The `writematrix` function in MATLAB is used to write a matrix to a file, allowing users to easily export data in various formats like CSV or text files.
Here's a code snippet demonstrating its usage:
% Define a matrix
data = [1, 2, 3; 4, 5, 6; 7, 8, 9];
% Write the matrix to a CSV file
writematrix(data, 'output.csv');
Understanding writematrix
The `writematrix` function in MATLAB is a powerful tool designed to facilitate the exporting of matrices and other numeric data to external files quickly and efficiently. It can handle a variety of data types, making it an essential function for data analysis and sharing results.
Definition of writematrix
`writematrix` is a MATLAB function that allows users to write matrices to various file formats, including CSV, text, and Excel. The function's flexibility supports varying types of data, ensuring a seamless transition from MATLAB to external files.
Functionality
The primary purpose of `writematrix` is to write data from MATLAB into known formats that can be easily accessed by other software. It easily outputs numeric arrays, making it ideal for data analysis, reporting, and collaboration. Whether exporting results for a client or archiving data for future reference, `writematrix` is an invaluable asset.
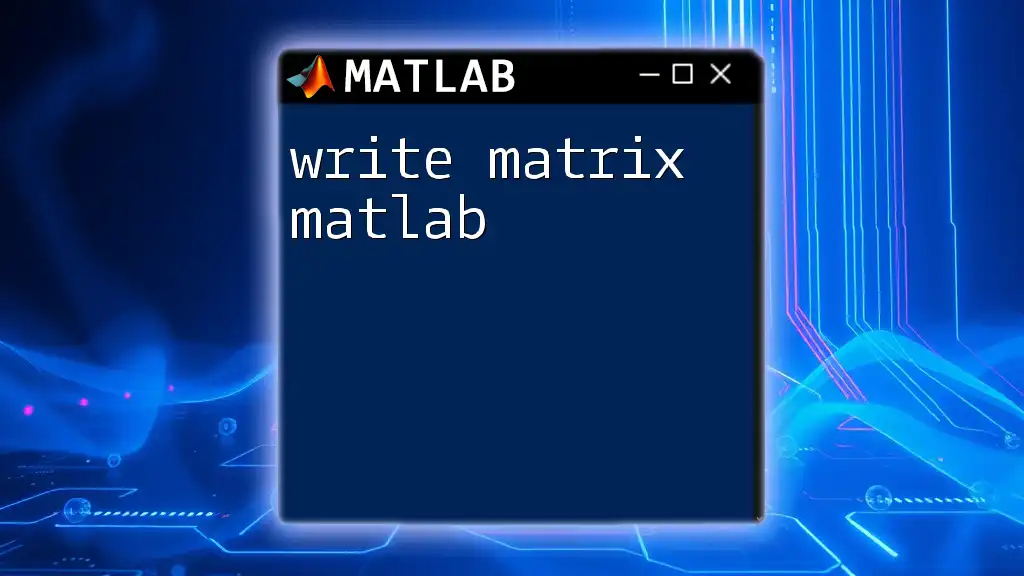
Syntax of writematrix
Basic Syntax
The basic syntax for `writematrix` is straightforward:
writematrix(A)
In this syntax, A represents the matrix you wish to write to a file. This is the fundamental form of the function and will create a default file named 'Untitled.csv' in the current directory.
Advanced Syntax Options
For a more tailored output, users can utilize the advanced syntax:
writematrix(A, filename, Name, Value)
- filename: Specifies the desired name for the output file, allowing you to customize your file organization.
- Name, Value: These are optional name-value pairs that enable customization of various parameters:
- 'Delimiter': Specifies the character used to separate values. Default is a comma, but it can be set to tab, space, etc.
- 'FileType': Allow users to define the type of output file such as 'text' or 'spreadsheet.'
- 'Sheet': For Excel files, this defines the sheet where data will be written, making it particularly useful when working with multiple datasets.
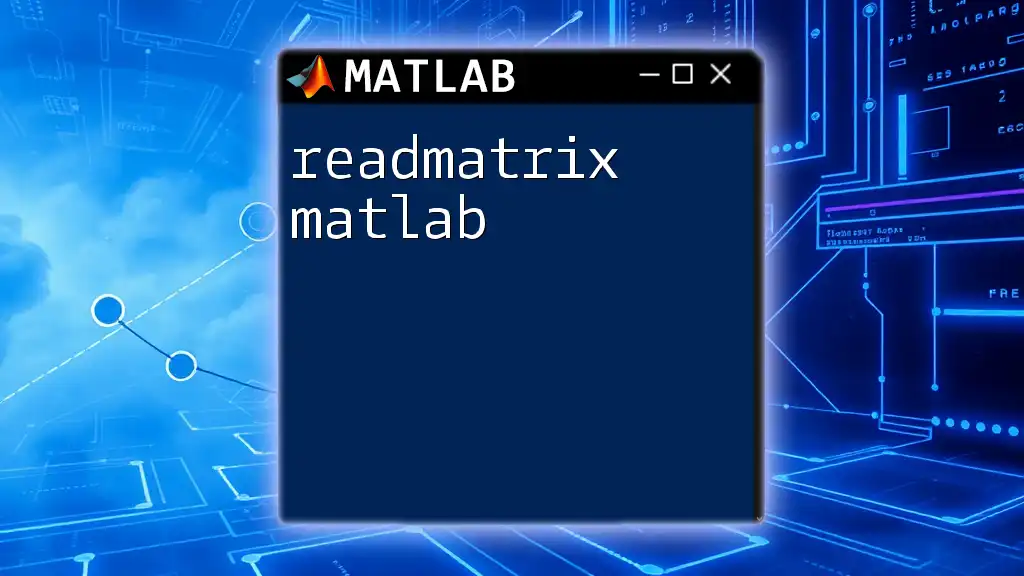
Writing Matrices to Different File Formats
CSV Files
How to Write to CSV
Writing to CSV files is one of the most common uses of `writematrix`. The process is as simple as:
writematrix(A, 'data.csv')
Example
Consider the following example where we create a 5x5 matrix of random numbers and export it as a CSV file:
A = rand(5); % Create a 5x5 random matrix
writematrix(A, 'data.csv'); % Write to 'data.csv'
After running this code, users can easily open the `data.csv` file in applications like Excel or any text editor to inspect the exported data.
Text Files
Writing to Text Files
Exporting matrices to text files is also seamless. The syntax allows specifying delimiters, such as tabs:
writematrix(A, 'data.txt', 'Delimiter', 'tab')
Example
Here's an example where we write a random matrix to a tab-delimited text file:
writematrix(A, 'data.txt', 'Delimiter', 'tab'); % Write to 'data.txt' with tab as the delimiter
This command creates a text file named `data.txt`, which users can review in any text editor, presenting the matrix data in a readable format.
Excel Files
Writing to Excel
`writematrix` also supports exporting data to Excel files. The syntax resembles the previous examples but includes a sheet option:
writematrix(A, 'data.xlsx', 'Sheet', 'Sheet1')
Example
To write a matrix to an Excel file, you might use:
writematrix(A, 'data.xlsx', 'Sheet', 'MyData'); % Write to 'MyData' sheet of 'data.xlsx'
This command places the matrix in a specifically named sheet, ensuring clarity when working with multiple datasets.
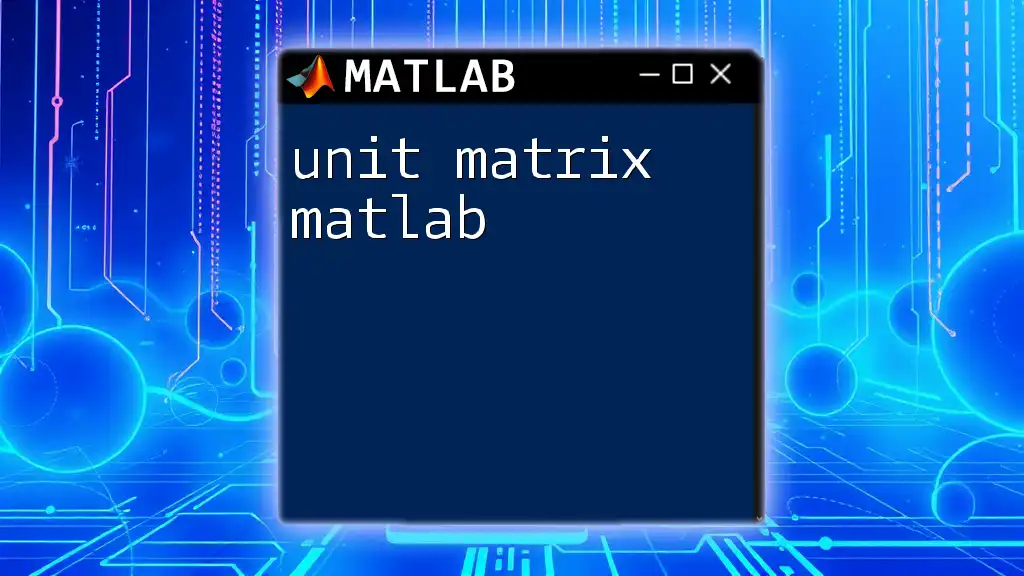
Handling Different Data Types
Writing Tables
For instances where matrices need to be converted to tables before exporting, users can do so using the `table` function, ensuring that the data maintains its structure. For example:
T = array2table(A); % Convert matrix A to table T
writematrix(T, 'data_table.csv'); % Write the table to a CSV file
Mixed Data Types
When dealing with matrices that contain mixed data types, it’s advisable to convert the matrix to a table using `array2table` before writing it out. This ensures that all data types are appropriately formatted and exported.
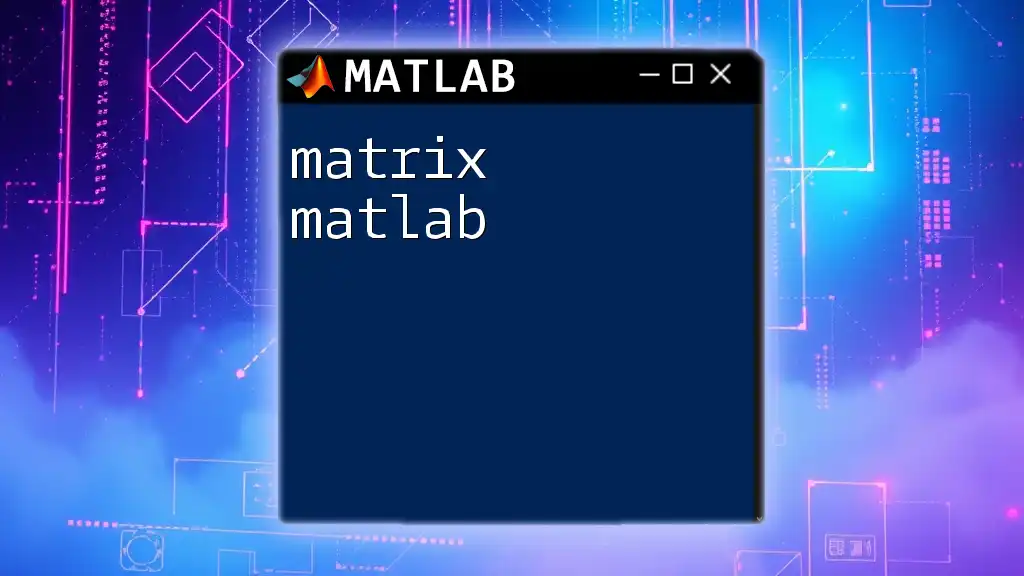
Common Errors and Troubleshooting
File Not Found Errors
Users often encounter "file not found" errors when the specified file path is incorrect. Double-check the path or ensure you have the required permissions for the directory you are writing to.
Data Loss on Open
Sometimes, data might appear truncated or corrupted when files are opened. This can happen if the specified format does not align with the data being written. Using the proper delimiter and file type is crucial for maintaining data integrity.
General Tips for Avoiding Errors
- Verify File Name and Path: Always ensure that the file name and path are correctly specified.
- Check Data Types: Ensure that data types align with the expected output format.
- Do a Test Write: Initially writing a smaller matrix can prevent larger errors.
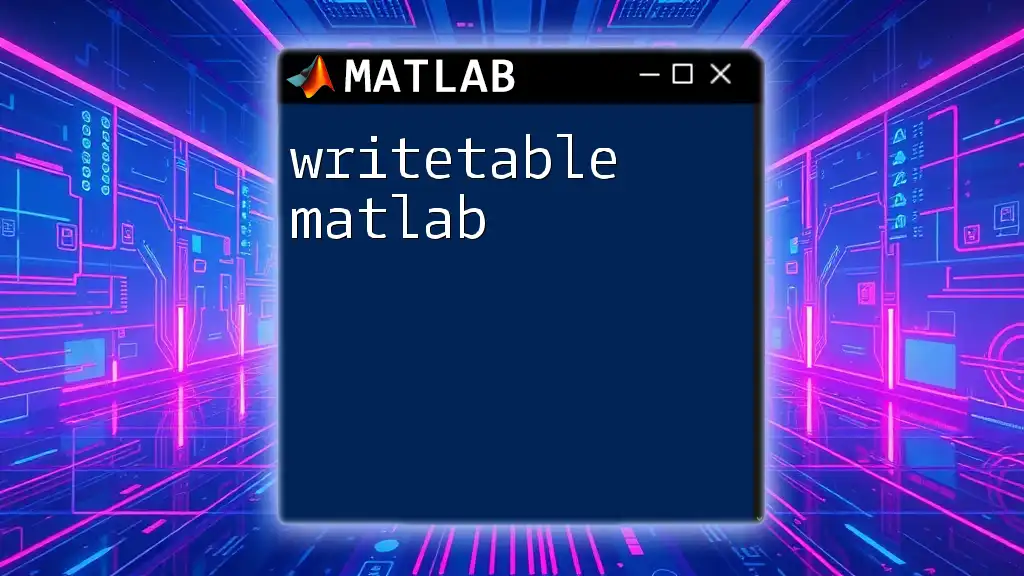
Real-World Applications of writematrix
Data Analysis Projects
Researchers and analysts utilize `writematrix` frequently in data analysis projects to export results for visualization, reporting, or archiving. The ability to send matrices outside of MATLAB enhances workflow efficiency.
Collaboration and Sharing
In collaborative environments, sharing matrices or datasets is vital. `writematrix` provides an organized way to distribute data among team members or clients, making it easier to discuss and analyze results collaboratively.
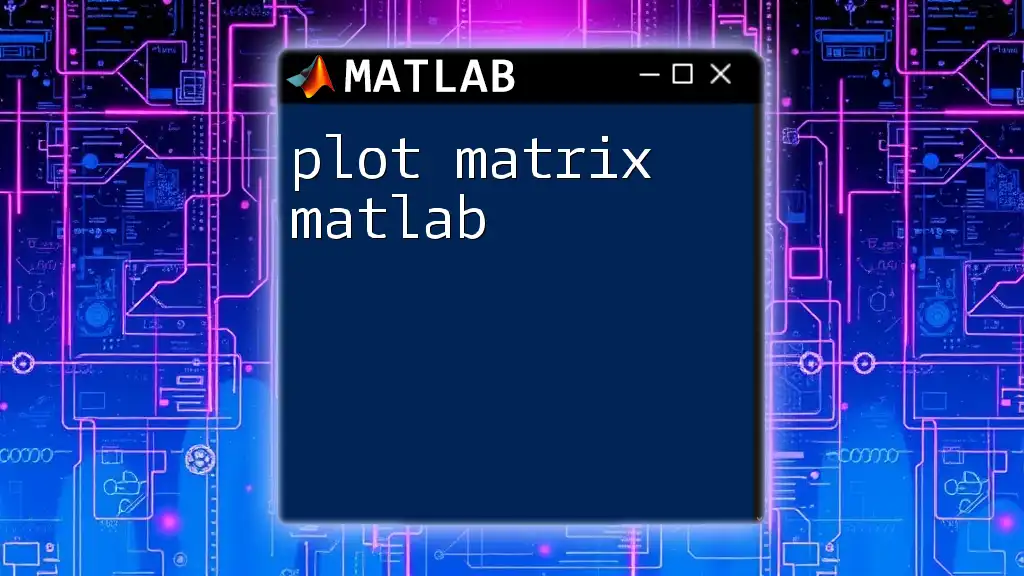
Conclusion
Understanding `writematrix` is paramount for effective data management within MATLAB. Practicing this function will empower users to manage and export their data smoothly, enhancing their overall data analysis capabilities. Engage with `writematrix` today and improve your data handling skills within MATLAB.
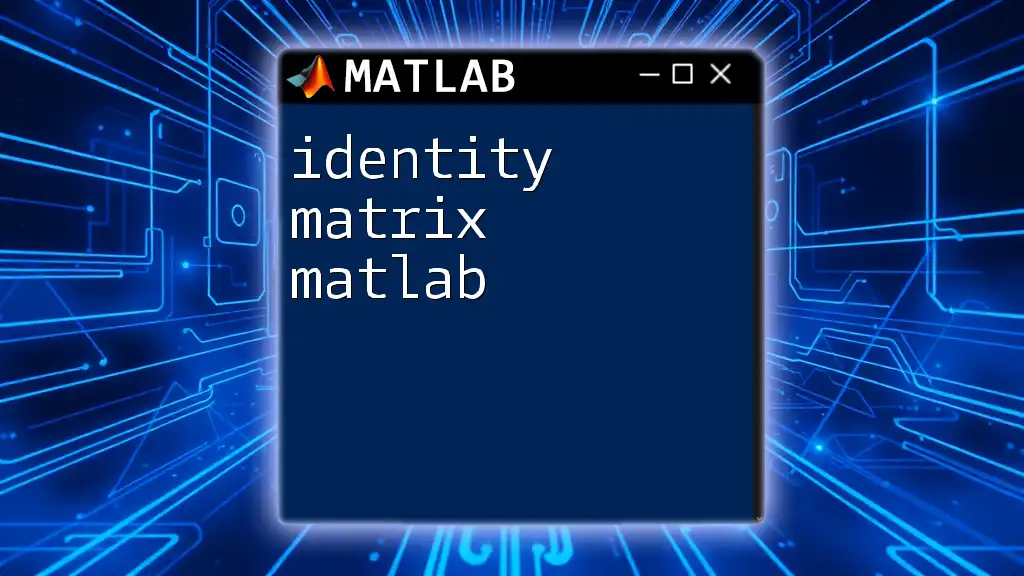
Additional Resources
For further reading and deeper exploration of other functionalities, refer to the official MATLAB documentation on `writematrix`. Consider exploring examples and exercises that focus on writing to different file formats to enhance your learning experience.
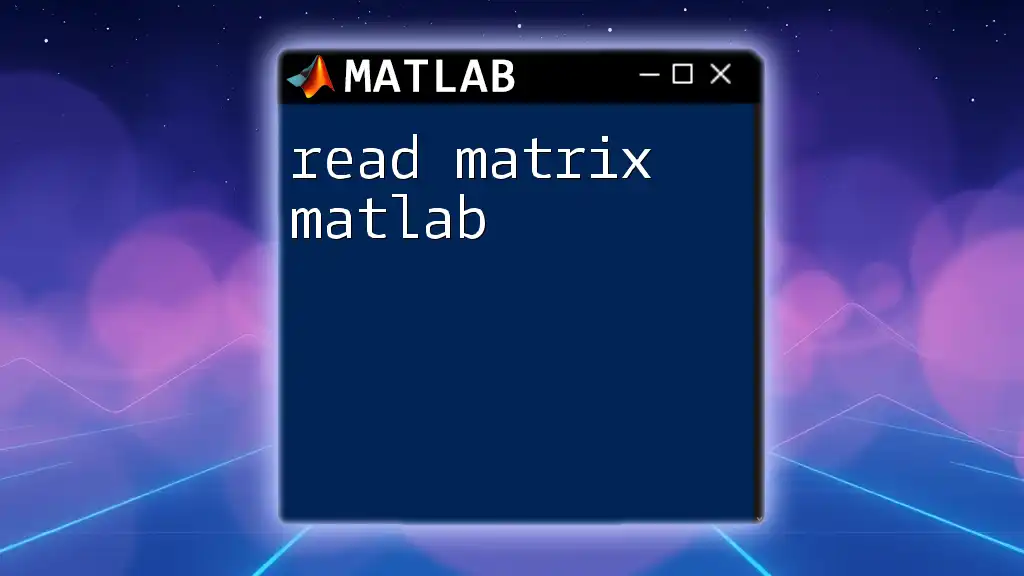
Call to Action
Join our MATLAB community today! Participate in discussions and access resources to improve your proficiency in using MATLAB effectively. Our platform provides invaluable support to individuals eager to learn and master MATLAB commands.