To transpose a matrix in MATLAB, you can use the apostrophe (`'`) operator, which flips the matrix over its diagonal, swapping the row and column indices of each element.
Here's a code snippet demonstrating how to transpose a matrix:
A = [1, 2, 3; 4, 5, 6]; % Original matrix
B = A'; % Transposed matrix
Understanding Matrix Transposition
What is Matrix Transposition?
Matrix transposition is a fundamental operation in linear algebra where the rows of a matrix become columns and the columns become rows. For a matrix \( A \) of dimensions \( m \times n \), its transpose, denoted as \( A' \) or \( \text{transpose}(A) \), will have dimensions \( n \times m \).
For instance, if we transpose a \( 2 \times 3 \) matrix, we end up with a \( 3 \times 2 \) matrix. This simple operation is crucial in many mathematical computations, particularly in solving linear equations and in statistics.
Practical Importance of Transposing Matrices
Transposing matrices is not merely a theoretical exercise; it has numerous practical applications in various fields, including but not limited to:
- Linear Algebra: Many solutions to systems of equations require transposed matrices.
- Statistics: Data analysis often involves rearranging datasets, facilitating computations like covariance and correlation.
- Engineering: In fields like electrical and mechanical engineering, transposed matrices are vital for transformations and modeling physical systems.
The ability to quickly and effectively utilize matrix transposition can greatly enhance data manipulation efficiency.
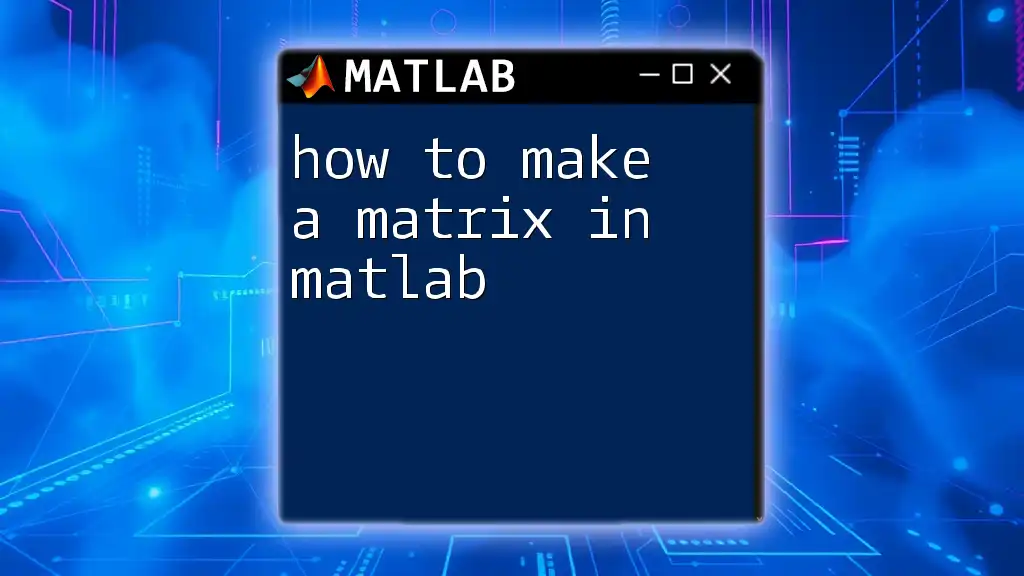
Basic Transposition in MATLAB
Using Apostrophe Operator
In MATLAB, the simplest way to transpose a matrix is by using the apostrophe operator (`'`). This operator efficiently transforms the rows of a matrix into columns and vice versa.
Syntax and Usage
To transpose a matrix using the apostrophe operator, you would do the following:
- Define your matrix.
- Apply the apostrophe operator.
Here’s a quick example:
A = [1 2 3; 4 5 6]; % Define a 2x3 matrix
B = A'; % Transposing matrix A
After running the above code, matrix \( B \) would look like:
B =
1 4
2 5
3 6
Using the `transpose` Function
MATLAB also provides a built-in function called `transpose` for performing the same operation. While the apostrophe operator is common, using the function can sometimes enhance code readability.
Using the `transpose` Function
The usage is similar to the apostrophe operator:
A = [1 2 3; 4 5 6]; % Define a 2x3 matrix
B = transpose(A); % Transposing matrix A
Both methods will yield the same result, but the choice of which to use may depend on personal or team coding standards.
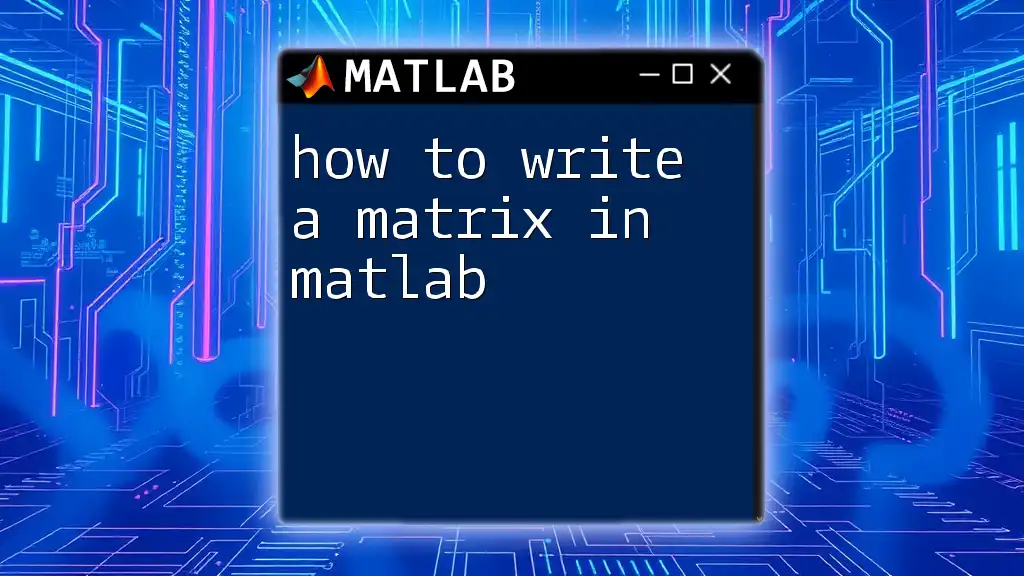
Advanced Transposition Techniques
Complex Numbers and Transposition
When working with complex numbers, it’s essential to understand that transposing a matrix has specific behaviors. By default, using the apostrophe operator will produce the standard transpose. To obtain a conjugate transpose—where complex components are also conjugated—one must use the dot apostrophe operator or the `ctranspose` function.
Key Considerations When Transposing Complex Matrices
Here is an example:
C = [1+1i, 2; 3, 4+2i];
D = C'; % Standard transpose
E = C.'; % Conjugate transpose
In the above code, matrix \( D \) results in a basic transposition, while matrix \( E \) takes the complex numbers into account by conjugating them, which is crucial in many mathematical applications.
Transposing Multidimensional Arrays
MATLAB allows transposing not just 2D matrices but also higher-dimensional arrays. For multidimensional matrices—those with three or more dimensions—transposing does not directly apply in the traditional sense.
Understanding 3D and Higher-Dimensional Matrices
To transpose a 3D matrix, you will need to use the `permute` function, which reorders the dimensions of the matrix according to specified rules. This flexibility is key to advanced data manipulations.
Here’s how you can transpose a 3D array:
F = rand(2, 3, 4); % Create a random 2x3x4 array
G = permute(F, [2, 1, 3]); % Swap the first two dimensions
In this example, the function `permute` allows for a more controlled transposition where you can specify how dimensions should be rearranged.
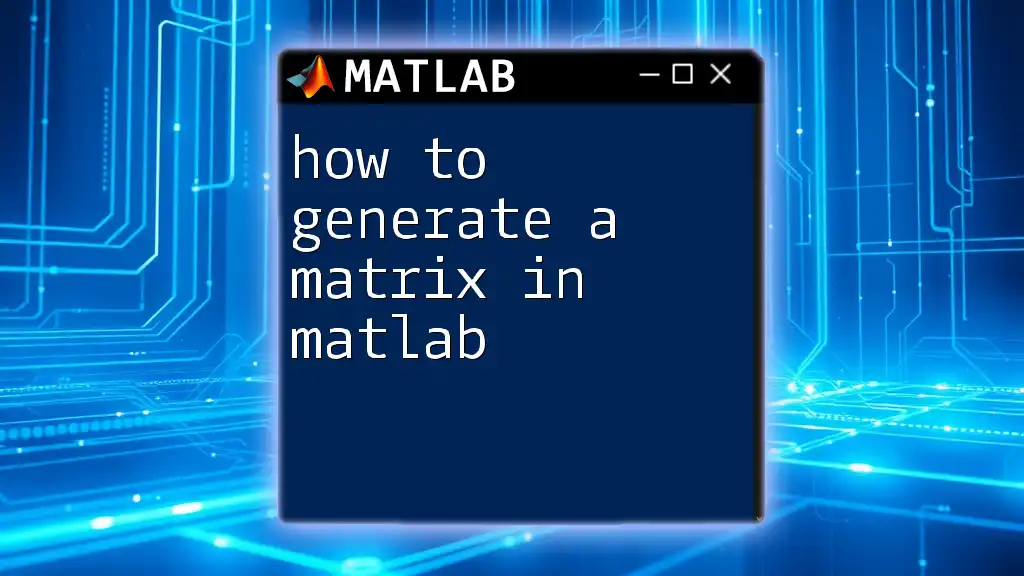
Common Errors and Troubleshooting
Types of Errors When Transposing
When performing matrix transposition in MATLAB, you might encounter some common errors. One of the most frequent issues is dimension mismatches, especially when trying to concatenate matrices after transposing.
For example:
A = [1, 2; 3, 4; 5, 6];
% Trying to concatenate transposed version with the original will cause an error
How to Fix Transposition Errors
To resolve transposition errors, ensure that the dimensions of matrices align correctly for operations like addition or concatenation. Familiarizing yourself with MATLAB’s error messages can significantly aid in debugging.
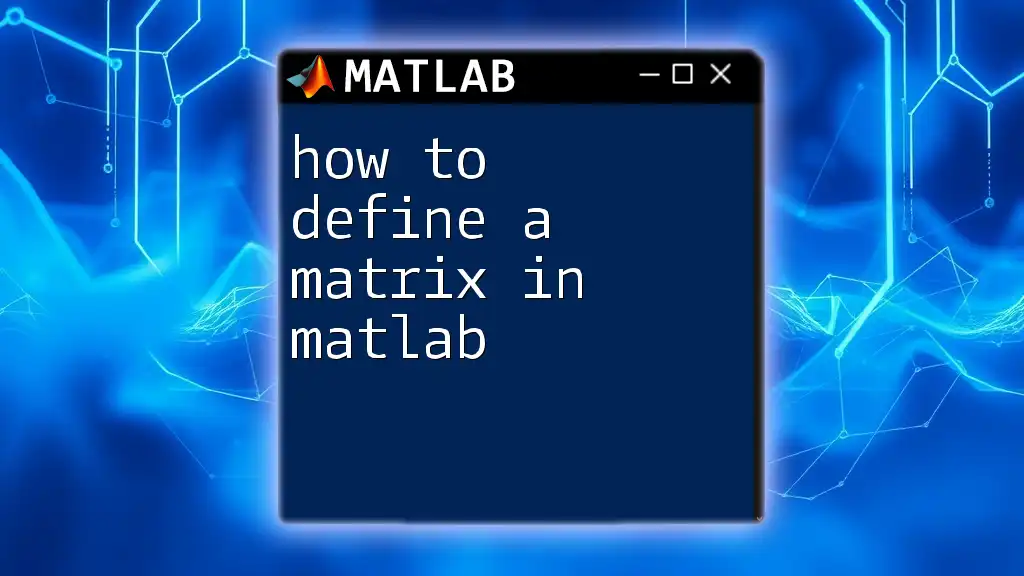
Real-World Applications of Matrix Transposition
Data Analysis and Statistics
Transposing matrices is a crucial step in data analysis. Depending on how data is structured, transposition might be necessary to prepare datasets for statistical evaluation or visualization.
For example, consider the following snippet that demonstrates transposing a dataset:
data = rand(5, 2); % Sample data
transposedData = data'; % Prepare for analysis
Here, `data` might represent features collected over observations, and transposing it organizes the data better for analysis or modeling purposes.
Engineering Applications
In engineering, transposed matrices are often used for system modeling and simulations. For instance, when formulating equations in control systems, transposes may be employed to switch between state-space representations. This application highlights the necessity to know how to transpose matrices effectively in engineering contexts.
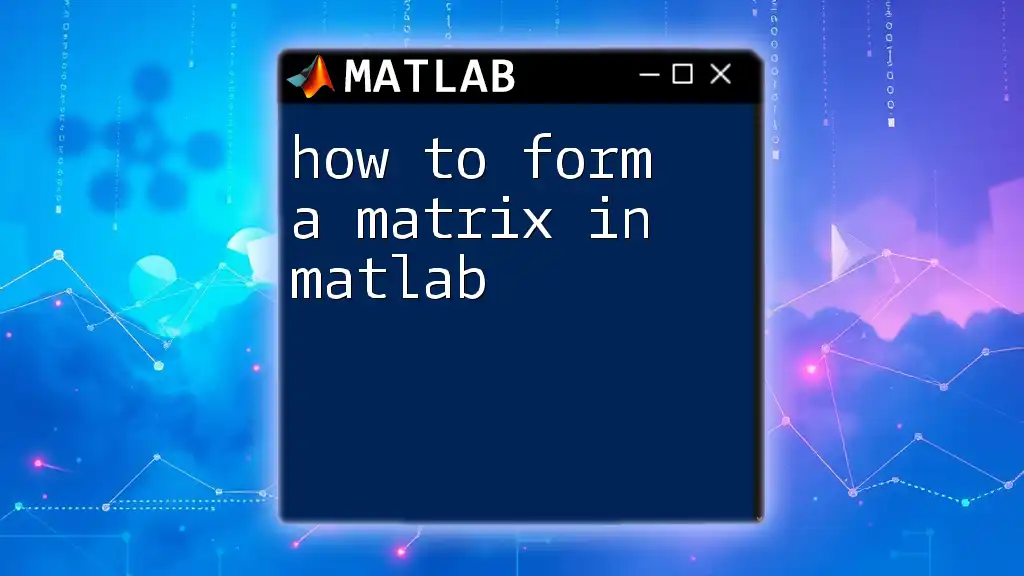
Conclusion
Through this comprehensive exploration of how to transpose a matrix in MATLAB, we have covered everything from basic commands to advanced applications involving complex and multidimensional arrays. Being adept at transposing matrices can enhance your efficiency and effectiveness in MATLAB programming.
Practice is vital, so experiment with different types of matrices and observe how transposing changes the structure and usefulness of your data. Feel empowered to reach out to your community for collaborative learning and improvement!
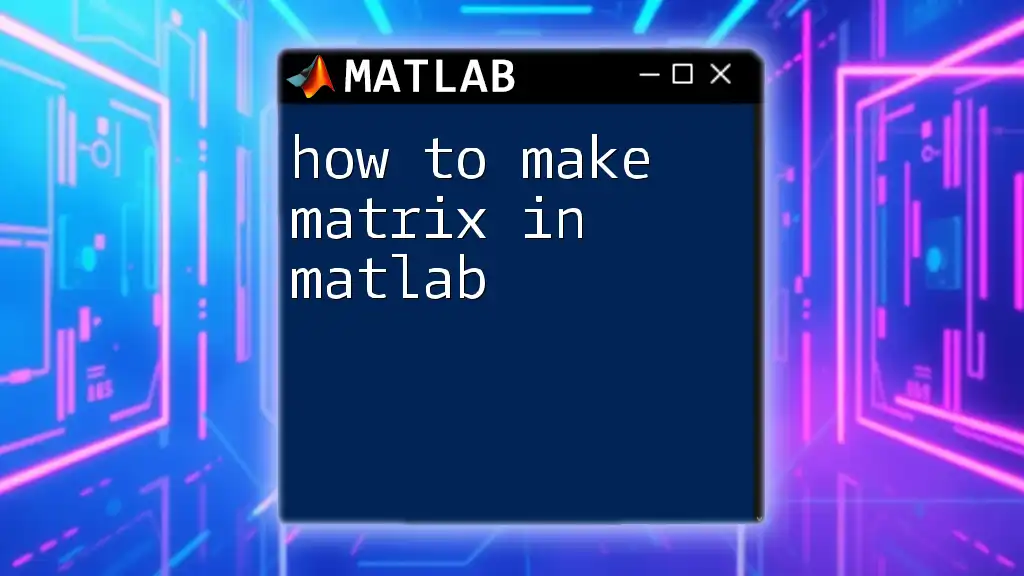
Additional Resources
Further Reading and Learning
To deepen your knowledge of MATLAB and matrix operations, consider exploring textbooks, online courses, and the extensive resources available in the official MATLAB documentation and community forums.
Sample Exercises
Try the following exercises to reinforce your understanding:
-
Transpose the following matrix:
X = [1, 2, 3; 4, 5, 6; 7, 8, 9];
-
Create a complex matrix, transpose it, and compare results with its conjugate transpose.
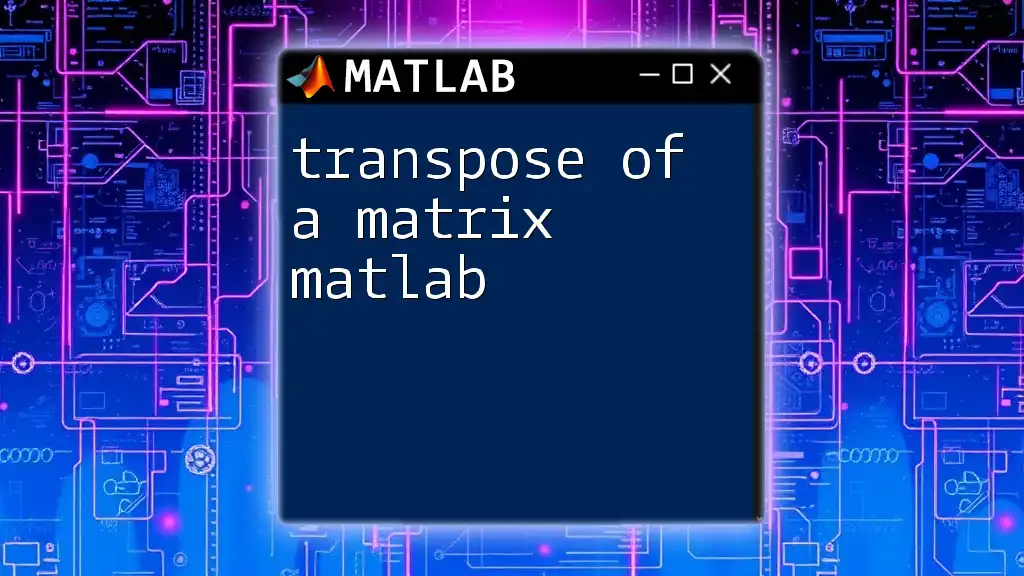
FAQs
What is the difference between a transpose and a conjugate transpose?
The standard transpose switches rows and columns, while the conjugate transpose also takes the complex conjugate of each element, which is crucial when working with complex numbers.
Can I transpose an empty matrix in MATLAB?
Yes, an empty matrix can be transposed without any issue. The result will still be an empty matrix.
Is matrix transposition computationally expensive?
In most cases, matrix transposition is efficient in MATLAB, but the computational load can increase with the size of the matrix, particularly in very large datasets.