In MATLAB, a table is a data type that allows you to store and manipulate heterogeneous data in a structured format, similar to a spreadsheet.
Here’s a simple example of creating a table in MATLAB:
% Creating a table with two variables: 'Name' and 'Age'
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [25; 30; 22];
T = table(Names, Ages);
disp(T);
What is a Table in MATLAB?
A table in MATLAB is a data type that allows you to store and manage data in a structured format. Unlike arrays, tables can contain different types of data in each column, making them particularly useful for handling data sets such as those used in data analysis, statistics, and machine learning.
Tables provide a clear way to perform various data operations due to their readability and column-based format. Each column in a table can be thought of as a variable with a name, and rows represent observations.
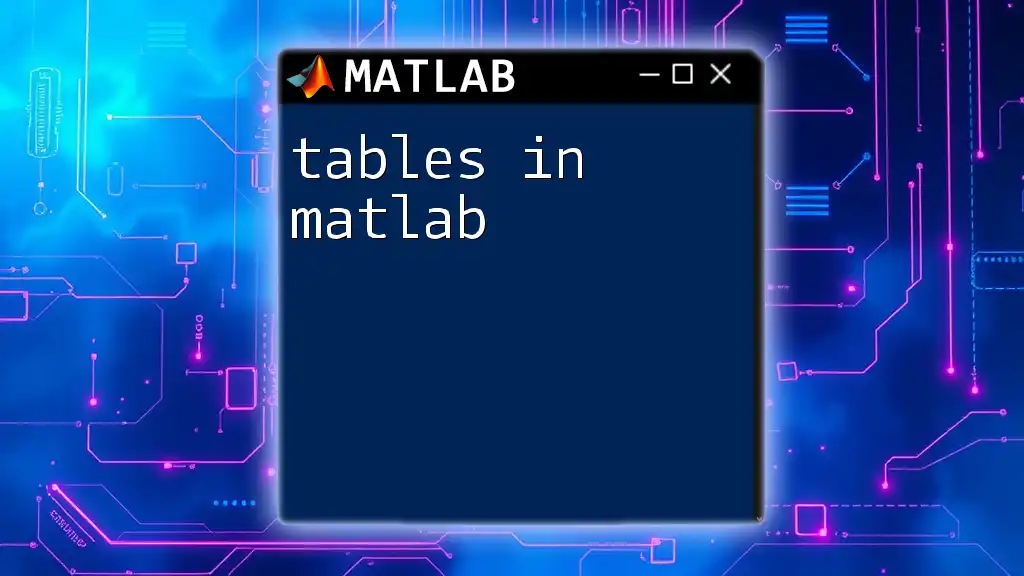
Why Use Tables?
Using tables has several advantages over other data types in MATLAB:
- Mixed Data Types: Tables can hold columns of different data types all in one structure, such as numeric, categorical, and strings.
- Improved Readability: Tables allow for clear labeling of data columns, which makes understanding and analyzing data easier.
- Built-in Functions: MATLAB includes numerous built-in functions designed specifically for tables, making operations like sorting, filtering, and grouping more straightforward than with standard arrays.
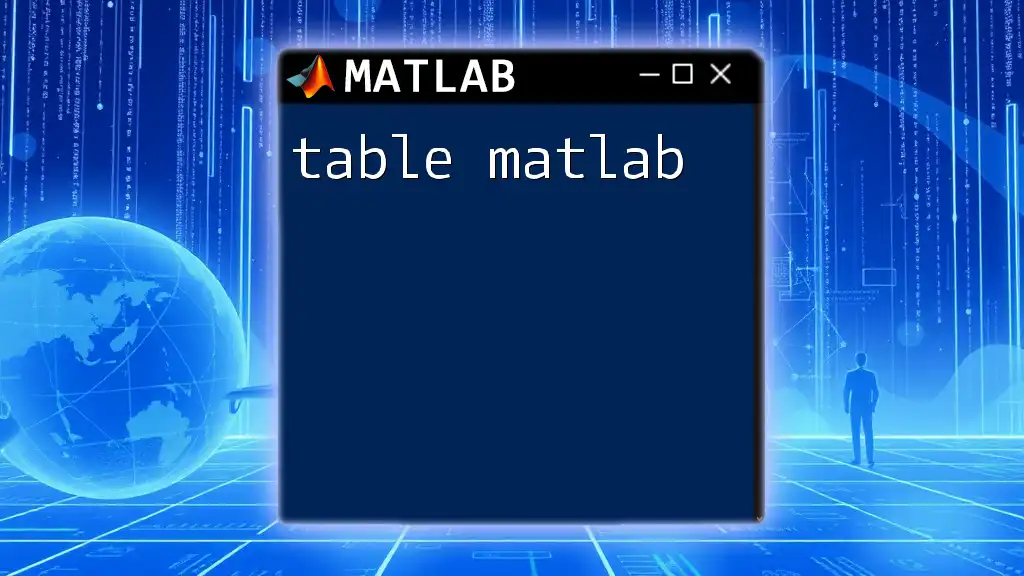
Creating Tables in MATLAB
Basic Table Creation
You can create a table in MATLAB by using the `table` function. This function takes column data as inputs.
For example:
A = [1; 2; 3];
B = ["A"; "B"; "C"];
T = table(A, B);
In this example, `A` is a column of numeric values, while `B` is a column of string values. The resulting table `T` organizes these two columns together, providing both clarity and functionality.
Specifying Column Names
You can explicitly define the names of the columns while creating the table. This is useful for better readability and data management. Here’s how to do it:
T = table(A, B, 'VariableNames', {'Numbers', 'Letters'});
In this command, `Numbers` and `Letters` are the column names that allow for easier data reference later.
Importing Data into Tables
Importing data into a table is made straightforward with the `readtable` function. This function allows you to read data from various file formats, such as CSV or Excel files. For example:
T = readtable('data.csv');
This command imports the contents of `data.csv` directly into a MATLAB table, where each column of the file corresponds to a column in the table.
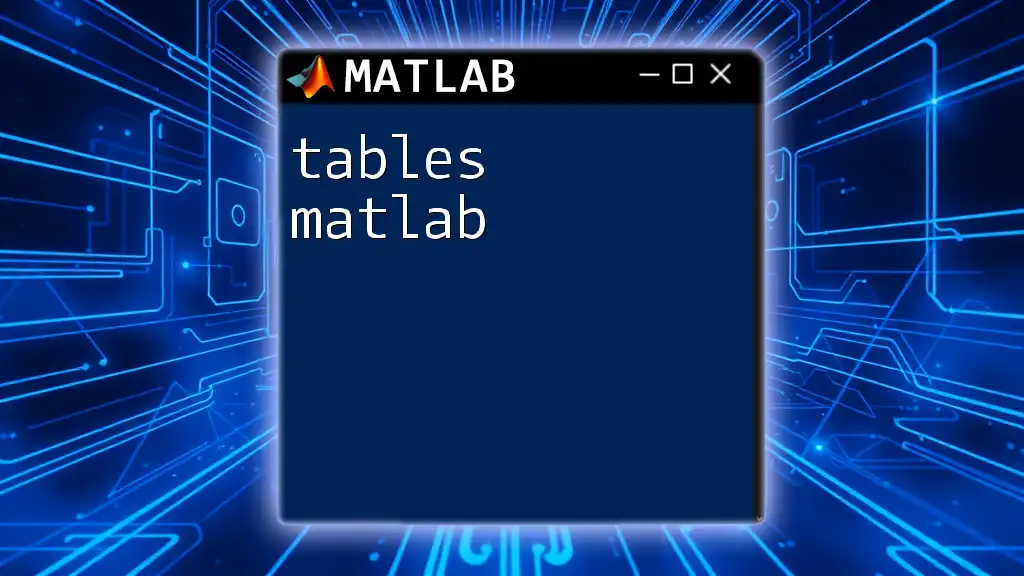
Accessing and Modifying Table Data
Accessing Data
You can access data in a table using row and column indexing. To access the first row of the table, you can use:
firstRow = T(1, :);
If you want to extract the values from a specific column, such as `Letters`, you can do so by:
lettersColumn = T.Letters;
By using column names, you can retrieve specific data much more intuitively compared to using numeric index values.
Modifying Data
Modifying entries in a table is as simple as accessing data. To update specific values in a table, use indexing. For example:
T.Numbers(1) = 10;
This command updates the first entry in the `Numbers` column to `10`.
Adding New Rows and Columns
You can easily append new rows or add new columns to existing tables.
Appending Rows: You can create a new row by defining new data and concatenating it to the existing table:
newRow = {4, "D"};
T = [T; newRow];
This adds a new row at the end of the table `T`.
Adding Columns: To add a new variable to a table, you can assign a new column like so:
T.NewColumn = [10; 20; 30; 40];
This statement introduces a new column named `NewColumn` to the table.
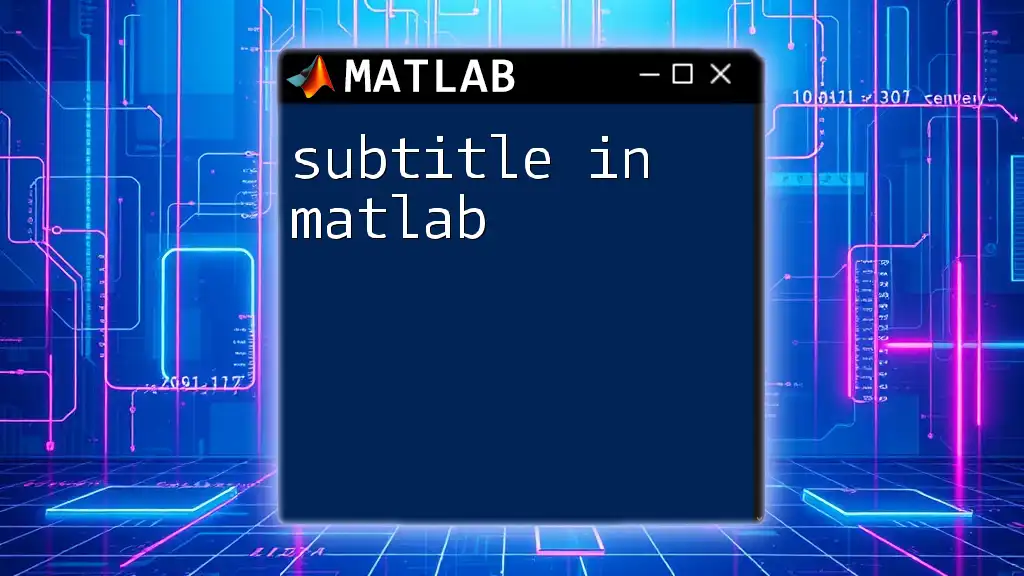
Data Manipulation and Analysis
Sorting Tables
Data within tables can be sorted based on specified column values using the `sortrows` function. For instance:
T_sorted = sortrows(T, 'Numbers');
This command sorts table `T` based on the values in the `Numbers` column, facilitating easier data analysis and interpretation.
Filtering Rows
Using logical indexing allows you to filter rows based on certain criteria. For example, if you want to obtain a table that contains only the rows where `Numbers` are greater than `2`, you can do:
filteredTable = T(T.Numbers > 2, :);
This provides a new table containing only the relevant entries, allowing for easier data management.
Handling Missing Data
Managing missing data is crucial in any data analysis process. MATLAB provides the `rmmissing` function, which removes rows containing `NaN` values from the table:
T = rmmissing(T);
This operation is essential for ensuring data quality and integrity during analytical processes.
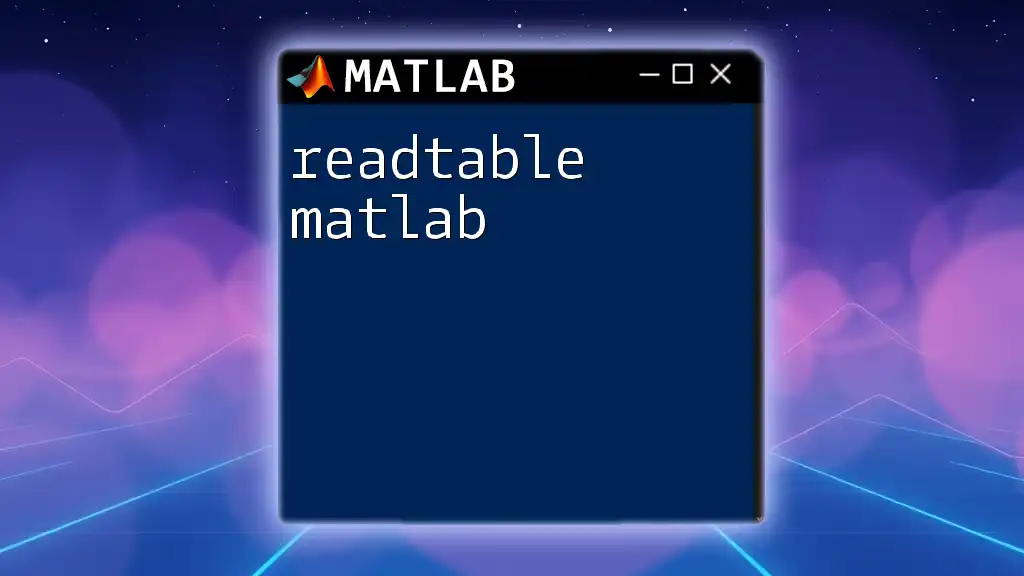
Visualizing Table Data
Plotting Table Data
Creating visualizations directly from tables can provide quick insights into the data. You can generate plots simply by referencing the table columns:
plot(T.Numbers, 'o-');
This code snippet will create a simple plot of the `Numbers` variable, revealing trends or patterns that may be present in your dataset.
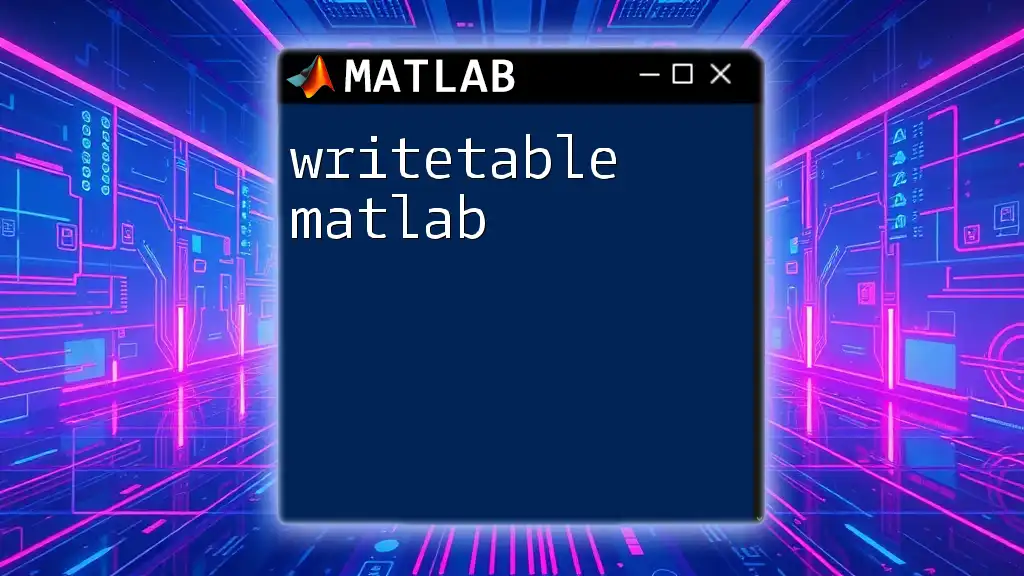
Advanced Table Operations
Merging Tables
Joining two tables is a common operation in data analysis. Using the `outerjoin` function, you can merge two tables based on common rows:
T2 = table([1; 2; 3], ["X"; "Y"; "Z"], 'VariableNames', {'Num', 'Char'});
mergedTable = outerjoin(T, T2, 'MergeKeys', true);
This command combines `T` and `T2` while merging their key variables, enhancing your data manipulation capabilities.
Grouping Data
The `groupsummary` function allows users to analyze data by summarizing it based on groups defined by one or more variables. For instance:
summaryTable = groupsummary(T, 'Letters', 'mean', 'Numbers');
This will generate a summary table that provides the mean of `Numbers` for each unique value in the `Letters` column, which can be highly useful for quick statistical analyses.
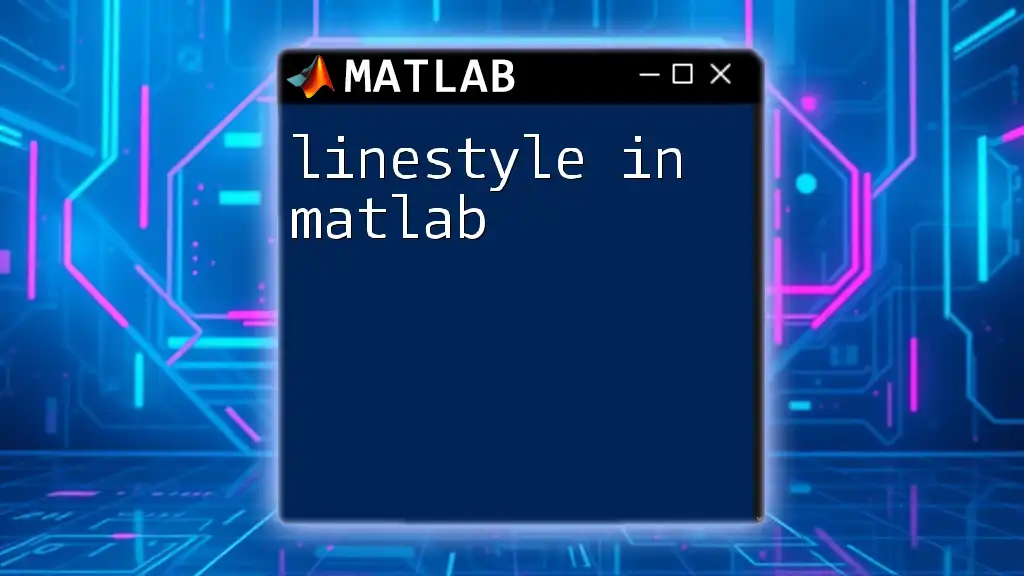
Best Practices for Using Tables in MATLAB
Performance Tips: While tables are extremely versatile, be mindful of performance. For very large datasets, consider whether a simpler data type might be more efficient.
Organizing Data: Use meaningful column names and maintain a neat structure when creating tables. This practice enhances documentation and makes it easier for others (or your future self) to understand the data layout.
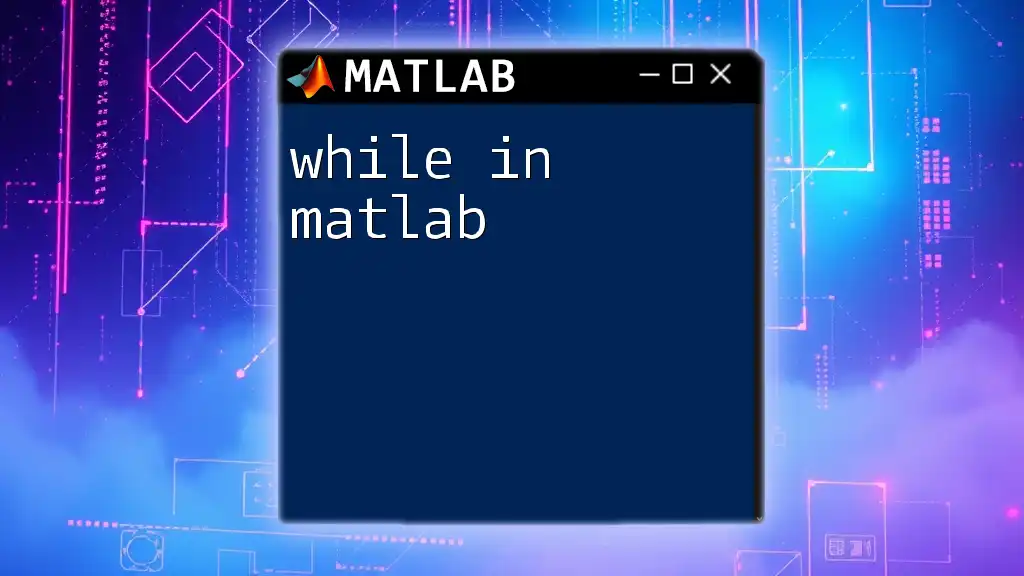
Conclusion
Tables in MATLAB provide a powerful way to organize, manipulate, and analyze data efficiently. With their ability to hold mixed data types, built-in functions for manipulation, and clear structure, they are an indispensable tool for any data analysis task. By understanding and utilizing the various functionalities of tables, you can significantly enhance your data management capabilities in MATLAB. For further learning, don’t hesitate to explore MATLAB's extensive documentation and online resources for tables.