The `eig` function in MATLAB computes the eigenvalues and eigenvectors of a square matrix, which is essential for understanding the properties of linear transformations.
Here’s a code snippet to demonstrate the usage of the `eig` function:
A = [4, 2; 1, 3]; % Define a 2x2 matrix
[eigenVectors, eigenValues] = eig(A); % Compute the eigenvalues and eigenvectors
Understanding Eigenvalues and Eigenvectors
Definition of Eigenvalues
In linear algebra, eigenvalues are scalars associated with a given linear transformation represented by a matrix. Mathematically, if \( A \) is a square matrix, then a scalar \( \lambda \) is considered an eigenvalue of \( A \) if there exists a non-zero vector \( v \) such that:
\[ A v = \lambda v \]
This equation essentially states that when the matrix \( A \) multiplies the vector \( v \), the result is simply the vector \( v \) scaled by the factor \( \lambda \). The significance of eigenvalues lies in their ability to indicate how a transformation affects the space spanned by the corresponding eigenvectors.
Definition of Eigenvectors
Eigenvectors are the non-zero vectors \( v \) that correspond to the eigenvalues of a matrix. Each eigenvector points in a direction that remains invariant under the transformation associated with the matrix. In essence, while the matrix might stretch or compress the vector, it does not change its direction when multiplied by the eigenvalue. This intrinsic property makes eigenvectors crucial in many applications, like stability analysis and dimensionality reduction.
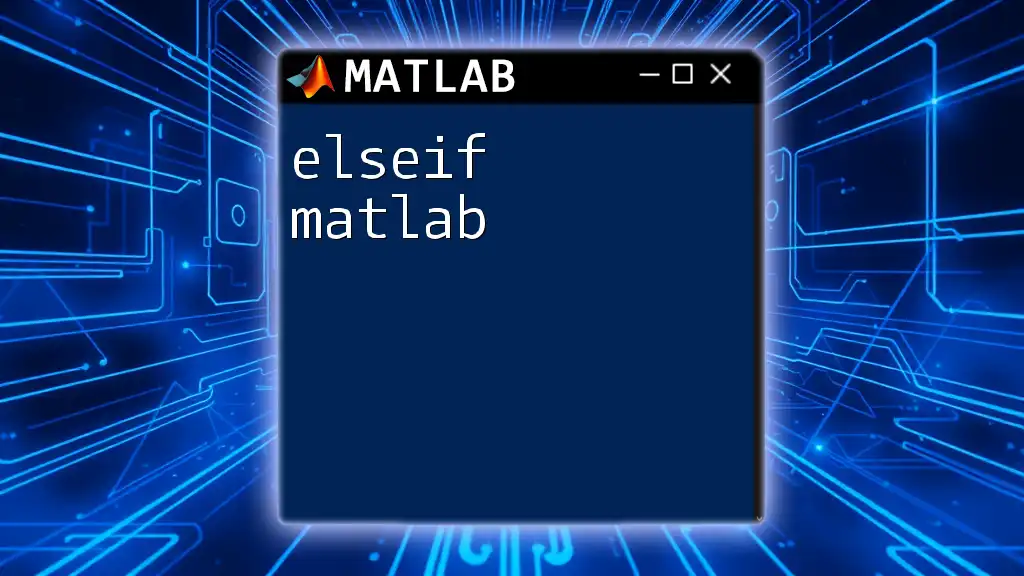
Overview of the `eig` Function in MATLAB
Purpose of the `eig` Function
The `eig` function in MATLAB is a powerful tool designed to compute the eigenvalues and eigenvectors of matrices. This function enables users to explore and understand the eigen properties of matrices, which is essential in various mathematical, engineering, and scientific applications.
Syntax of the `eig` Function
The basic syntax for using the `eig` function is as follows:
D = eig(A)
In this case, `A` is the input matrix, and `D` will return the eigenvalues of matrix `A`.
For obtaining both eigenvalues and eigenvectors, you can use:
[V, D] = eig(A)
Here, `V` contains the eigenvectors, while `D` is a diagonal matrix with the eigenvalues on its diagonal.
Furthermore, you can solve the generalized eigenvalue problem by using:
[V, D] = eig(A, B)
Where `A` and `B` are square matrices, and this form allows for greater flexibility in many applications.
Input Parameters Explained
The primary input is the square matrix `A`, which must be of dimensions \( n \times n \). In cases where matrix `B` is provided, it typically represents a second matrix in scenarios like performance evaluation or stability thresholds, which can modify the eigenvalue calculations.
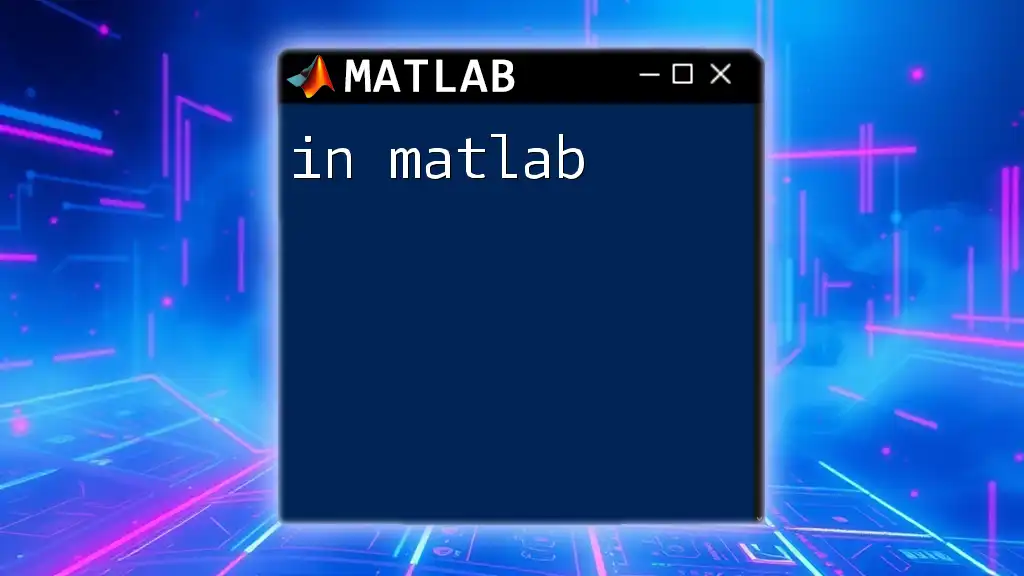
Using the `eig` Function
Example: Calculating Eigenvalues
To illustrate the calculation of eigenvalues using MATLAB, consider the following code snippet:
A = [4 2; 1 3];
eigenvalues = eig(A);
In this example, `A` is a \( 2 \times 2 \) matrix. When we execute this code, MATLAB returns the eigenvalues of matrix `A`, allowing us to verify and analyze its properties. The output will provide insights into how the transformation represented by `A` affects the space it operates on.
Example: Calculating Eigenvectors
Now, let's calculate both the eigenvalues and eigenvectors of matrix `A`:
[V, D] = eig(A);
Here, `V` holds the eigenvectors, while `D` contains the corresponding eigenvalues arranged in a diagonal format. Each column of `V` is an eigenvector, and the corresponding diagonal entry in `D` indicates the eigenvalue associated with that vector.
By examining the matrix `V`, we gain valuable insight into the directions in which the transformations represented by `A` exhibit specific behaviors (e.g., stretching or flipping).
Example: Eigenvalues of a Complex Matrix
Exploring eigenvalues in the context of complex matrices can reveal even more intricate behaviors. For instance, consider the matrix:
A = [1 2+1i; 2-1i 3];
eigenvalues = eig(A);
The output will yield complex eigenvalues, demonstrating the versatility of the `eig` function in handling non-real numbers. Understanding these complexities can be significant when delving into fields where complex numbers arise, such as control theory or signal processing.
Example: Solving a System of Linear Equations Using Eigenvalues
Eigenvalues and eigenvectors can also serve as powerful tools in solving systems of linear equations. For example:
A = [3 1; 1 2];
b = [9; 8];
x = V * (D \ (V' * b));
In this snippet, we leverage the eigenvalues and eigenvectors to solve the system represented by the matrix `A`. The formulation illustrates how `eig` can facilitate deeper insights into linear systems, allowing for efficient solutions of potentially multivariate problems.
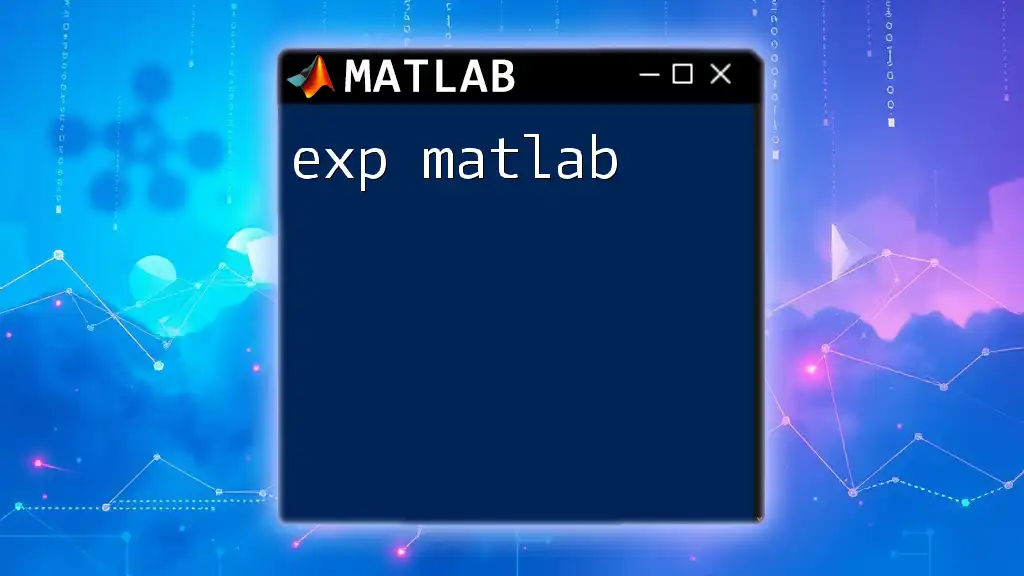
Practical Applications of Eigenvalues and Eigenvectors
Engineering Applications
In engineering, the concepts of eigenvalues and eigenvectors are vital for analyzing structures and systems. For example, in vibrations analysis, engineers use eigenvalues to determine the natural frequencies of systems, crucial for ensuring stability and safety in constructions.
Finance Applications
In finance, eigenvalues play a crucial role in risk assessment and portfolio optimization. By analyzing covariance matrices and finding eigenvalues, financial analysts can identify the underlying factors affecting the movement of asset prices, aiding in maximizing returns while minimizing risk.
Physics Applications
In physics, especially in quantum mechanics, eigenvalues and eigenvectors are essential for understanding system behavior. Operators are often expressed in matrix form, and the eigenvalues represent the observable quantities, such as energy levels, offering insights into the physical world's structure and behaviors.
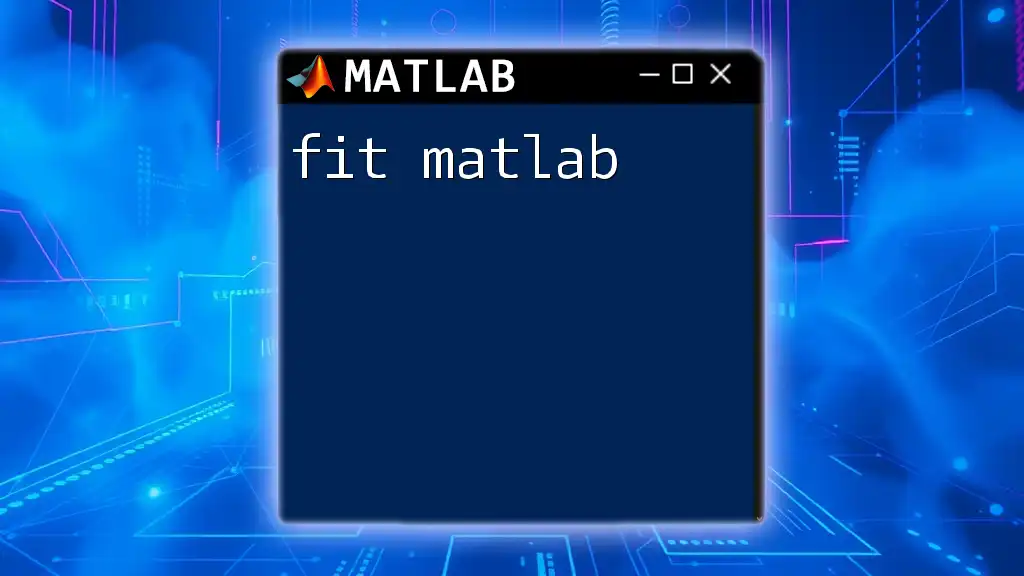
Common Errors and Troubleshooting
Common Mistakes While Using `eig`
A prevalent error among users is attempting to apply the `eig` function to non-square matrices. The `eig` function is strictly defined for square matrices, and providing a non-square matrix will lead to errors or unexpected results. Ensure that the dimensions of your matrix align correctly prior to computation.
Tips for Troubleshooting
To validate your results from the `eig` function, you can check the properties of the eigenvalues and eigenvectors. For example, an eigenvalue \( \lambda \) paired with its eigenvector \( v \) should satisfy the equation \( A v = \lambda v \). Furthermore, checking for matrix properties such as symmetry or definiteness can sometimes clarify unexpected outputs and guide the analysis process.
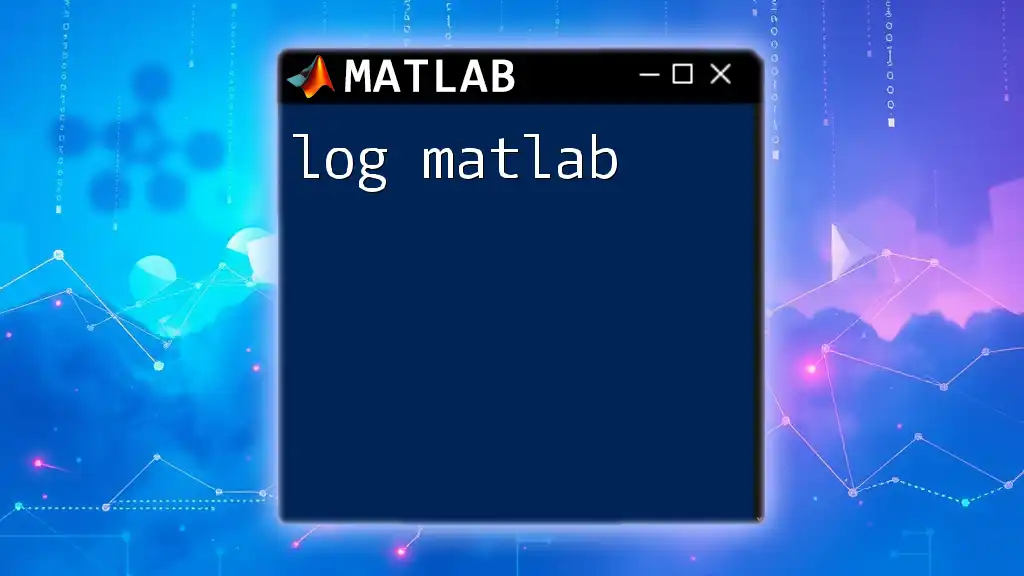
Conclusion
The `eig` function in MATLAB stands as a powerful utility in the realm of linear algebra, enabling users to explore eigenvalues and eigenvectors that reveal insights into matrix behaviors and transformations. This fundamental understanding proves critical across various domains, from engineering to finance and physics. By delving deeper into the workings of eigenvalues and leveraging the capabilities of `eig`, practitioners can unlock advanced analytical techniques and foster greater expertise in their respective fields.
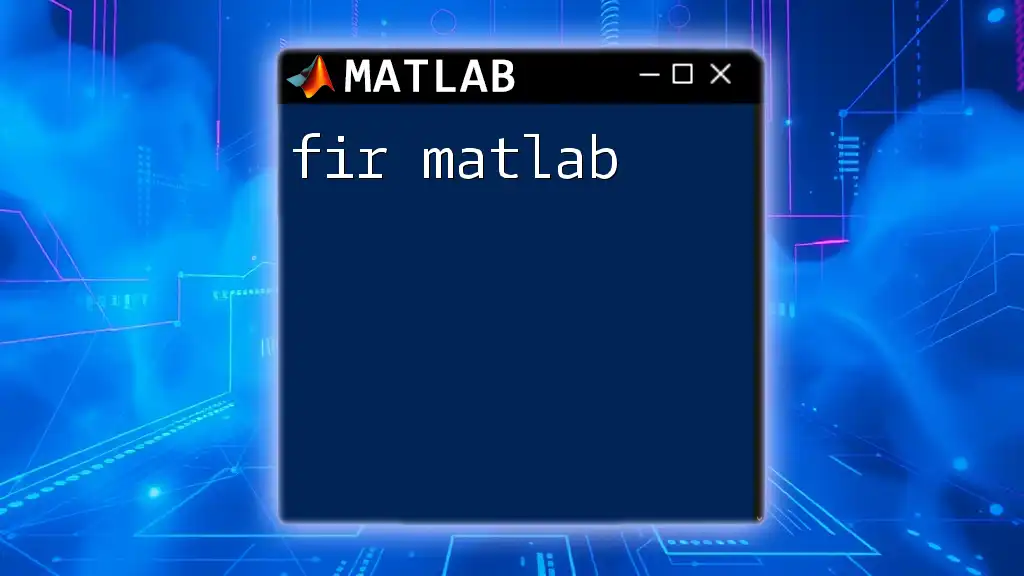
Additional Resources
To further enhance your understanding of the `eig` function and its applications, consider visiting the official MATLAB documentation. Engaging with community forums or participating in workshops can also provide invaluable hands-on experience. For those looking to deepen their knowledge, there are various reading materials available that delve into the applications of eigenvalues and eigenvectors across different disciplines.