CO2 modeling in MATLAB involves simulating the dynamics of carbon dioxide concentration using differential equations to represent interactions within the atmosphere and ecosystem; here’s a simple example of simulating a basic exponential growth model for CO2 levels:
% Simple CO2 growth model
t = 0:0.1:50; % time from 0 to 50 years
CO2_initial = 400; % initial CO2 concentration in ppm
growth_rate = 0.02; % growth rate per year
CO2_levels = CO2_initial * exp(growth_rate * t); % CO2 concentration over time
plot(t, CO2_levels);
title('CO2 Concentration Over Time');
xlabel('Time (years)');
ylabel('CO2 Concentration (ppm)');
grid on;
Understanding CO2 Modelling
What is CO2 Modelling?
CO2 modelling refers to the simulation and analysis of carbon dioxide levels in the atmosphere, emphasizing their sources, sinks, and overall impact on climate change. This practice is essential for climate scientists and environmentalists who aim to understand and mitigate the effects of greenhouse gases. By identifying trends and making projections, policymakers and researchers can make informed decisions.
Key Concepts in CO2 Modelling
Carbon Cycle: The carbon cycle is the process through which carbon is exchanged among the Earth's atmosphere, oceans, soil, and living organisms. Understanding this cycle is vital when modelling CO2, as it helps in identifying how carbon is sequestered or released back into the atmosphere through natural and anthropogenic processes.
Greenhouse Gases: CO2 plays a significant role as a greenhouse gas, affecting Earth's temperature. Other gases, like methane (CH4) and nitrous oxide (N2O), also contribute significantly to warming. Understanding their interactions is key in creating an effective CO2 model.
Modeling Objectives: The primary objective of CO2 modelling could vary from assessing a region's carbon footprint to analyzing long-term trends in global emissions. Defining a clear goal will guide the modelling process and methodologies.
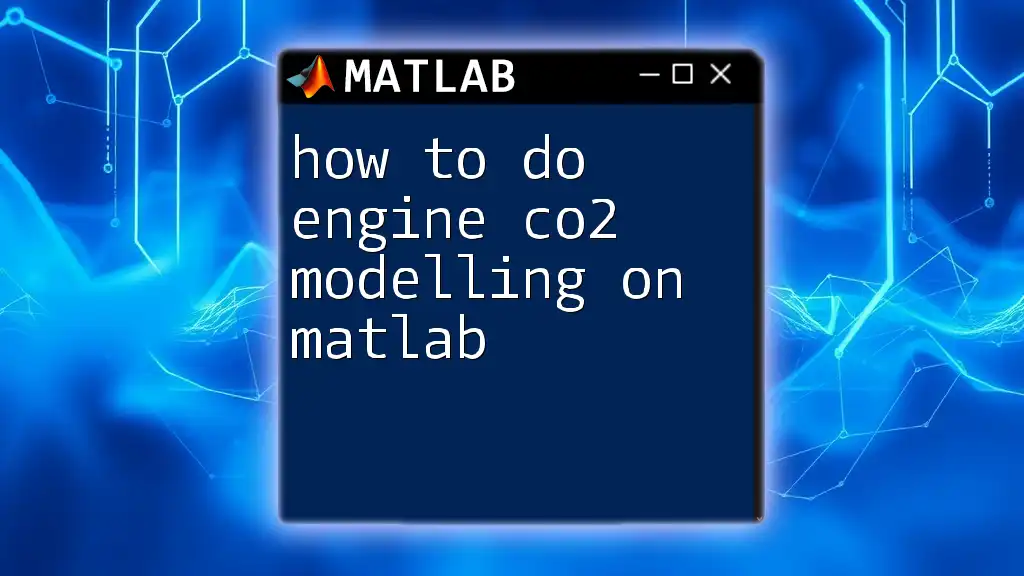
Getting Started with MATLAB
Setting Up MATLAB for CO2 Modelling
To start modeling CO2 in MATLAB, ensure that you have the appropriate version of MATLAB installed along with the necessary toolboxes. The Statistics and Machine Learning Toolbox is particularly crucial, as it facilitates various analyses and predictive modelling techniques, while the Curve Fitting Toolbox can be used to fit diverse mathematical models to your data.
Basic MATLAB Commands for Beginners
Before diving into modelling, familiarize yourself with some basic commands. The following commands will help to set up your workspace correctly:
clear; clc; close all;
- `clear`: Removes all variables from the workspace.
- `clc`: Clears the Command Window.
- `close all`: Closes all open figures.
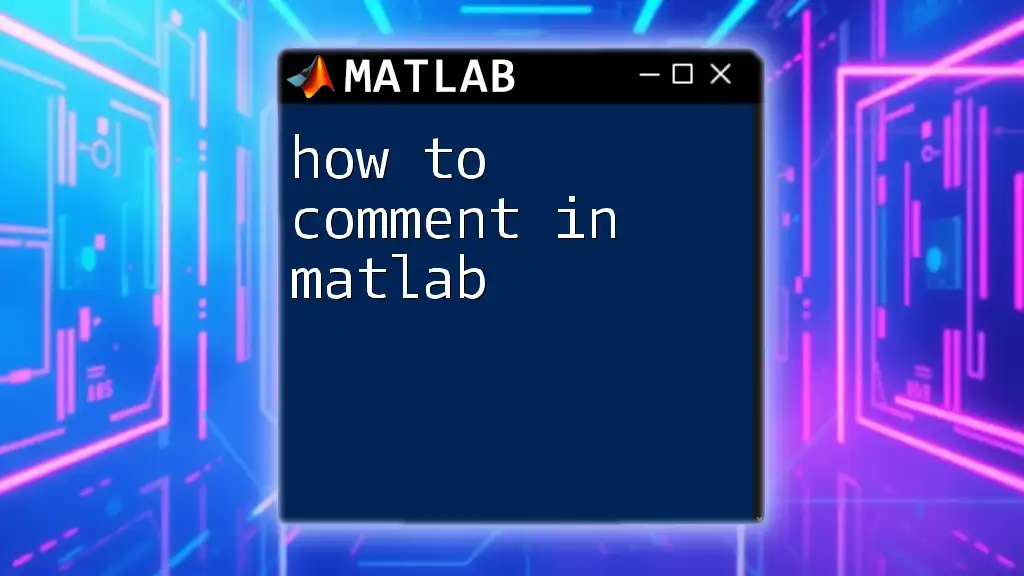
CO2 Data Acquisition and Preparation
Sources of CO2 Data
CO2 data can be sourced from various locations and methods. Researchers often use satellite data, which provides a broad perspective on atmospheric gas concentrations, and data from ground stations that offer localized information. Open-source databases, like NOAA's Global Monitoring Laboratory, provide accessible datasets for researchers.
Importing Data into MATLAB
After identifying your data source, the next step is importing the data into MATLAB. Different file formats can be used. For instance, if you are using a CSV file, you can easily read it with:
data = readtable('co2_data.csv');
Here, `readtable` imports the CSV file into a table, a versatile data structure in MATLAB.
Data Preprocessing
Once the data is imported, it often needs preprocessing to enhance its quality. This can include handling missing values or normalizing the data.
A common step is to remove rows with NaN values:
% Remove rows with NaN values
data = rmmissing(data);
Preprocessing ensures that subsequent analyses and modelling are based on accurate and complete data.
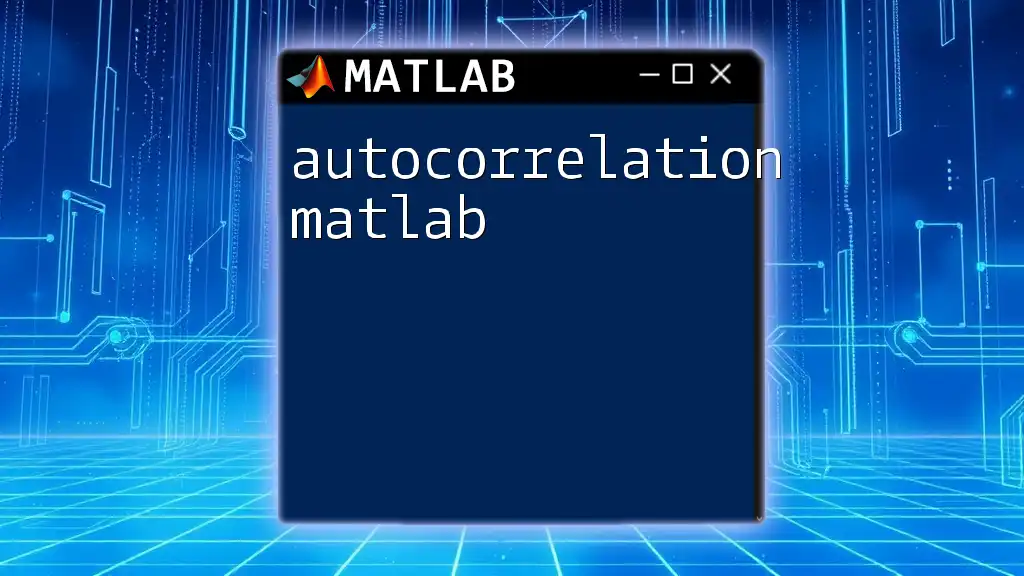
Developing the CO2 Model
Choosing the Right Model
Selecting the appropriate model is crucial. Common options include:
- Simple Linear Regression: Best for identifying straightforward trends.
- Exponential Growth Models: Useful when projecting future emissions based on past trends.
When choosing a model, consider factors that influence CO2 levels, such as seasonal variations and human activities.
Implementing the Model in MATLAB
Setting Up a Simple Linear Regression
For establishing a basic CO2 model, simple linear regression can serve as a starting point. This model can identify relationships between CO2 levels and time, represented as follows:
% Linear regression example
x = data.Year; % Independent variable: Year
y = data.CO2; % Dependent variable: CO2 Levels
model = fit(x, y, 'poly1'); % Fit a linear model
The `fit` function creates a linear fit for the data, setting the foundation for your model.
Testing Model Fit
Post fitting, it’s crucial to assess the model's performance. Common metrics include R-squared and Root Mean Square Error (RMSE). You can evaluate your model using:
y_pred = model(x); % Predicted values from the model
residuals = y - y_pred; % Differences between actual and predicted values
RMSE = sqrt(mean(residuals.^2)); % Calculate RMSE
This calculation helps in understanding how well the model represents the observed values.
Visualizing the Model
Visualization is a powerful tool in data analysis. Plotting CO2 levels against time not only illustrates trends but also helps in identifying anomalies. The following code generates a straightforward plot:
figure;
plot(x, y, 'o', x, y_pred, '-r');
xlabel('Year');
ylabel('CO2 Levels (ppm)');
title('CO2 Levels Over Time');
legend('Observed', 'Predicted');
This plot provides a visual comparison between the observed data and what the model predicts, aiding the interpretation of your results.
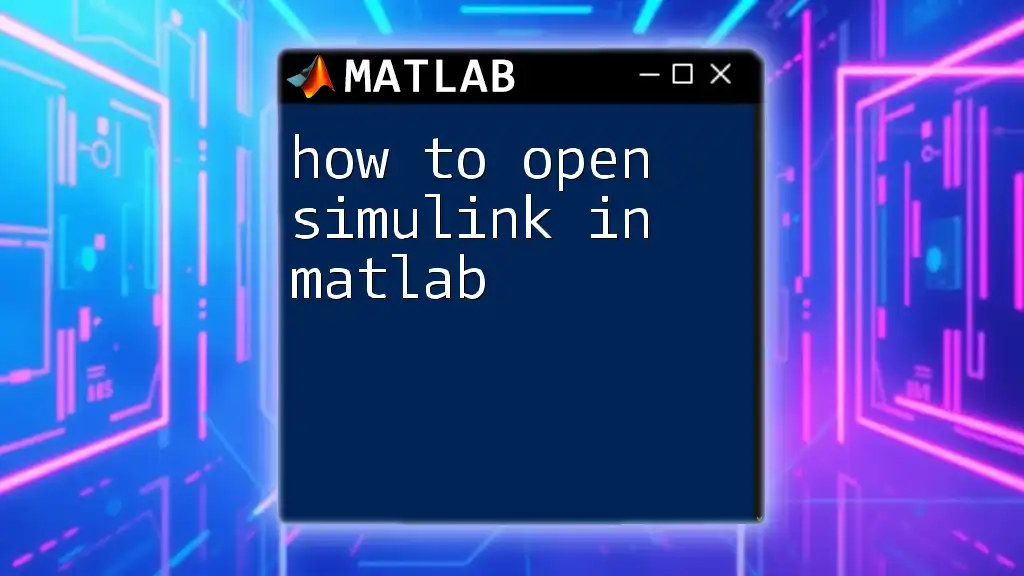
Advanced Modelling Techniques
Incorporating Seasonal Variability
When modelling CO2 levels, it’s essential to account for seasonal patterns since CO2 concentrations fluctuate throughout the year. Methods such as adding sinusoidal terms to your model can effectively capture these seasonal effects. Adjusting your approach to include factors like plant growth cycles can lead to more accurate predictions.
Machine Learning Approaches
For more complex relations, machine learning techniques provide advanced options. Neural networks, decision trees, and other algorithms can capture non-linearities in the data. A simple example of implementing a neural network in MATLAB can be done as follows:
inputs = data(:,1:end-1); % Features
targets = data.CO2; % Target variable
net = feedforwardnet(10); % Create a neural network with 10 hidden neurons
This neural network can then be trained using your CO2 data, allowing for more adaptive learning and potential accuracy improvements.
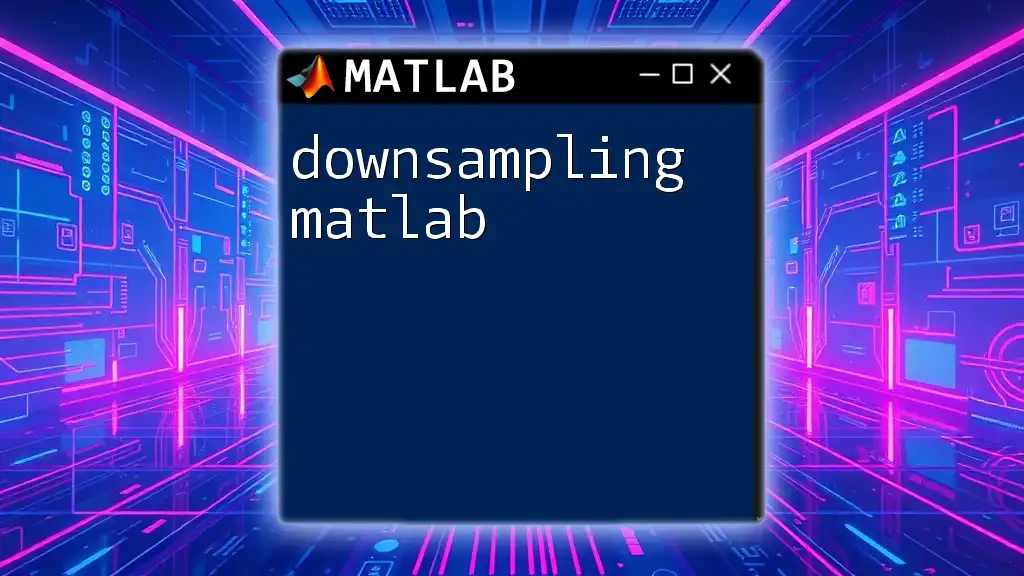
Conclusion
In conclusion, mastering how to do CO2 modelling on MATLAB involves understanding the intricacies of CO2 data, selecting appropriate models, and conducting thorough analysis and visualization. With the growing importance of environmental data, your ability to model CO2 dynamics will contribute significantly to achieving sustainable environmental goals.
Further Resources
Explore MATLAB's documentation and community forums for additional insights and troubleshooting tips. Numerous books and online courses are also available, offering deeper dives into CO2 modelling and MATLAB proficiency.
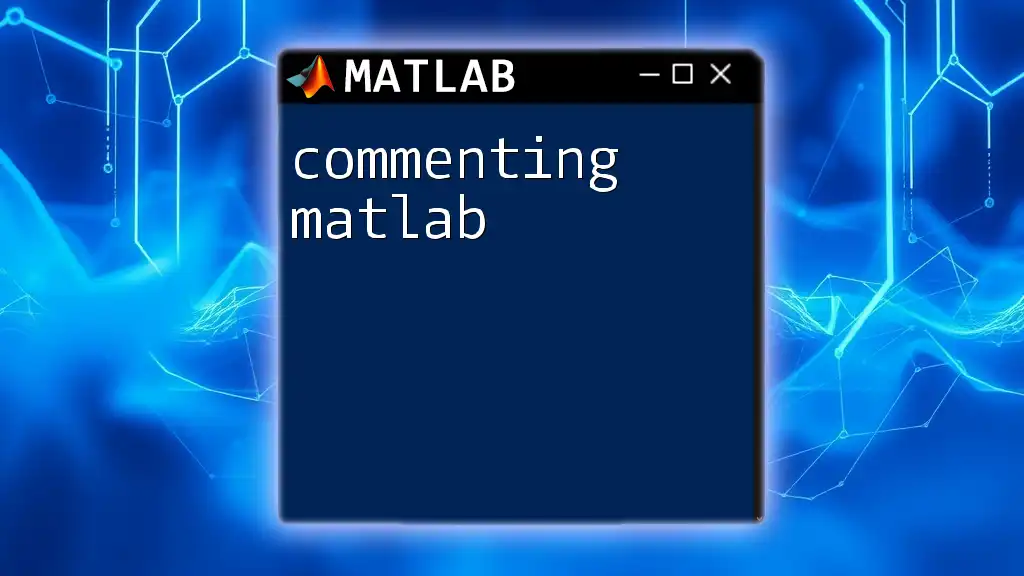
Call to Action
To enhance your skills further, consider joining our upcoming webinar on CO2 modelling in MATLAB, where hands-on examples will guide you through advanced techniques and applications. Dive into our platform for more tutorials and practical exercises designed to boost your understanding and expertise!