans numerous practical applications, making it a critical tool for engineers, scientists, and economists alike. By mastering this function along with its parameters and options, you can enhance your problem-solving skill set in MATLAB significantly.
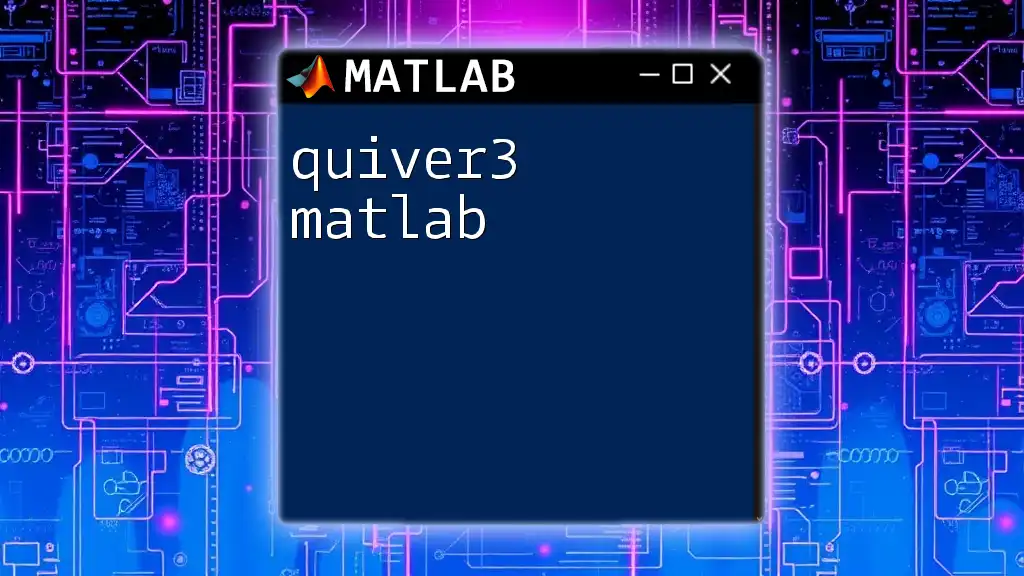
Further Learning Resources
To continue enhancing your MATLAB skills, refer to the official MATLAB documentation for comprehensive details on `fzero` and related functions. Additionally, consider enrolling in online resources, tutorials, or courses designed to deepen your understanding of MATLAB programming and numerical methods. With practice and exploration, you will become proficient in using `fzero` and be equipped to tackle a wider range of mathematical challenges.
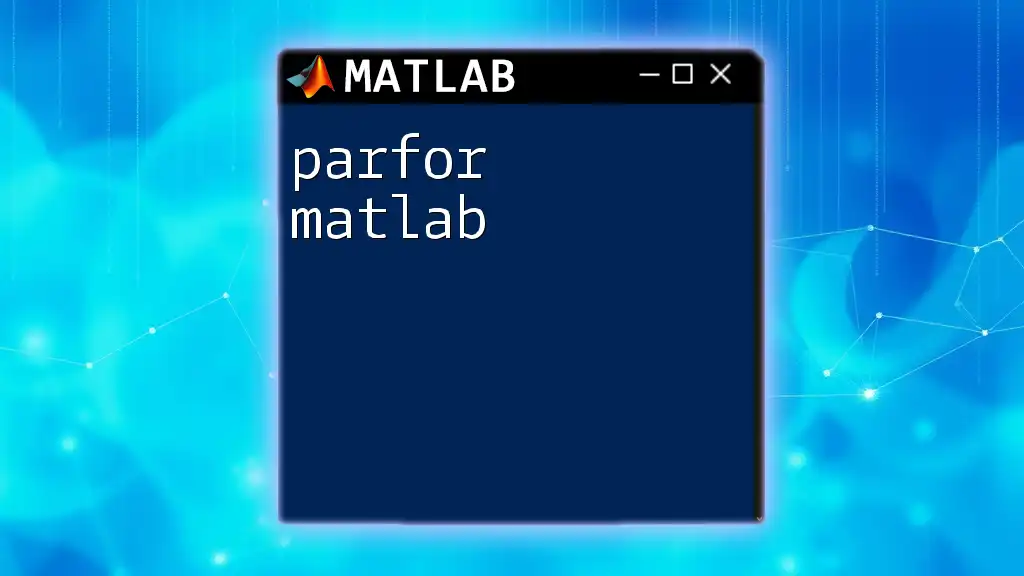
FAQs about `fzero`
What types of functions can `fzero` handle?
`fzero` can handle any continuous, nonlinear functions, provided a root exists in the vicinity of the guess.
How do I deal with complex roots in MATLAB?
For complex roots, consider using specialized functions such as `fsolve`, which are designed to handle more advanced cases and multi-variable scenarios.
Can I use `fzero` for multiple variables?
No, `fzero` is designed for single-variable functions. For multiple variables, refer to `fsolve`.
Is there a way to find the derivative using `fzero`?
`fzero` specifically searches for roots but does not calculate derivatives. For derivative calculations, you can use `diff` or symbolic computation functions in MATLAB.