Logical indexing in MATLAB allows you to select elements of an array that meet specific logical conditions by creating a logical array where true values correspond to the desired elements.
Here’s a simple example to illustrate logical indexing:
% Creating an array
A = [1, 2, 3, 4, 5, 6];
% Logical indexing to find elements greater than 3
B = A(A > 3);
In this code, `B` will contain the elements `[4, 5, 6]` from the array `A`.
What is Logical Indexing?
Logical indexing in MATLAB is a powerful technique that allows you to access and manipulate elements of arrays based on specific conditions. It utilizes logical arrays—arrays of true (1) and false (0) values—to create a subset of data that meets a defined criterion. This method is both efficient and intuitive, making it a cornerstone of MATLAB data manipulation.
In MATLAB, logical arrays are generated by applying relational operators (such as `<`, `>`, `==`, etc.) to numeric arrays. The resulting logical array can then be used to index into the original data set, providing you with a way to selectively retrieve and manipulate values.
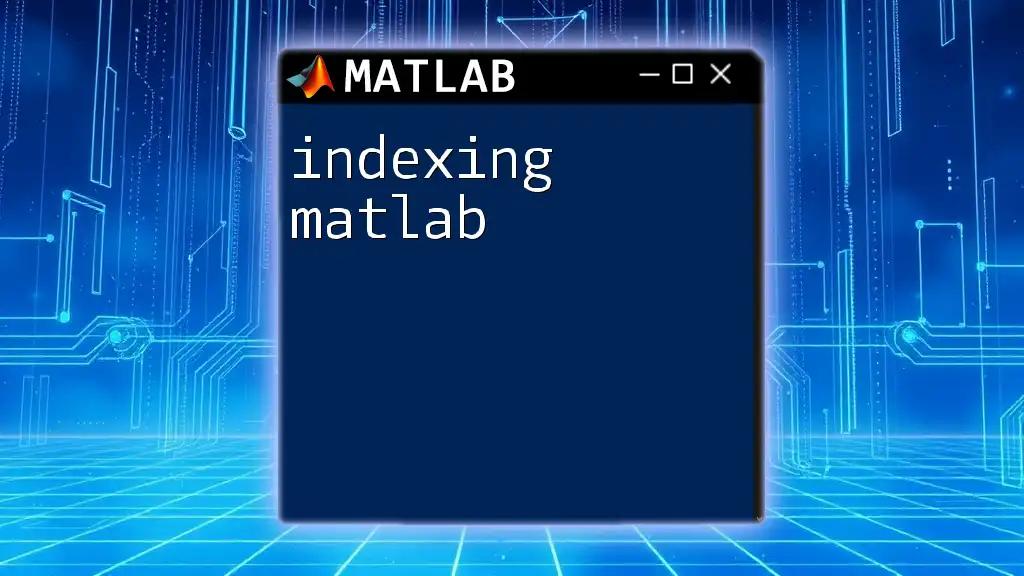
How Logical Indexing Works
Creation of Logical Arrays
Creating a logical array is straightforward. For instance, consider the following MATLAB code that generates a logical array based on a condition:
data = [1, 2, 3, 4, 5];
logicalArray = data > 3;
In this example, `logicalArray` will be `[0, 0, 0, 1, 1]`, indicating that only the elements 4 and 5 are greater than 3. This logical array can be utilized to index into the original `data` array.
Explanation of Logical True and False Values
True values (represented by 1) correspond to conditions that are met, while false values (represented by 0) indicate conditions that are not met. Logical indexing can be applied to both vectors and matrices, making it a versatile tool for data manipulation and analysis.
Use of Logical Indexing with Matrices and Vectors
Logical indexing is not limited to one-dimensional arrays. For multidimensional arrays, you can still use logical arrays effectively. For example, given the following 2D matrix:
matrix = [1, 2; 3, 4; 5, 6];
logicalMatrix = matrix > 3;
The resulting `logicalMatrix` will be:
[0, 0;
0, 1;
1, 1]
You can then use this logical array to extract values greater than 3:
filteredMatrix = matrix(logicalMatrix);
The output will be:
4
5
6
This shows how logical indexing can help quickly filter data in a matrix format.
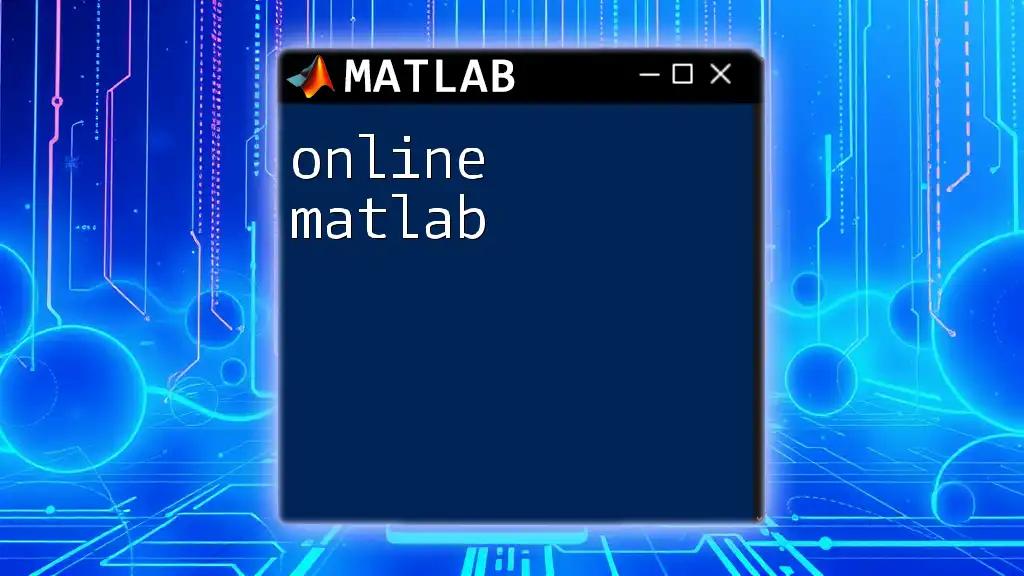
Common Use Cases for Logical Indexing
Filtering Data
One of the primary applications of logical indexing in MATLAB is filtering data based on specific criteria. This is essential for data analysis, where you often need to separate data points of interest from the rest.
Example: Filtering elements greater than a certain value
data = [1, 2, 3, 4, 5];
logicalArray = data > 3;
filteredData = data(logicalArray);
In this case, `filteredData` will yield `[4, 5]`, allowing you to focus only on the elements that meet your condition.
Modifying Data
Logical indexing can also be employed to modify specific values within an array based on set conditions. This can be especially useful during data preprocessing and cleanup.
Example: Changing values based on a condition
data = [1, 2, 3, 4, 5];
logicalArray = data > 3;
data(logicalArray) = 10; % Set values greater than 3 to 10
After executing this code, the modified `data` will be `[1, 2, 3, 10, 10]`. This demonstrates how easily you can make bulk modifications using logical indexing.
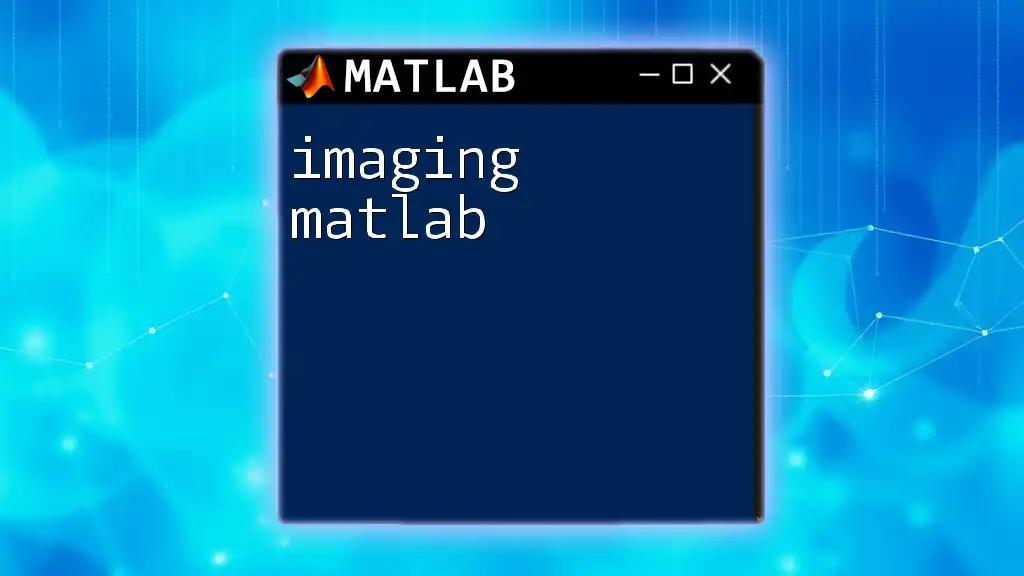
Combining Logical Conditions
In mathematical and analytical contexts, you may need to combine multiple conditions to filter or manipulate data effectively. MATLAB provides logical operators for this purpose:
- AND (`&`): Returns true if both conditions are true.
- OR (`|`): Returns true if at least one condition is true.
- NOT (`~`): Returns true if a condition is false.
Example: Using multiple conditions
data = [1, 2, 3, 4, 5];
logicalArray = (data > 2) & (data < 5);
filteredData = data(logicalArray);
In this example, `filteredData` will contain the values `[3, 4]`, which are the elements that are both greater than 2 and less than 5.
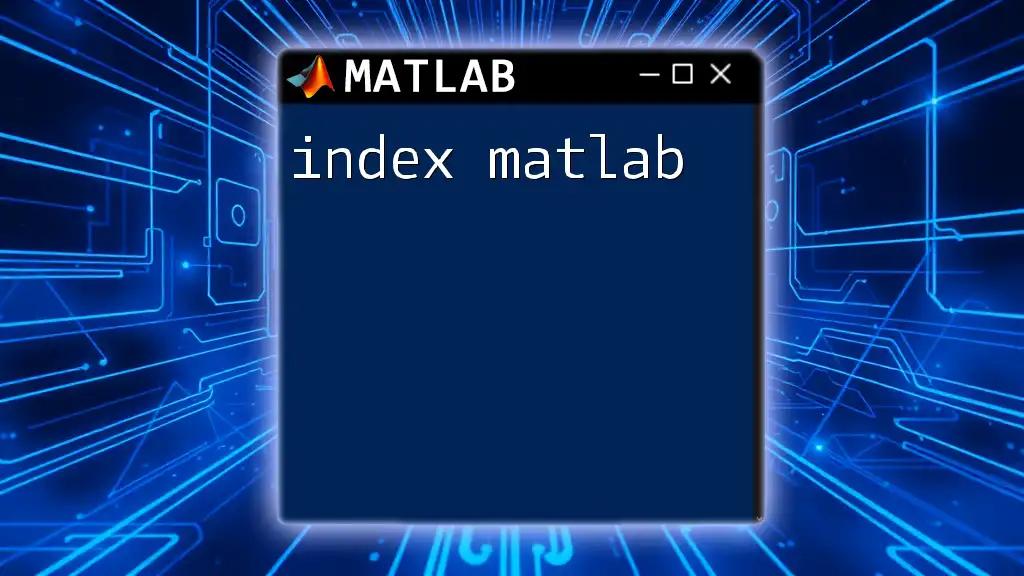
Advanced Logical Indexing Techniques
Using the `find` Function
The `find` function is extremely useful when you need to retrieve the indices of elements in an array that meet a certain condition. This can be a significant advantage when dealing with large datasets.
Example: Finding indices of elements that meet a condition
data = [1, 2, 3, 4, 5];
indices = find(data > 3);
In this case, `indices` will hold the values `[4, 5]`, indicating the positions of the elements in `data` that are greater than 3.
Logical Indexing with Multidimensional Arrays
Logical indexing is not just limited to vectors; you can apply it to multidimensional arrays as well, making it even more powerful.
Example: Working with matrices
matrix = [1, 2; 3, 4; 5, 6];
logicalMatrix = matrix > 3;
filteredMatrix = matrix(logicalMatrix);
The `filteredMatrix` will contain the values `[4; 5; 6]`, showing how logical indexing facilitates effective data manipulation across different array dimensions.

Best Practices for Using Logical Indexing
When utilizing logical indexing in MATLAB, consider the following best practices to enhance code efficiency and readability:
- Keep Logical Conditions Simple: Complex conditions can clutter your code. Break them down into smaller, manageable steps if necessary.
- Be Mindful of Memory Usage: Logical indexing can lead to the creation of many temporary arrays. If you're working with large datasets, monitor memory usage to avoid performance issues.
- Use Clear and Descriptive Variable Names: This will help make your code more understandable, especially when sharing it with others or revisiting it later.
By following these guidelines, you'll write cleaner, more efficient logical indexing code that enhances your overall MATLAB experience.

Conclusion
In summary, logical indexing in MATLAB is an invaluable technique for efficiently accessing and manipulating data. By understanding how to create and use logical arrays, you can perform a wide array of data filtering and modification tasks with ease. As you practice and implement logical indexing in your projects, the benefits of this method will become increasingly apparent.

Additional Resources
For further information on logical indexing in MATLAB, consider exploring the official MATLAB documentation. Additionally, a variety of books and online resources are available to deepen your knowledge in this and other MATLAB concepts. Make sure to check out our upcoming workshops and courses, which will provide you with hands-on experiences and practical applications to enhance your MATLAB skills.