To label axes in MATLAB, use the `xlabel` and `ylabel` functions to set the labels for the x-axis and y-axis, respectively.
Here's a code snippet to demonstrate:
% Example of labeling axes in MATLAB
x = 0:0.1:10;
y = sin(x);
plot(x, y);
xlabel('X Axis Label'); % Label for x-axis
ylabel('Y Axis Label'); % Label for y-axis
title('Sine Wave Example'); % Title of the plot
Understanding Axes in MATLAB
What Are Axes?
Axes are a fundamental part of any graph or chart created in MATLAB. They serve as the framework for visualizing data, providing reference points against which data can be understood. Axes are crucial for interpreting the values represented in plots and ensuring clarity in communication of insights.
Types of Axes in MATLAB
MATLAB offers various types of axes depending on the representation needed for your data:
- Cartesian Axes: The most common type, used for standard 2D and 3D plots.
- Polar Axes: Used for graphs that require circular representation, such as polar plots.
- Geographic Axes: Useful for displaying data on maps and geographical representations.
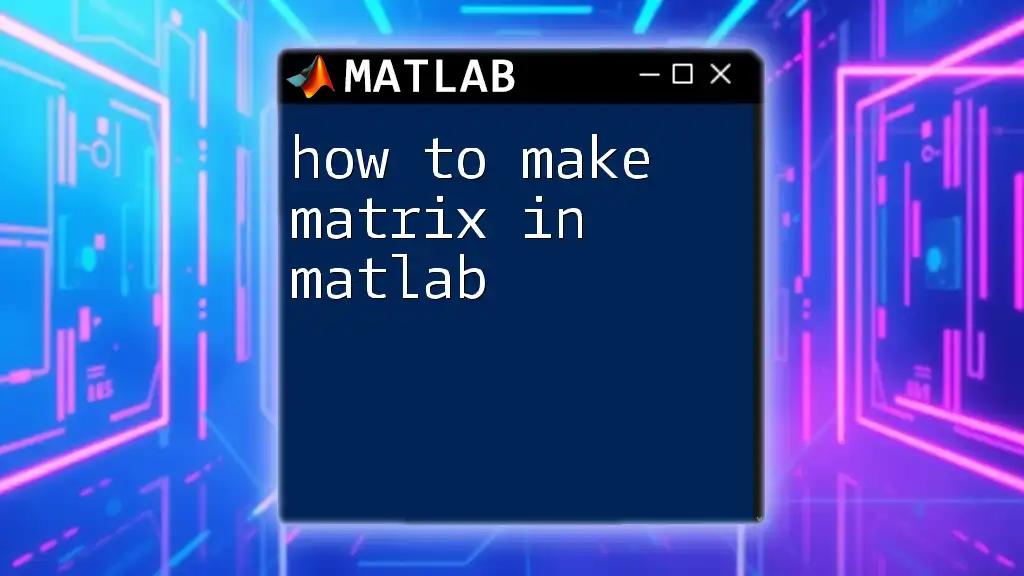
Getting Started with Axis Labeling
Why Use Axis Labels?
Using axis labels is essential for making your graphs self-explanatory. Labels provide context, indicating what each axis represents and helping viewers grasp the story behind the data at a glance. Proper labeling enhances the interpretability of graphs and contributes to more effective data analysis.
Basic Syntax for Labeling Axes
In MATLAB, you can label the x-axis, y-axis, and z-axis using specific functions. The syntax for each is straightforward:
- `xlabel('Your X-Axis Label')`
- `ylabel('Your Y-Axis Label')`
- `zlabel('Your Z-Axis Label')`
This usage is critical, as it ensures that anyone viewing the graph understands the dimensions of the data they're observing.
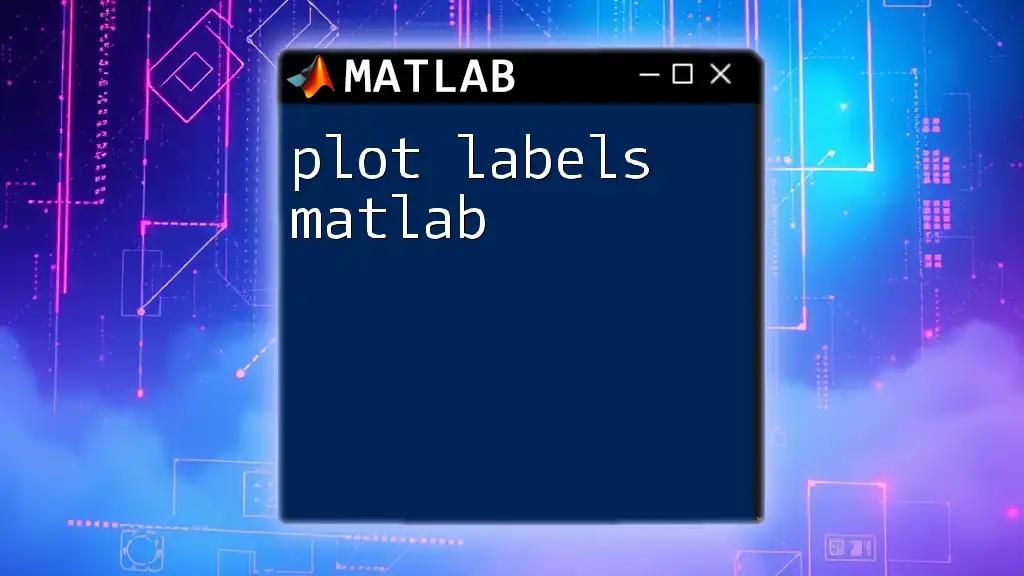
Labeling the X-Axis
Using `xlabel` Function
To label the x-axis, you simply use the `xlabel` function. Here’s a basic implementation:
xlabel('Your X-Axis Label')
Example with a Simple Plot
Let’s look at an example with a random dataset:
x = 1:10;
y = rand(1, 10);
plot(x, y);
xlabel('Sample X-Axis Label');
In this example, `Sample X-Axis Label` identifies the values on the x-axis for your data points.
Customizing the X-Axis Label
You may want to customize the appearance of your axis label for better visibility or aesthetics. This can include adjustments to font size, font weight, and color:
xlabel('Sample X-Axis Label', 'FontSize', 12, 'FontWeight', 'bold', 'Color', 'red');
By modifying these parameters, you can control how the label appears, making it more appealing and easier to read.
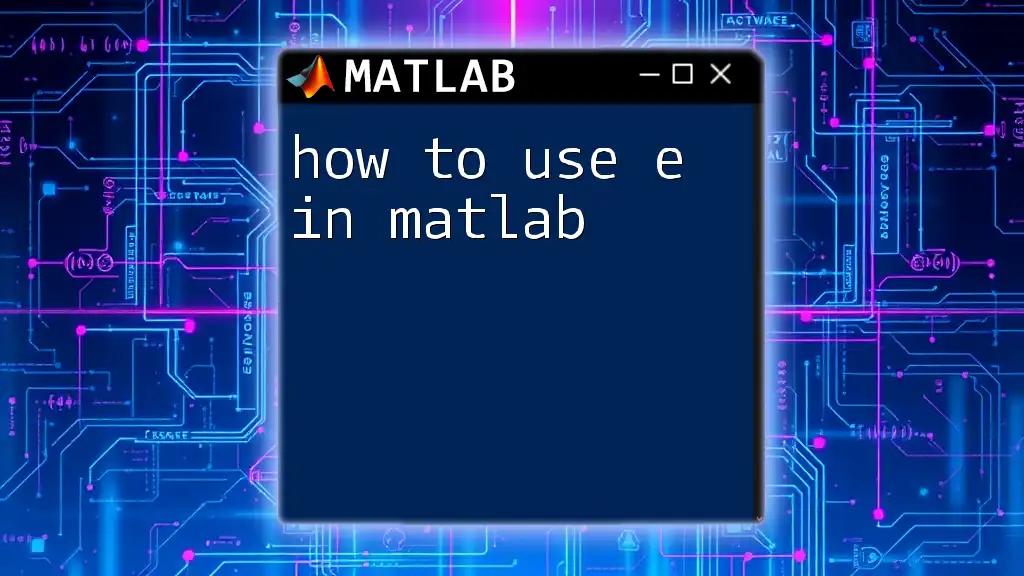
Labeling the Y-Axis
Using `ylabel` Function
Similar to labeling the x-axis, you use the `ylabel` function for the y-axis:
ylabel('Your Y-Axis Label')
Example with a Simple Plot
Continuing with the previous example, let’s add a y-axis label:
plot(x, y);
ylabel('Sample Y-Axis Label');
This clearly denotes what the y-axis represents in relation to the plotted data.
Customizing the Y-Axis Label
Customizing labels on the y-axis follows the same principles as the x-axis:
ylabel('Sample Y-Axis Label', 'FontSize', 12, 'FontWeight', 'bold', 'Color', 'blue');
Adjusting these parameters not only improves appearance but can also align with the overall color scheme of your visualization.
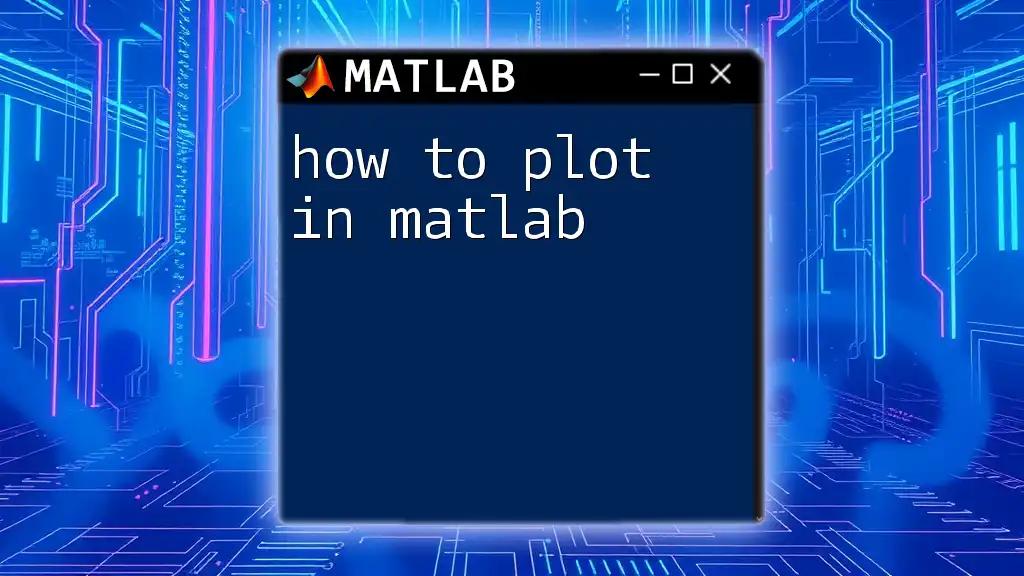
Labeling the Z-Axis
Using `zlabel` Function
For 3D plots, the z-axis requires its own label, accomplished through the `zlabel` function. Here’s the basic format:
zlabel('Your Z-Axis Label')
Example with a 3D Plot
Here’s how you can implement this in a 3D plot using the `surf` function:
[X,Y,Z] = peaks;
surf(X, Y, Z);
zlabel('Sample Z-Axis Label');
This example showcases how to label the z-axis in a three-dimensional space, adding clarity to the analysis.
Customizing the Z-Axis Label
Customization options for the z-axis label are analogous to those for the y and x axes:
zlabel('Sample Z-Axis Label', 'FontSize', 12, 'FontWeight', 'bold', 'Color', 'green');
This consistency allows for a coherent presentation across different axis labels.
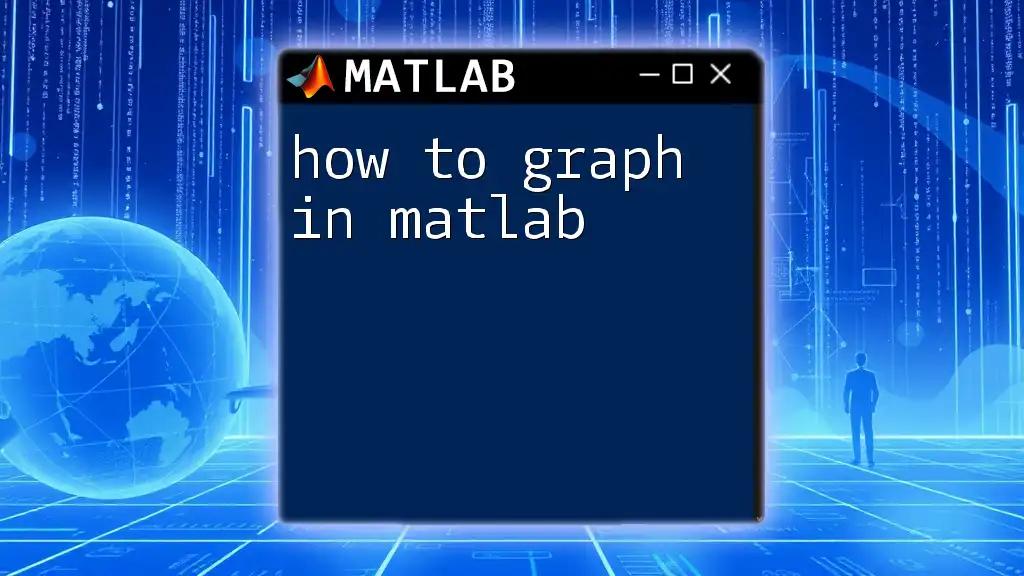
Adding Title and Legends
Importance of Titles and Legends
Titles and legends enhance the richness of your visualizations. While axis labels explicate the x, y, and z axes, a well-crafted title summarizes the entire plot's purpose. Legends provide clarity when multiple datasets or categories are represented, ensuring that each data group is easily identifiable.
Using `title` and `legend` Functions
You can add a title using the `title` function:
title('Sample Plot Title');
To include a legend that differentiates multiple data series:
legend('Dataset 1', 'Dataset 2');
By incorporating both a title and a legend, your graph becomes significantly more informative.

Multi-Plot Figures and Subplots
Labeling Axes in Subplots
When you have multiple plots (subplots) in a single figure, you can label each subplot individually using the same axis label functions. Here’s how you can do it:
subplot(1,2,1);
plot(x,y);
xlabel('X1');
ylabel('Y1');
title('First Plot');
subplot(1,2,2);
plot(y,x);
xlabel('X2');
ylabel('Y2');
title('Second Plot');
This example demonstrates how to manage axis labeling across different plots within the same figure canvas effectively, helping viewers distinguish between multiple datasets.
Coordinating Labels Across Figures
For consistent presentations, ensure that the style and positioning of labels are uniform across all subplots. This practice enhances clarity and professionalism in your visual outputs.
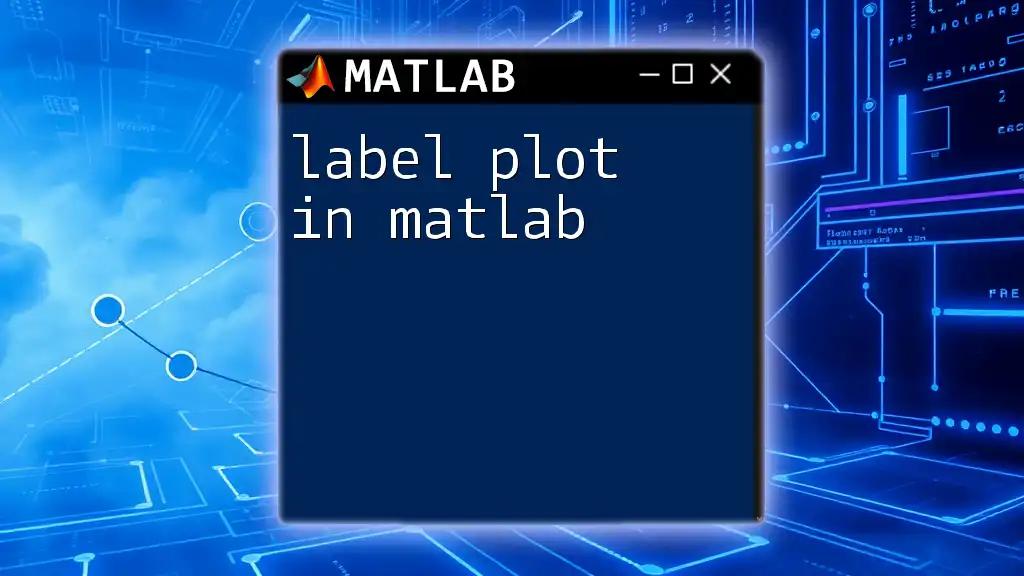
Common Errors and Troubleshooting
Common Issues With Axis Labeling
While labeling axes in MATLAB is often straightforward, various issues may arise. Some common problems to be aware of include:
- Label Overlaps: Sometimes labels may unintentionally overlap or run off the graph.
- Color Visibility: Labels may become difficult to read against certain plot backgrounds or data colors.
- Incorrect Font Sizes: Too large or too small font sizes can affect readability.
Troubleshooting Steps
To address these common errors, here are some helpful troubleshooting steps:
- Adjust Label Positions: Use the `'Position'` property in your label functions to move labels around.
- Change Font Size and Color: Experiment with different sizes and contrasting colors to ensure visibility.
- Use `set` for Fine Tuning: MATLAB's `set` function allows for detailed adjustments to label properties, ensuring everything aligns well visually.
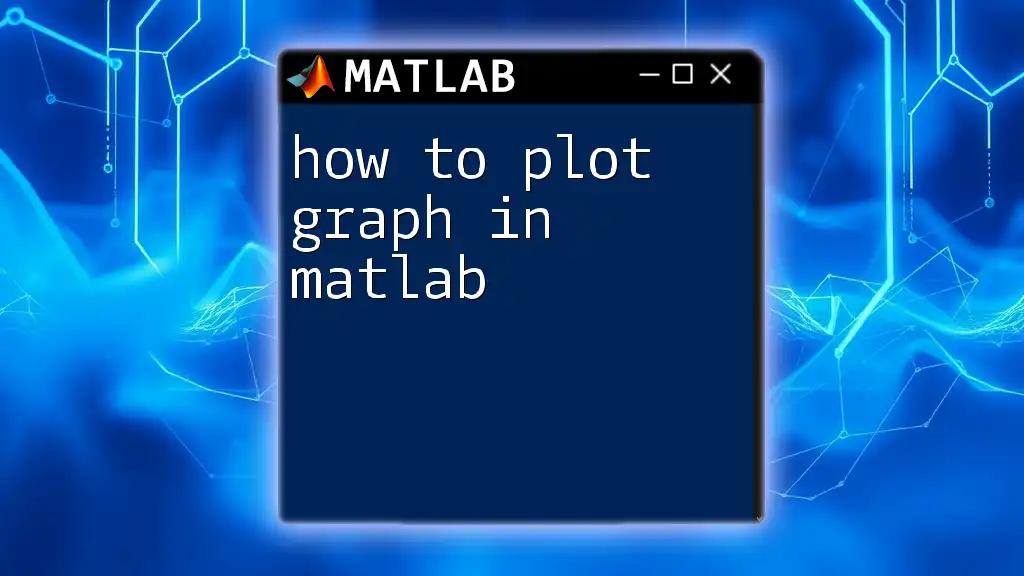
Conclusion
Labeling axes in MATLAB effectively is a vital skill for anyone looking to enhance their data visualizations. With the knowledge of various labeling functions, customization options, and troubleshooting techniques, you can create clear, informative, and visually appealing plots that communicate your data's story.
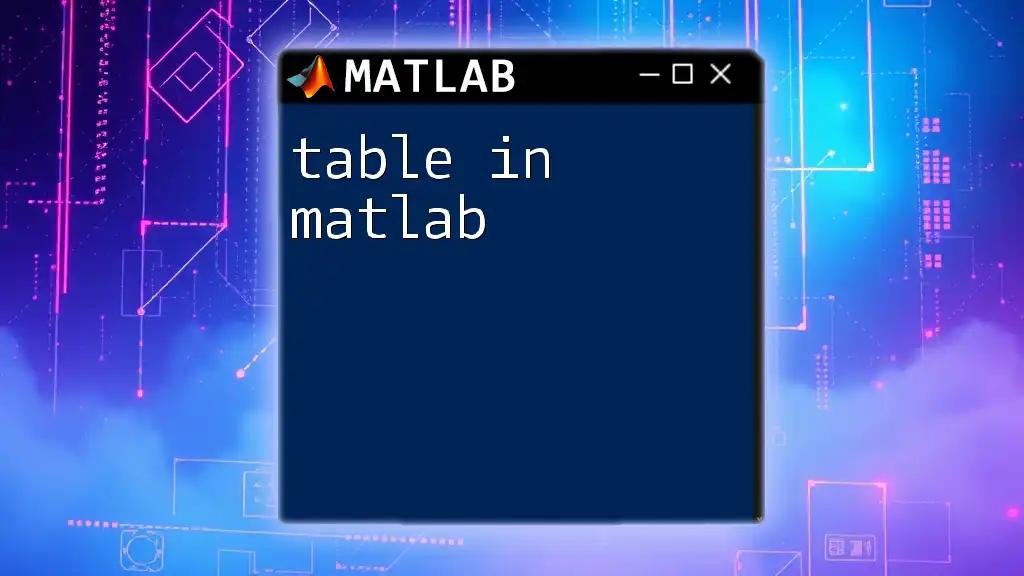
Additional Resources
For further exploration and mastery of MATLAB axis labeling, consider reviewing the MATLAB documentation and engaging with suggested tutorials that focus on graphical representations. Practicing these concepts will equip you with the tools needed to convey your insights through data visualization accurately.