Octave is an open-source alternative to MATLAB that offers similar functionality and syntax, making it an accessible tool for users familiar with MATLAB commands.
Here's a simple code snippet using Octave syntax:
% Example: Creating a simple plot in Octave
x = 0:0.1:10; % Generate values from 0 to 10 with increment of 0.1
y = sin(x); % Calculate the sine of each x value
plot(x, y); % Create the plot
title('Sine Wave'); % Add a title to the plot
xlabel('X-axis'); % Label the x-axis
ylabel('Y-axis'); % Label the y-axis
What is Octave MATLAB?
GNU Octave is a high-level programming language primarily intended for numerical computations. It is often seen as a free alternative to MATLAB, sharing a significant subset of its syntax and features. This compatibility allows users familiar with MATLAB to transition easily into Octave, making it an excellent choice for those seeking cost-effective computing solutions without sacrificing functionality.
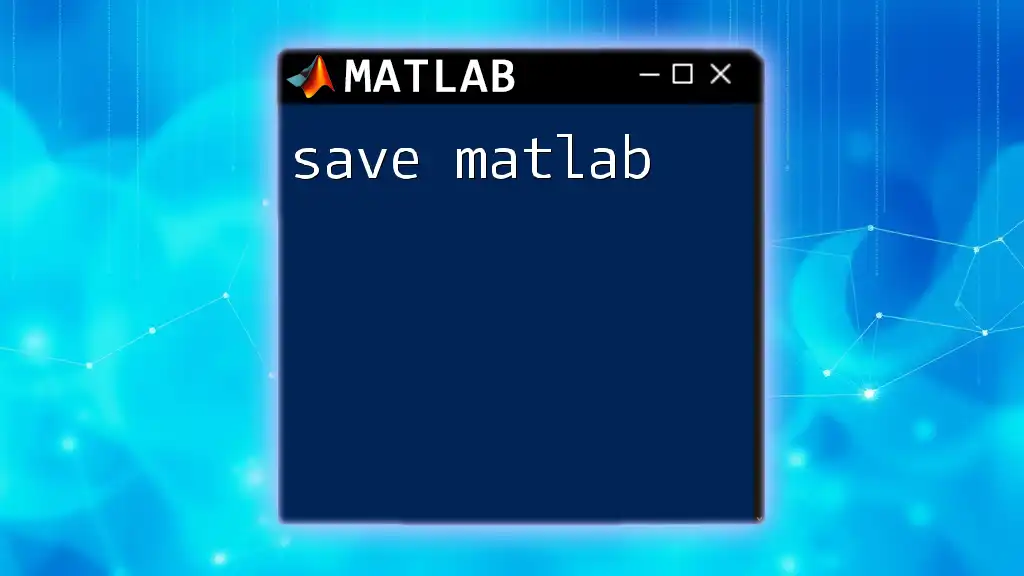
Setting Up Octave
Installation Process
To begin using Octave, the first step is to install it on your system. The installation process varies based on your platform:
- Windows: Download the installer from the GNU Octave website. Follow the on-screen instructions to complete the installation.
- macOS: For macOS, you can use Homebrew (`brew install octave`) or download the installer directly from the official site.
- Linux: Most Linux distributions have Octave available through their package managers. Use the command `sudo apt-get install octave` for Ubuntu-based systems.
Initial Configuration
Once installed, it is important to configure your Octave environment to suit your preferences. You can adjust settings such as the default working directory, script editor preferences, and the appearance of the command window. Familiarizing yourself with these settings will enhance your productivity as you work with Octave.
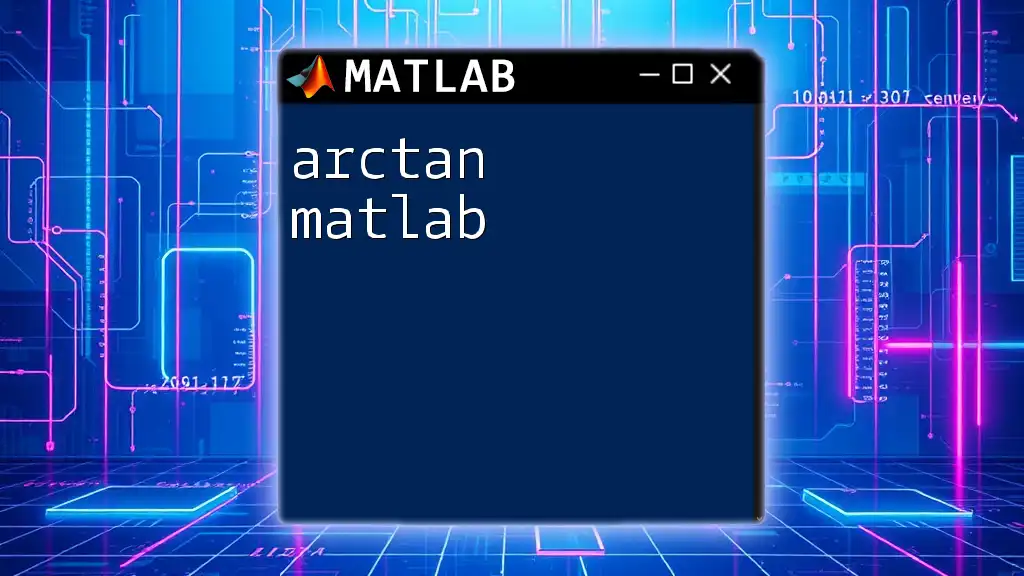
Core Features of Octave
Syntax Overview
One of the standout features of Octave is its similarity to MATLAB syntax, making it easy for users to adapt. For example, creating a matrix is straightforward:
a = [1, 2, 3; 4, 5, 6]
This command initializes a 2x3 matrix, showcasing Octave's intuitive and human-readable syntax.
Data Types and Structures
Understanding the various data types and structures in Octave is crucial. Octave supports several core types:
- Scalars: Single numeric values.
- Vectors: One-dimensional arrays that can be row or column vectors.
- Matrices: Two-dimensional arrays, like the example above.
Additionally, Octave provides more complex data structures, including cell arrays (which can hold different types of data) and structures (which can group different types of data together).
Built-in Functions
Octave comes equipped with numerous built-in functions that facilitate mathematical and statistical calculations. Below are some commonly used functions:
- Mathematical Functions: Calculate properties like sine, cosine, and exponential values. For example:
x = [0, pi/2, pi];
y = sin(x);
- Statistical Functions: Analyze data to get statistical measures. For instance, to calculate the mean of a vector:
x = [1, 2, 3, 4];
mean_value = mean(x);
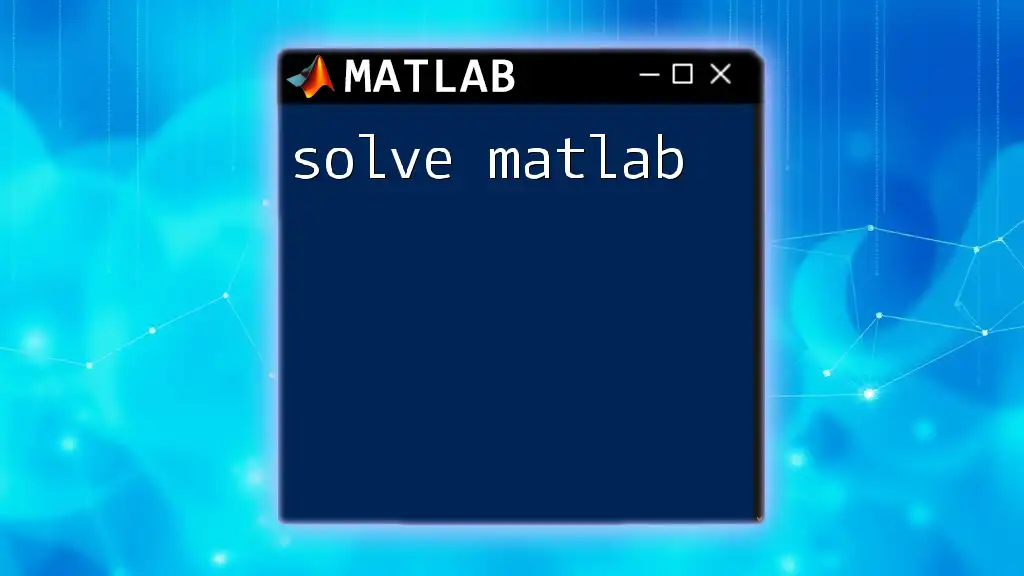
Programming in Octave
Control Structures
Control structures are fundamental in programming, allowing you to control the flow of your code. In Octave, you can use conditionals and loops:
- Conditionals: Use `if`, `else`, and `switch` to execute code conditionally. Example of an `if` statement:
if x > 10
disp('x is greater than 10');
else
disp('x is 10 or less');
end
- Loops: `for` and `while` loops allow you to iterate over ranges or perform actions repeatedly. An example utilizing a `for` loop to display numbers from 1 to 5:
for i = 1:5
disp(i);
end
Functions in Octave
Creating reusable code is essential for effective programming. In Octave, you can define functions to encapsulate specific behaviors. Here’s how to declare a simple function that squares an input:
function output = myFunc(x)
output = x^2;
end
This function can then be called by passing a numerical argument to it.
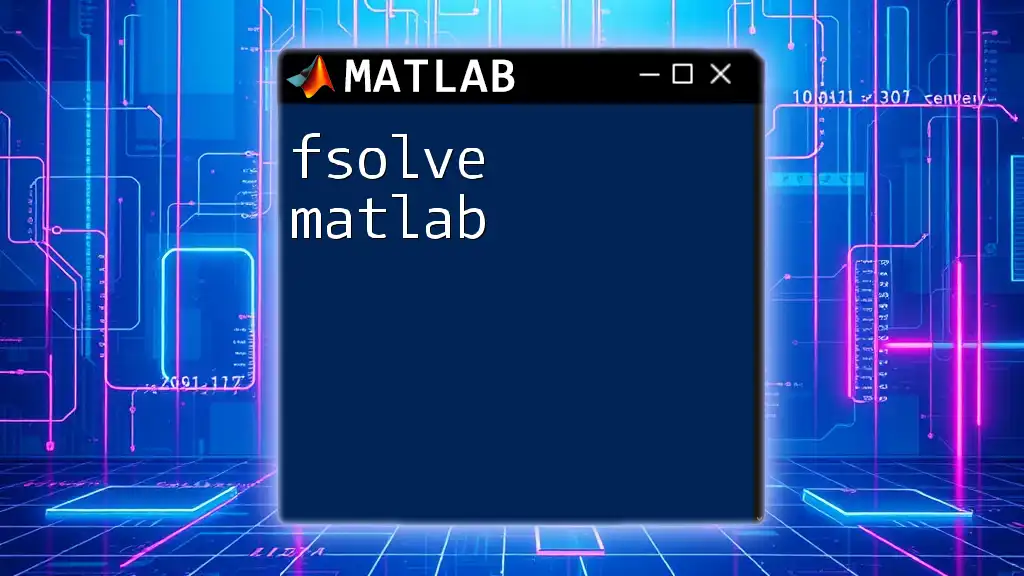
Plotting and Visualization
Graphing Basics
Visualizing data is crucial in data analysis and modeling. Octave provides powerful plotting functions. A simple plot can be created using:
x = 0:0.1:2*pi;
y = sin(x);
plot(x, y);
This command generates a basic sine wave chart.
Customizing Plots
To enhance your visualizations, you can customize plots by adding titles, labels, and legends. Here’s an example of a customized plot:
plot(x, y);
title('Sine Wave');
xlabel('Angle (radians)');
ylabel('Sine');
legend('sin(x)');
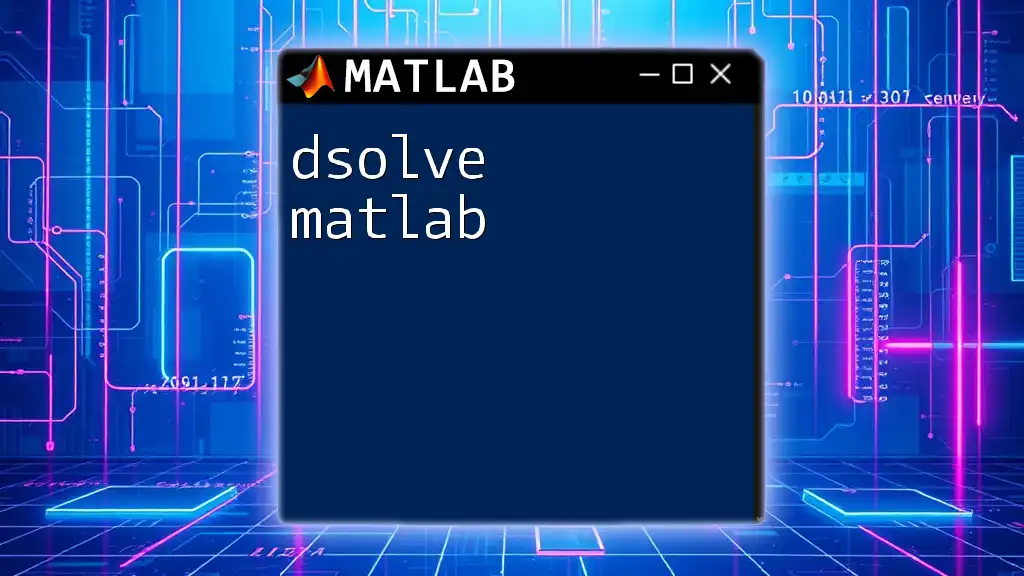
Advanced Features
Files and Input/Output
Loading and saving data is vital when working with larger datasets. Octave supports various formats, including CSV. You can read a CSV file with:
data = csvread('data.csv');
This command loads the data from 'data.csv' into a variable named `data`, allowing for further manipulations.
Packages and Extensions
One of Octave's strengths is its extensibility through packages. These packages can add new functions and enhance the capabilities of Octave. To install and use a package, you first need to ensure you have it available:
pkg install -forge image;
pkg load image;
This example installs and loads the image processing package from the Forge repository.
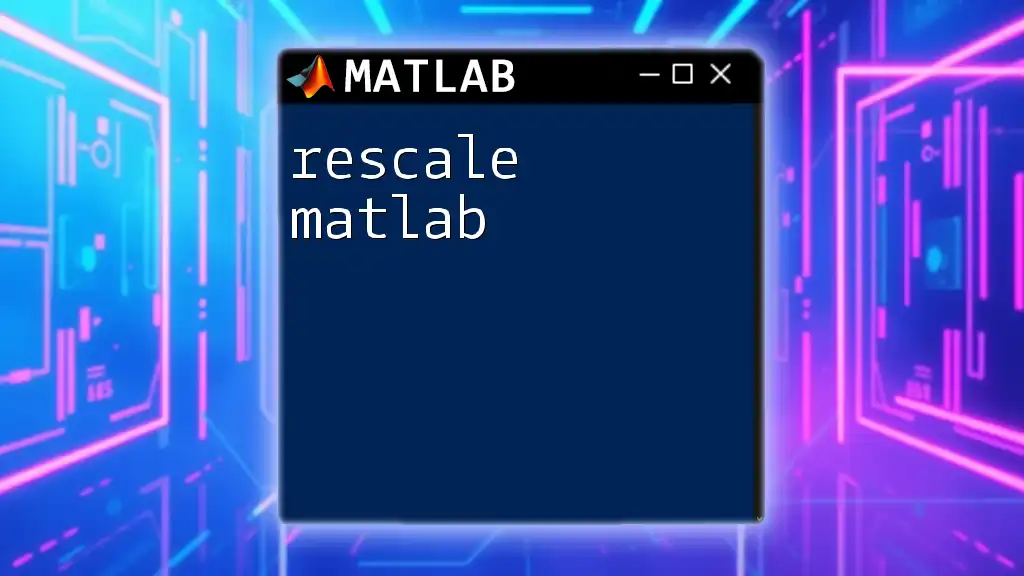
Compatibility with MATLAB
Running MATLAB Code in Octave
Due to its design, Octave can run a substantial number of MATLAB scripts. Most basic MATLAB scripts will execute without alteration in Octave. However, users should be aware of potential incompatibilities in specific toolbox functions or GUI components.
Using Octave in Parallel with MATLAB
Using Octave and MATLAB in tandem can leverage the strengths of both platforms. You might choose Octave for exploratory data analysis due to its free nature while utilizing MATLAB for specific advanced functionalities or proprietary toolboxes.
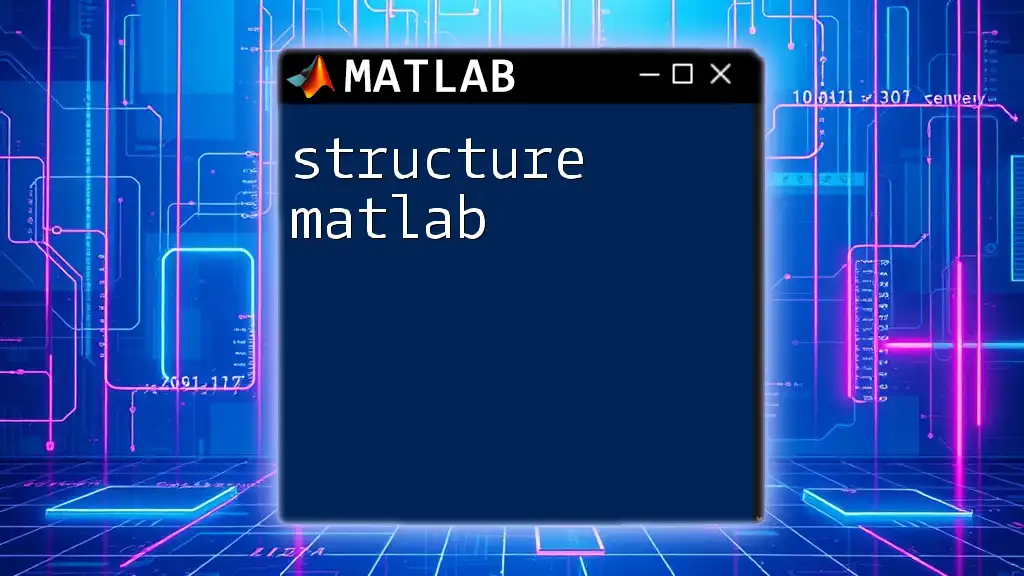
Educational Resources
Learning Materials
To maximize your understanding of Octave, it’s beneficial to tap into various learning resources. Online courses, tutorials, and textbooks focused on both Octave and MATLAB are excellent starting points for deeper learning.
Community and Support
Octave boasts a supportive community. Engage with forums, user groups, and official documentation to enhance your learning and solve any challenges you might encounter.
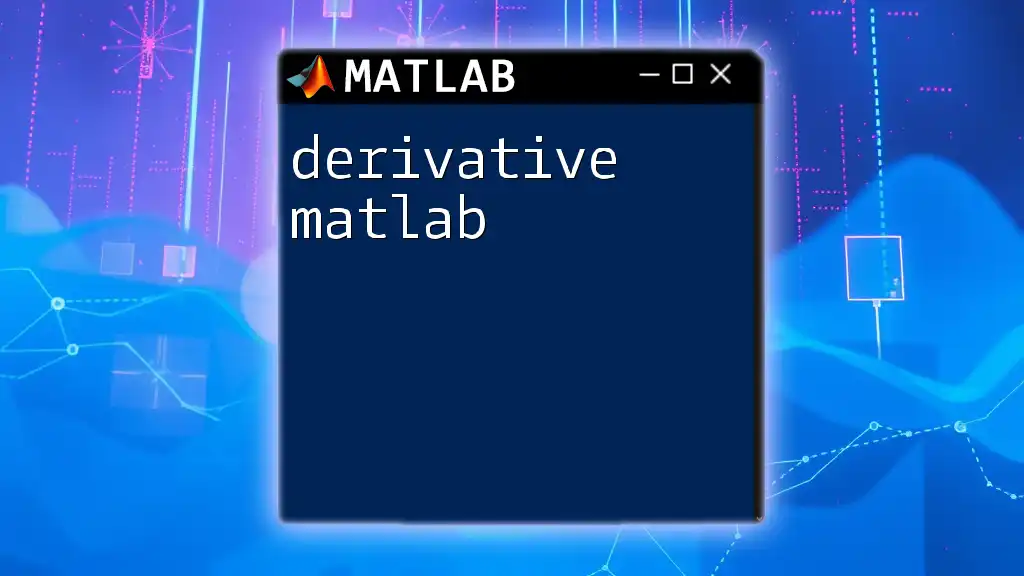
Conclusion
In conclusion, Octave offers a powerful, open-source alternative to MATLAB that retains many of its functionalities. Its ease of use and similar syntax make it a practical choice for anyone looking to perform numerical calculations without the financial overhead of proprietary software. We encourage you to explore Octave further and leverage its capabilities in your projects.
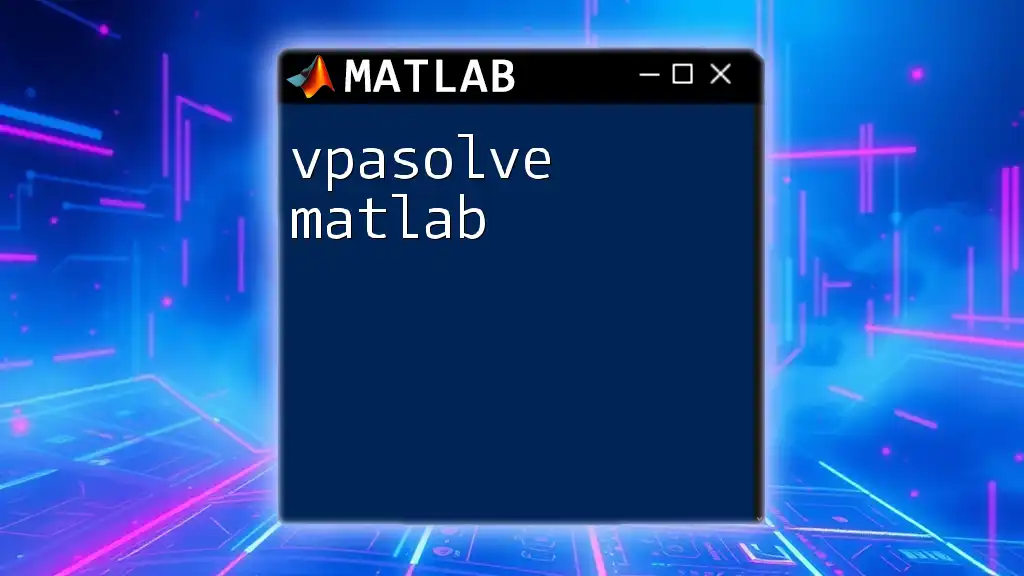
Further Reading
To broaden your knowledge and skills, be sure to explore additional tutorials and documentation, which can provide advanced insights and practical examples to master both Octave and MATLAB.