To define a matrix in MATLAB, you can use square brackets to enclose the elements, separating rows with semicolons and columns with spaces or commas. Here’s a simple example:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
Understanding Matrices
What is a Matrix?
A matrix is a rectangular array of numbers, symbols, or expressions, arranged in rows and columns. In MATLAB, matrices are fundamental as they serve as the primary data type. Understanding matrix dimensions—expressed in terms of rows and columns—is key to effective matrix manipulation.
For example, consider a simple 2x3 matrix:
A = [1 2 3; 4 5 6]
In this case, matrix A has 2 rows and 3 columns. The way you define and manipulate matrices becomes crucial for performing mathematical operations and data analysis in MATLAB.
Types of Matrices
Matrices can take various forms, classified based on their dimensions and characteristics:
-
Row Matrix: A matrix with a single row.
-
Column Matrix: A matrix with a single column.
-
Square Matrix: A matrix with an equal number of rows and columns, such as:
B = [1 2; 3 4]; % A 2x2 square matrix
-
Zero Matrix: A matrix where all elements are zero, e.g.,
Z = zeros(2, 3); % A 2x3 matrix of zeros
-
Identity Matrix: A square matrix containing ones on the main diagonal and zeros elsewhere:
I = eye(3); % A 3x3 identity matrix
Understanding these matrix types allows you to apply appropriate mathematical operations and analyses tailored to your data.
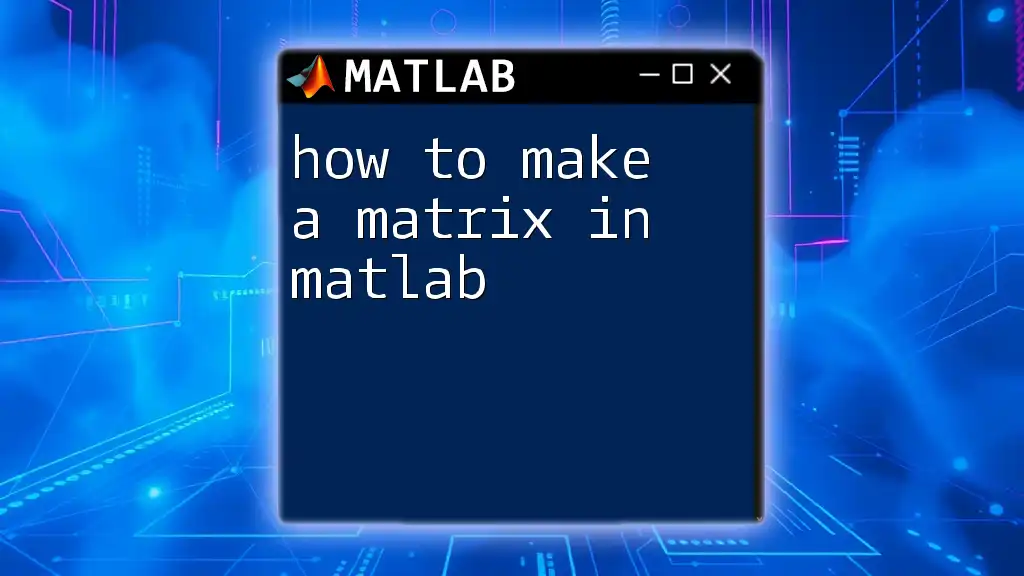
Defining a Matrix in MATLAB
Basic Matrix Creation
Defining a matrix in MATLAB is straightforward and can be done using square brackets. Elements within the matrix are separated by spaces, while rows are separated by semicolons.
For instance, to define a simple matrix, you can use:
A = [1 2 3; 4 5 6]
This command creates a 2x3 matrix named A. Remember, the use of semicolons to denote new rows is essential for accurately defining matrices in MATLAB.
Creating Row and Column Vectors
MATLAB allows users to create both row and column vectors, which are useful for representing one-dimensional data.
- A row vector can be defined as follows:
rowVec = [1 2 3 4 5];
- A column vector is defined by using semicolons to separate the elements:
colVec = [1; 2; 3; 4; 5];
Both types of vectors are merely specific instances of matrices and can be utilized in various mathematical contexts.
Using MATLAB Functions to Create Matrices
Several built-in MATLAB functions offer convenient ways to create matrices quickly and efficiently:
Using the `zeros` Function
To create a matrix filled with zeros, you can utilize the `zeros` function. This is particularly useful when initializing matrices for large computations.
Z = zeros(3, 4); % Creates a 3x4 zero matrix
This command generates a matrix with 3 rows and 4 columns, all initialized to zero.
Using the `ones` Function
Similarly, the `ones` function creates a matrix where all elements are set to one. This can be beneficial for algorithms that require a starting point of all ones.
O = ones(2, 5); % Creates a 2x5 matrix of ones
Using the `eye` Function
The `eye` function creates an identity matrix, which is particularly useful in linear algebra.
I = eye(4); % Creates a 4x4 identity matrix
This results in a 4x4 matrix where all diagonal values are 1, and all non-diagonal values are 0.
Using the `rand` Function
To create a matrix filled with random values, you can use the `rand` function. This can be particularly useful in simulations or scenarios where random sampling is necessary.
R = rand(3, 2); % Creates a 3x2 matrix of random values
This command produces a 3x2 matrix with random numbers drawn from a uniform distribution over the interval (0,1).
Creating Matrices with Specific Patterns
Using Colons to Generate Sequences
MATLAB offers a powerful feature with the colon operator to quickly generate sequences. This can help create row vectors with regularly spaced elements.
seqVec = 1:2:10; % Generates [1 3 5 7 9]
With this command, you generate a sequence starting from 1, increasing by 2 each time, until it reaches 10.
Using `meshgrid` to Create a Grid of Coordinates
For applications involving 2D plots or spatial data, the `meshgrid` function can be extremely useful. It creates matrices from coordinate vectors.
[X, Y] = meshgrid(1:3, 1:4);
The resulting matrices X and Y will represent a grid of coordinates which can be used for various analytical tasks, such as 3D plotting.
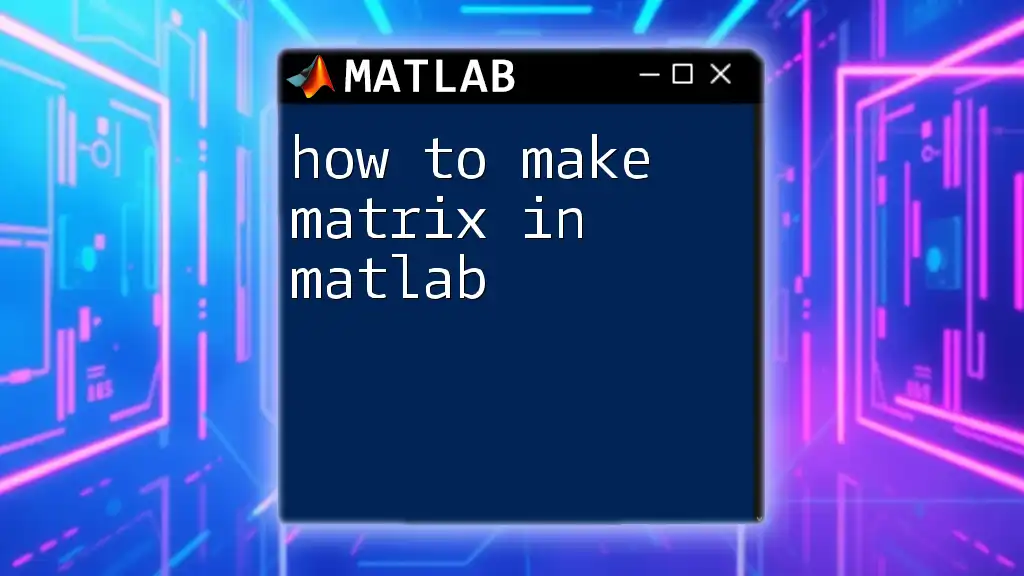
Manipulating Matrices
Accessing Elements
Accessing specific elements in a matrix is a fundamental operation. You can index into the matrix using parentheses and specifying the row and column number.
For example, accessing an element from matrix A defined earlier:
element = A(2, 3); % Accesses the element in the 2nd row and 3rd column
This command retrieves the value located at that position, which in this example would be 6.
Modifying Matrices
Changing individual elements within a matrix is also straightforward. By specifying the index of the element you wish to change, you can directly update its value:
A(1, 2) = 10; % Changes the element in the 1st row, 2nd column to 10
Now, the modified matrix A reflects this change, demonstrating how dynamic matrix handling can be in MATLAB.
Resizing Matrices
If you need to change the dimensions of a matrix after it has been defined, the `reshape` function is your tool for the job. This enables you to alter the size while preserving the data.
B = reshape(A, 3, 2); % Reshapes matrix A to a matrix with 3 rows and 2 columns
Keep in mind that the total number of elements must remain unchanged. This feature is particularly valuable for data analysis and transformations.
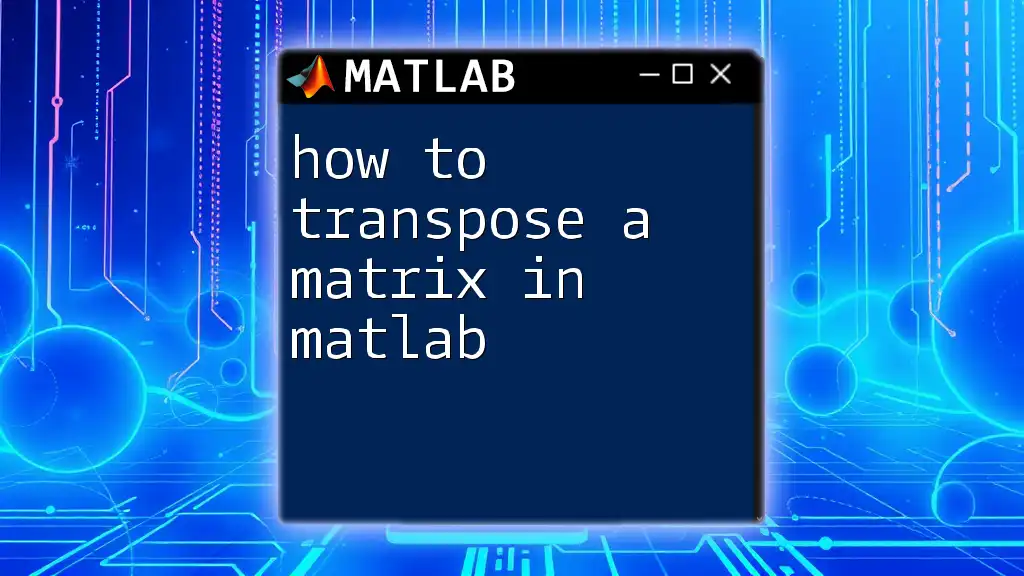
Conclusion
In summary, defining a matrix in MATLAB is a fundamental skill essential for engaging with mathematical computations and data analysis. By mastering the basic definitions, matrix types, and manipulation techniques, you can leverage this powerful tool in your work. Always remember to practice the examples provided to solidify your understanding and improve your proficiency in using MATLAB for matrix operations.
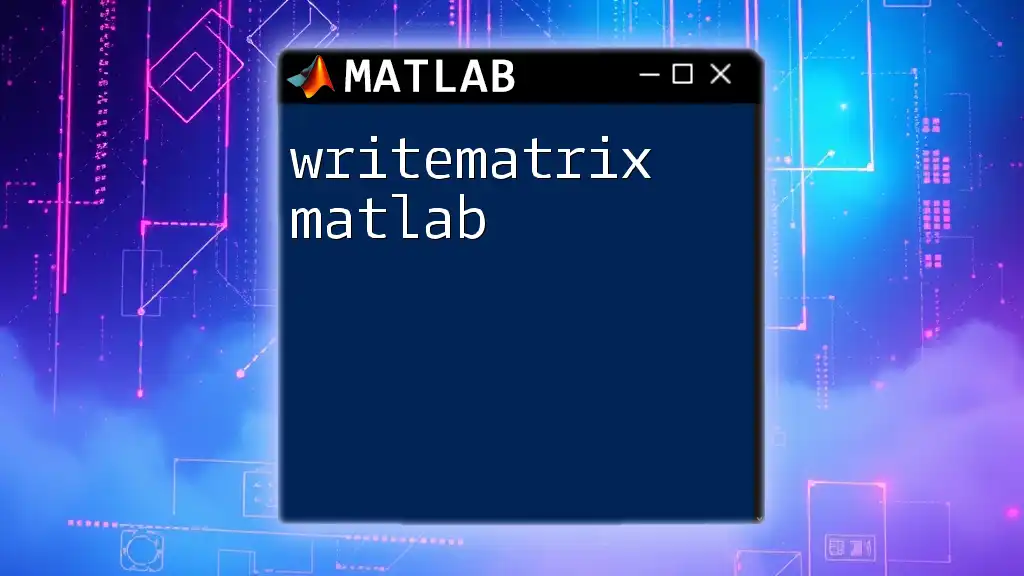
Additional Resources
For further exploration beyond the basics, consider checking the official MATLAB documentation, engaging in online tutorials, or participating in community forums dedicated to MATLAB. These resources can provide deeper insights and enhance your skills in matrix handling and other advanced functionalities in MATLAB.
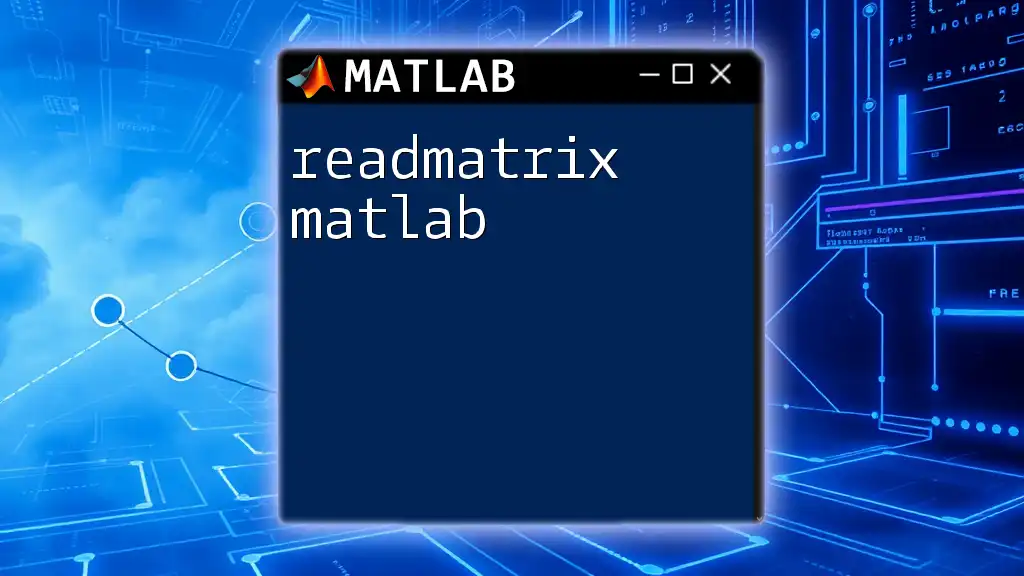
FAQs
How can I define a matrix with random integer values?
You can create a matrix with random integer values by using a combination of the `randi` function. For example:
randomMatrix = randi(10, 3, 3); % Creates a 3x3 matrix with random integers from 1 to 10
What are the differences between row vectors and column vectors?
A row vector is a matrix with a single row, whereas a column vector is a matrix with a single column. Row vectors are defined with spaces, while column vectors use semicolons for separation.
How to convert a matrix to a vector?
To convert a matrix into a vector, you can use the `(:)` operator, which stacks all elements into a single column vector. For instance:
columnVec = A(:); % Converts matrix A into a single column vector
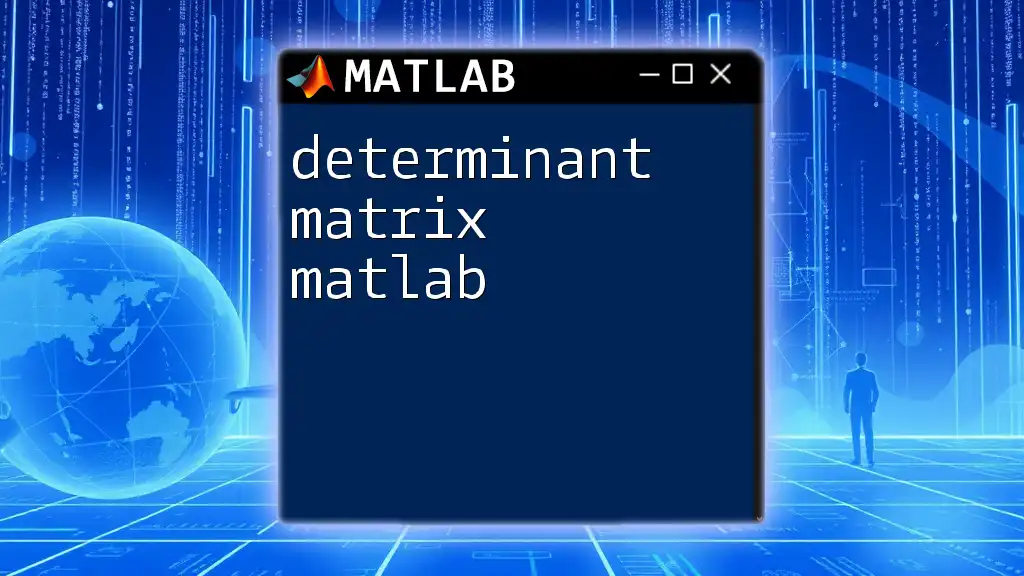
Final Thoughts
The versatility and power of matrix operations in MATLAB open doors to various applications across multiple domains. By becoming adept at defining matrices, you'll enhance your analytical capabilities and better equip yourself for the challenges of data analysis and mathematical modeling in your field.