To fit a curve in MATLAB, you can use the `fit` function, which allows you to specify a model type and provides a best-fit curve for your data. Here's an example code snippet:
x = [1, 2, 3, 4, 5]; % Sample data points
y = [2.2, 2.8, 3.6, 4.5, 5.1]; % Corresponding y values
f = fit(x', y', 'poly1'); % Fit a linear polynomial (first degree)
plot(f, x, y); % Plot the fitted curve along with the data points
Understanding Curve Fitting
What is Curve Fitting?
Curve fitting is a method used to create a curve that best represents a set of data points. The goal is to find a mathematical function that approximates the underlying trend in the data, enabling predictions or insights about trends. Curve fitting can be linear, where a straight line (or polynomial of degree one) is used, or nonlinear, where more complex functions—such as exponentials or polynomials of higher degree—are employed.
Curve fitting is crucial in various fields, including data analysis, engineering, and science. It allows researchers to interpret data, test hypotheses, and make predictions based on observed patterns.
Types of Curve Fitting
Linear Curve Fitting is the simplest form of curve fitting. In this approach, data is fitted with a straight line, which is useful when the data shows a constant rate of change. Linear fitting is mathematically represented by the equation \( y = mx + b \), where \( m \) is the slope and \( b \) is the intercept.
Nonlinear Curve Fitting involves fitting data with more complex functions. This type of fitting is essential when the relationship between the variables is not constant and can vary significantly. Common nonlinear models include quadratic, exponential, and logarithmic functions. Understanding the nature of your data is critical when choosing the appropriate fitting technique.
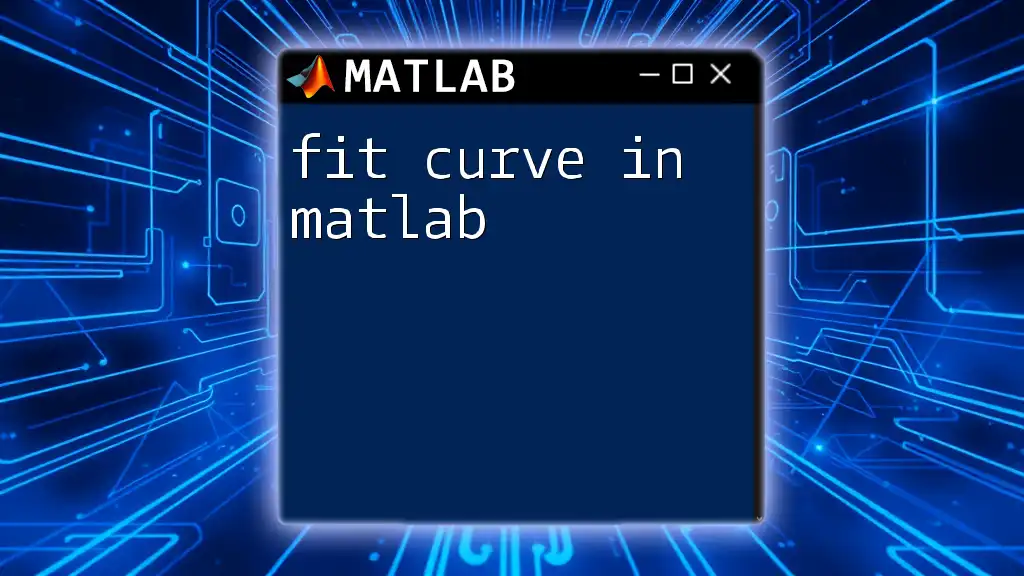
Getting Started with MATLAB
Setting Up MATLAB
Installing MATLAB is straightforward, and it provides an extensive environment for data analysis and visualization. After installing, familiarize yourself with the interface, including the workspace, command window, and various toolboxes available for curve fitting.
Importing Data
Before fitting a curve in MATLAB, it’s essential to import your dataset effectively. The most common method is using the `readtable` function for reading CSV files. For example:
data = readtable('yourdata.csv');
Once imported, clean and preprocess the data. This step might involve handling missing values or outliers, ensuring that the dataset is ready for analysis.
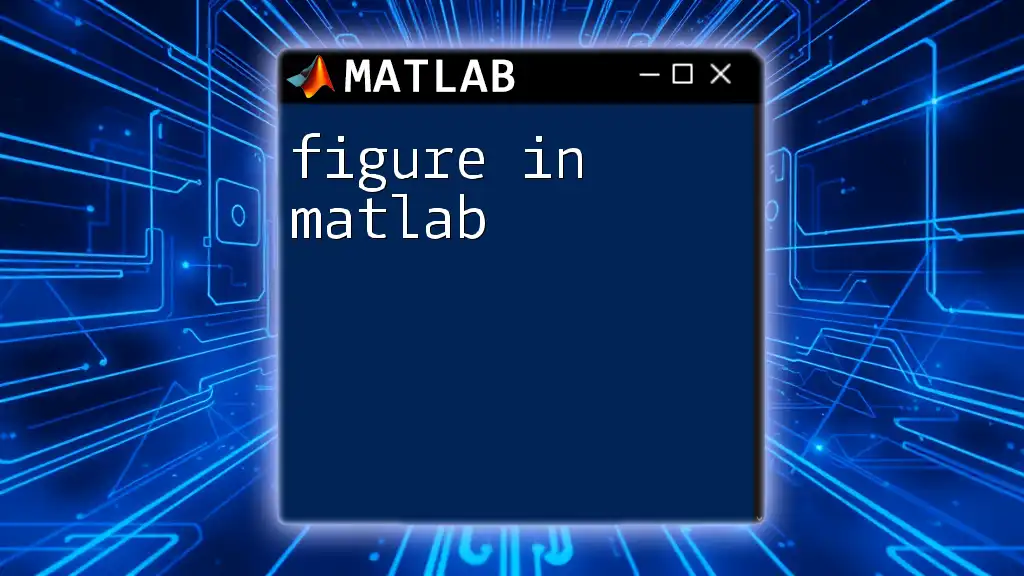
Fitting a Curve in MATLAB
Using Built-in Functions
Linear Curve Fitting with `polyfit`
To fit a curve linearly in MATLAB, the `polyfit` function can be used. This function fits a polynomial to a set of data points and is particularly useful for linear and polynomial fitting.
For instance, to fit a linear model to a dataset, you can execute:
x = [1, 2, 3, 4, 5];
y = [2.1, 4.1, 5.9, 8.2, 10.1];
p = polyfit(x, y, 1); % Linear fit (first degree polynomial)
Here, `p` contains the coefficients of the linear equation. To evaluate how well this model fits your data, use the `polyval` function:
y_fit = polyval(p, x);
Interpreting the Results
The output coefficients from `polyfit` give you a clear insight into the linear relationship between `x` and `y`. Plot the original data points and the fitted line to visualize the fit:
figure;
plot(x, y, 'o', x, y_fit, '-');
xlabel('x');
ylabel('y');
title('Linear Fit');
legend('Data', 'Fit');
Nonlinear Curve Fitting with `fit`
For nonlinear fitting, the `fit` function allows you to specify various models. Choose a model that resembles the nature of the data; for instance, if you suspect a quadratic relationship, you can use:
x = [1, 2, 3, 4, 5];
y = [2, 4.5, 6.5, 8, 10];
ft = fit(x', y', 'poly2'); % Quadratic fit
This command fits a quadratic polynomial to your data, providing a more accurate representation of nonlinear relationships.
Interpreting the Fitted Results
The `fit` function provides an object that contains useful information regarding the curve fit, including coefficients and statistics indicating the goodness of fit. Visualizing this fit is critical:
plot(ft, x, y);
Customizing the Fit
Adjusting Fit Options
MATLAB allows you to customize fit options to enhance accuracy. This customization might involve setting tolerances, weights, or specifying bounds for coefficients, which can significantly impact the output of your models.
Residual Analysis
Residuals, the differences between the observed and fitted values, are vital for assessing the fit quality. To analyze residuals and ensure that they form a random pattern, compute them using:
y_fit = feval(ft, x);
residuals = y - y_fit;
Visualize residuals by plotting them against the independent variable:
figure; plot(x, residuals, 'o');
xlabel('x');
ylabel('Residuals');
title('Residual Plot');
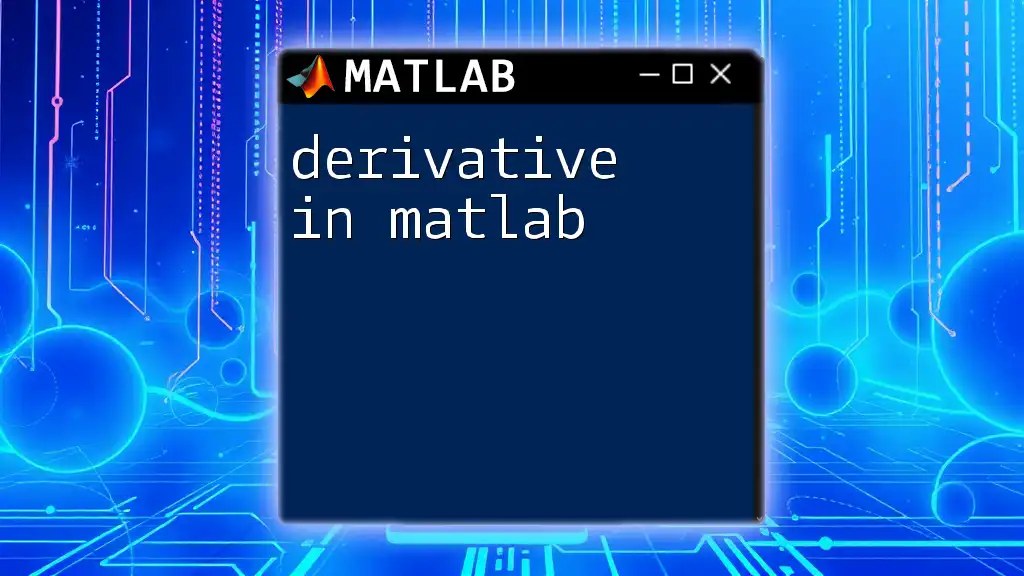
Visualizing the Curve Fit
Plotting the Fit
Combining original data points with fitted results in a single plot is vital for clarity. This representation allows for easy visual verification of the fit's accuracy:
plot(x, y, 'o', x, feval(ft, x), '-');
legend('Data', 'Fit');
grid on;
Including Confidence Intervals
Incorporating confidence intervals enhances the analysis by displaying the uncertainty around the fit. Use:
[y_fit, delta] = predint(ft, x, 0.95, 'functional', 'off');
hold on;
plot(x, y_fit + delta, 'r--');
plot(x, y_fit - delta, 'r--');
hold off;
This code snippet provides a visual representation of potential variability around the fitted curve.
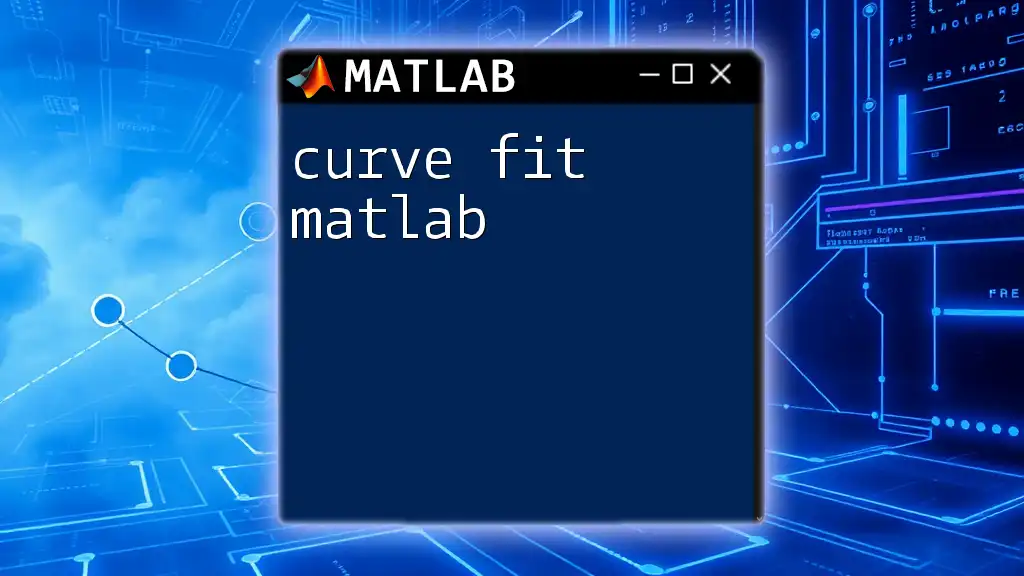
Practical Applications of Curve Fitting
Real-world Examples
Consider an environmental scientist analyzing pollutant levels over time. They can apply curve fitting techniques to predict future levels based on historical data. Similarly, in finance, regression models predict stock prices based on patterns observed in historical data. These examples underline the importance of understanding how to fit a curve in MATLAB to facilitate data-driven decision-making.
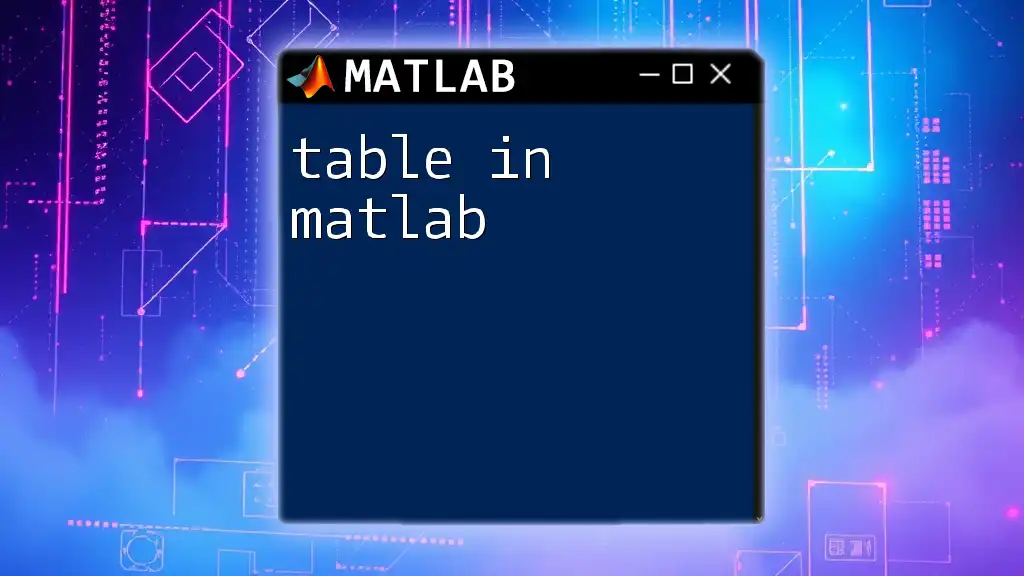
Common Pitfalls and Troubleshooting
Errors in Fitting
While fitting curves, common mistakes include selecting the wrong model type or failing to preprocess the data adequately. Always review the output to ensure it aligns with expectations.
Resources for Further Learning
For those eager to dive deeper into MATLAB curve fitting, various resources can aid your journey. Online MATLAB documentation and community forums provide valuable insights and assistance. Engaging with these communities can accelerate learning and help overcome challenges.
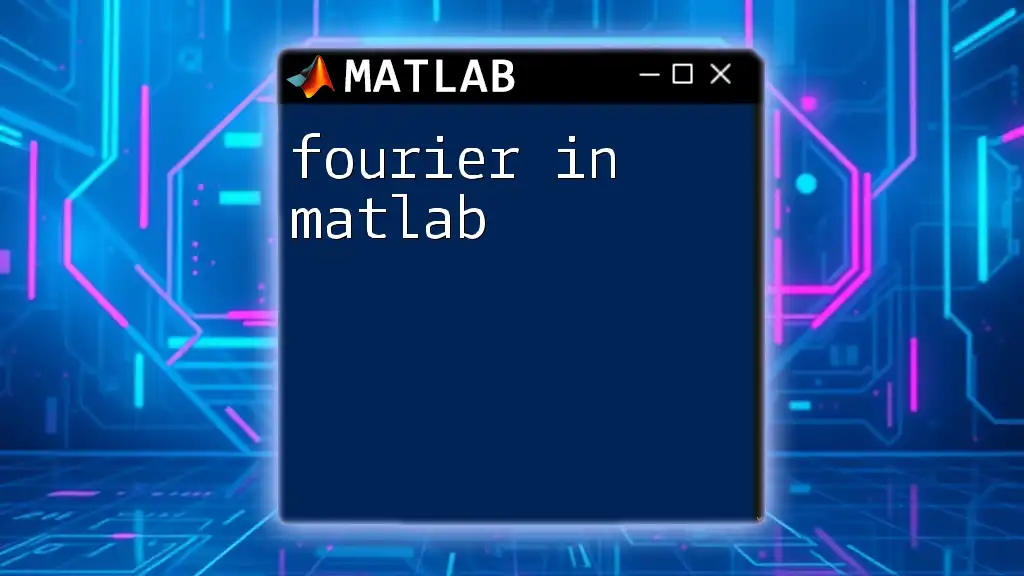
Conclusion
In summary, understanding how to fit a curve in MATLAB is a critical skill for analyzing data. From linear and nonlinear fittings to customizing your models, the steps highlighted provide a practical approach to mastering curve fitting in MATLAB. With practice and experimentation, you will enhance your capabilities in data analysis and predictive modeling, essentially enabling better insights from your data.