To write a function in MATLAB, you define it using the `function` keyword, specify the output and input parameters, and then include the code to execute within the function body.
function output = myFunction(input)
output = input^2; % Example: returns the square of the input
end
Understanding Functions in MATLAB
What is a Function?
A function in MATLAB is a reusable block of code that performs a specific task. It is defined with a unique name and can accept input parameters while returning output values.
Unlike scripts, which are sequences of MATLAB commands executed together, functions promote code reusability and modularity. This enables you to break down complex problems into smaller, manageable pieces, making your code easier to understand and maintain.
Why Use Functions?
Functions offer several compelling benefits:
- Modularity: You can develop smaller, self-contained pieces of code that can be tested independently.
- Reusability: Once written, functions can be reused throughout your projects, saving time and effort.
- Simplicity: Functions can encapsulate complex tasks, allowing you to call a single function rather than writing out the entire procedure each time.
- Readability: By using clear function names and comments, your code becomes more intuitive, streamlining future modifications.
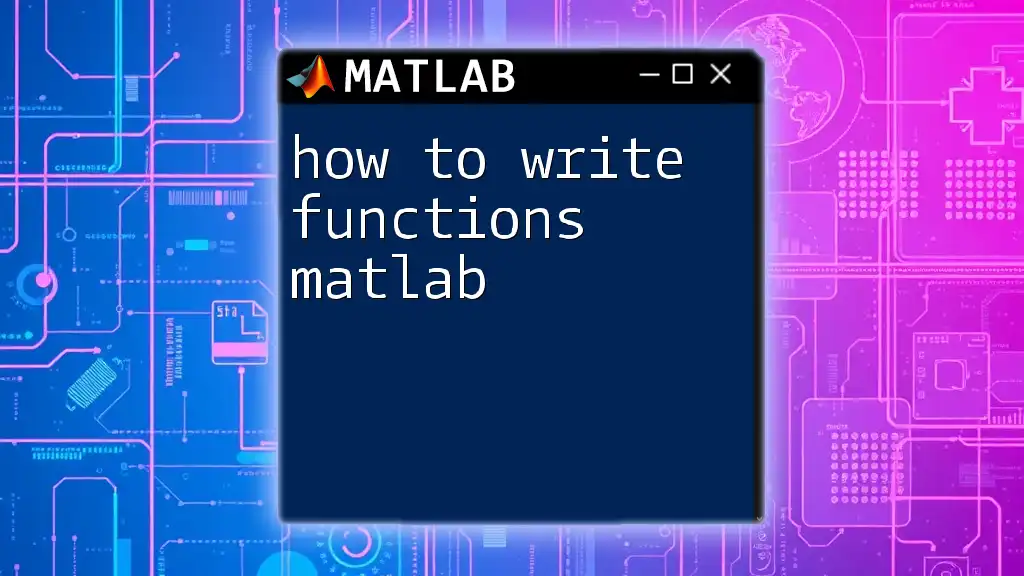
Basic Structure of a Function
Syntax of a Function in MATLAB
The general format for defining a function in MATLAB is:
function [output1, output2] = functionName(input1, input2)
This syntax indicates that a function starts with the `function` keyword, includes a list of output variables in square brackets, is followed by the function name, and finally lists the input parameters in parentheses.
Example of a Simple Function
Let’s create a simple function that adds two numbers together.
function sum = addNumbers(a, b)
sum = a + b;
end
In this example, the function addNumbers takes two inputs, a and b, and returns their sum. The function's purpose is concise and straightforward, making it easy to use and understand.
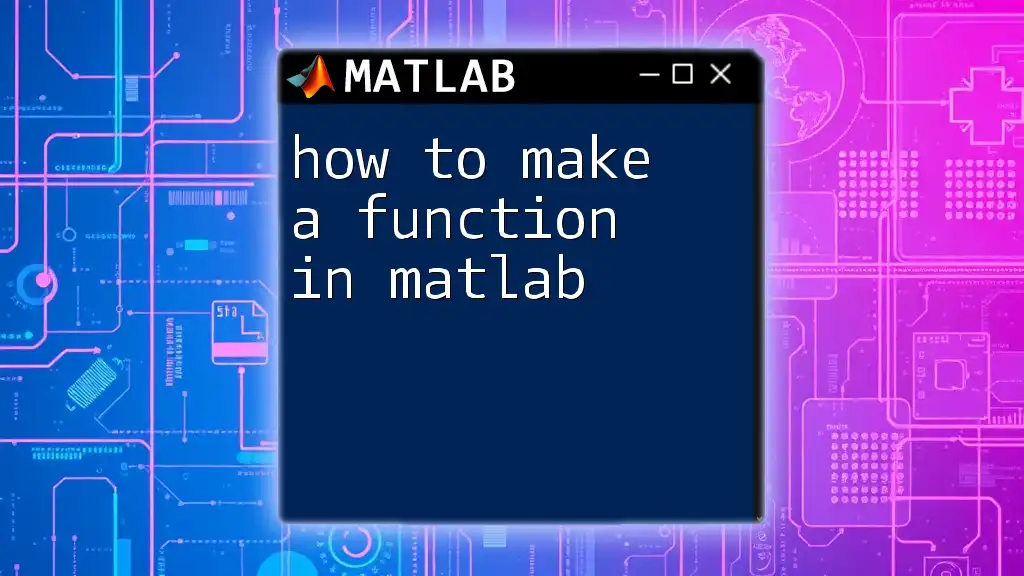
Writing a MATLAB Function Step-by-Step
Step 1: Define the Function
Choosing a meaningful name is key when defining your function. The name should clearly describe what the function does, making it easier for others (or yourself in the future) to understand its purpose.
Step 2: Specify Input and Output Variables
When defining input and output variables, strive for clarity. Use descriptive names that convey the data's meaning. For example, instead of naming an input variable x, consider naming it inputValue.
Step 3: Implement the Function Logic
When writing the core logic of the function, ensure that it's straightforward. For instance, let’s create a function that computes the factorial of a number.
function result = factorial(n)
if n == 0
result = 1;
else
result = n * factorial(n - 1);
end
end
In this recursive function:
- The base case is when n equals 0, returning 1.
- Otherwise, the function calls itself, multiplying n by the factorial of n-1. This elegant solution showcases how functions can express complex calculations succinctly.
Step 4: Save the Function File
When saving your function, always use a filename that matches the function name. In this case, save it as addNumbers.m or factorial.m. MATLAB requires that the function's filename correspond exactly to the function name in order for it to be recognized.
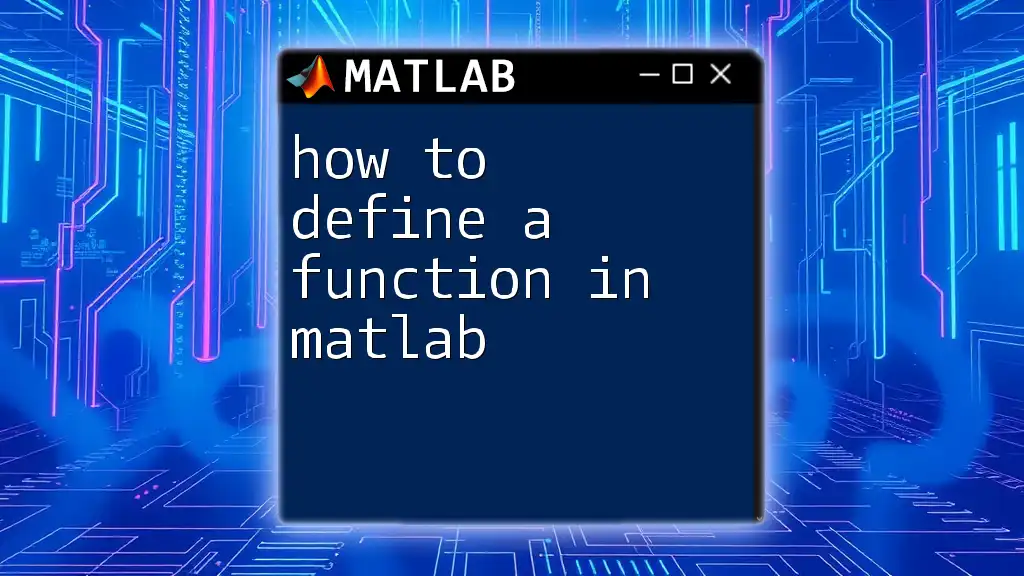
Calling a MATLAB Function
How to Call a Function
Calling a function in MATLAB is straightforward. The syntax is as follows:
result = functionName(args);
For example, to call the addNumbers function we defined earlier, you would write:
result = addNumbers(10, 5);
This line of code will store the output in the variable result.
Handling Multiple Outputs
Functions in MATLAB can return multiple outputs. The number of output arguments can be checked using `nargout`. Here’s an example of a function that returns both the sum and the product of two numbers:
function [sum, product] = mathOperations(a, b)
sum = a + b;
product = a * b;
end
When calling this function, you can capture the outputs as follows:
[aSum, aProduct] = mathOperations(4, 5);
By specifying the function’s output variables, you can effectively manage multiple results from a single function call.
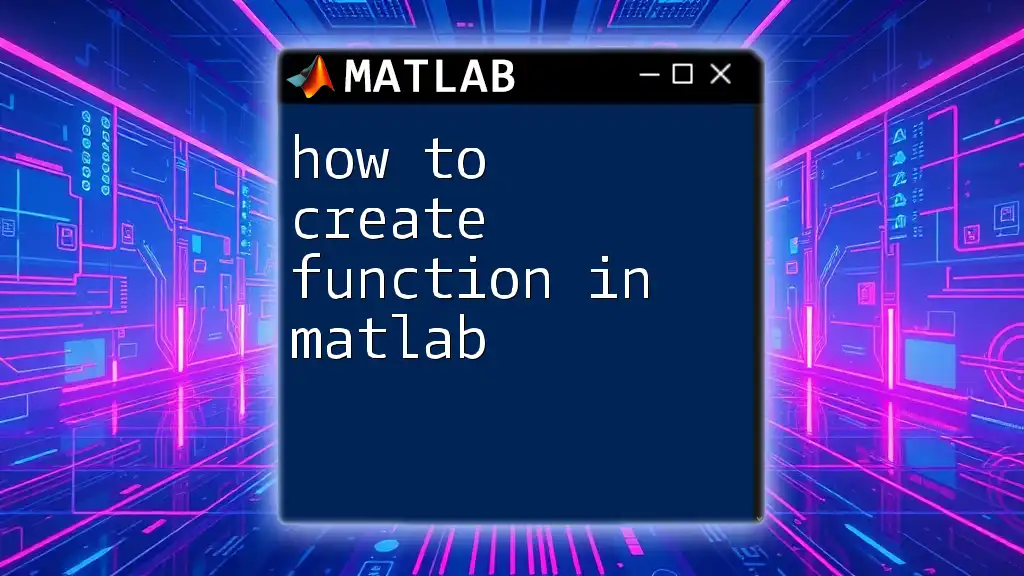
Tips and Best Practices
Keeping Functions Concise
It is essential to keep functions simple and focused. A good function should perform one specific task. This makes it easier to debug and understand. If your function starts becoming lengthy, consider breaking it down into smaller sub-functions.
Documentation and Comments
Always include comments within your functions to explain the logic behind your code. This is especially critical for complex portions of your function. Use a help comment at the beginning to describe the function's purpose and usage, as shown below:
% Function to add two numbers
function sum = addNumbers(a, b)
% This function takes two inputs and returns their sum
sum = a + b;
end
Debugging Functions
Debugging is an essential skill. Common issues include incorrect input types and variable scope problems. Use MATLAB's built-in debugging tools, such as breakpoints, to step through your functions and inspect variables at runtime. This helps you identify errors effectively.
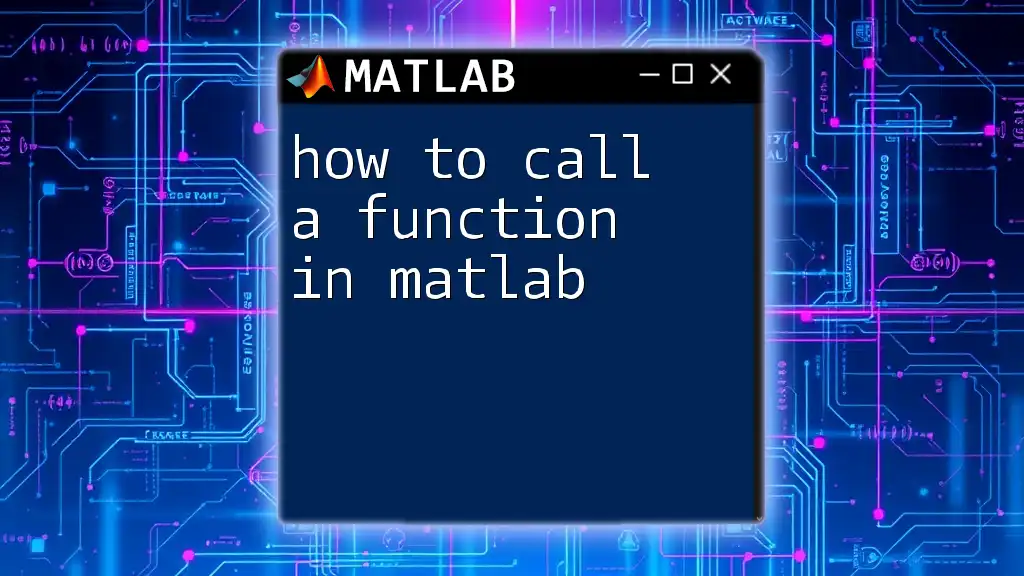
Conclusion
In summary, knowing how to write a function in MATLAB is a fundamental skill that enhances your programming efficiency. By understanding the structure, implementation, and best practices of functions, you can create cleaner, more manageable code. Remember to experiment with creating your own functions and refer to MATLAB's documentation for further learning resources. Happy coding!