The `fsolve` function in MATLAB is used to find the roots of a system of nonlinear equations by providing an initial guess for the solution.
Here’s a simple code snippet demonstrating its use:
% Define the system of equations
function F = myEquations(x)
F(1) = x(1)^2 + x(2)^2 - 4; % x^2 + y^2 = 4
F(2) = x(1) - x(2) - 1; % x - y = 1
end
% Initial guess
x0 = [1; 1];
% Solve the system of equations
solution = fsolve(@myEquations, x0);
disp(solution);
Understanding Nonlinear Equations
What are Nonlinear Equations?
Nonlinear equations are mathematical expressions in which the variable(s) are raised to a power greater than one or are involved in products with other variable(s). Unlike linear equations, which graph as straight lines, nonlinear equations can result in curves, surfaces, or more complex shapes.
For example, the equation \( x^2 + y^2 = 1 \) describes a circle, demonstrating nonlinearity due to the terms being squared. In contrast, the equation \( 2x + 3y = 6 \) is linear as it can be graphed as a straight line.
Why Use fsolve?
Solving nonlinear equations analytically can often be complex or even impossible. Numerical methods like fsolve provide a powerful alternative to find approximate solutions. The fsolve function in MATLAB specializes in solving systems of nonlinear equations by employing iterative techniques.
Using fsolve is particularly beneficial when dealing with real-world problems where exact solutions might not exist or when the equations are too intricate for analytical methods. Common applications include fields like engineering, physics, finance, and any domain requiring optimization or root-finding.
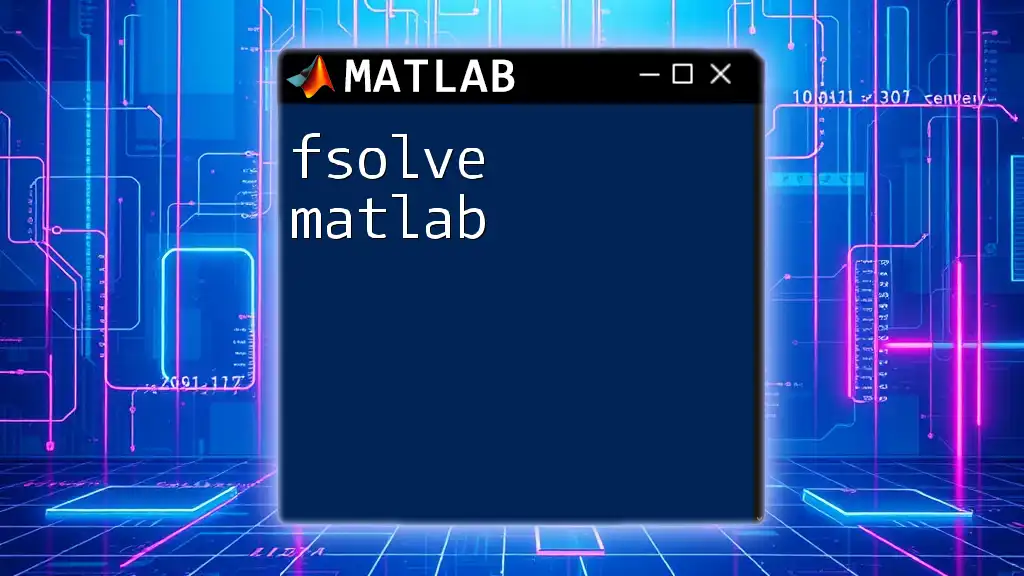
Getting Started with fsolve
Required Toolboxes
Before using fsolve, ensure that you have the MATLAB Optimization Toolbox. This toolbox is necessary for the function's advanced numerical capabilities. Check your installed toolboxes by navigating to the "Add-Ons" menu in MATLAB or by running the following command:
ver
If you don’t see the Optimization Toolbox listed, you can install it via the Add-Ons menu in MATLAB.
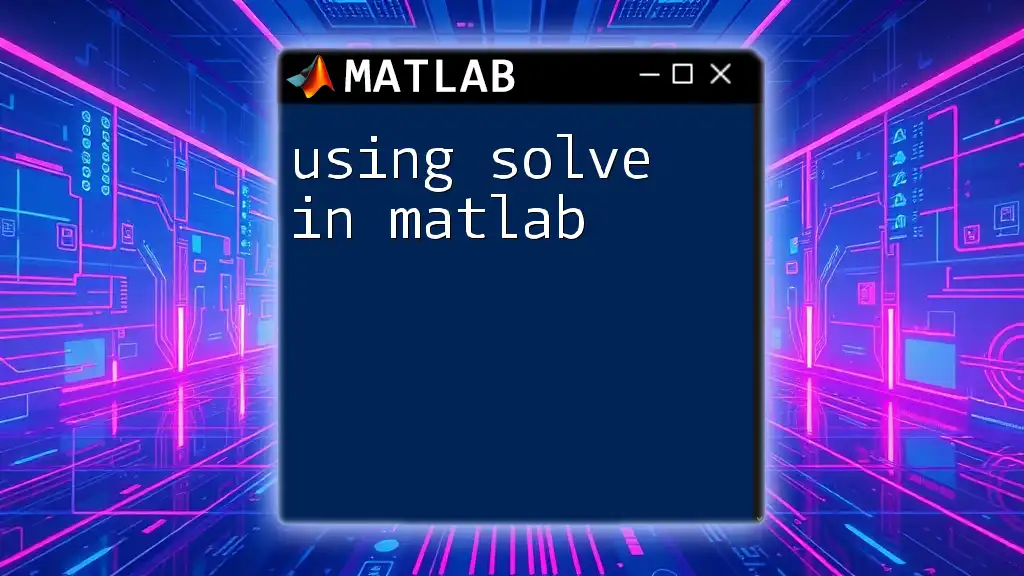
Basic Syntax of fsolve
Structure of the Command
The typical syntax for fsolve is as follows:
x = fsolve(fun, x0, options)
Components of the Syntax:
- fun: This can be a function handle or an anonymous function that defines the equations to solve.
- x0: An initial guess for the solution. Providing a good starting point is crucial for fsolve to converge to the correct solution.
- options: This optional argument allows for customization of the solver's settings (e.g., tolerances, display format).
Example of Basic Usage
Here’s a straightforward example demonstrating how to use fsolve to find the root of a basic polynomial equation:
fun = @(x) x.^2 - 4;
x0 = 1;
solution = fsolve(fun, x0);
In this example, we define a function fun that represents the equation \( x^2 - 4 = 0 \). The initial guess \( x0 = 1 \) directs fsolve to begin at \( x = 1 \). Running the above code will yield \( \text{solution} = 2 \) which is one root of the equation (the other root is -2).
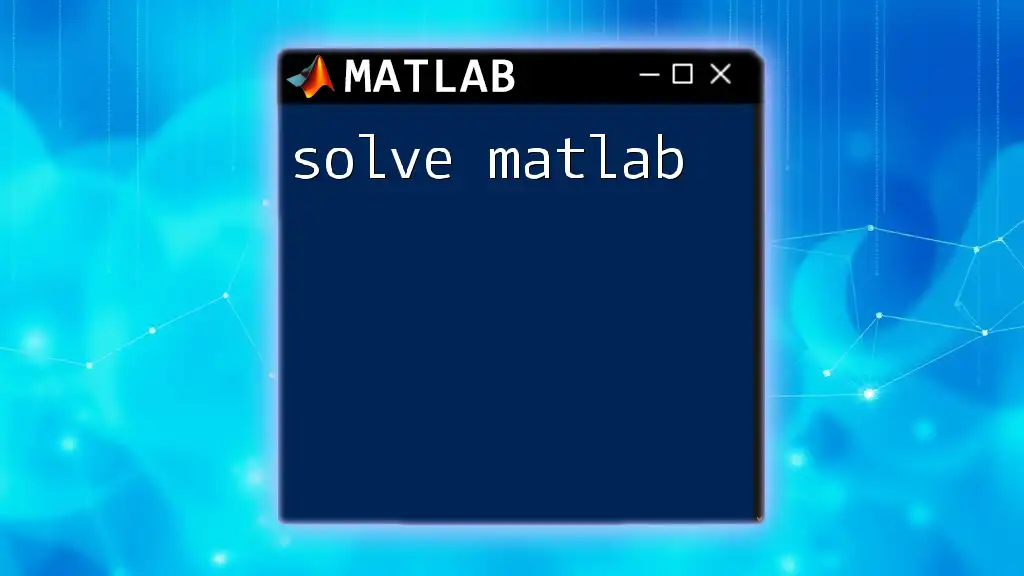
Advanced Usage of fsolve
Using fsolve with Multiple Equations
Fsolve can also be utilized to handle systems of nonlinear equations. For instance, consider the system of two equations represented in vector form:
- \( x_1^2 + x_2^2 - 1 = 0 \)
- \( x_1^2 - x_2 = 0 \)
The corresponding MATLAB code would look like this:
fun = @(x) [x(1)^2 + x(2)^2 - 1; x(1)^2 - x(2)];
x0 = [0; 0];
solution = fsolve(fun, x0);
Here, x(1) and x(2) represent the variables. The initial guess is set to the point \( [0; 0] \). Upon running this code, you'll find that the solution yields the intersection points of these equations on a graph.
Setting Options for fsolve
Customizing the behavior of fsolve can enhance its performance altogether. Use the `optimoptions` function to adjust settings like tolerances and output display:
options = optimoptions('fsolve', 'Display', 'iter', 'TolFun', 1e-6);
solution = fsolve(fun, x0, options);
In this example, the `Display` option is set to iter, allowing you to see the progress during iterations. TolFun specifies the tolerance for convergence—lowering this value typically requires finer solution accuracy.
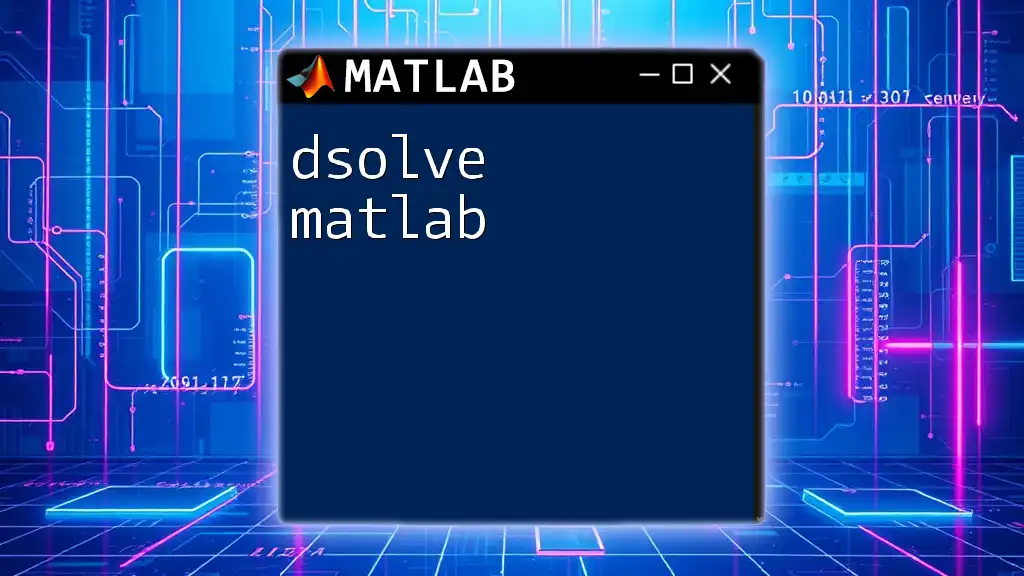
Error Handling and Debugging
Common Errors with fsolve
While using fsolve, you may encounter several common errors. For instance, if you receive a message indicating “Solution is not feasible,” it likely means that the initial guess does not lead to a valid solution or that the equations are inconsistent.
To troubleshoot these issues, consider the following strategies:
- Reassess your initial guess: A better estimation can often lead to successful convergence.
- Simplify your equations: Ensure they are correctly formulated and check for any erroneous terms.
Tips for Successful Convergence
For optimal performance when using fsolve, adhere to these guidelines:
- Choose Initial Guesses Wisely: A good initial guess can significantly improve convergence speed and accuracy. Visualize your equations to make informed guesses.
- Normalize Equations: Scaling down large numbers can help improve numerical stability and convergence.
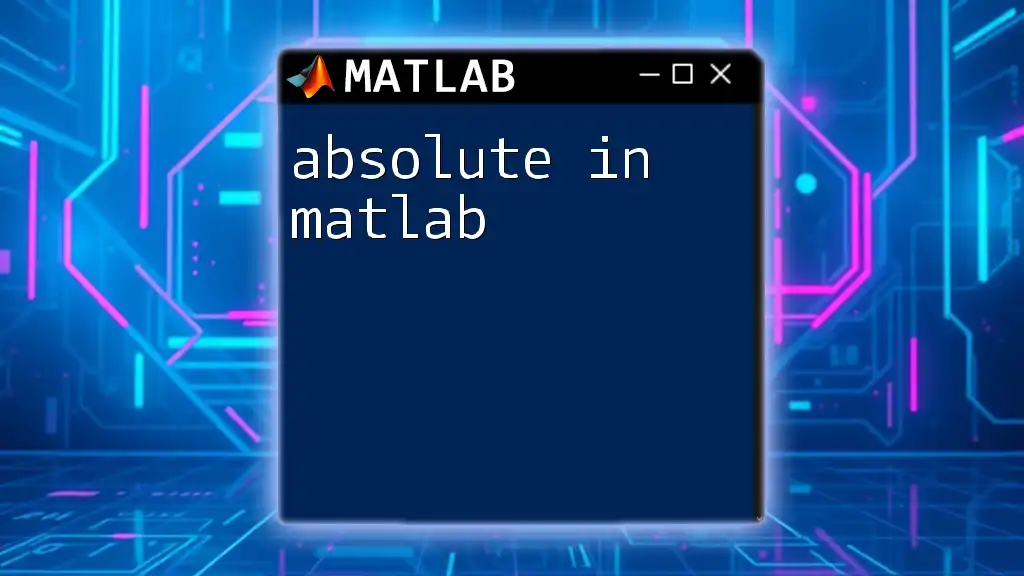
Practical Applications of fsolve
Real-World Example: Engineering Design
In mechanical engineering, you might find yourself needing to solve nonlinear equations while designing structures. For instance, consider a scenario where you're computing stress and strain in materials using the nonlinear relationships defined by the material properties. Using fsolve, you can quickly obtain solutions that are critical for validating your designs.
Applications in Finance
In the finance sector, fsolve can be employed to model complex financial instruments. For example, if you are evaluating the price of an option using the Black-Scholes model, you might encounter nonlinear equations that require numerical solutions. Here’s a simplified illustration:
fun = @(x) option_price(x(1), x(2)) - market_price; % Define your equations
x0 = [initial_guess1; initial_guess2]; % Initial estimates
solution = fsolve(fun, x0);
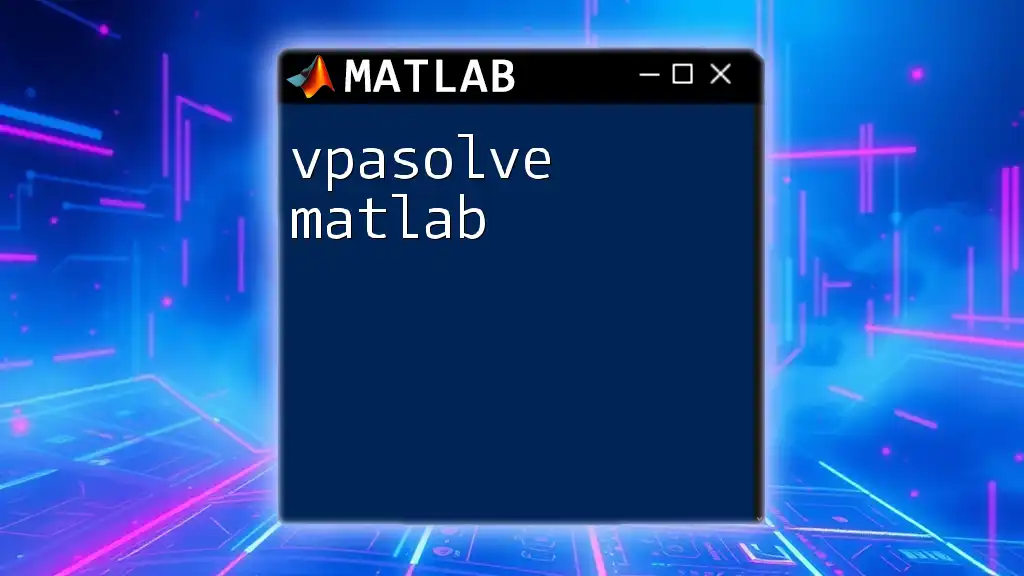
Conclusion
The fsolve function in MATLAB is a powerful tool for tackling systems of nonlinear equations effectively. By understanding its syntax, options, and practical applications, users can solve complex scientific and engineering problems with ease. Remember to experiment with different types of equations and embrace the iterative nature of numerical computation.
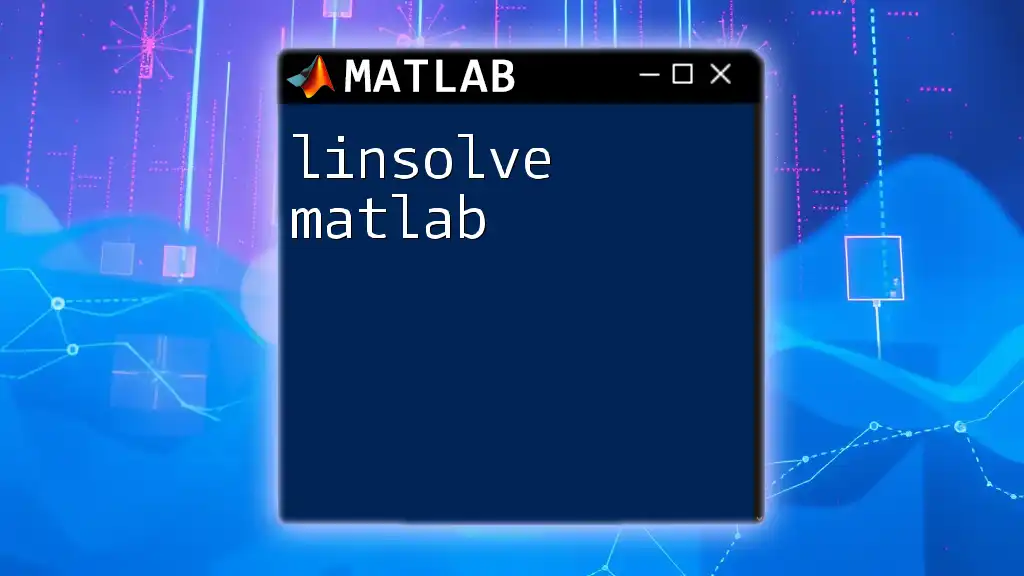
Additional Resources
For deeper learning, consider exploring the official MATLAB documentation for fsolve and related functions. Numerous textbooks and online courses offer extensive coverage of MATLAB's capabilities, enhancing your skills for more advanced applications.
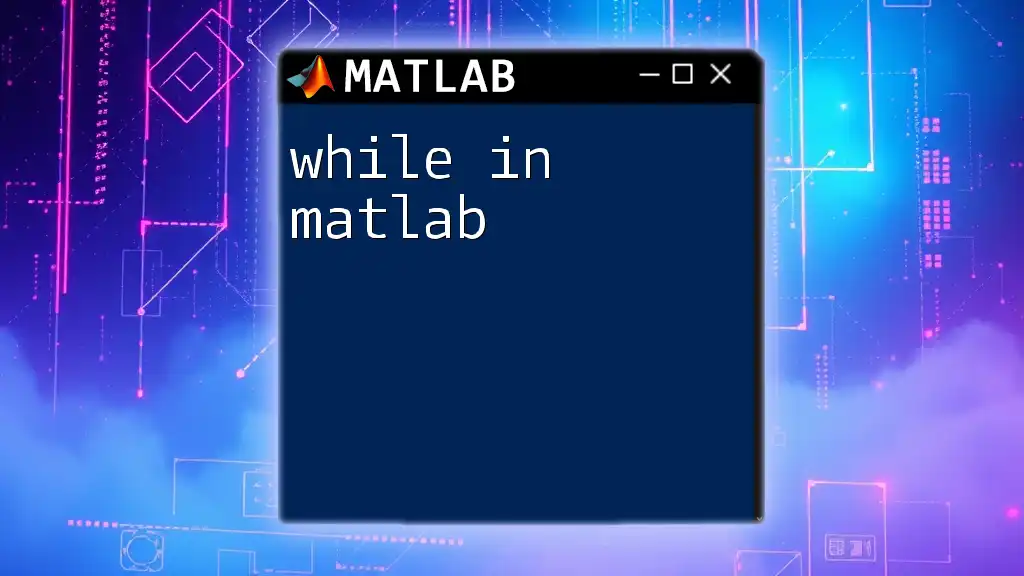
FAQs
What are the limitations of fsolve?
Despite its power, fsolve may struggle with certain highly complex or poorly conditioned equations. It's essential to understand when numerical methods may not yield accurate results and consider alternatives such as symbolic computation or specialized algorithms.
How does fsolve compare to other solvers in MATLAB?
While primarily used for nonlinear equations, fsolve is one option among several solvers in MATLAB, such as `lsqnonlin` (for least-squares problems) and `fminunc` (for unconstrained optimization). Each function caters to specific problem types, so your choice should align with the nature of the equations you’re addressing.