To write a function in MATLAB, you define it using the `function` keyword followed by the output variable(s), the function name, and the input variable(s) in a specific format.
Here's a simple example of a MATLAB function that adds two numbers:
function result = addNumbers(a, b)
result = a + b;
end
Understanding the Basics of Functions in MATLAB
What is a Function?
A function in MATLAB is a block of code that performs a specific task and can accept input arguments and return output arguments. Functions help to encapsulate pieces of logic, making your code modular and easier to maintain. By using functions, you can label complex operations with a name, improving the clarity of your code.
Why Use Functions?
Using functions brings several benefits:
- Code Reusability: You can define a function once and call it whenever needed, saving both time and effort in coding.
- Improved Readability: Well-named functions can make your code much easier to understand at a glance.
- Easier Debugging: If a function doesn’t work as expected, you can isolate it from the rest of the code, making it easier to identify issues.
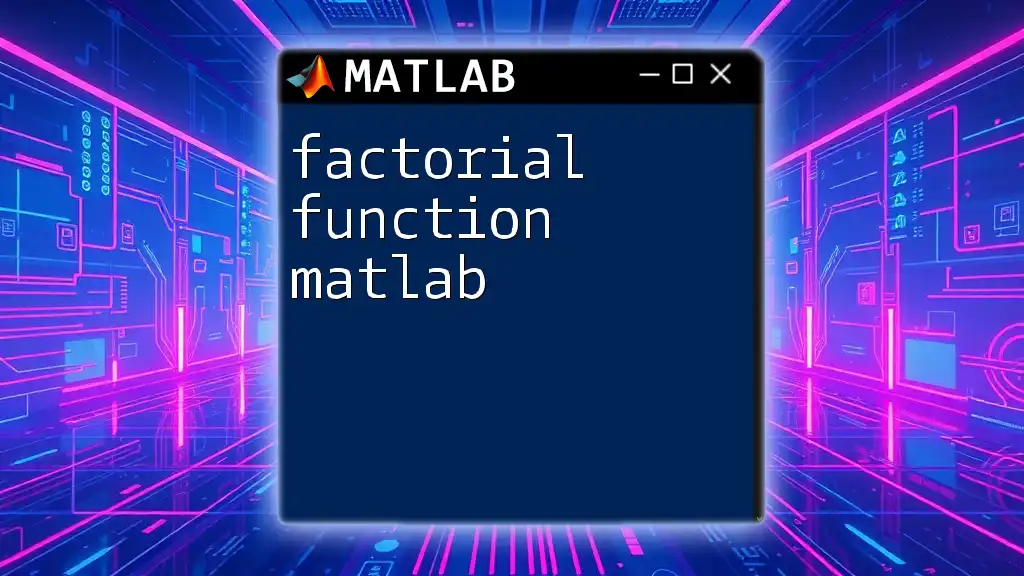
Creating Your First Function
Setting Up the Function File
To create a function, you need to create a separate file that adheres to some naming rules. The filename must match the function name, and it should have a `.m` extension. For example, if you’re creating a function named `myFirstFunction`, you should name your file `myFirstFunction.m`.
Syntax of a Function
The basic syntax for creating a function in MATLAB is:
function [output] = functionName(input)
- function: The keyword that tells MATLAB you are defining a function.
- output: This can be one output or multiple outputs enclosed in square brackets.
- functionName: The name of your function, which should reflect its purpose.
- input: Variables that you pass into your function.
Writing Simple Functions
Let’s write a simple function to add two numbers. You can create a new file `addNumbers.m` and insert the following code:
function sum = addNumbers(a, b)
sum = a + b;
end
In this example, `a` and `b` are input parameters, and the function returns the result of their addition stored in `sum`.
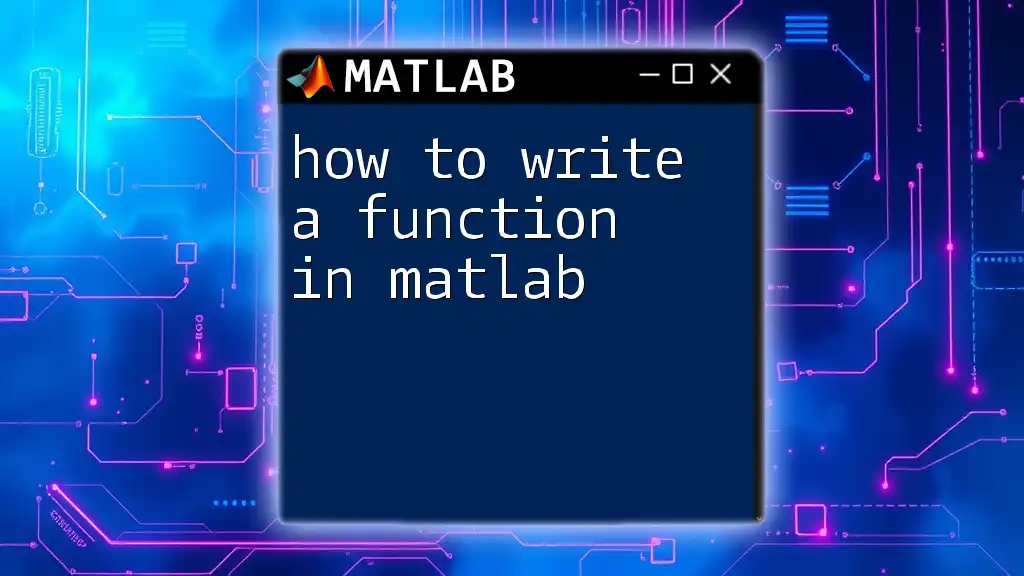
Function Inputs and Outputs
Input Arguments
You can pass various types of inputs to functions, including scalars, vectors, and matrices. When writing functions, ensure you handle different types of input elegantly.
Output Arguments
MATLAB functions can return multiple outputs. To do this, you enclose the output variables in square brackets. For example, you can create a function that calculates both the sum and the difference of two numbers as shown below:
function [sum, difference] = calculate(a, b)
sum = a + b;
difference = a - b;
end
In this case, calling `calculate(5, 3)` will provide both a sum of 8 and a difference of 2.
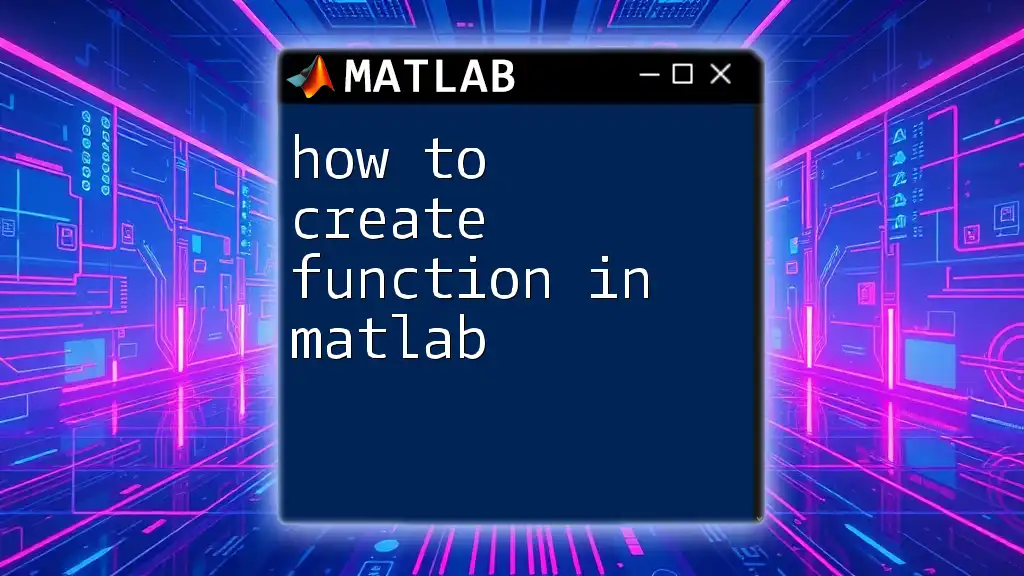
Function Overloading
What is Function Overloading?
Function overloading allows you to define multiple functions with the same name but different behaviors based on their input types or the number of inputs. This feature makes it easier to handle various data types without having to create uniquely named functions for each case.
Implementing Function Overloading
You can implement function overloading by checking the input type within a function. Below is an example of a function that calculates the square of a number, but also validates the input type:
function y = square(x)
if isnumeric(x)
y = x^2;
else
error('Input must be a number');
end
end
This approach allows the same function name to be used, simplifying your code structure.
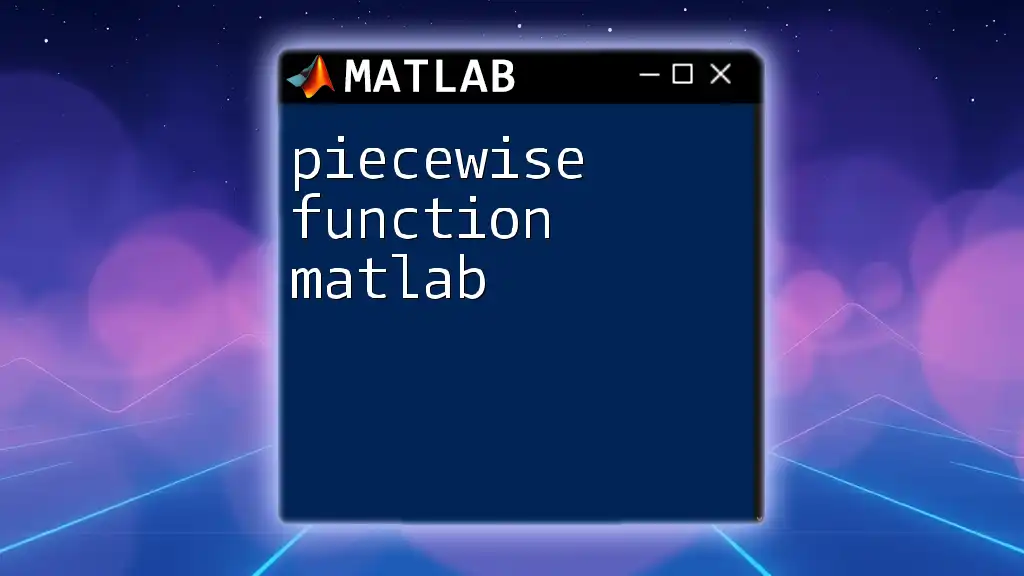
Scope and Persistence of Variables
Local vs Global Variables
Variables defined inside a function have local scope, meaning they cannot be accessed outside that function. In contrast, global variables are available across all functions. Use global variables cautiously, as they can lead to unexpected behaviors if mismanaged.
Using `global` Keyword
To use a global variable, declare it in both the main workspace and within your function. For instance:
global myGlobalVar;
myGlobalVar = 10; % declaring global variable
This will allow you to access `myGlobalVar` from any function that includes the `global` declaration.
Persistent Variables
Persistent variables keep their value between function calls. They are initialized only once during the function workflow. This can be particularly useful for maintaining state without making the variable globally available.
Here’s an example using a persistent variable:
function count = myCounter()
persistent c;
if isempty(c)
c = 0;
end
c = c + 1;
count = c;
end
In this case, every time `myCounter` is called, it increments the value of `c` without resetting it.
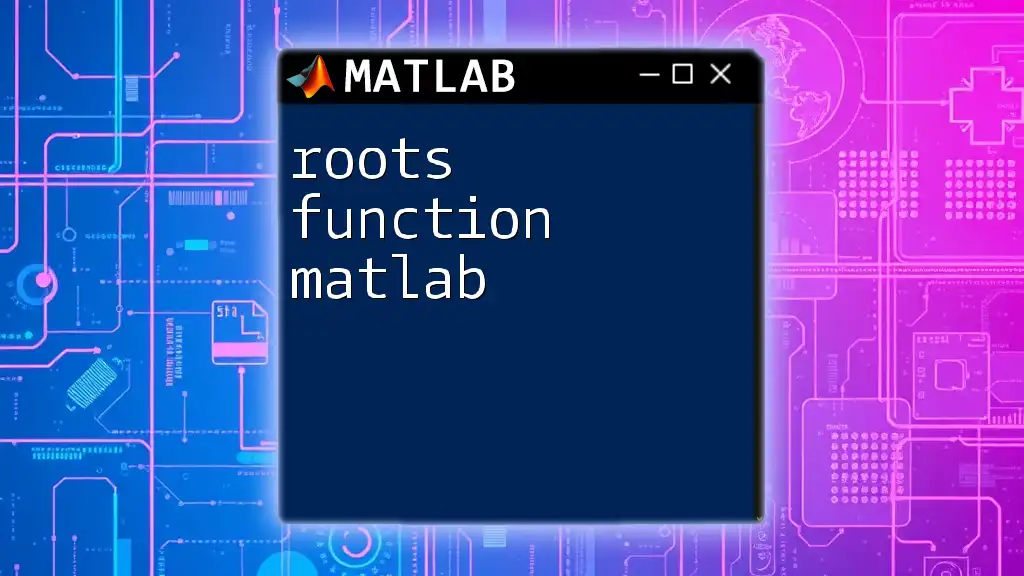
Advanced Function Topics
Anonymous Functions
Anonymous functions are one-liners that allow defining simple functions without creating a separate file. They are particularly useful for quick calculations. Here’s a quick example:
f = @(x) x.^2; % anonymous function to square a number
y = f(5); % y will be 25
They can simplify code snippets, especially when passing functions as arguments.
Nested Functions
Nested functions are functions defined within another function. They have access to the parent function’s variables, making them useful for organizing related tasks in functional layers.
Here's how to create a nested function:
function outerFunction()
function innerFunction()
disp('This is a nested function.');
end
innerFunction(); % calling the nested function
end
Function Handles
A function handle is a MATLAB data type that represents a function. Function handles are incredibly powerful when passing functions to other functions or creating callback functions. You can create a function handle using the `@` symbol:
handle = @addNumbers; % creating a function handle for addNumbers
result = handle(2, 3); % result will be 5
This flexibility makes it easy to reference functions without needing to know the full context in which they operate.
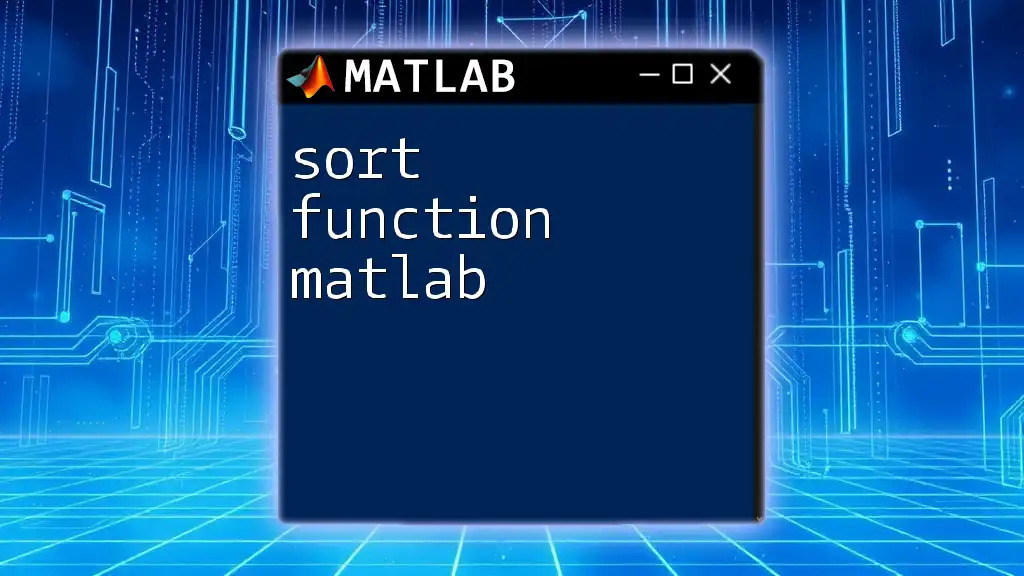
Debugging Functions in MATLAB
Common Errors and Debugging Tips
When writing functions, several common issues may arise, such as incorrect input types, undefined variables, or logical errors. Debugging is an important skill for any MATLAB user.
To troubleshoot your functions, consider using breakpoints in the MATLAB editor. This allows you to pause execution and inspect variable values. Additionally, using the `disp` function can help trace output values during execution, making it easier to follow the flow of your code.
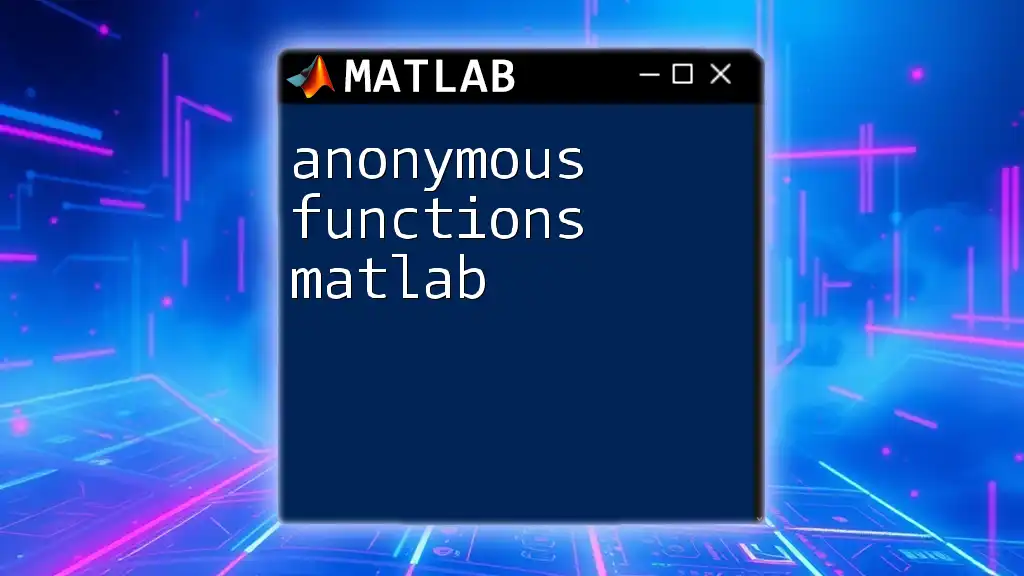
Conclusion
Understanding how to write functions in MATLAB greatly enhances your programming capabilities. By modularizing your code into functions, you not only simplify coding but also help others understand and utilize your code effectively.
Practice writing various functions as described in this guide to master the art of MATLAB programming. As you develop your skills, consider sharing your experiences or questions in the comments section. Stay tuned for more tutorials that will elevate your MATLAB expertise!