The determinant of a matrix in MATLAB can be easily calculated using the `det` function, which takes the matrix as an input parameter.
A = [1 2; 3 4]; % Example matrix
d = det(A); % Calculate the determinant
disp(d); % Display the result
Overview of Determinants in Mathematics
What is a Determinant?
A determinant is a scalar value that can be computed from the elements of a square matrix. It is a crucial concept in linear algebra, encapsulating information about the matrix's properties. The determinant provides insights into the matrix's invertibility, eigenvalues, and geometric interpretations.
For instance, the determinant can help us determine whether a system of linear equations has a unique solution. If the determinant of a matrix is zero, the matrix is singular, meaning it does not have an inverse, and the equations are either dependent or inconsistent.
The Role of Determinants in Matrix Theory
Determinants play an essential role in matrix theory. They relate to several properties:
- Invertibility: A matrix is invertible, meaning it has an inverse, if and only if its determinant is non-zero.
- Eigenvalues: Determinants can help calculate eigenvalues of a matrix, which are critical in understanding the matrix's behavior.
The applications of determinants span various fields, including engineering, physics, and computer science, where linear transformations and systems of equations are prevalent.
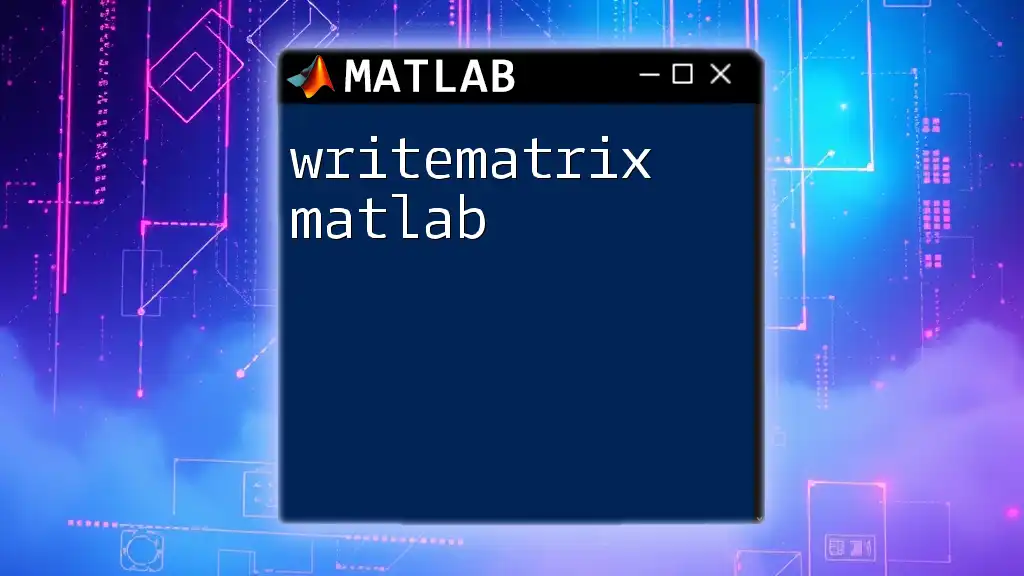
Getting Started with MATLAB
Introduction to MATLAB
MATLAB is a high-performance programming language and environment designed for technical computing. It is widely favored for its ability to perform operations on matrices and arrays efficiently, making it a perfect choice for computations involving determinants.
Setting Up Your Environment
Before you can compute determinants in MATLAB, you'll need to set up your environment:
- Installation: Install MATLAB from the MathWorks website, following the instructions provided.
- Overview of the Interface: Familiarize yourself with the Command Window, Editor, and Workspace. Knowing where to find tools and commands will enhance your learning experience.
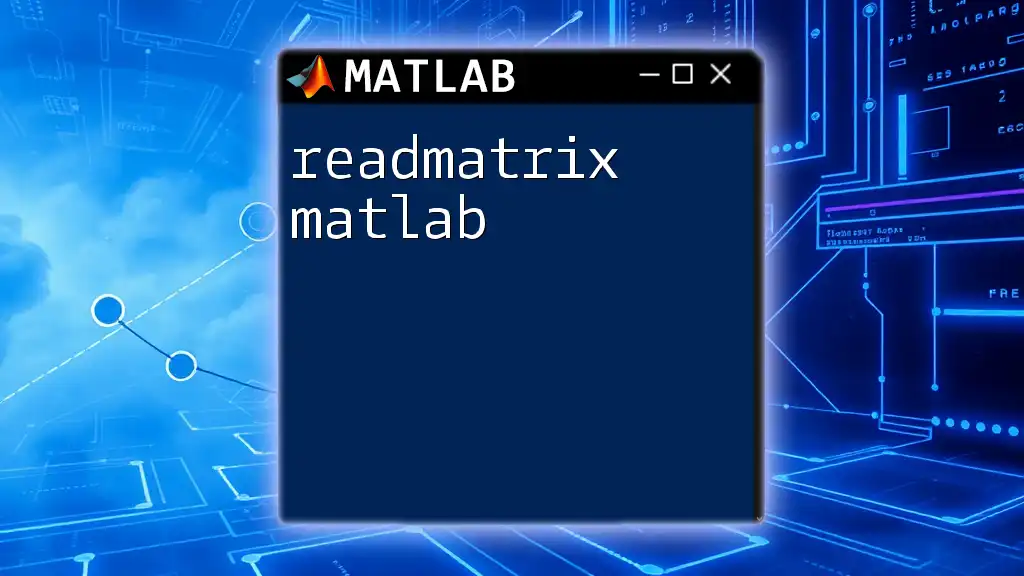
Computing the Determinant of a Matrix in MATLAB
Syntax of the Det Function
In MATLAB, you can compute the determinant of a matrix using the `det` function. The most basic syntax is:
det(A)
Where `A` is the square matrix for which you want to calculate the determinant.
Example: Simple 2x2 Matrix
Let's start with a simple example involving a 2x2 matrix. Consider the following code snippet:
A = [2, 3; 1, 4];
result = det(A);
fprintf('The determinant of A is: %f\n', result);
When you run this code, MATLAB computes the determinant of the matrix `A`. The output will be `The determinant of A is: 5.000000`. This determinant signifies that the matrix is invertible.
Example: Larger Matrices
Now, let's compute the determinant of a 3x3 matrix. Here’s the code:
B = [1, 2, 3; 0, 1, 4; 5, 6, 0];
resultB = det(B);
fprintf('The determinant of B is: %f\n', resultB);
Upon executing this code, MATLAB will calculate and display the determinant of matrix `B`, which will show you how larger matrices behave differently than smaller ones.
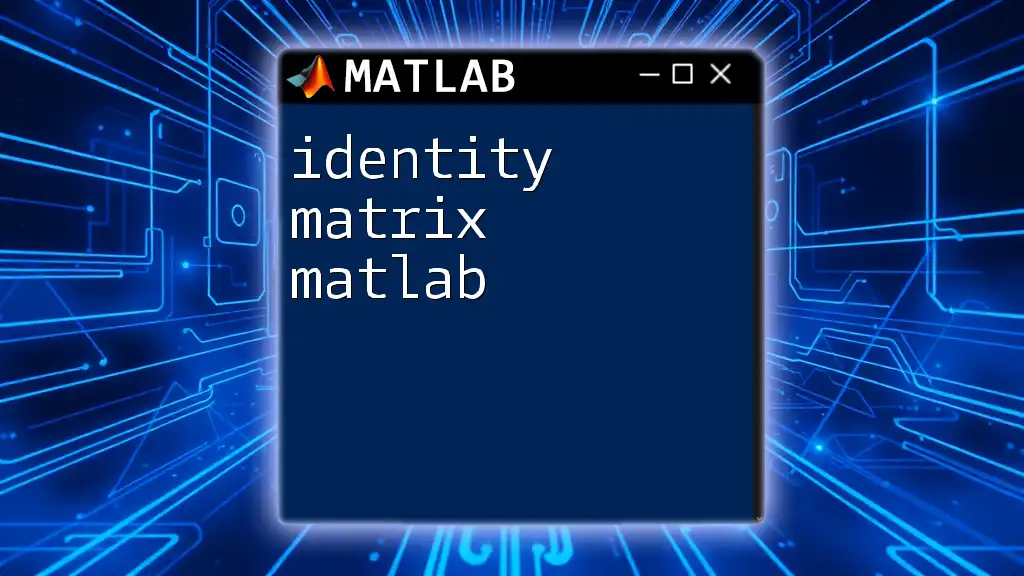
Understanding Determinants with Examples
Computing Determinants of Different Types of Matrices
Understanding how determinants function within various types of matrices is crucial. Here are some common cases:
- Upper Triangular Matrix: Its determinant is simply the product of its diagonal elements. For example:
C = [1, 2, 3; 0, 4, 5; 0, 0, 6];
detC = det(C);
fprintf('The determinant of C is: %f\n', detC);
- Diagonal Matrix: Similar to the upper triangular matrix, the determinant is the product of the diagonal elements.
D = [2, 0, 0; 0, 3, 0; 0, 0, 4];
detD = det(D);
fprintf('The determinant of D is: %f\n', detD);
- Singular Matrix: If a matrix is singular (e.g., two rows are multiples), its determinant will be zero.
E = [1, 2; 2, 4];
detE = det(E);
fprintf('The determinant of E is: %f\n', detE);
In this case, the output will indicate that the matrix `E` is singular.
Geometric Interpretation of the Determinant
The determinant has a profound geometric interpretation: the absolute value of the determinant represents the volume of the parallelepiped formed by the column vectors of the matrix. A determinant of zero suggests that the vectors are coplanar (they do not span a three-dimensional space).
You can visualize this concept using MATLAB plots. For instance, a code snippet could illustrate the transformation of geometric shapes when the matrix is applied.
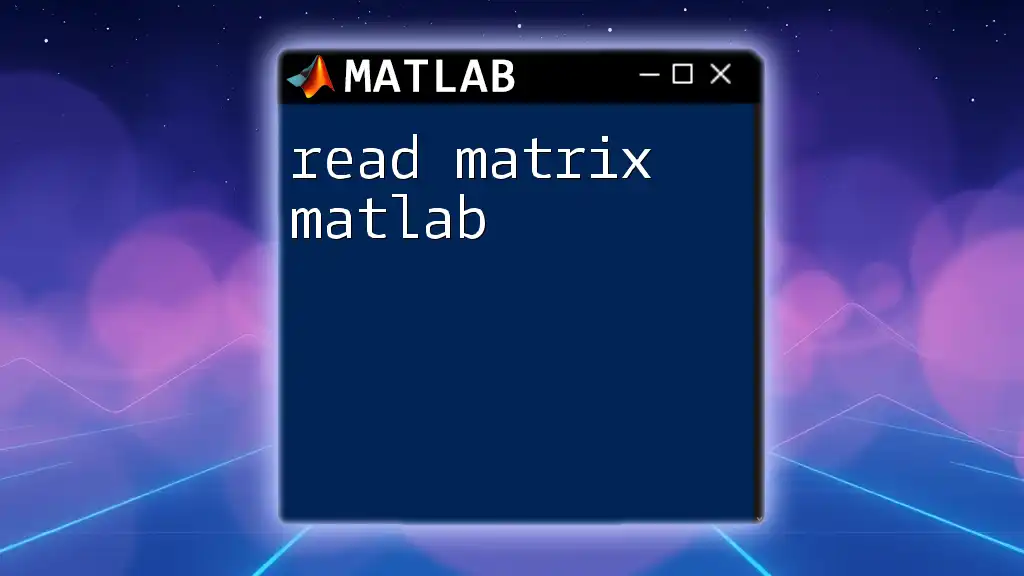
Advanced Topics
Properties of Determinants
The properties of determinants are vital for matrix computations:
- Row Operations: Swapping two rows changes the sign of the determinant. Multiplying a row by a scalar results in the determinant being multiplied by that scalar.
- Matrix Inverse: The determinant of the inverse of a matrix is the reciprocal of the original determinant, provided the original is non-zero.
Determinant and Matrix Factorizations
Understanding how determinants relate to factorizations is key in numerical methods. For example, using LU decomposition, you can calculate:
[L, U, P] = lu(F); % where F is your matrix
detF = det(L) * det(U); % if L is lower triangular and U is upper triangular
This illustrates how determinant calculations can be integrated into more complex matrix manipulations.
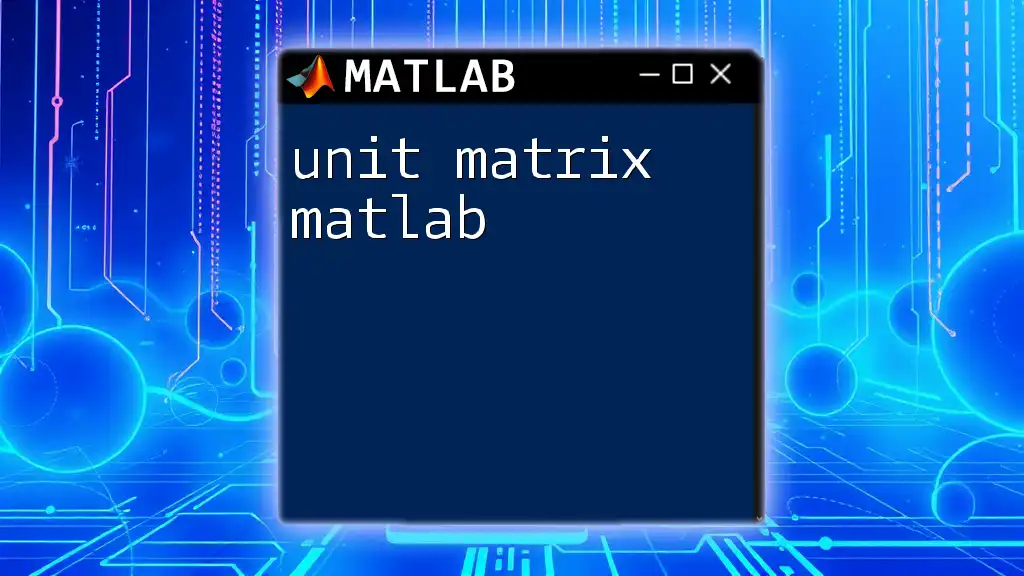
Troubleshooting Common Issues
Common Errors When Calculating Determinants
When using the `det` function, you may encounter various errors. Here are a few common mistakes:
- Trying to compute the determinant of a non-square matrix will result in an error. Always check the dimensions of your matrix.
- Using an empty matrix also yields an error. Ensure your matrix has valid entries.
Performance Issues
Calculating determinants for large matrices can become computationally intensive. To optimize performance:
- Consider using matrix factorizations.
- Use built-in functions that are optimized for computational efficiency in MATLAB.
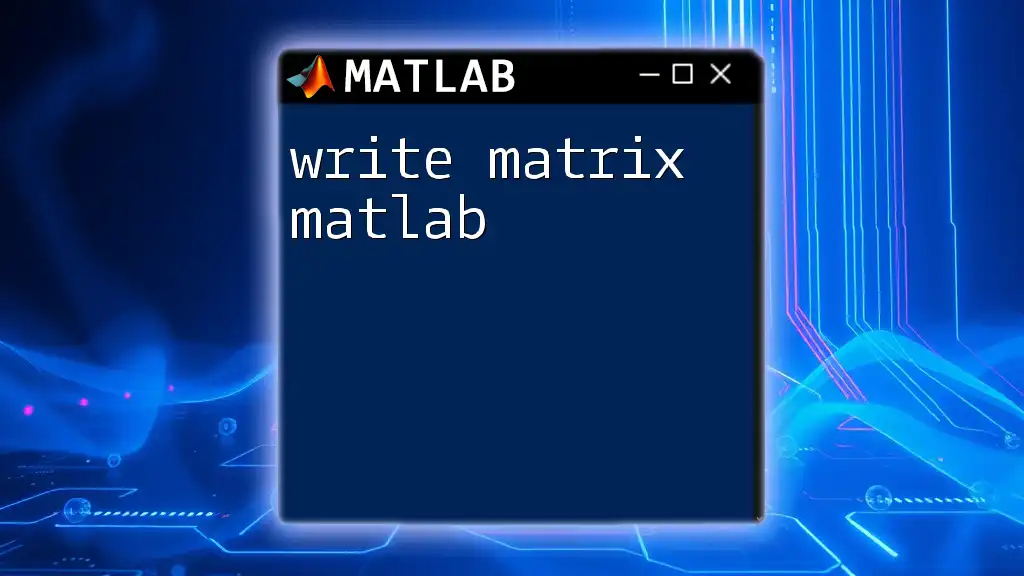
Conclusion
Summary of Key Points
Throughout this guide, we explored the concept of the determinant, its calculation in MATLAB, and its geometric significance. Understanding determinants is invaluable in various mathematical and engineering domains, as they provide critical information about matrix behaviors.
Further Resources
For those seeking to expand their knowledge, the official MATLAB documentation is an excellent starting point. Consider exploring textbooks focusing on linear algebra and numerical methods for additional insights.
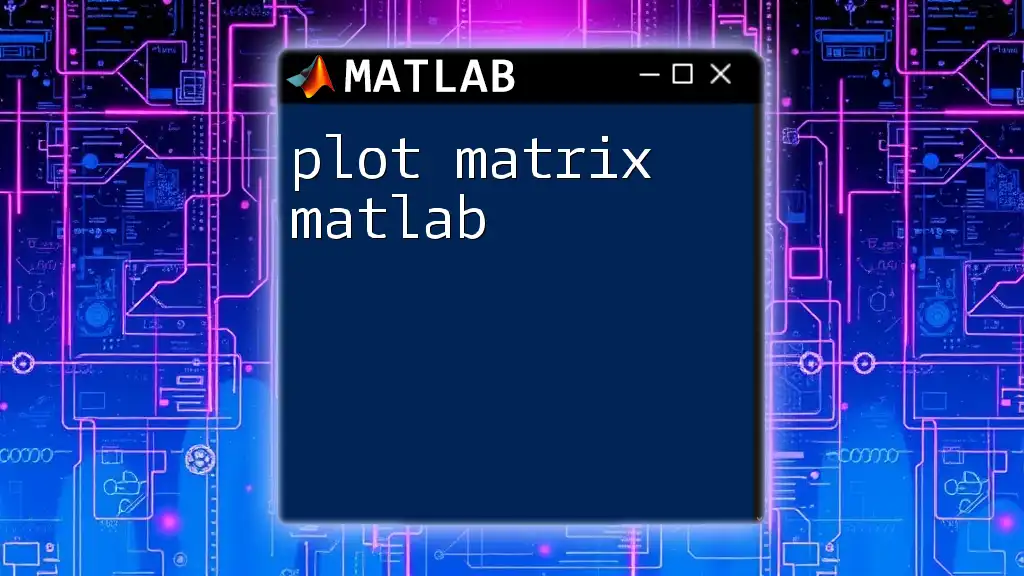
Call to Action
Dive into MATLAB and try calculating the determinants of various matrices yourself! Share your experiences or any questions you have in the comments below. Your journey with matrices and determinants is just beginning!