The `rref` function in MATLAB computes the reduced row echelon form of a matrix, simplifying it for easier interpretation and solution of linear systems.
Here’s a simple code snippet demonstrating its usage:
A = [1 2 3; 4 5 6; 7 8 9];
R = rref(A);
Understanding Reduced Row Echelon Form
Definition and Characteristics
What is Reduced Row Echelon Form?
The Reduced Row Echelon Form (rref) is a form of a matrix that simplifies solving systems of linear equations. A matrix is in rref if it meets the following criteria:
- Leading Entries: Each leading entry (the first non-zero number from the left, called a pivot) in a non-zero row is 1.
- Zero Rows: Any rows consisting entirely of zeros are at the bottom of the matrix.
- Column Rule: Each leading 1 is the only non-zero entry in its column, which means all entries in the column above and below the leading 1 are zeros.
These characteristics make it easier to interpret the solutions to linear equations represented by the matrix.
Key Properties of rref
- Unique Representation: Every matrix has a unique rref, which simplifies computations and interpretations.
- Useful for Solving Linear Systems: rref helps determine whether a system of equations has a unique solution, no solution, or infinitely many solutions.
- Preservation of Equivalence: The rref of a matrix preserves the equivalence of linear systems, meaning the solutions remain unchanged.
Example of rref
To illustrate the concept, let's take a simple matrix and convert it to its rref step by step.
Consider the matrix:
A = [1 2 3; 4 5 6; 7 8 9];
By employing the rref command, you can obtain its reduced row echelon form easily:
R = rref(A);
disp(R);
This command results in:
1 0 -1
0 1 2
0 0 0
This shows that the original system can be simplified, revealing clear relationships between the variables.
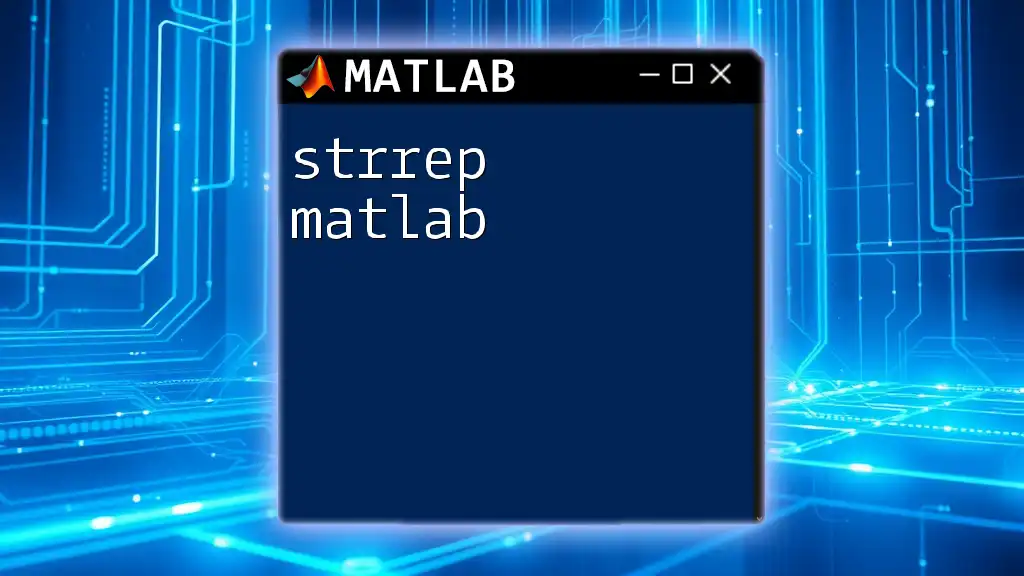
Using the rref Command in MATLAB
Syntax and Functionality
Basic Syntax
The general syntax for the `rref` command in MATLAB is straightforward:
R = rref(A)
Where A is your matrix of interest. The output R is the matrix in reduced row echelon form.
Output Explanation
When you run the command, MATLAB processes the matrix and returns the rref version, which is critical for interpreting solutions and understanding the underlying structure of the data or equations.
Advanced Usage of rref
Working with Augmented Matrices and Systems of Equations
When dealing with systems of linear equations, we often represent them in an augmented matrix. An augmented matrix combines the coefficients of the variables and the constants from the equations into one matrix.
For example, let's see how to solve the following system of equations:
- x + 2y + 3z = 1
- 4x + 5y + 6z = 0
- 7x + 8y + 9z = 1
We can represent this as an augmented matrix:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
b = [1; 0; 1];
Ab = [A, b]; % Creating the augmented matrix
R = rref(Ab);
disp(R);
The result will show you how to interpret the system's solutions based on the rref, providing insights into whether there are unique, infinite, or no solutions.
Handling Special Cases
Dealing with Singular Matrices
A singular matrix is one that does not have an inverse, often due to linear dependencies among the rows. In such cases, the rref can reveal important information.
Running the command on a singular matrix:
A = [1 2 3; 4 5 6; 7 8 9];
R = rref(A);
disp(R);
The output could highlight a row of zeros, indicating that the system has either no solution or infinitely many solutions based on the context of your equations.
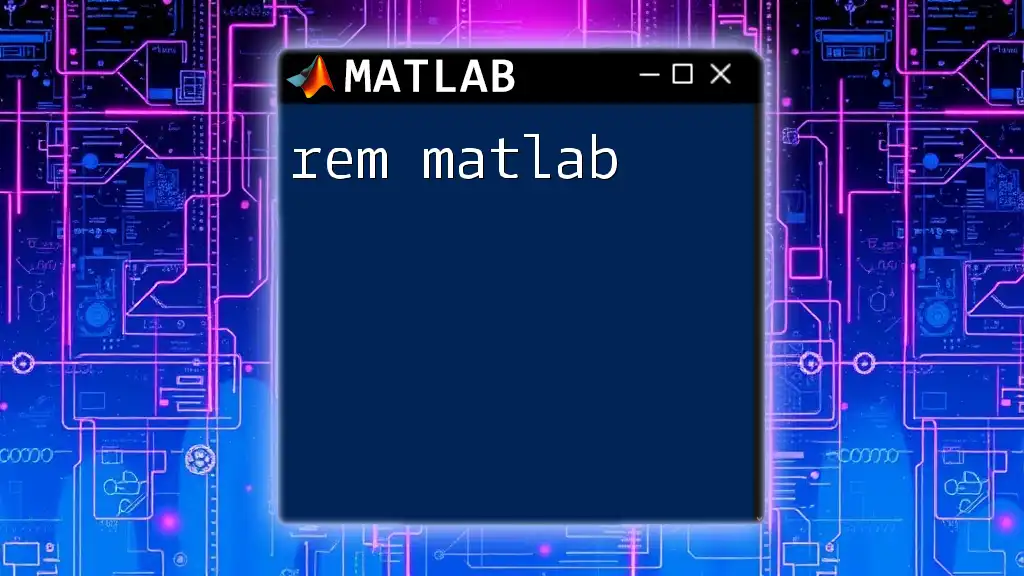
Practical Applications of rref
Solving Linear Systems
Real-world Application
The rref function is essential in numerous fields, including engineering, computer science, and economics. By transforming a matrix into rref, one can easily solve complex linear systems, making it a fundamental tool in operations research or resource allocation issues.
Data Analysis
rref in Data Reduction
In data science, rref can help reduce the complexity of data sets by identifying unique relationships among variables, especially in dimensionality reduction techniques. This can facilitate machine learning model training and improve the interpretability of findings.
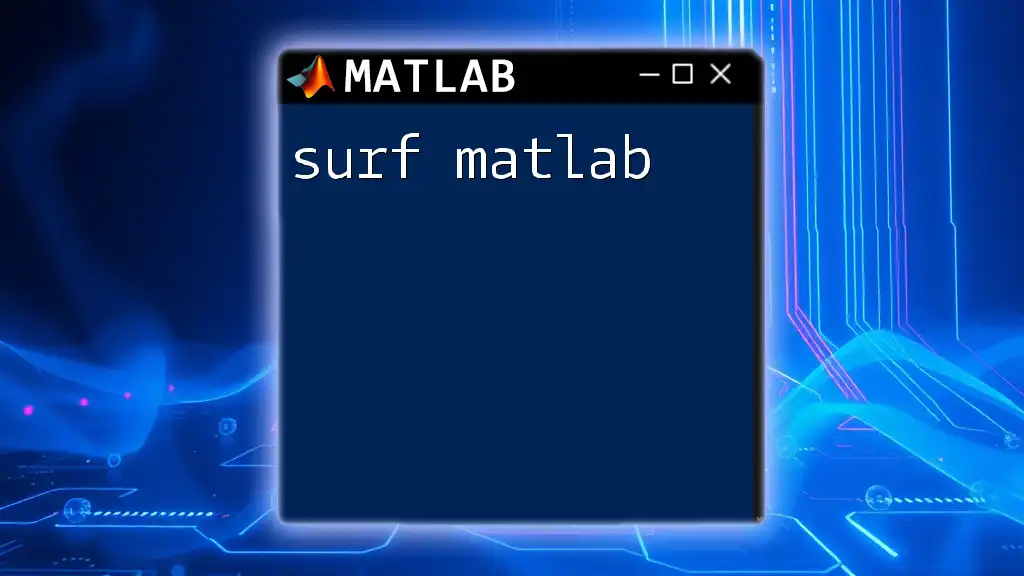
Common Errors and Troubleshooting
Understanding Errors in rref Function
Users might face errors when using the rref command, particularly with singular matrices or incorrectly formatted inputs. Always ensure that your matrix is well-defined and appropriately structured before applying the rref function.
Tips for Avoiding Errors
- Double-check matrix dimensions to ensure compatibility.
- Use the `rank` function to determine if a matrix is singular before calling rref.
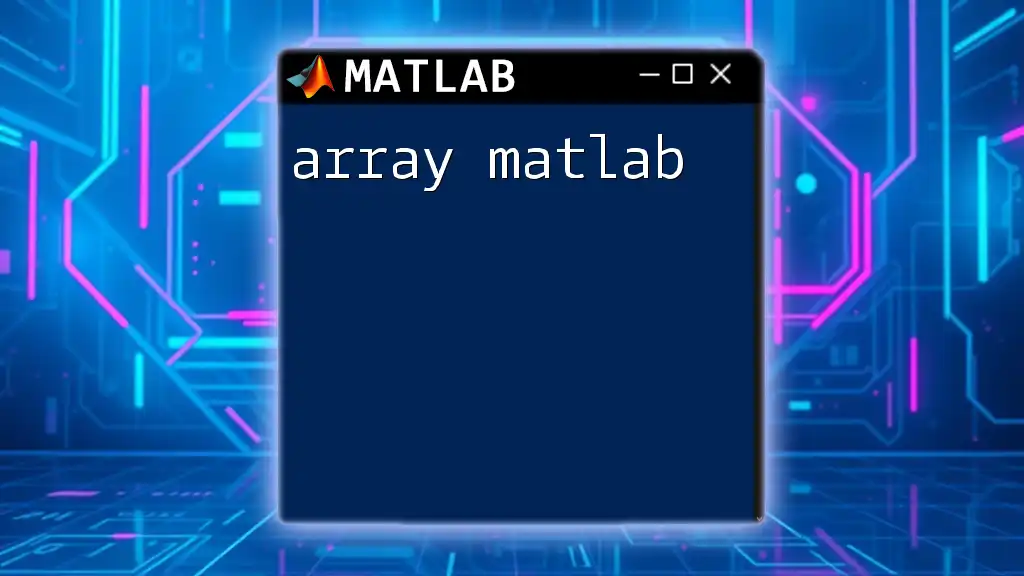
Conclusion
Mastering the rref function in MATLAB is a vital skill for anyone involved in linear algebra, data analysis, or related fields. Whether you're solving systems of equations or simplifying complex datasets, the rref command offers a powerful approach to understanding and manipulating matrix forms.
Encouraging Further Learning
For those eager to expand their knowledge, many resources and tutorials are available. Engaging in MATLAB classes or exploring online materials can deepen understanding and proficiency in utilizing commands like rref effectively.
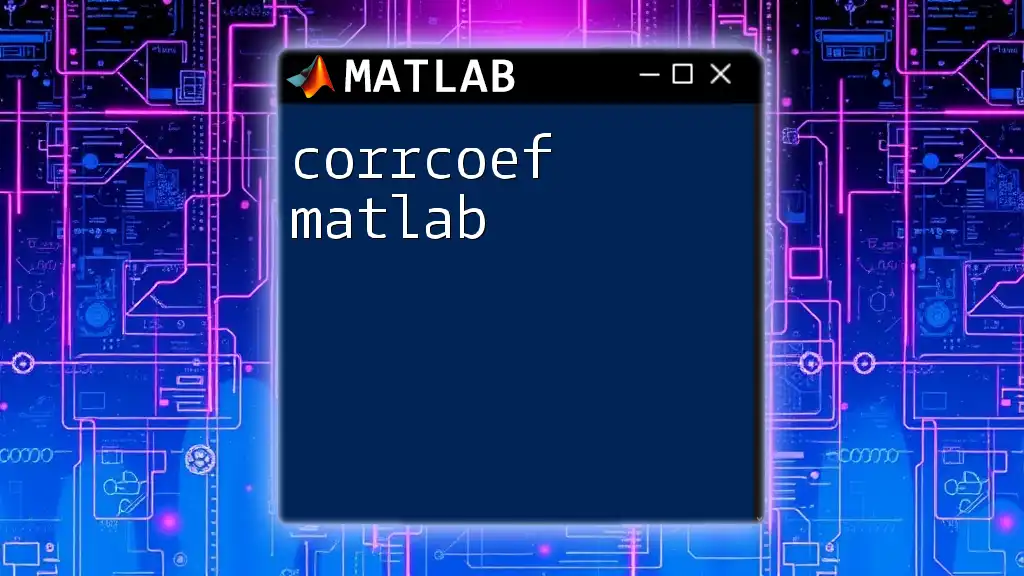
Additional Resources
For additional reading, consider reviewing the MATLAB documentation for rref, which provides comprehensive details and examples. Furthermore, several recommended books on linear algebra can significantly enhance your theoretical and practical understanding of the topic.
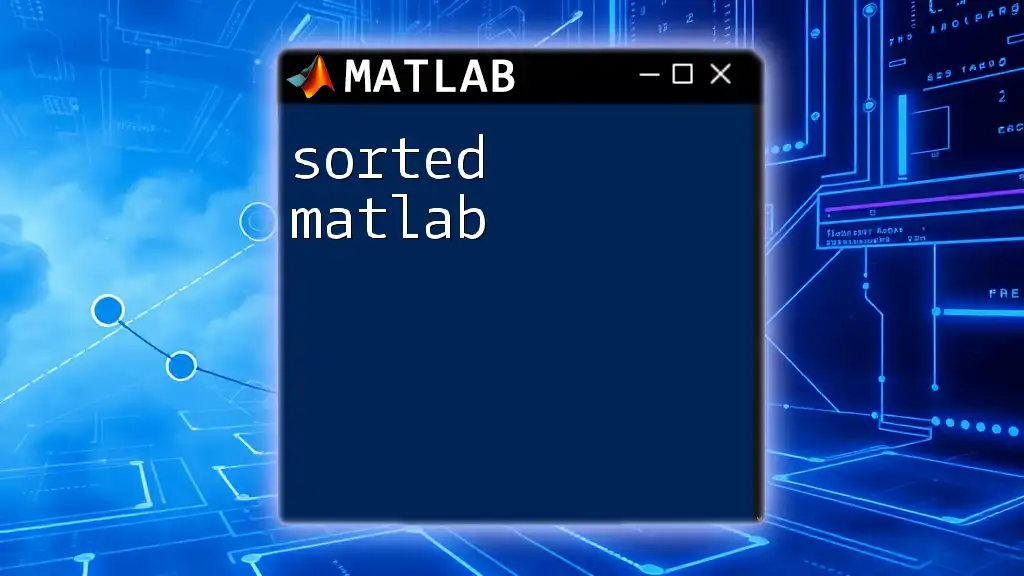
Call to Action
Join us for our upcoming MATLAB classes to elevate your skills and explore more about matrix functions, including rref and beyond!