The `nargin` function in MATLAB is used to determine the number of input arguments that were passed to a function.
Here's a simple example:
function exampleFunction(varargin)
disp(['Number of input arguments: ', num2str(nargin)]);
end
What is `nargin`?
`nargin` is a built-in function in MATLAB that plays a crucial role in managing function input arguments. It returns the number of input arguments that are passed to a function when it is called. The primary benefit of `nargin` is that it allows developers to create more flexible functions by accommodating varying numbers of input parameters without needing to define every possible combination of inputs.
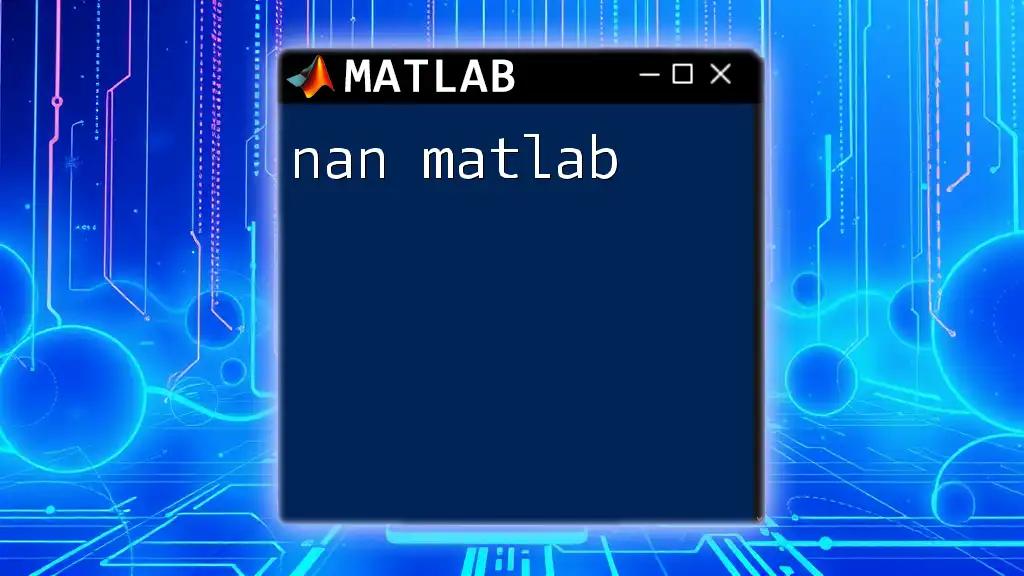
Why Use `nargin`?
Using `nargin` enhances function flexibility, enabling developers to create functions that can handle optional arguments efficiently. For instance, in situations where you want a function to perform a specific operation based on one or more inputs, `nargin` can determine how the function behaves, leading to cleaner, more maintainable code.
Managing Variable Inputs
In practical terms, this means you can create a single function that performs multiple operations based on the number of inputs provided. This approach not only simplifies code management but also improves user experience by allowing customization.
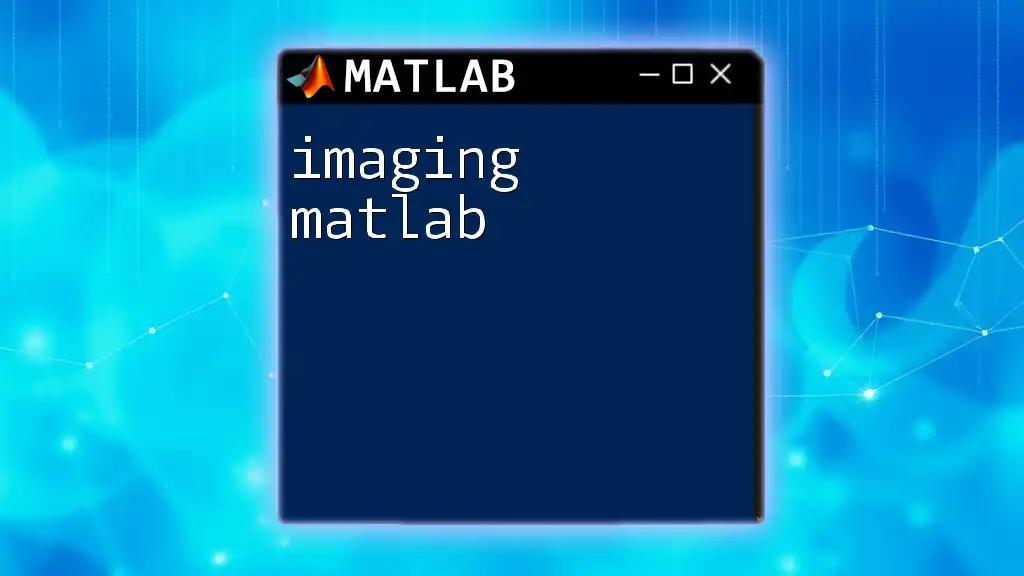
Understanding Function Inputs in MATLAB
Overview of Function Arguments
Positional Input Arguments
In MATLAB, positional input arguments are those that the function expects in a specific order. Each argument corresponds to a specific variable within the function. For example, if you have a function defined as `function myFunc(a, b)`, `a` and `b` are positional arguments required in that order.
Optional Input Arguments
Optional input arguments are those that a function can accept but are not mandatory. These arguments can provide additional functionality or settings, which is particularly useful for enhancing a function's capabilities without adding complexity.
Variable Input Arguments
Functions can also use variable input arguments, which are defined with the `varargin` notation. This notation allows for an unspecified number of inputs. Here, `nargin` can be instrumental in determining how many arguments were provided.
How `nargin` Works
The `nargin` function operates by returning an integer value that reflects the quantity of inputs received by the function. For example, if you define a function with three potential inputs, calling it with just one will return a value of 1 when using `nargin`.
Difference Between `nargin` and `nargout`
To clarify, `nargout` is another built-in function that returns the number of output arguments a function can produce. While `nargin` focuses on inputs, `nargout` allows you to control how many outputs are returned, which can further enhance function versatility.
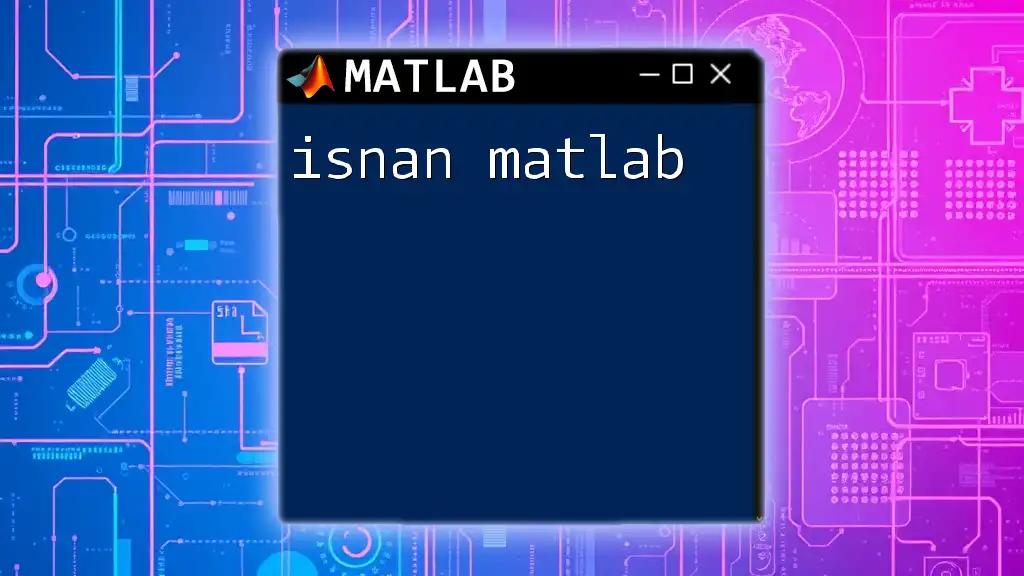
Practical Applications of `nargin`
Basic Use Cases
Creating Functions with Optional Parameters
You can use `nargin` effectively to create functions that set default values for optional parameters. Consider the following example:
function myFunction(arg1, arg2, arg3)
if nargin < 3
arg3 = 'default value'; % Default value for arg3
end
fprintf('arg1: %s, arg2: %s, arg3: %s\n', arg1, arg2, arg3);
end
In this scenario, if the user calls `myFunction('first', 'second')`, `arg3` will automatically be set to `"default value"` since only two arguments were provided.
Advanced Applications
Dynamic Function Behavior Based on Input Count
You can allow your functions to adapt their behavior based on the number of inputs. For example:
function myOperation(varargin)
switch nargin
case 1
fprintf('Only one input: %s\n', varargin{1});
case 2
fprintf('Two inputs: %s and %s\n', varargin{1}, varargin{2});
otherwise
fprintf('More than two inputs\n');
end
end
In this example, `myOperation` performs different actions depending on how many arguments the user supplies.
Error Handling with `nargin`
When developing functions, it is often essential to validate input arguments. Here’s how you can achieve this with `nargin`:
function validateInput(arg1, arg2)
if nargin < 2
error('Two inputs are required.')
end
% Proceed with function logic
end
This function checks whether at least two arguments were provided and raises an error if not, ensuring that the function's logic is only executed with valid inputs.
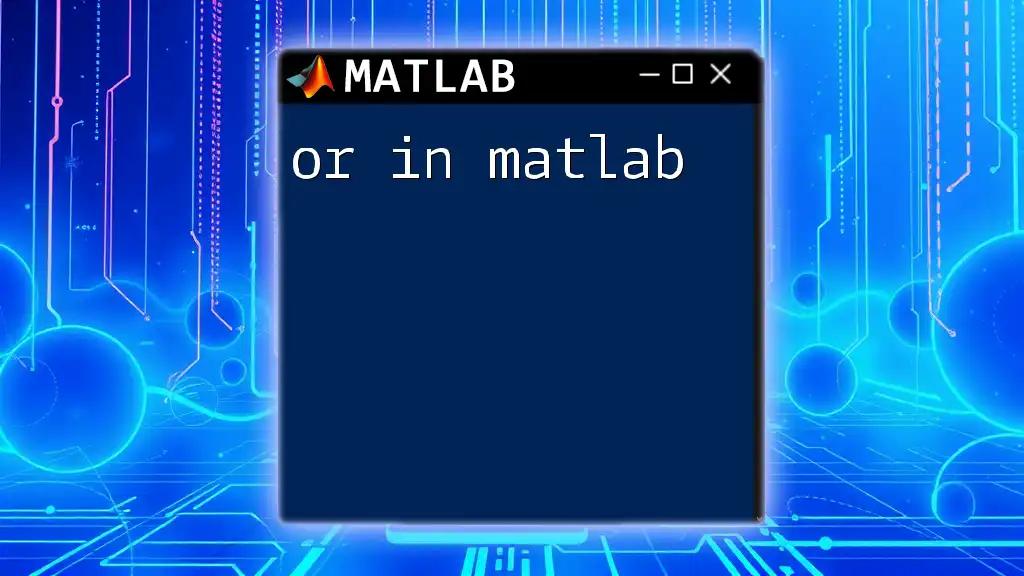
Examples of Common Functions Using `nargin`
MATLAB Built-in Functions
MATLAB has numerous built-in functions that utilize `nargin` to manage variable input handling effectively. For example, the `plot` function behaves differently when passing varying numbers of inputs, allowing users to customize their plots seamlessly based on their data size.
User-defined Functions
Creating robust user-defined functions using `nargin` is essential for attaining high-quality MATLAB code. Consider a function that encompasses different mathematical operations where the input might vary depending on the operation required.
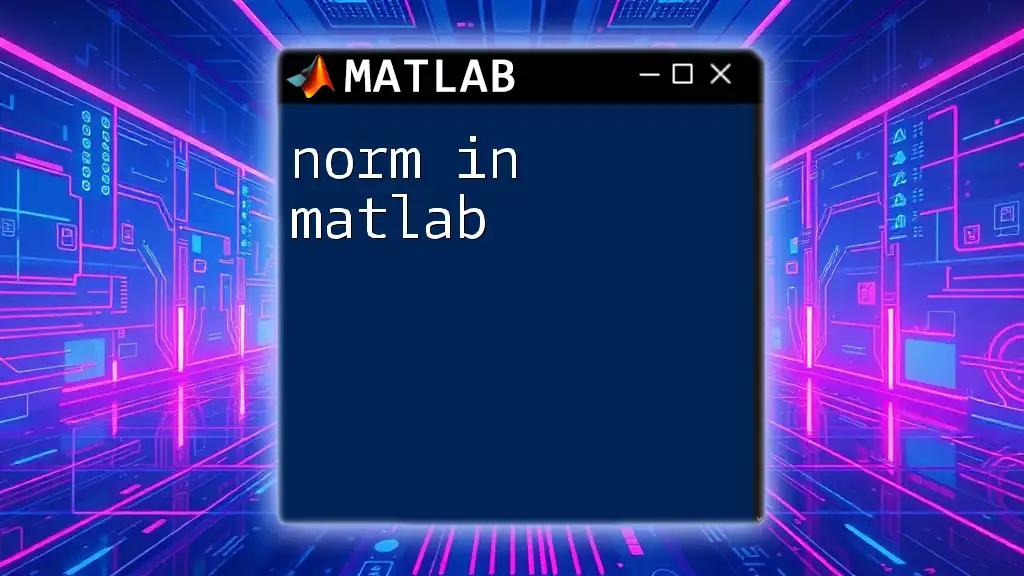
Best Practices for Using `nargin`
Tips for Implementation
When implementing `nargin`, it’s essential to define its use early in your function design. Determine whether your function will have optional parameters and how you want to structure the inputs. Additionally, avoid overly complicated input validation, as this can lead to performance bottlenecks.
Performance Considerations
While `nargin` is a useful tool, be mindful of potential performance overhead in complex functions involving many optional parameters. Ensure you optimize your functions to maintain efficient execution and prevent unnecessary computational delays.
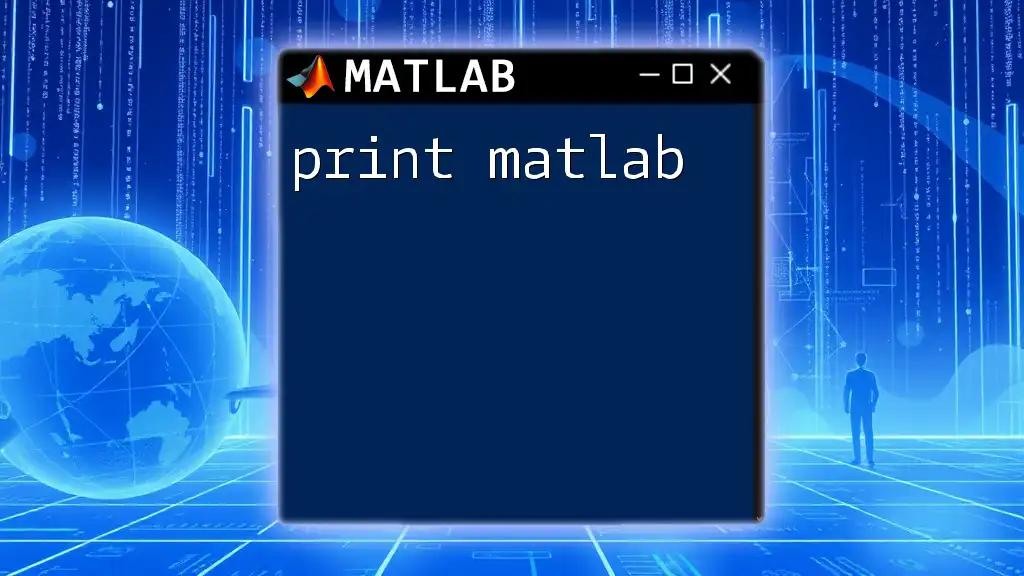
Conclusion
Mastering `nargin` in MATLAB is vital for developing dynamic, user-friendly functions. It offers a robust method for handling input arguments efficiently, allowing developers to create versatile and maintainable code. As you progress in your MATLAB journey, exploring `nargin` will undoubtedly enhance your programming capabilities and simplify function management.
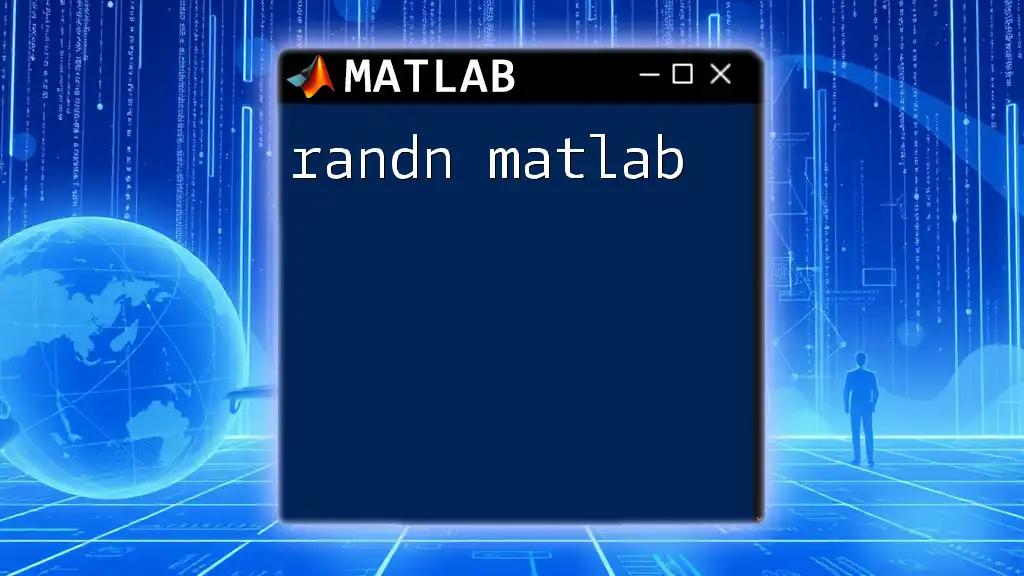
Additional Resources
For further reading on `nargin` and other MATLAB features, consider checking out the official MATLAB documentation, community forums, and online tutorials that provide deeper insights into this powerful function.
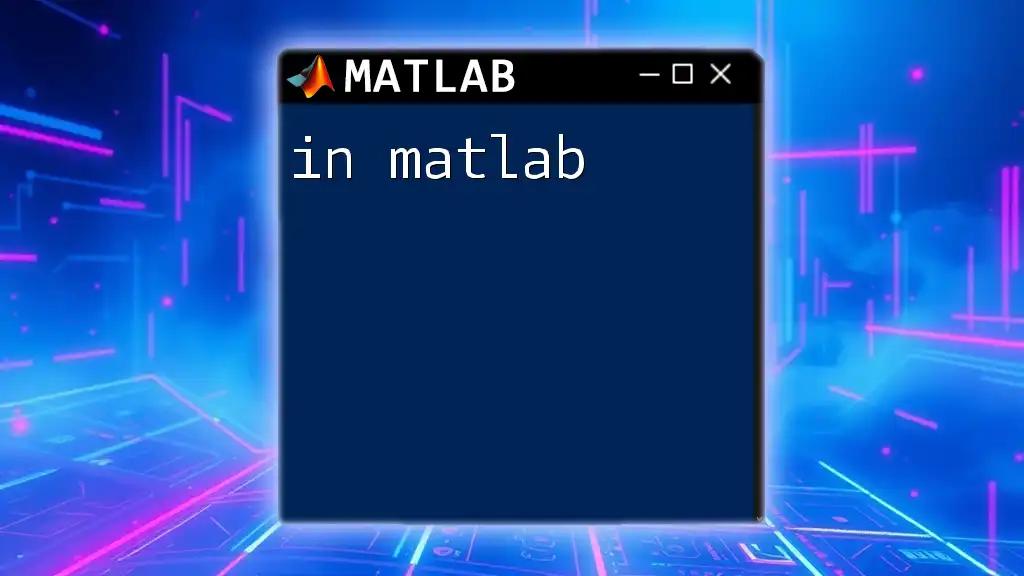
Call to Action
If you're interested in improving your MATLAB skills, join our community for more tips, guides, and training sessions tailored to your needs. Don’t hesitate to reach out for personalized tutoring to help you master MATLAB efficiently!