To create a function in MATLAB, define it using the `function` keyword followed by the output variable(s), the function name, and input variable(s) within a dedicated file or script.
function output = myFunction(input)
output = input^2; % This function squares the input value
end
Understanding Functions in MATLAB
What is a Function?
A function in programming is a self-contained block of code designed to perform a specific task. Unlike scripts, which execute a series of commands without any input or output parameters, functions encapsulate logic and can accept inputs while returning outputs. This encapsulation allows for modular programming, where complex problems can be broken down into smaller, manageable pieces.
Why Use Functions?
Functions are essential for several reasons:
- Code reusability: Once a function is written, it can be called multiple times, reducing code duplication.
- Improving readability: Functions help to separate concerns; they make the code easier to read and understand.
- Simplifying debugging: By isolating parts of the program using functions, finding and fixing bugs becomes more straightforward.
Example: Consider a situation where we need to calculate the area of several rectangles. Instead of repeating the code for the area calculation each time, we can define it as a function, call it whenever needed, and only modify it in one place if necessary.

Basics of MATLAB Function Syntax
Structure of a Function
The basic structure of a MATLAB function follows this format:
function [output1, output2] = functionName(input1, input2)
% body of the function
end
In this structure:
- The function keyword indicates that you are defining a function.
- The square brackets `[]` denote the outputs, allowing for multiple return values.
- The parentheses `()` hold the function's inputs.
Breaking Down the Syntax
The `function` Keyword
The `function` keyword signals the start of a new function definition. It's essential to use this keyword, as it tells MATLAB to treat the following code as a separate entity.
Function Name
Function names must be unique and follow certain rules:
- Must start with a letter
- Can include letters, numbers, and underscores
- Best practices suggest using descriptive names that clearly indicate the function's purpose, enhancing readability.
Inputs and Outputs
MATLAB allows you to specify input and output variables using the aforementioned brackets. For instance, if a function computes both the area and perimeter of a rectangle, it can return two outputs as follows:
function [area, perimeter] = computeRectangleProps(length, width)
area = length * width;
perimeter = 2 * (length + width);
end

Creating Your First MATLAB Function
Step-by-Step Example
Let’s create a simple function that computes the area of a rectangle.
Here’s how to write the function:
function area = computeArea(length, width)
% computes the area of a rectangle
area = length * width;
end
Save this function with the filename `computeArea.m`. MATLAB requires that the filename matches the function name.
Testing the Function
After saving your function, you can call it from the MATLAB command window. For example:
result = computeArea(5, 10);
disp(result); % Output should be 50
This command will display the area calculated by the `computeArea` function, illustrating its practical application.

Advanced Function Features
Variable Number of Inputs
MATLAB provides the ability to handle a variable number of input arguments by using `varargin`. This is particularly useful when the number of inputs can change.
For example, let’s create a function that computes the average of a set of numbers:
function avg = computeAverage(varargin)
avg = mean(cell2mat(varargin));
end
Nested Functions
Nested functions are functions defined within another function. They have access to the parent function's workspace, which makes them powerful for specific applications.
When to Use Them: Nested functions are useful when you need to break down a parent function into smaller tasks without exposing those tasks to the outside code.
Example of a nested function:
function outerFunction(x)
function innerFunction(y)
disp(y);
end
innerFunction(x);
end
Anonymous Functions
Anonymous functions are single-line functions that do not require a separate file. They can be useful for quick calculations or for passing short operations into other functions.
Syntax and Example:
square = @(x) x.^2;
disp(square(4)); % Output should be 16
Anonymous functions offer flexibility and are often used in conjunction with other functions, such as `arrayfun` or `cellfun`.
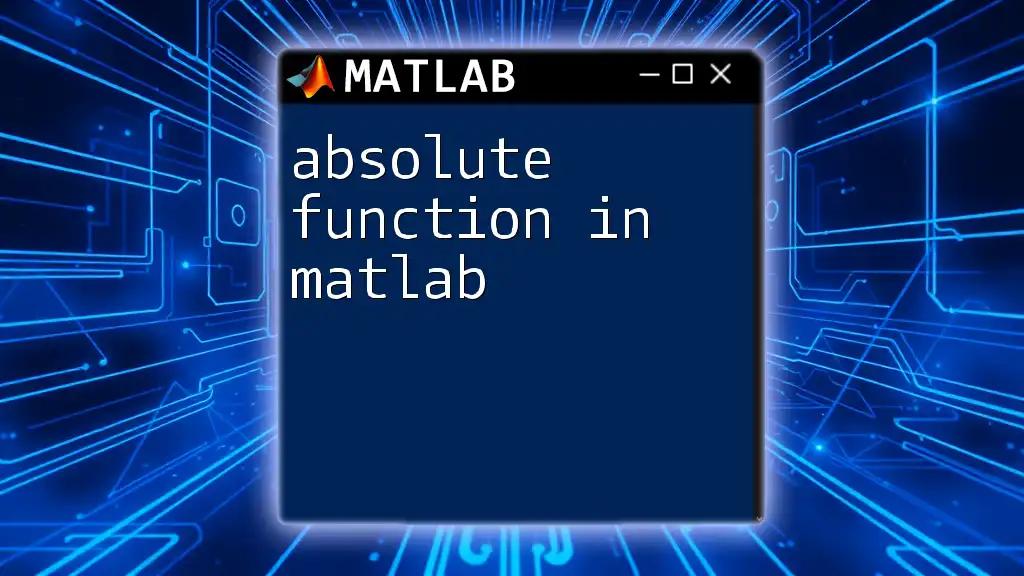
Best Practices for Writing MATLAB Functions
Documenting Your Function
Documentation is critical for understanding the purpose and mechanics of your function. You can include comments at the beginning to explain what the function does, its inputs, and outputs.
Here’s an example of a well-documented function:
function result = power(base, exponent)
% POWER Calculate the power of a base raised to an exponent
% Inputs:
% base - the base number
% exponent - the exponent to raise the base to
result = base^exponent;
end
By documenting your functions properly, you improve usability for both yourself and others who may utilize your code in the future.
Error Handling
Error handling is an essential aspect of function development. Using `error` and `warning` statements allows you to manage unexpected inputs or conditions gracefully.
For example, consider the need to ensure that division by zero does not occur. You could write:
function result = safeDivision(numerator, denominator)
if denominator == 0
error('Division by zero is not allowed.');
end
result = numerator / denominator;
end
This function checks the denominator before performing the division, preventing potential runtime errors.

Conclusion
This comprehensive guide has navigated through the essential components of how to make a function in MATLAB. You’ve learned about defining functions, handling inputs and outputs, and explored advanced features like nested and anonymous functions. Additionally, the significance of proper documentation and error handling was emphasized.
Now, it's time to practice! Take these concepts and apply them to your own projects—creating functions will not only help you organize your code but also enhance its readability and maintainability.
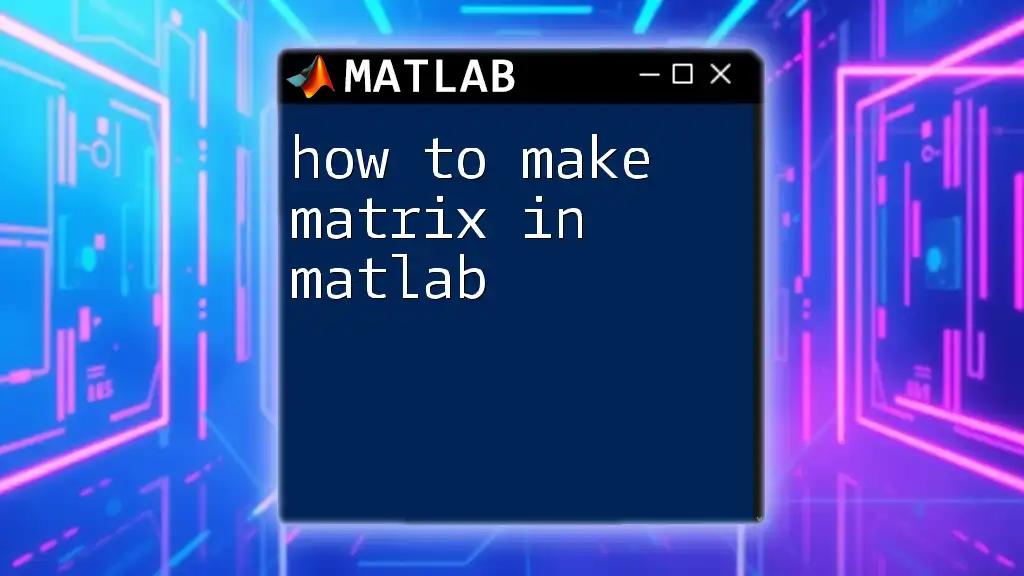
Additional Resources
For further reading and deeper understanding, check out MATLAB's official documentation regarding functions, as they provide extensive examples and tutorials that complement this guide.
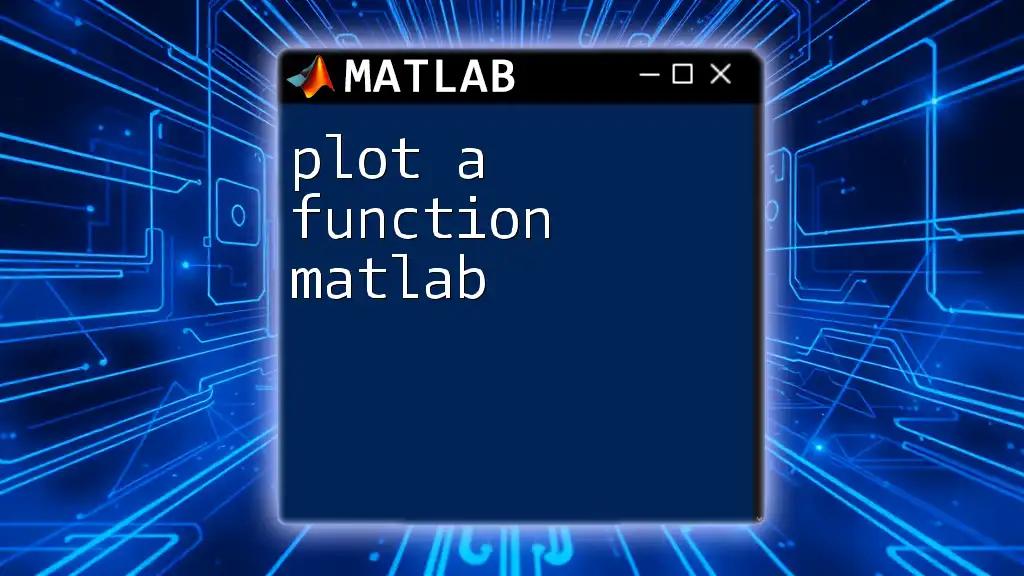
Call to Action
If you found this article helpful, consider subscribing to our newsletter for more MATLAB tips and tricks. Stay tuned for our upcoming posts on more advanced MATLAB programming techniques and data visualization!