Commenting in MATLAB is crucial for providing explanations or notes within the code without affecting its execution, and it can be done using the percent sign (`%`) for single-line comments or the `%%` for section breaks.
Here’s a code snippet demonstrating comments in MATLAB:
% This is a single-line comment
x = 5; % Assigning the value 5 to variable x
%% This is a section header
y = x^2; % Calculating the square of x
Understanding Comments in MATLAB
What are Comments?
Comments in MATLAB are text annotations that help explain what certain parts of the code do. They are entirely ignored by the MATLAB interpreter and serve to improve the readability and maintainability of your code. Commenting in MATLAB allows programmers to provide insight and context that might not be immediately apparent from the code itself.
Types of Comments in MATLAB
MATLAB supports two primary types of comments:
-
Single-line Comments: These comments start with a percentage sign (`%`). Any text following the `%` on that line will be treated as a comment.
-
Multi-line Comments: This type of comment begins with `%{` and ends with `%}`. This allows for a block of text to be commented out, which is particularly useful for longer explanations or notes.
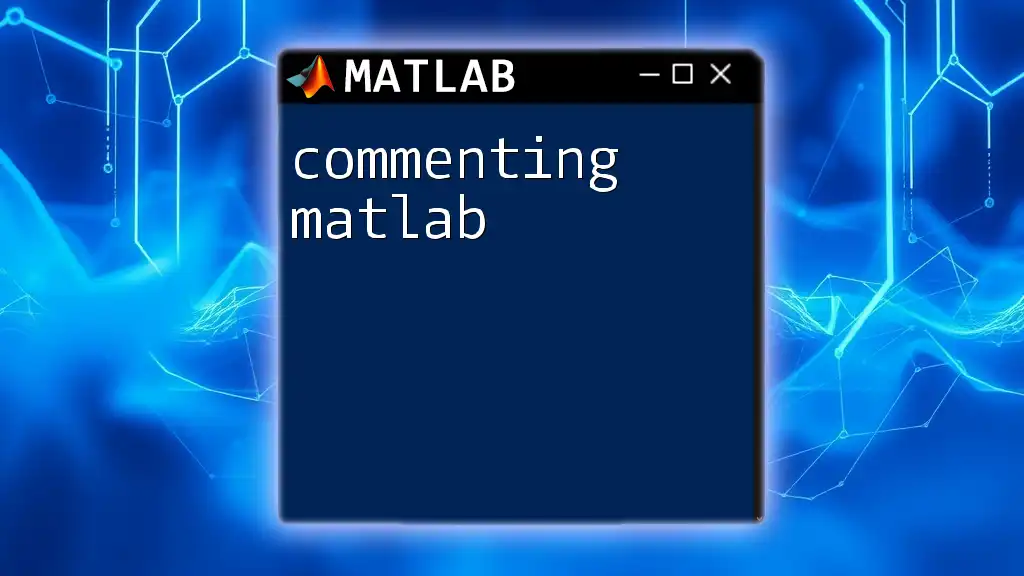
How to Use Comments Effectively
Writing Single-line Comments
Single-line comments are straightforward and can be added anywhere in your code. They are best used for brief clarifications or reminders. For example:
% This is a single-line comment in MATLAB
a = 5; % Assigning 5 to variable 'a'
In the example above, the comment clarifies the purpose of the assignment statement. Best Practice: Always aim to keep your comments concise; explain the "why" rather than just stating the "what".
Writing Multi-line Comments
Multi-line comments allow you to elaborate more extensively without cluttering your code with multiple single-line comments. Here's an example:
%{
This is a multi-line comment in MATLAB.
It allows for more detailed explanations
about code functionality.
%}
This style is perfect for documenting complex calculations or algorithms, making it clear why certain decisions were made or how the code operates.
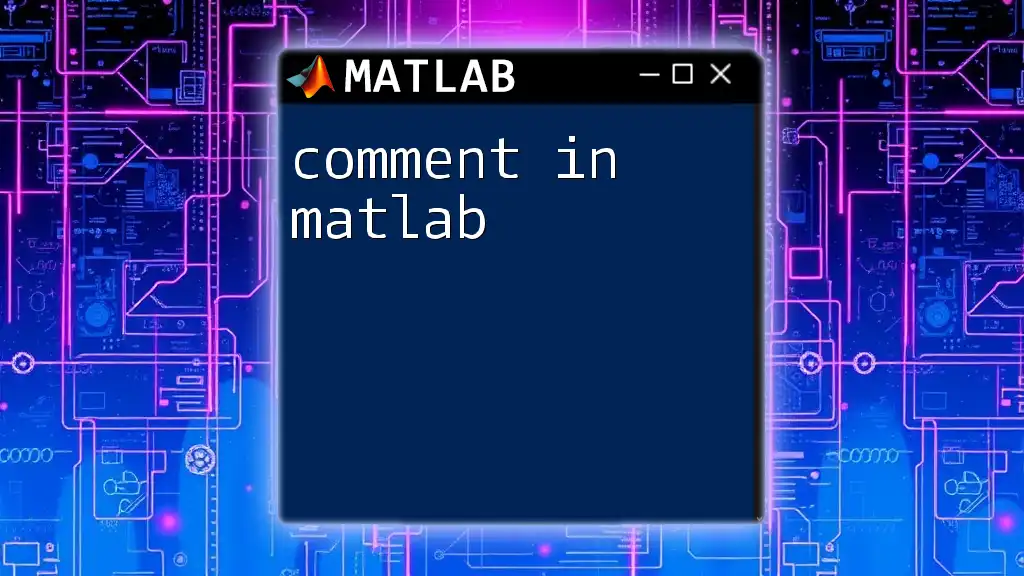
Enhancing Code Readability with Comments
Commenting for Clarity
Clear comments improve the understanding of your code, especially for others or even yourself when revisiting code after some time. For instance:
% Calculate the square of a number
number = 10;
square = number^2; % Square calculation
In this snippet, the comment above the variable assignment succinctly describes its purpose. By clearly stating the operation being performed, you allow anyone reading the code to grasp the intention quickly.
Example: Commenting a Function
Consider a simple MATLAB function that adds two numbers. Properly commenting your function enhances its clarity:
function result = addNumbers(a, b)
% addNumbers adds two numbers and returns the result.
result = a + b; % Sum of 'a' and 'b'
end
Here, the function comment specifies its purpose upfront, while the inline comment explains the computation. This approach aids in understanding the full scope of the function at a glance.
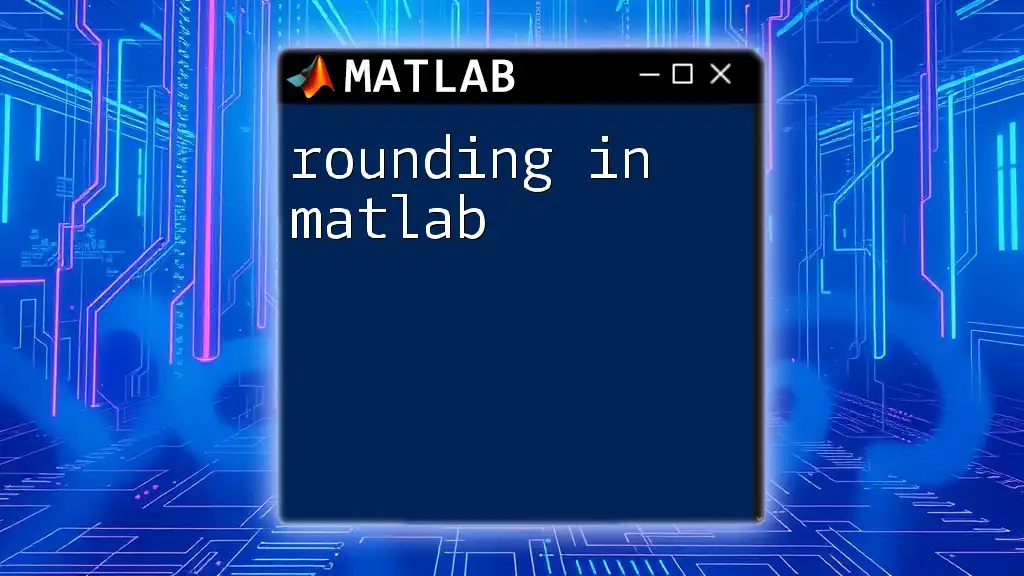
Best Practices for Commenting in MATLAB
Commenting Style
Consistency is key when it comes to commenting style. Use the same approach throughout your codebase to avoid confusion. Comments should enhance, not detract from, the readability of your code.
Avoiding Obvious Comments is another best practice. Comments should not be redundant or state the obvious. For example, instead of commenting "Increment index," simply write your code clearly enough that comments become unnecessary.
When and Where to Comment
Effective commenting includes knowing when and where to include comments. Generally, it’s beneficial to:
- Place comments before key sections of code that perform distinct functions or calculations.
- Follow complex code lines with comments to clarify their purpose.
By doing this, you ensure your code remains readable and that your logic is easily understood.
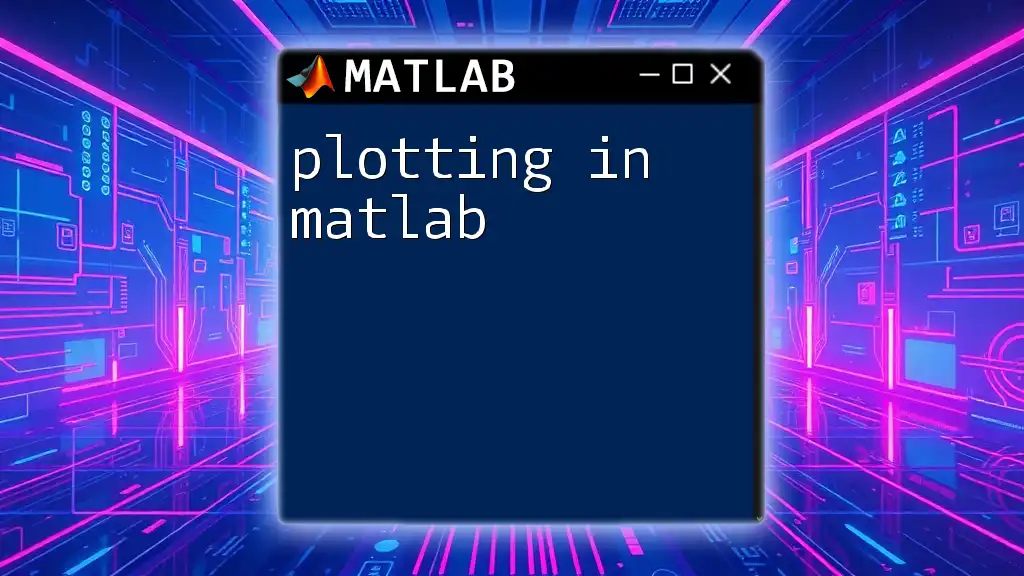
Common Mistakes to Avoid in Commenting
Overloading with Comments
One common pitfall in commenting in MATLAB is the practice of over-commenting, which can lead to clutter. While comments are beneficial, too many can obscure what the code is doing. Aim for a balanced approach.
Neglecting Comment Maintenance
As your code evolves, so should your comments. Neglected comments can mislead and confuse. Always review and update comments to ensure they accurately reflect the current functionality of your code.
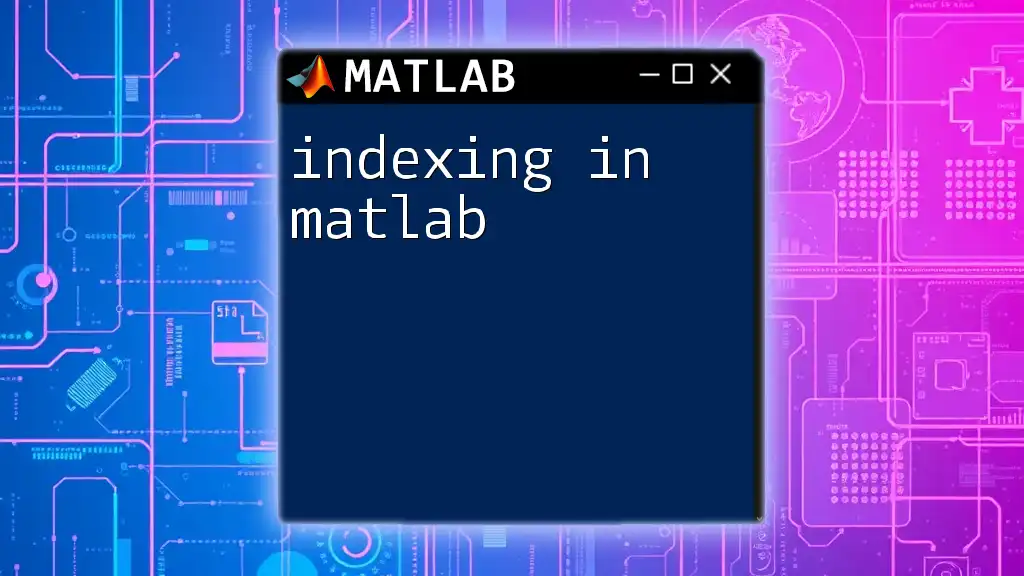
Conclusion
Effective commenting in MATLAB is a crucial practice that enhances the readability, maintainability, and overall quality of your code. By understanding how to use single-line and multi-line comments appropriately, you will significantly improve the experience for yourself and anyone who might work with your code in the future.
Remember to keep comments concise but informative, maintain consistency in style, and regularly update them as your code changes. Implementing these practices will lead to clearer, more understandable MATLAB code.
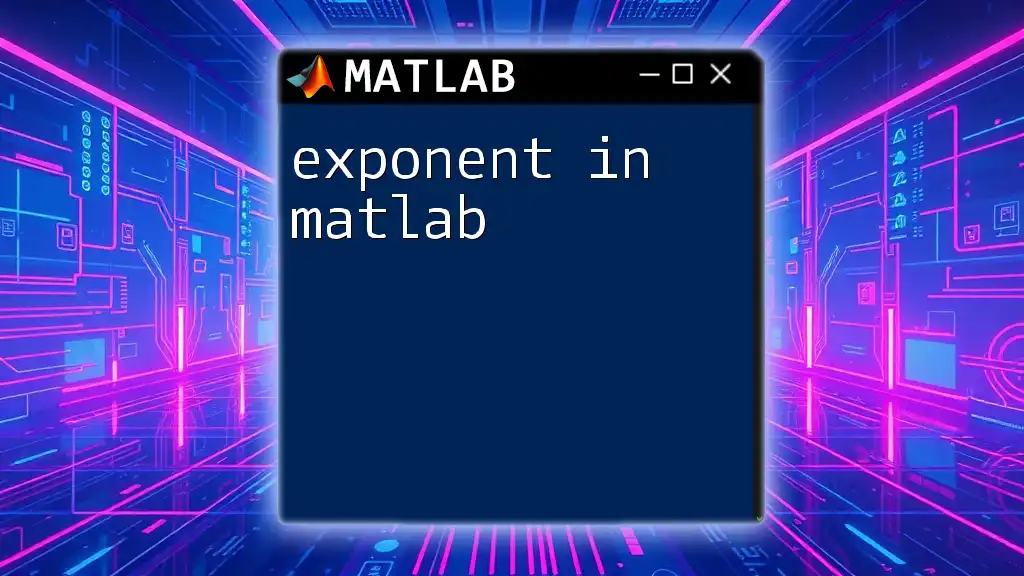
Additional Resources
For further information, you can refer to the [MATLAB official documentation on comments](https://www.mathworks.com/help/matlab/matlab_intro.html). Also, explore recommended best practices in MATLAB programming to continue enhancing your skills.
Example Exercises
To reinforce your understanding, try these exercises:
- Review a provided code snippet without comments, then add appropriate comments based on your understanding.
- Take a complex function, and write comments that explain each step of the way.
By practicing these techniques, you will become proficient in commenting in MATLAB, making your code more accessible and maintainable.