In MATLAB, the `gcf` command returns the handle of the current figure, allowing you to manipulate or query the properties of that figure programmatically.
figHandle = gcf; % Get the handle of the current figure
Understanding Figures in MATLAB
What is a Figure?
In MATLAB, a figure is a graphical window where plots and data visualizations are displayed. Figures play a crucial role in presenting graphical data clearly and effectively, allowing users to analyze trends, patterns, and relationships visually. Without figures, the data analyzed using MATLAB would be hard to interpret.
Creating a Figure
You can create a new figure window by using the `figure` command. This command initializes a new figure where plots can be rendered. For instance, you can execute the following commands to create a random plot:
figure; % Creates a new figure window
plot(rand(1,10)); % Plots random data
In this example, `figure` opens a new window, and `plot(rand(1,10))` generates a line plot of 10 random values.
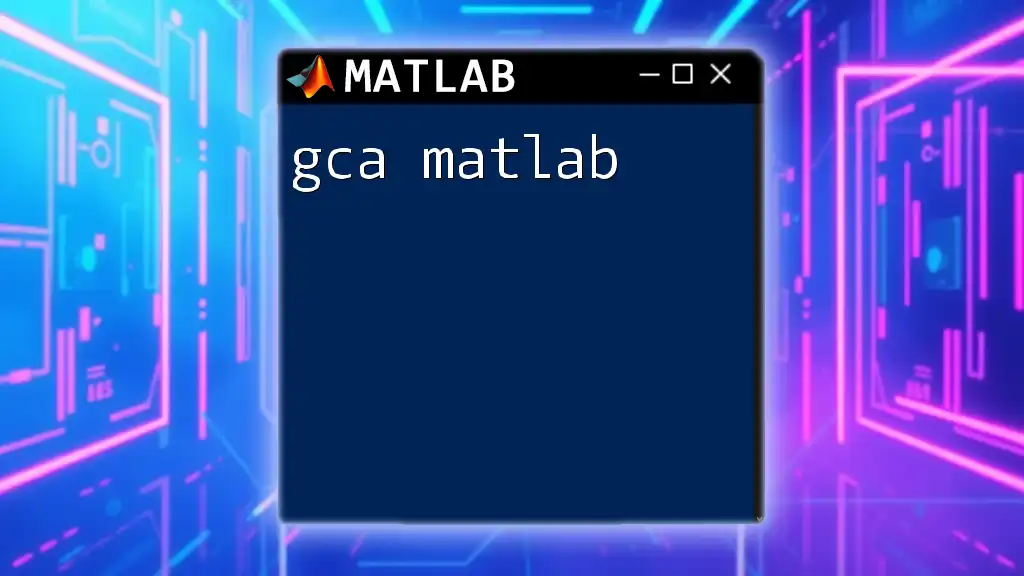
The gcf Function
What is gcf?
The command `gcf`, which stands for "get current figure," is an important MATLAB function that retrieves the handle of the currently active figure. Using `gcf` allows users to work with the figure that is currently being used without having to explicitly refer to its handle each time.
Syntax of gcf
The syntax for using `gcf` is straightforward. Simply call the function, and it returns a handle to the current figure:
fig = gcf; % Assigns the handle of the current figure to variable fig
Once you have the handle stored in a variable, you can manipulate or retrieve properties of the current figure.
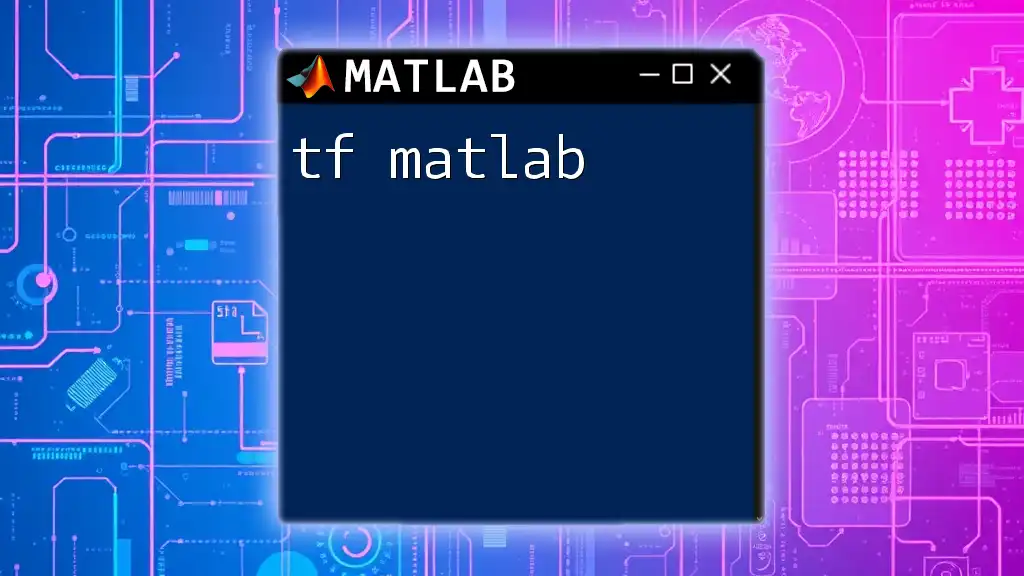
Practical Uses of gcf
Accessing Current Figure Properties
Using `gcf`, you can access various properties of the current figure. Some key properties include the figure's title, color, size, and visibility. For example, if you want to retrieve the title of the currently active figure, you can use:
figTitle = get(gcf, 'Name'); % Gets the title of the current figure
This command captures the name of the figure into the variable `figTitle`.
Modifying the Current Figure
Changing Figure Properties
With `gcf`, changing properties of the current figure is straightforward. For instance, if you wish to set the figure's background color to white, the command would be:
set(gcf, 'Color', 'w'); % Changes figure background color to white
Adding Titles and Labels
Adding titles and labels enhances the communicative power of figures. To add a title to the current figure, execute:
title('My Random Data Plot'); % Adds a title to the current figure
This code effectively labels the figure, making it informative for anyone viewing it.
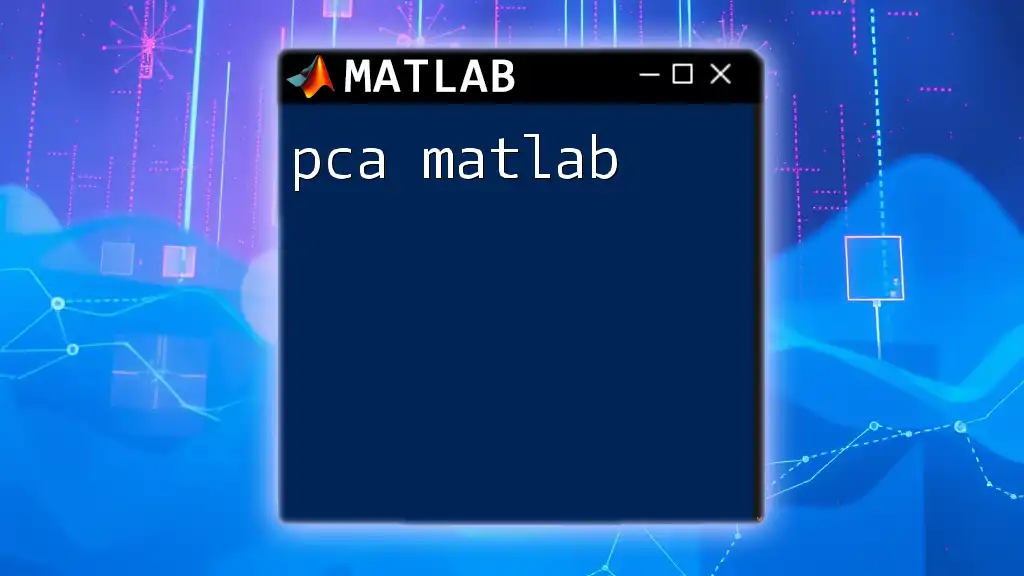
Common Mistakes with gcf
Not Using gcf After Creating a Plot
One common mistake is failing to use `gcf` properly after creating a plot. For instance, if you create a new figure but do not use `gcf`, you might lose the reference to the figure, leading to unexpected results. Here's an illustration of this:
figure; % Create new figure
plot(rand(1,10));
% Failing to call gcf afterward may lead to confusion when you want to modify this figure
Overriding Figures
Another crucial point to keep in mind is the risk of accidentally overriding the current figure. If you create a new figure without first retrieving the current one via `gcf`, you may end up losing access to the previous figure. Thus, it’s essential to manage figures carefully, especially when working with multiple figures simultaneously.
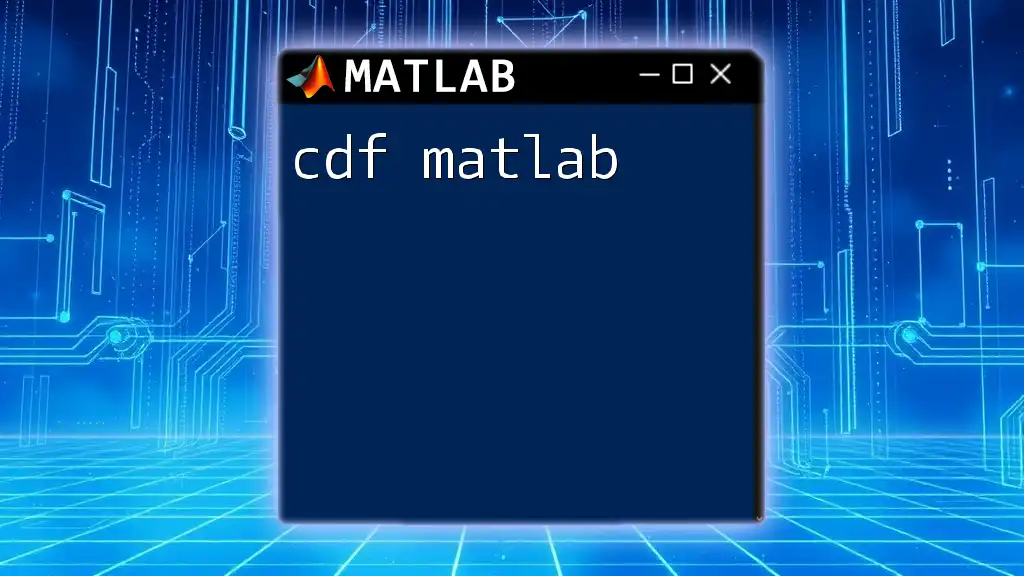
Best Practices
Always Check if a Figure Exists
Before modifying the current figure properties, it's wise to ensure that a figure exists. You can check this using the `ishandle` function along with `gcf` as follows:
if ishandle(gcf)
% Your code to modify the figure here
end
Using this conditional check prevents unexpected errors and ensures your code runs smoothly.
Naming Figures for Clarity
It's also a best practice to assign clear names to figures using `gcf`. Naming figures aids in identifying them later, especially when you are handling multiple figures. You can assign a name with this command:
set(gcf, 'Name', 'My Figure 1'); % Assign a name to the current figure
Doing so makes it easier to keep track of different plots throughout your analysis.
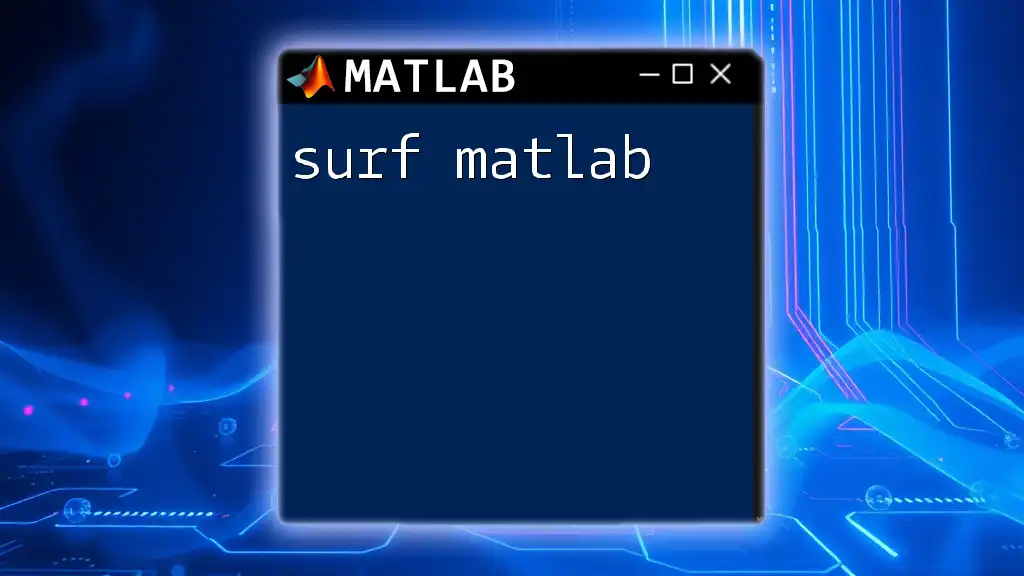
Conclusion
The `gcf` command in MATLAB is a simple yet powerful tool that greatly enhances your ability to manage and manipulate figures. Mastering the use of `gcf` can significantly improve your data visualization skills. As you experiment with `gcf` in your MATLAB scripts, you’ll discover its invaluable role in accessing and modifying figures efficiently.
Don’t hesitate to explore and play with this command to fully leverage its potential in your MATLAB projects!