In MATLAB, you can create a matrix by inputting the elements within square brackets, separating the rows with semicolons and the columns with spaces or commas. Here’s a code snippet to create a simple 2x2 matrix:
A = [1, 2; 3, 4];
Understanding Matrices in MATLAB
What is a Matrix?
A matrix in MATLAB is a two-dimensional array of numbers arranged in rows and columns. Matrices can be used to represent a variety of mathematical concepts, from simple data storage to complex structures used in engineering and scientific calculations. Matrices play a crucial role in fields such as computer vision, machine learning, signal processing, and more.
Why Use Matrices?
Matrices allow for efficient computations and are central to linear algebra, which forms the basis for many algorithms in data analysis and optimization. By understanding how to manipulate and operate with matrices, you can solve systems of equations, perform transformations, and analyze data quickly. Common applications include:
- Solving linear equations
- Image processing
- Simulating systems in physics and engineering
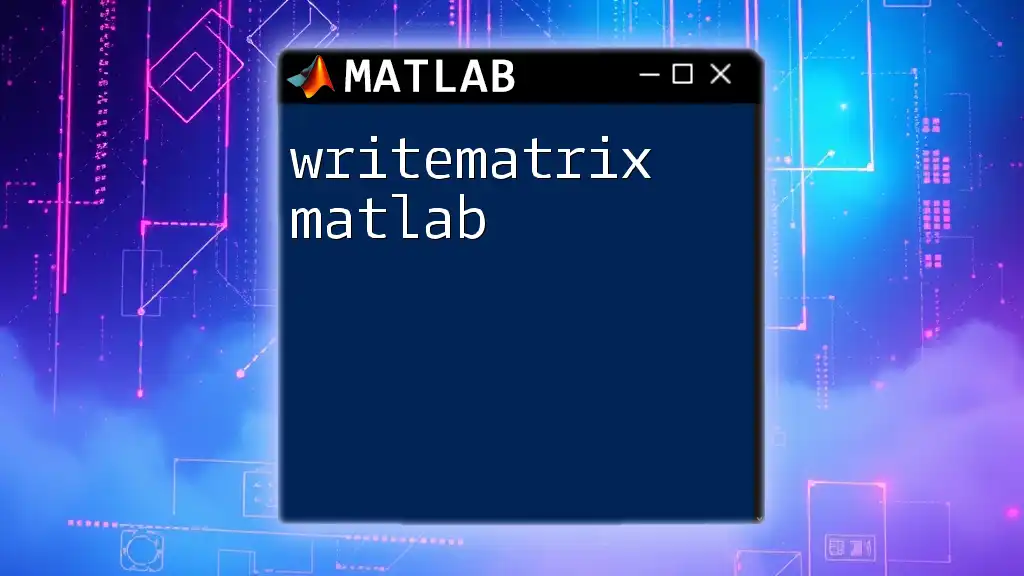
Creating a Matrix in MATLAB
Using Brackets
In MATLAB, creating a matrix can be easily accomplished using square brackets. Rows are separated by semicolons, while columns are separated by commas. For example:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
This command generates a 3x3 matrix named `A`. Each row of the matrix is separated by a semicolon, and the entries within a row are separated by commas.
Using the `zeros`, `ones`, and `eye` Functions
MATLAB provides several built-in functions that enable you to create matrices of a specific type:
- Creating a Matrix with Zeros
B = zeros(3); % 3x3 matrix of zeros
The `zeros` function is particularly useful when initializing matrices that will be filled with computed values later.
- Creating a Matrix with Ones
C = ones(2,4); % 2x4 matrix of ones
The `ones` function is handy for creating a matrix filled with ones, which can be useful in algorithms requiring a uniformity.
- Creating an Identity Matrix
D = eye(4); % 4x4 identity matrix
The `eye` function produces an identity matrix, crucial in matrix operations, particularly for matrix inversion and linear transformations.
Using Random Matrices
The generation of matrices with random values can be achieved using the `rand` function. For example:
E = rand(3); % 3x3 matrix with random values
Random matrices often model stochastic processes or serve as initial conditions in simulations.
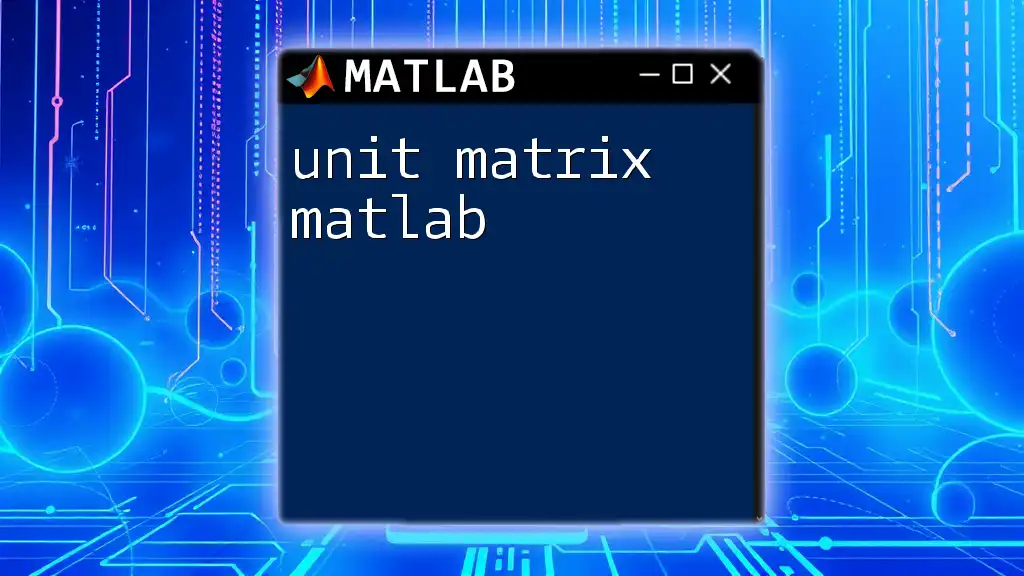
Accessing and Modifying Matrices
Indexing Basics
Accessing the elements of a MATLAB matrix is straightforward. You can use parentheses for indexing. For example:
value = A(2, 3); % Accessing element in row 2, column 3
This retrieves the element from the second row and the third column of matrix `A`. Understanding indexing is essential for data manipulation in MATLAB.
Modifying Matrix Elements
If you want to update a specific element, you simply assign a new value as follows:
A(1, 1) = 10; % Changing the value of the first element
This command modifies the first element of matrix `A` to 10. Familiarity with accessing and modifying elements is foundational for effective matrix manipulation.
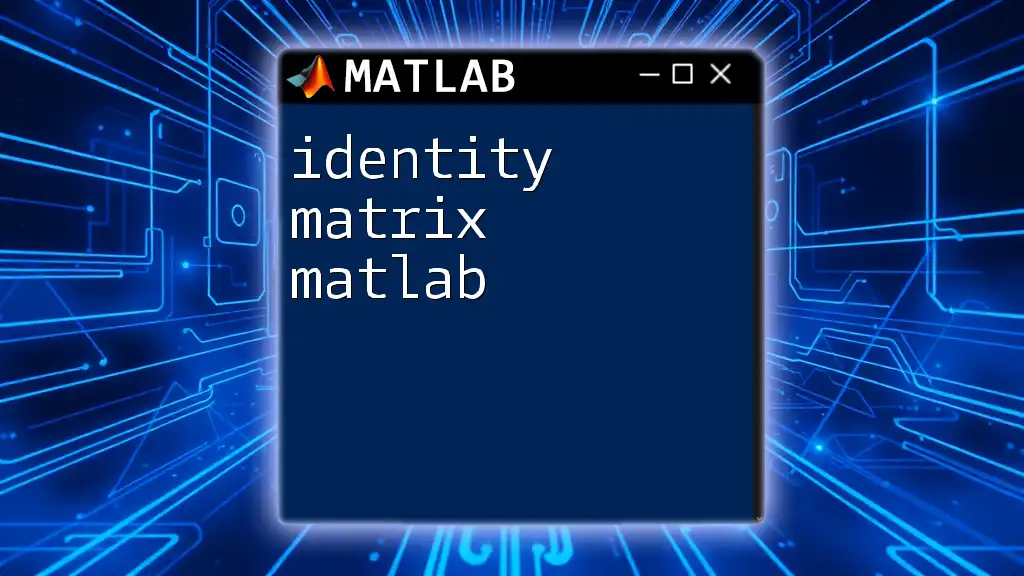
Matrix Operations
Basic Operations
MATLAB makes it easy to perform various matrix operations, including addition and subtraction. For example:
F = A + B; % Matrix addition
G = A - B; % Matrix subtraction
When adding or subtracting matrices, ensure that the matrices have the same dimensions; otherwise, MATLAB will return an error.
Multiplication
Matrix multiplication is distinct from element-wise multiplication. The command for matrix multiplication is:
H = A * F; % Matrix multiplication
This operation follows rules specific to linear algebra. If both matrices are n x m and m x p, the result will be an n x p matrix.
For element-wise multiplication, use the `.*` operator:
J = A .* B; % Element-wise multiplication
Understanding the difference between these two operations is crucial for successful matrix calculations.
Transpose of a Matrix
To transpose a matrix, which swaps the rows and columns, you use the transpose operator:
A_transpose = A'; % Transposing a matrix
Transposed matrices are frequently used in mathematical proofs and various transformations within algorithms.
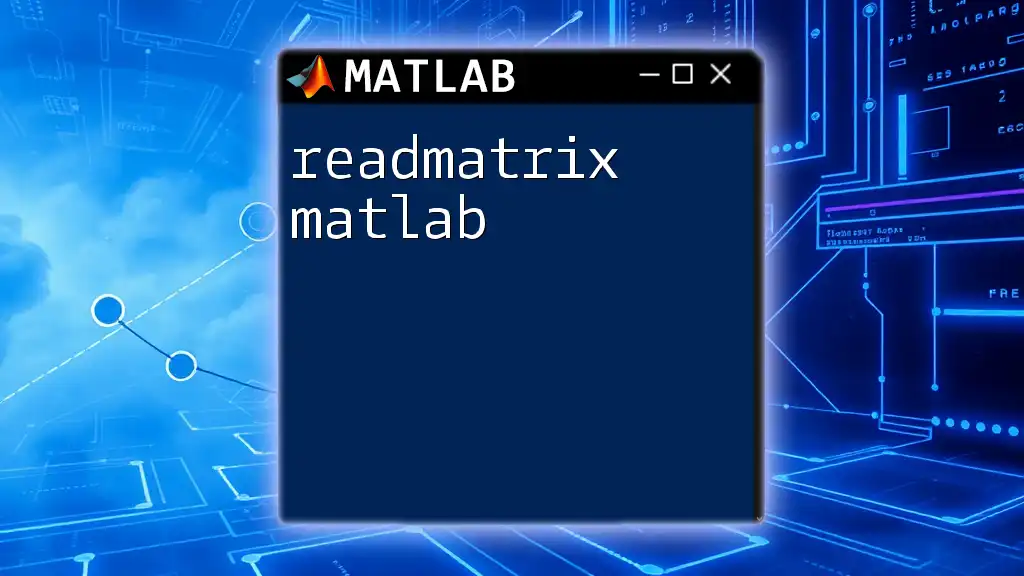
Reshaping and Combining Matrices
Reshaping Matrices
The `reshape` function allows you to change the dimensions of a matrix without changing the underlying data:
K = reshape(A, 1, 9); % Reshaping A into a row vector
This command converts matrix `A` into a 1x9 row vector. Reshaping is useful for organizing data in various formats for analysis or plotting.
Concatenating Matrices
You can combine matrices using square brackets either vertically or horizontally:
L = [A; B]; % Vertical concatenation
M = [A, C]; % Horizontal concatenation
Horizontal concatenation combines matrices side by side, while vertical concatenation stacks matrices atop one another. This ability allows for the construction of larger datasets or complex structures.
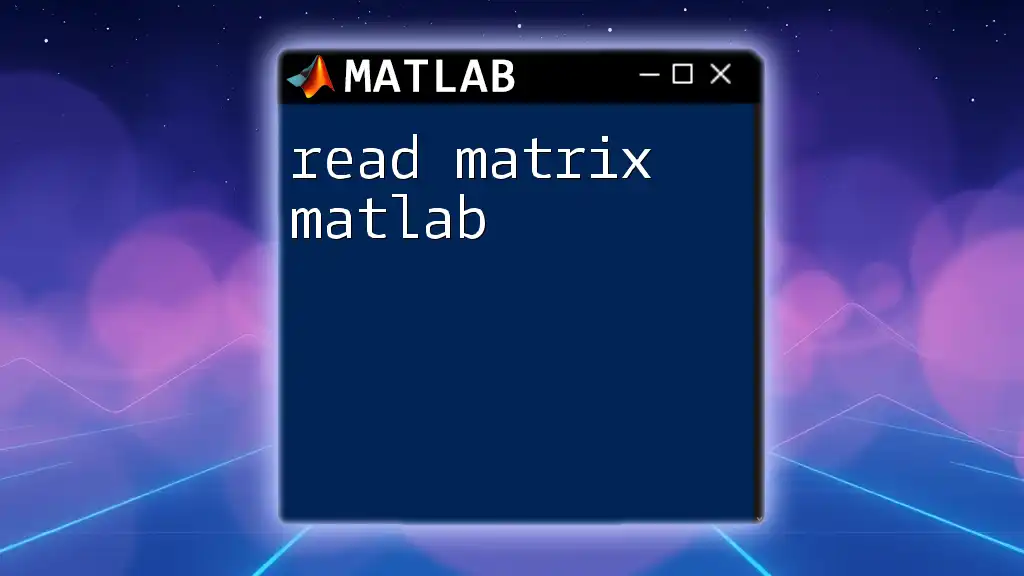
Saving and Loading Matrices
Saving Matrices to Files
To preserve your work, use the `save` function to write a matrix to a file:
save('myMatrix.mat', 'A'); % Save matrix A to a file
You can specify the format (e.g., ASCII, binary) based on your needs, making it easy to share or retrieve data.
Loading Matrices from Files
If you need to access saved matrices, you can retrieve them using:
load('myMatrix.mat'); % Load the saved matrix
Efficient saving and loading of matrices can streamline your workflow, especially when working with large datasets.
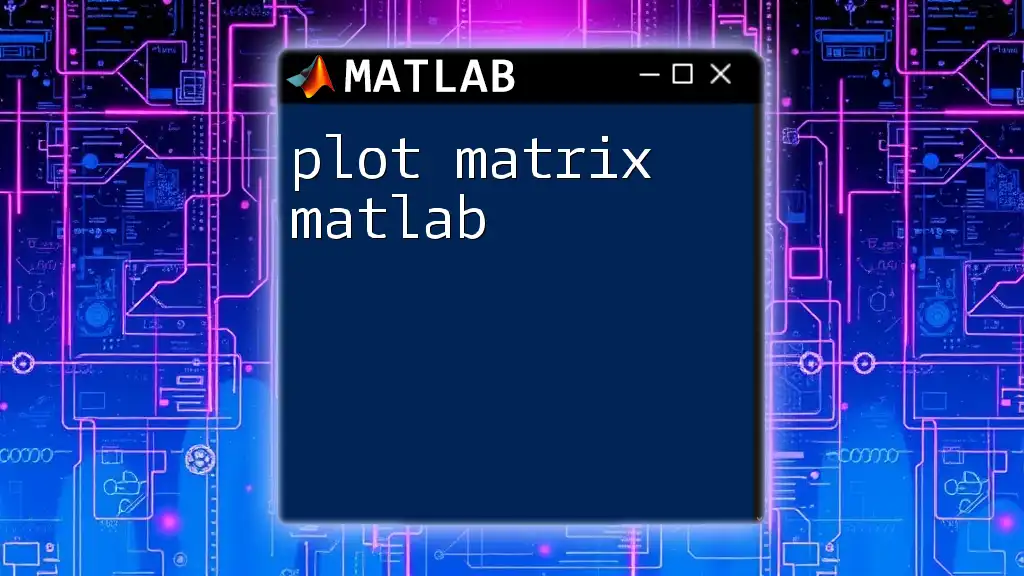
Best Practices for Using Matrices in MATLAB
Commenting Code
Commenting within your code helps improve readability and provides clarity for complex operations. Use the `%` sign to add comments, which can be especially useful for reminders on matrix functions and operations.
Organizing Code
Proper organization of your code is vital for readability and maintenance. Group related tasks, use functions to modularize code, and follow a standard style guide to enhance clarity.
Error Handling
Common errors in matrix manipulation include mismatched dimensions during operations and referencing data elements outside the matrix's size. Understanding error messages and using functions like `size()` can aid in debugging issues promptly.
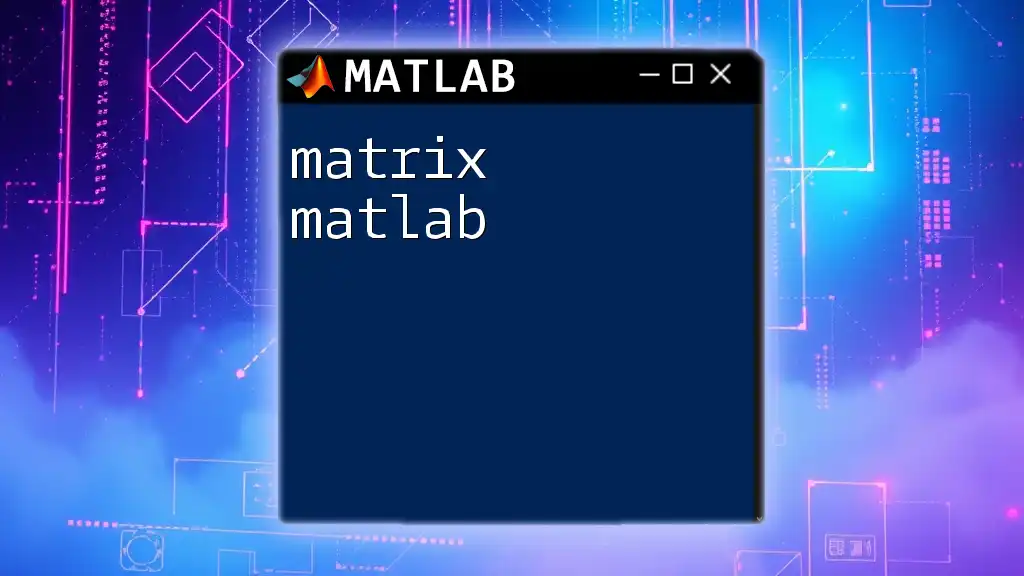
Conclusion
In this comprehensive guide on how to write matrix in MATLAB, you have explored the fundamental aspects of matrix creation, manipulation, and operations. Awakening a solid understanding of matrices can significantly enhance your MATLAB capabilities and open doors to diverse applications in various fields.
Now that you have the foundation, practice creating and manipulating matrices to deepen your understanding and strengthen your skills. This will not only elevate your proficiency in MATLAB but also equip you to tackle more complex mathematical challenges with confidence.
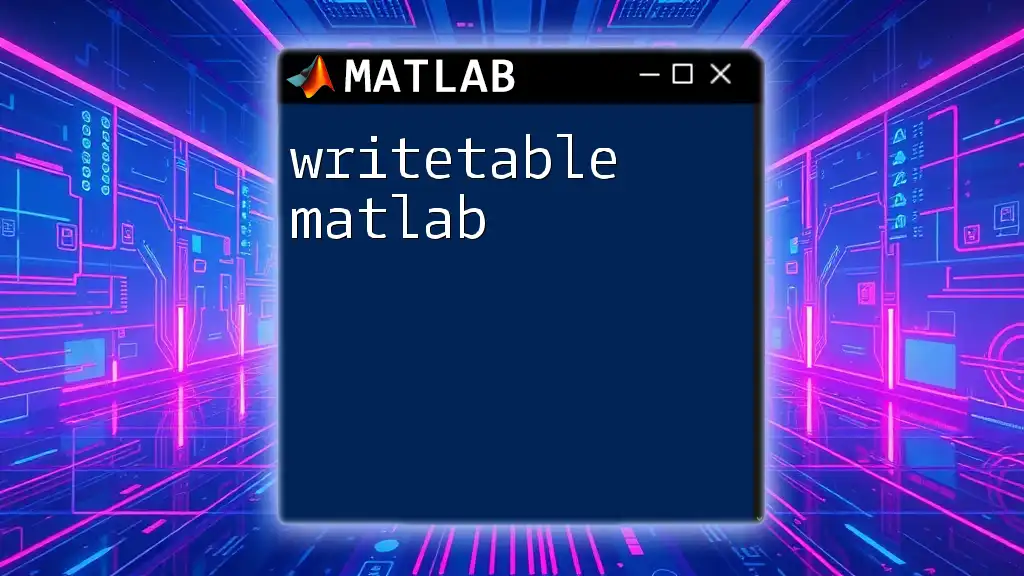
Further Resources
To continue expanding your knowledge, consider exploring MATLAB's documentation and community forums. These resources provide additional insights and support for your journey in mastering MATLAB and matrix operations.