The `solve` function in MATLAB is used to find the solutions of equations or systems of equations symbolically.
syms x; % Define the symbolic variable
solution = solve(x^2 - 4 == 0, x); % Solve the equation x^2 - 4 = 0
disp(solution); % Display the solutions
Understanding `solve`
What is `solve`?
The `solve` function in MATLAB is a powerful tool for symbolic computation that allows users to find solutions to mathematical equations. Whether you're dealing with algebraic equations, systems of equations, or even non-polynomial expressions, `solve` can handle it all. Its versatility makes it essential for engineers, scientists, and mathematicians alike, as it enables them to quickly derive formulae and understand system behavior without resorting to numerical approximations.
Syntax of `solve`
The general structure of the `solve` command can be described as follows:
solution = solve(equation, variable)
Here, equation refers to the mathematical expression you want to solve, and variable specifies the unknown(s) for which you seek a solution. You can also include options for returning multiple or specific solution types, making `solve` a flexible tool tailored to various requirements.
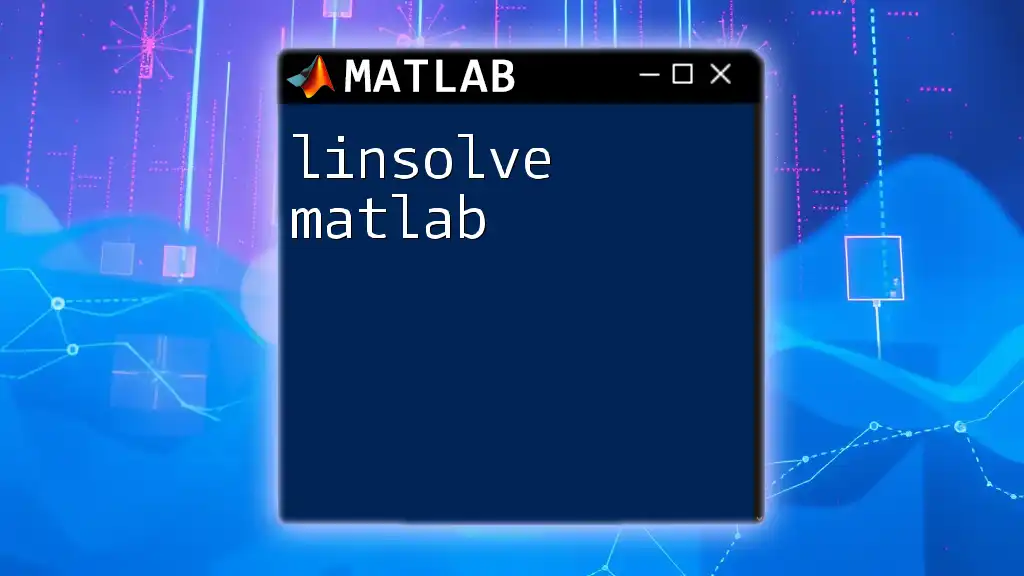
Getting Started with `solve`
Setting Up MATLAB for Symbolic Computation
Before diving into using `solve`, ensure that the Symbolic Math Toolbox is properly installed. You can check this by typing the following command in the MATLAB command window:
ver
Once confirmed, you can initiate symbolic computation by including this line in your script:
syms x y z
This command defines symbolic variables that can be used in subsequent equations.
Basic Examples of `solve`
Solving Simple Equations
Let’s start with a basic linear equation. Consider the equation \(2x + 3 = 7\). You can solve it as follows:
syms x;
equation = 2*x + 3 == 7;
solution = solve(equation, x)
Output Explanation: After executing the above code, MATLAB computes the value of \(x\) that satisfies the equation, returning \(x = 2\).
Solving Quadratic Equations
Now, let’s address a quadratic equation, such as \(x^2 - 5x + 6 = 0\):
syms x;
equation = x^2 - 5*x + 6 == 0;
solutions = solve(equation, x)
Output Explanation: This command will give you two solutions: \(x = 2\) and \(x = 3\). MATLAB adeptly manages multiple solutions, making it an excellent choice for polynomial equations.
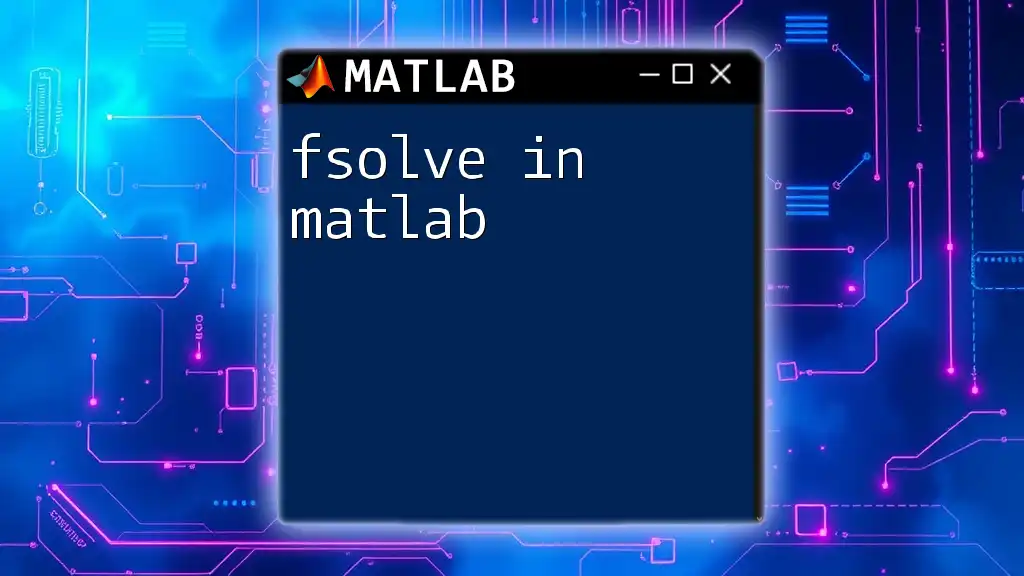
Advanced Usage of `solve`
Solving Systems of Equations
Linear Systems
Solving simultaneous equations is another strong point of `solve`. Consider a system of linear equations:
- \(2x + y = 10\)
- \(x - y = 1\)
You can represent this system in MATLAB as:
syms x y;
equations = [2*x + y == 10, x - y == 1];
solutions = solve(equations, [x, y])
Output Explanation: The code snippet will return the values \(x = 3\) and \(y = 4\). MATLAB effectively handles such systems, delivering concise solutions.
Nonlinear Systems
The `solve` function also extends to nonlinear systems. Consider the following system:
- \(x^2 + y^2 = 1\)
- \(x + y = 1\)
You can solve this system using:
syms x y;
equations = [x^2 + y^2 == 1, x + y == 1];
solutions = solve(equations, [x, y])
Output Explanation: This might yield multiple solutions—highlighting the functionality of `solve` in dealing with complex systems that involve nonlinear relationships.
Using `solve` for Symbolic Expressions
Non-Polynomial Equations
The flexibility of `solve` extends to trigonometric equations as well. For example, to solve the equation \(\sin(x) = 0.5\), you would write:
syms x;
equation = sin(x) == 0.5;
solutions = solve(equation, x)
Output Explanation: MATLAB will return a symbolic expression representing all solutions, taking into account multiple cycles of the sine function, which showcases its ability to handle periodic functions.
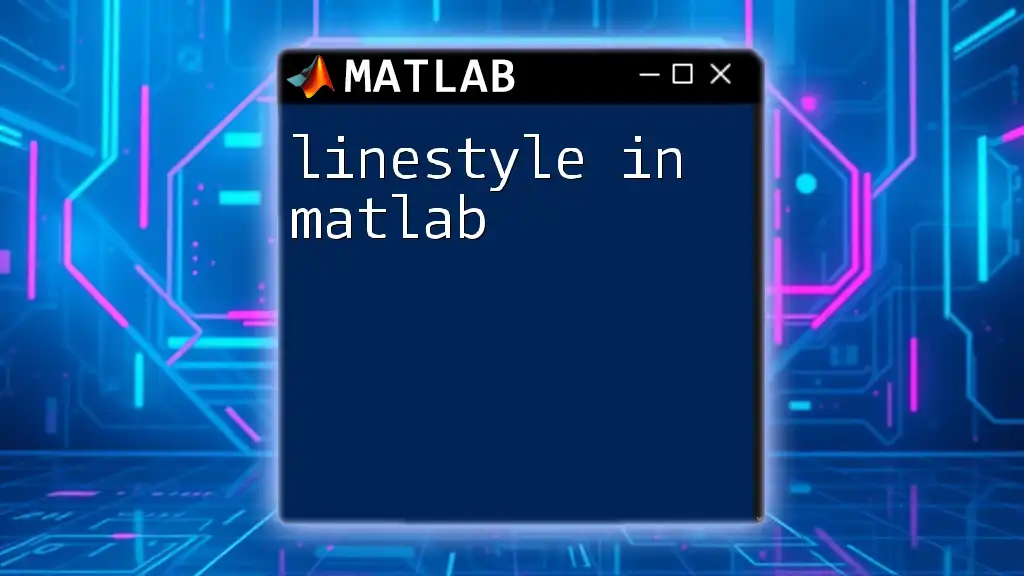
Tips and Tricks for Using `solve`
Handling No Solution or Infinitely Many Solutions
When using `solve`, you may encounter cases where no solutions exist (e.g., inconsistent equations) or where an infinite number of solutions are possible (e.g., dependent equations). Understanding the output in these scenarios is critical:
- No Solutions: MATLAB will appropriately notify you.
- Infinitely Many Solutions: MATLAB typically returns a parameterization of solutions.
Improving Performance
To enhance performance when using `solve`, consider the following:
- Rewrite Equations: Formulate equations in a simpler form if possible.
- Use Assumptions: The `assume` function allows you to specify conditions (e.g., real numbers), which can potentially speed up the solving process.
Example of using assumptions:
syms x real;
equation = x^2 - 4 == 0;
solutions = solve(equation, x)
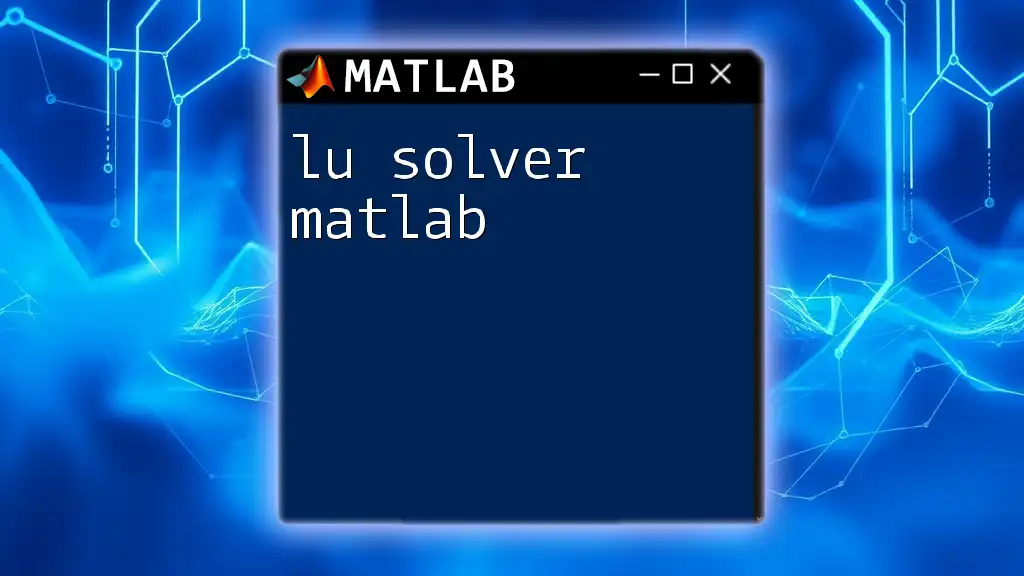
Common Errors When Using `solve`
Syntax Errors
Common mistakes often include forgetting to declare symbolic variables or incorrect representation of equations. Always review your syntax to ensure correct formatting.
Understanding Error Messages
MATLAB provides informative error messages that can guide troubleshooting. Familiarizing yourself with these messages will save time and improve your understanding of how `solve` operates.
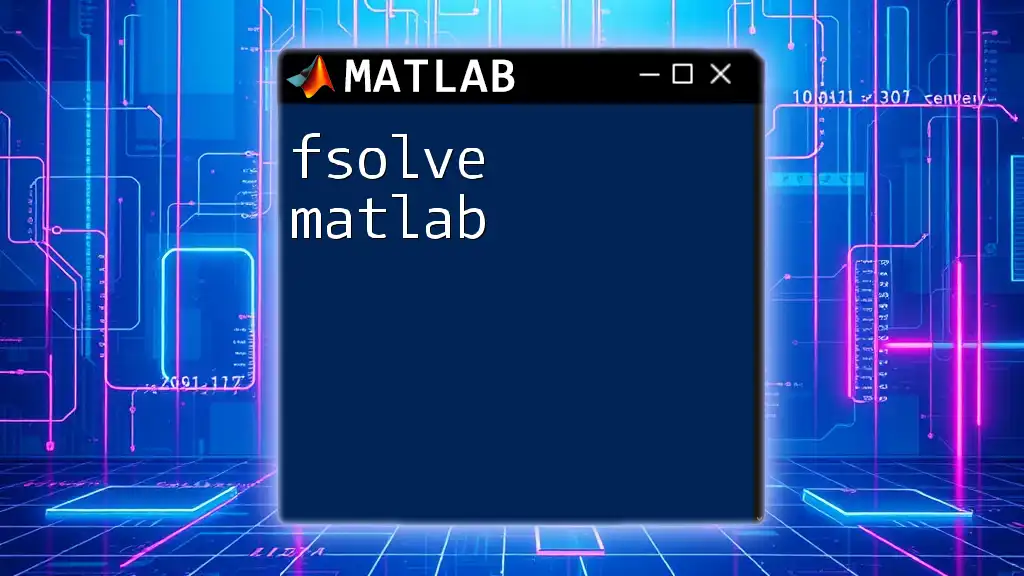
Conclusion
The `solve` function in MATLAB is a versatile and powerful tool that simplifies the process of finding solutions to a broad range of mathematical equations and systems. With its capability of handling both simple and complex scenarios, `using solve in matlab` not only aids in academic pursuits but also supports real-world applications across various fields.
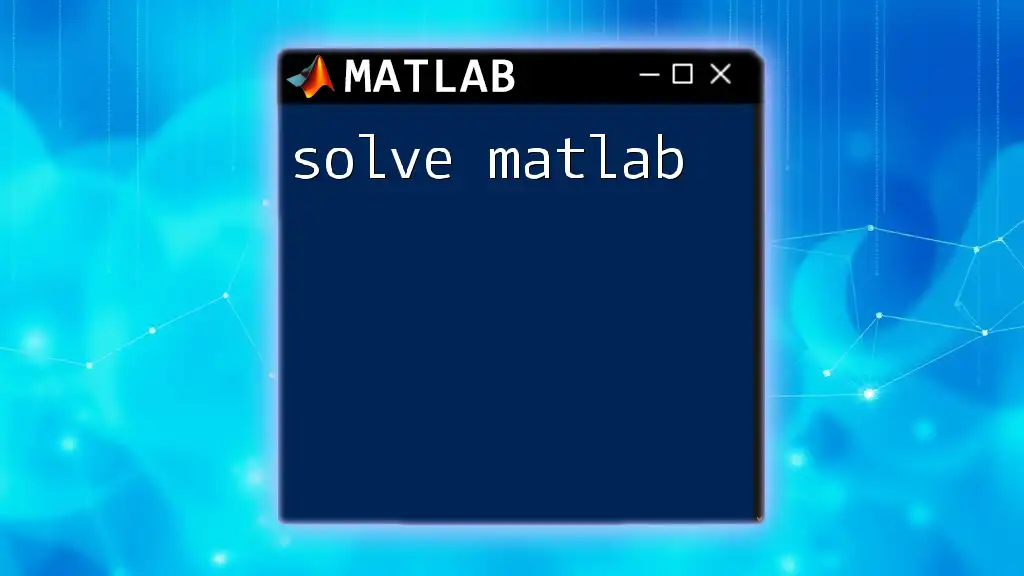
Additional Resources
For those looking to deepen their understanding of `solve`, consult the MATLAB documentation, explore online forums, and engage with tutorials that delve into advanced topics and troubleshooting strategies.
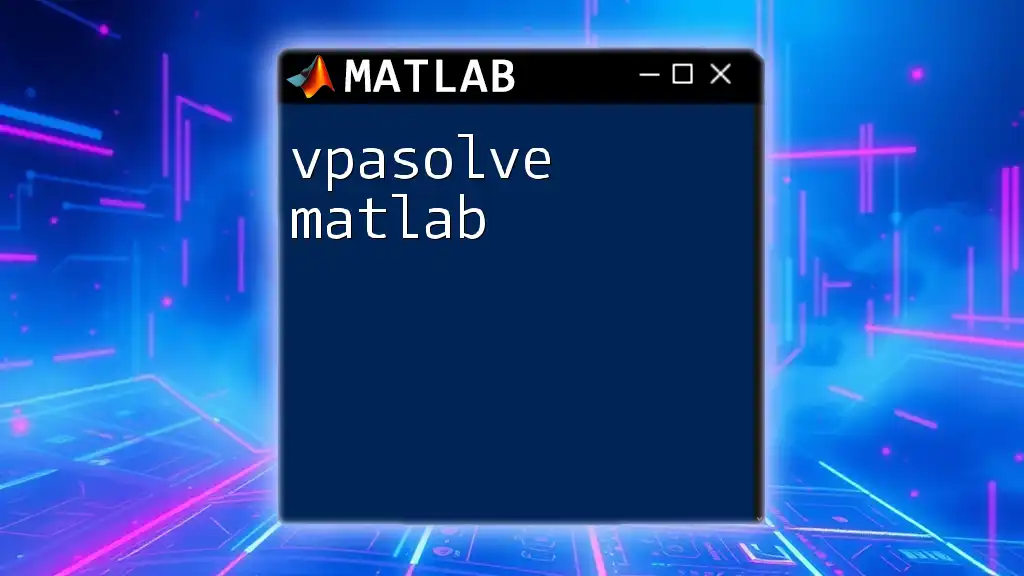
Call to Action
We invite you to experiment with `solve` in MATLAB, share your experiences, and ask questions regarding specific use cases. Engaging with the community can provide insights that enhance your learning journey!