In MATLAB, you can create a matrix by enclosing the elements in square brackets, separating the rows with semicolons, and the columns with spaces or commas.
A = [1 2 3; 4 5 6; 7 8 9];
Understanding Matrices in MATLAB
Definition of a Matrix
A matrix is essentially a rectangular array of numbers arranged in rows and columns. In MATLAB, matrices are fundamental data types that allow you to perform complex mathematical operations efficiently. For instance, the simplest form of a matrix is a two-dimensional array, represented like this:
A = [1 2 3; 4 5 6; 7 8 9];
This is a 3x3 matrix where the numbers are organized into three rows and three columns.
Different types of matrices exist, including:
- Row Matrix: A matrix with a single row (e.g., `[1 2 3]`).
- Column Matrix: A matrix with a single column (e.g., `[1; 2; 3]`).
- Square Matrix: A matrix where the number of rows and columns are equal (e.g., `[[1 2]; [3 4]]`).
Applications of Matrices
Matrices play a critical role in various fields, showcasing their versatility. Here are several applications:
- Engineering: Used extensively in control systems and signal processing to represent and manipulate data.
- Statistics: Essential for data representation and analysis, allowing for operations such as regression analysis.
- Computer Graphics: Matrices are vital for transformations, such as scaling, translation, and rotation in graphical applications.
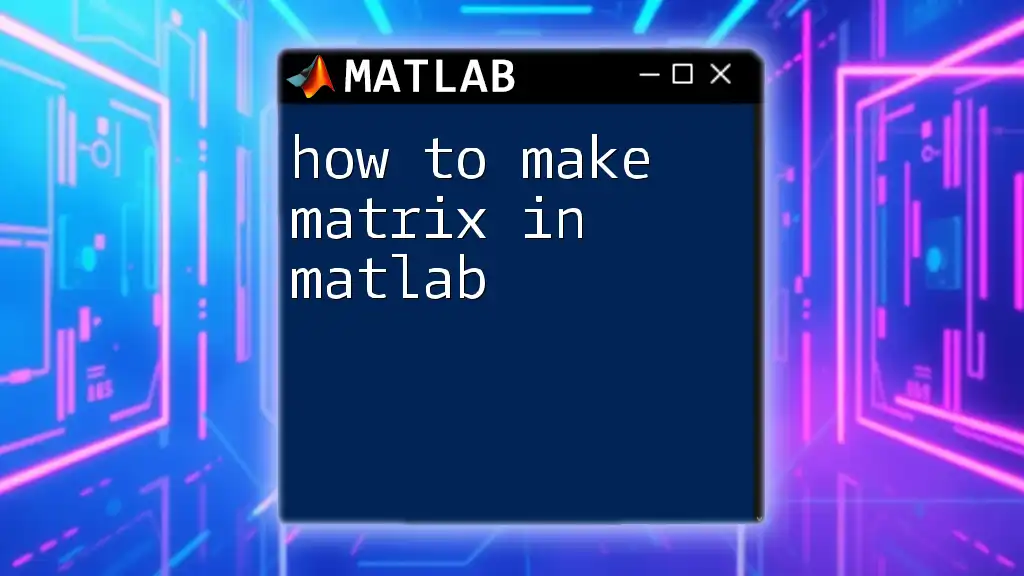
Creating a Matrix in MATLAB
Basic Syntax for Matrix Creation
To create a matrix in MATLAB, you simply enclose the elements in square brackets. Rows are separated by semicolons, while columns within the same row are separated by spaces or commas.
Here’s a simple example:
A = [1 2 3; 4 5 6; 7 8 9];
Creating 1D and 2D Matrices
Row and Column Vectors
A row vector is a 1xN matrix, while a column vector is an Nx1 matrix. Creating these in MATLAB is straightforward:
row_vector = [1, 2, 3]; % Row vector
column_vector = [1; 2; 3]; % Column vector
Creating 2D Matrices
You can create 2D matrices using standard brackets or with built-in functions. These include:
- `zeros`: Creates a matrix filled with zeros.
- `ones`: Creates a matrix filled with ones.
- `eye`: Generates an identity matrix.
Here are some examples:
Z = zeros(3); % 3x3 matrix of zeros
O = ones(2, 3); % 2x3 matrix of ones
I = eye(3); % 3x3 identity matrix
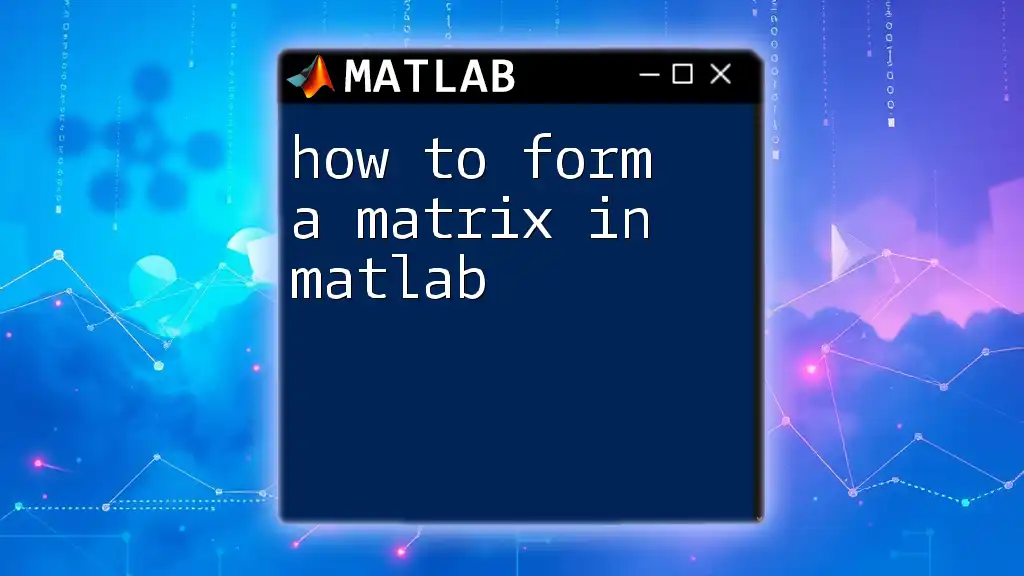
Advanced Matrix Creation Techniques
Using Functions for Matrix Generation
MATLAB provides various functions to create matrices dynamically. Some commonly used functions include:
- `rand`: Generates a matrix with random values between 0 and 1.
- `randi`: Produces a matrix with random integers within a specified range.
- `linspace`: Creates a row vector with linearly spaced elements.
Examples:
R = rand(3); % 3x3 matrix with random values
R_int = randi(10, 3, 3); % 3x3 matrix with random integers from 1 to 10
L = linspace(1, 10, 5); % Row vector of 5 points linearly spaced between 1 and 10
Concatenating Matrices
MATLAB allows you to concatenate matrices both horizontally and vertically, helping you build larger matrices from smaller ones.
- Vertical Concatenation: Adding rows to an existing matrix.
- Horizontal Concatenation: Appending columns to an existing matrix.
For example:
A = [1 2; 3 4];
B = [5 6];
C_row = [A; B]; % Vertical concatenation
C_col = [A, B']; % Horizontal concatenation
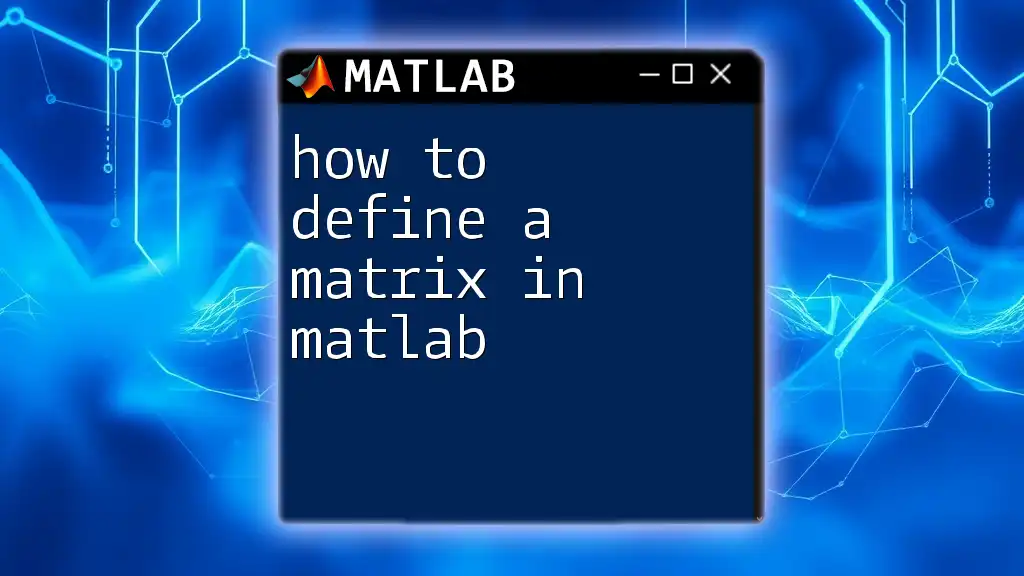
Accessing and Modifying Matrix Elements
Indexing in MATLAB
One of MATLAB’s strengths is its ability to easily access elements within a matrix. The indexing follows the syntax `matrix(row_index, column_index)`.
For example, to access the element at the 2nd row and 3rd column in matrix A, you would write:
element = A(2, 3); % Accessing the element in the 2nd row and 3rd column
You can also access entire rows or columns using colon notation. For instance, to access all rows of the 2nd column:
sub_matrix = A(:, 2); % Accessing all rows of the 2nd column
Modifying Matrix Elements
Modifying values within a matrix is as simple as assigning a new value to a specific index. Here’s how to do it:
A(1, 1) = 10; % Changing the first element to 10
This allows for dynamic manipulations of matrices, crucial for iterative algorithms and data processing.
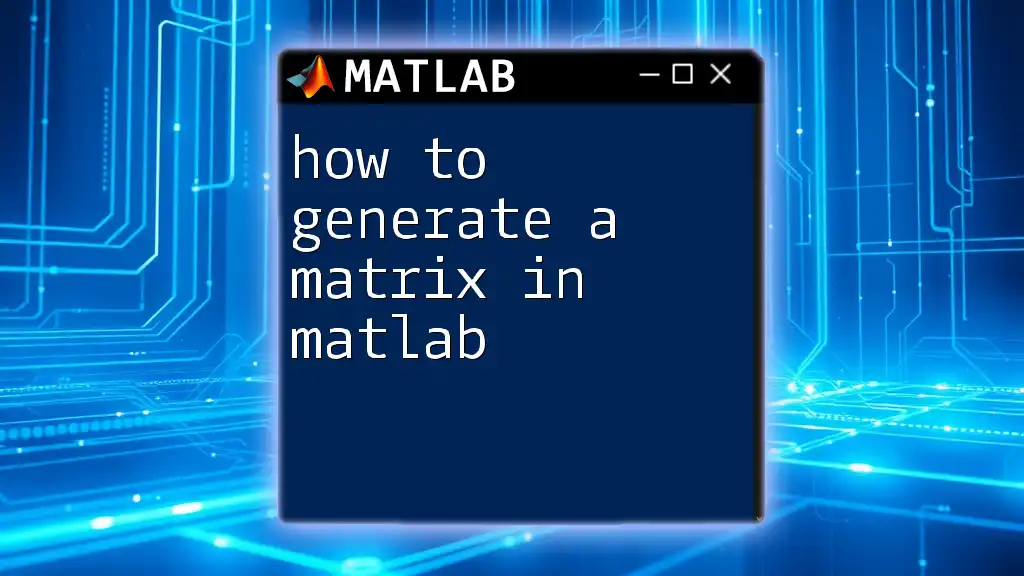
Useful Tips for Working with Matrices in MATLAB
When working with matrices in MATLAB, consider these best practices:
- Preallocate Memory: To improve performance, preallocate space for large matrices instead of dynamically growing them inside a loop.
- Vectorization: Use vectorized operations instead of loops wherever possible to speed up calculations and simplify code.
- Avoid Using Global Variables: Keep your workspace clean by avoiding unnecessary global variables, which can lead to confusion and bugs.
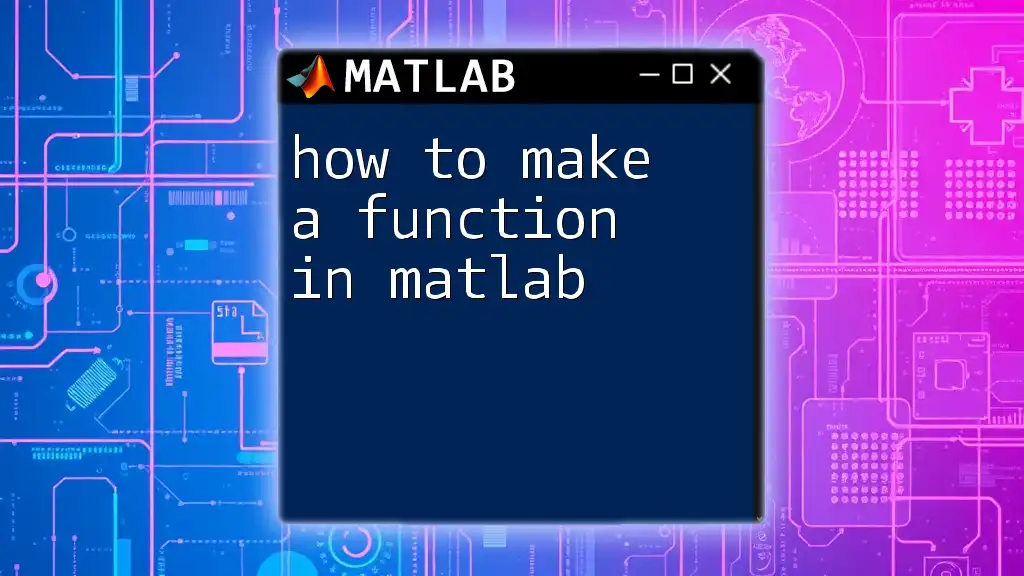
Conclusion
In this guide, we've delved into how to make a matrix in MATLAB, exploring everything from basic definitions to advanced creation techniques and practical applications. Mastering matrices is vital for effective data analysis and mathematical computations in MATLAB. Practice creating and manipulating matrices to strengthen your understanding, and consider exploring our MATLAB courses for a deeper dive into this powerful tool!