In MATLAB, functions are defined using the `function` keyword to create reusable code blocks that can accept inputs and return outputs, enhancing efficiency and organization in programming.
Here’s an example of a simple function that calculates the square of a number:
function result = squareNumber(x)
result = x^2;
end
Understanding MATLAB Functions
What is a Function?
In programming, a function is a block of code designed to perform a specific task. Functions can take inputs, process them, and return outputs. In MATLAB, functions serve a critical role in organizing code and enhancing code reusability.
Functions differ from scripts in that:
- Functions can accept inputs and return outputs.
- Scripts are sequences of commands without input/output interface and rely on workspace variables.
Advantages of Using Functions
The use of functions in MATLAB provides several significant advantages:
- Code Reusability: Once a function is created, it can be called multiple times throughout a program or even in different projects, reducing redundancy.
- Improved Organization and Readability: Functions allow you to break complex problems into smaller, manageable pieces, making the overall code easier to follow.
- Easier Debugging and Testing: Isolating specific tasks within functions enables easier validation and error identification, helping to streamline the debugging process.
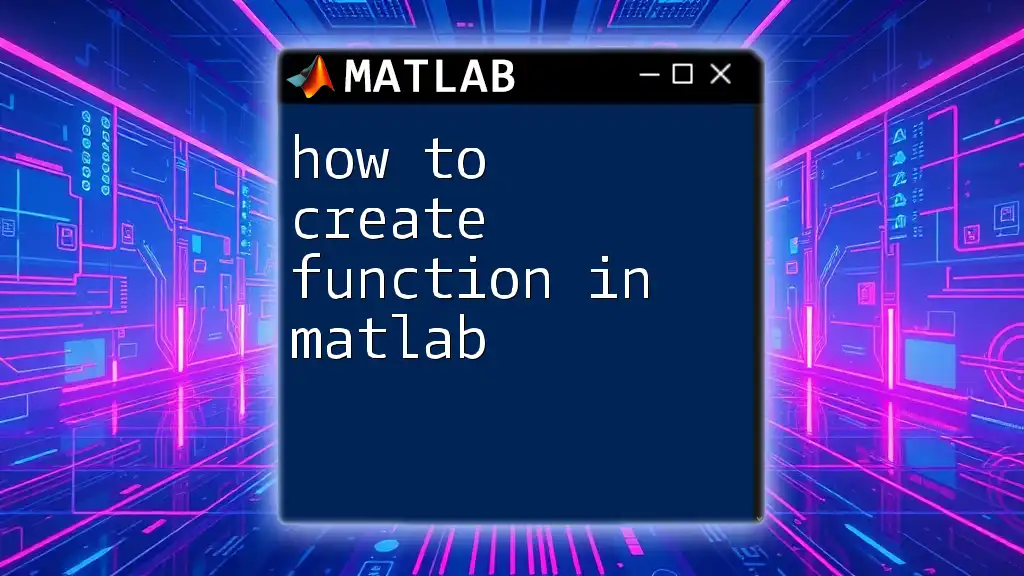
Creating a Function in MATLAB
Basic Function Structure
To create a function, you need to follow a specific syntax:
function output = functionName(inputs)
% Function code goes here
end
For example, consider a simple function that doubles its input:
function output = simpleFunction(input)
output = input * 2;
end
In this example, `simpleFunction` takes an input value, multiplies it by 2, and assigns the result to `output`.
Saving a Function
When you save a function, ensure that:
- The filename matches the function name exactly (case-sensitive).
- The file has a .m extension.
For instance, in the previous example, save the function as `simpleFunction.m`.
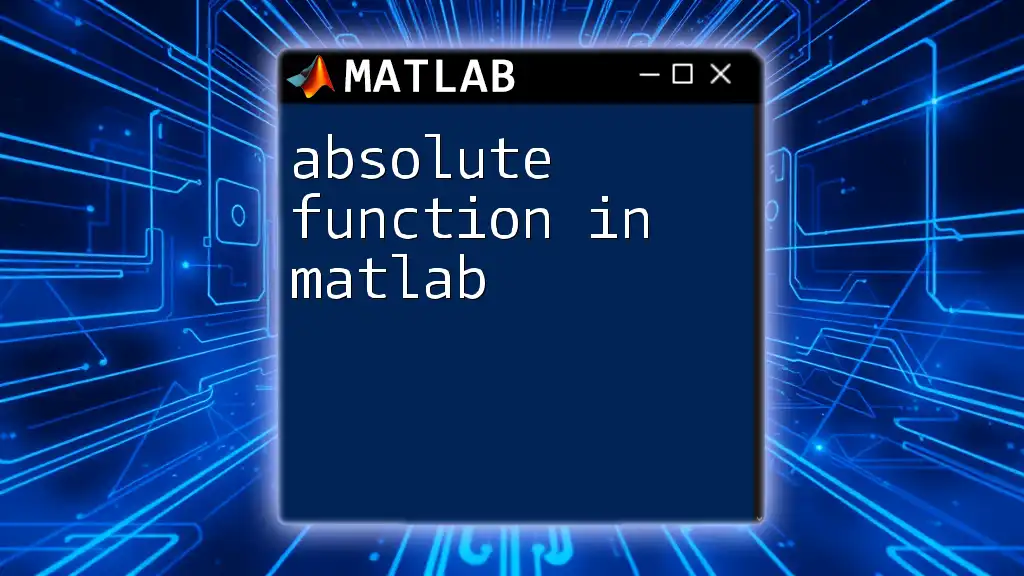
Calling Functions
How to Call a Function from the Command Window
Once you've defined your function, calling it is straightforward. For example, using the command window:
result = simpleFunction(5);
This command will execute the function `simpleFunction` with an input of `5` and store the resulting output in the variable `result`.
Calling Functions from Scripts
You can also call functions from a script. Create a new script named `testScript.m` with this content:
% script file: testScript.m
result = simpleFunction(10);
disp(result);
Running this script will display `20`, as the function doubles the input of `10`.
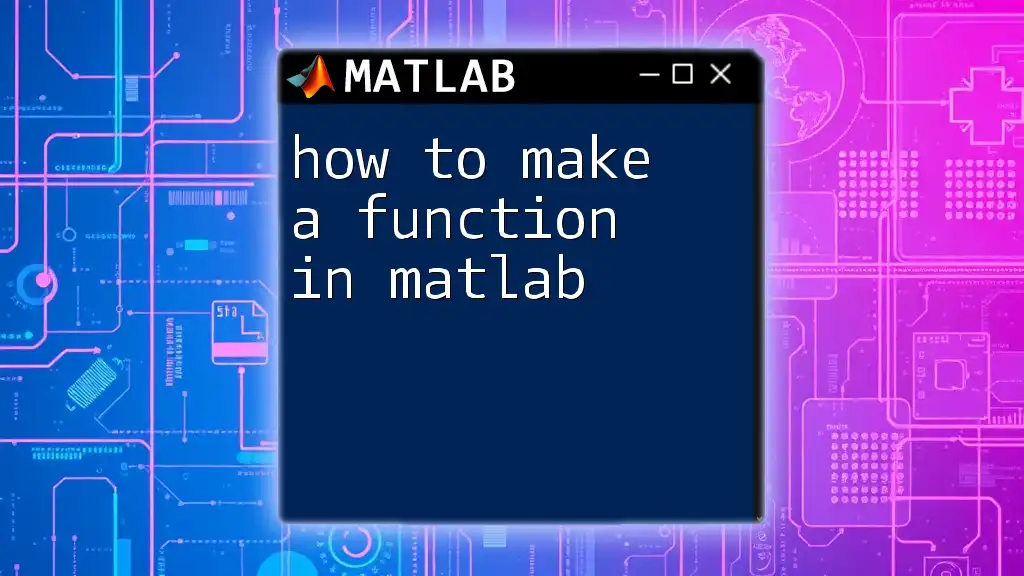
Function Inputs and Outputs
Defining Function Inputs
You can accept multiple inputs in a function. Here’s an example of a function that adds two numbers:
function sum = addNumbers(a, b)
sum = a + b;
end
To call this function:
result = addNumbers(3, 4);
Defining Function Outputs
Returning multiple outputs can be achieved by enclosing the output variables in square brackets:
function [sum, diff] = operateNumbers(a, b)
sum = a + b;
diff = a - b;
end
You can call and unpack the outputs like this:
[sumResult, diffResult] = operateNumbers(10, 5);
This will set `sumResult` to `15` and `diffResult` to `5`.
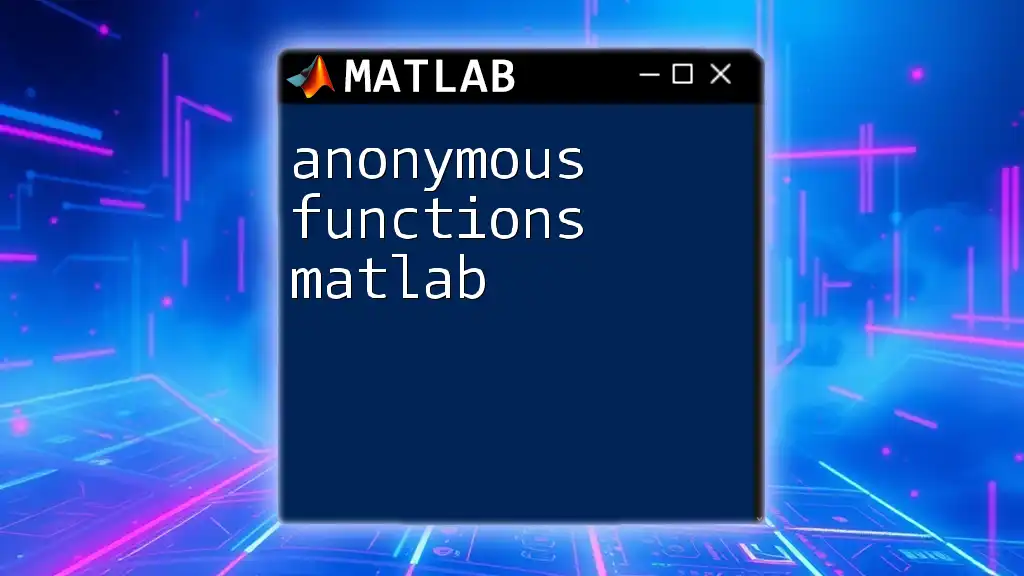
Variable Scope in Functions
Local vs Global Variables
Understanding variable scope is crucial when working with functions. Local variables are defined within a function and cannot be accessed outside of it, while global variables are accessible from any part of the code.
Using Global Variables
To use global variables in functions, declare them with the `global` keyword:
global gVar;
gVar = 100;
function output = useGlobal()
global gVar;
output = gVar + 10;
end
In this example, when you call `useGlobal`, it will return `110`.
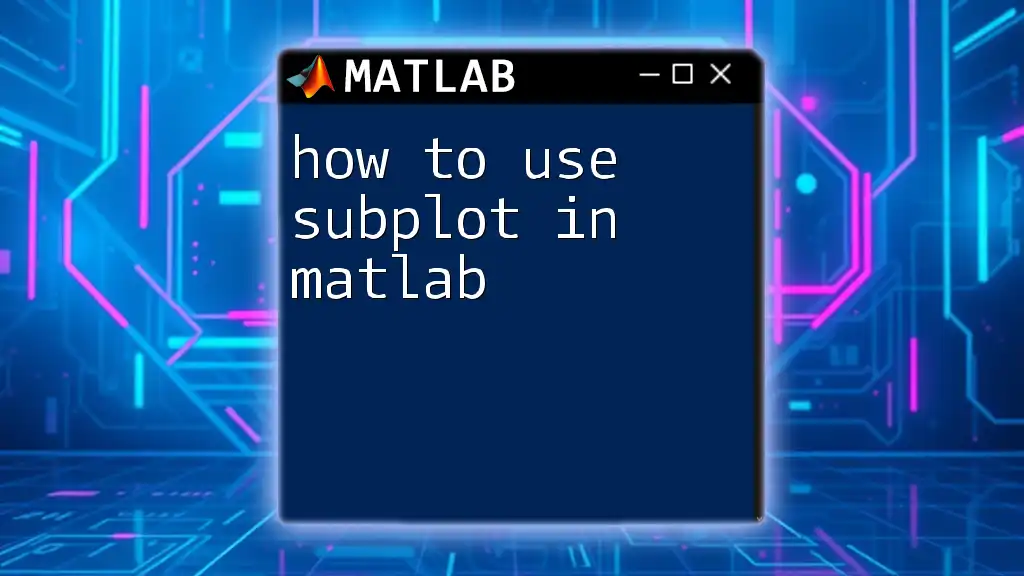
Built-in Functions and Custom Functions
Overview of Built-in Functions
MATLAB comes with a multitude of built-in functions that simplify many tasks. Some commonly used functions include:
- `sum()`: Calculates the sum of elements in an array.
- `mean()`: Computes the average.
- `max()`: Finds the maximum value.
Use the MATLAB documentation to explore and understand various built-in functions at your disposal.
Creating Custom Functions
Custom functions serve specific tasks that may not be addressed by built-in functions. Tips for optimizing your custom functions include:
- Keep functionalities focused and coherent.
- Avoid unnecessary computations within the function.
- Document your function to explain its purpose and usage.
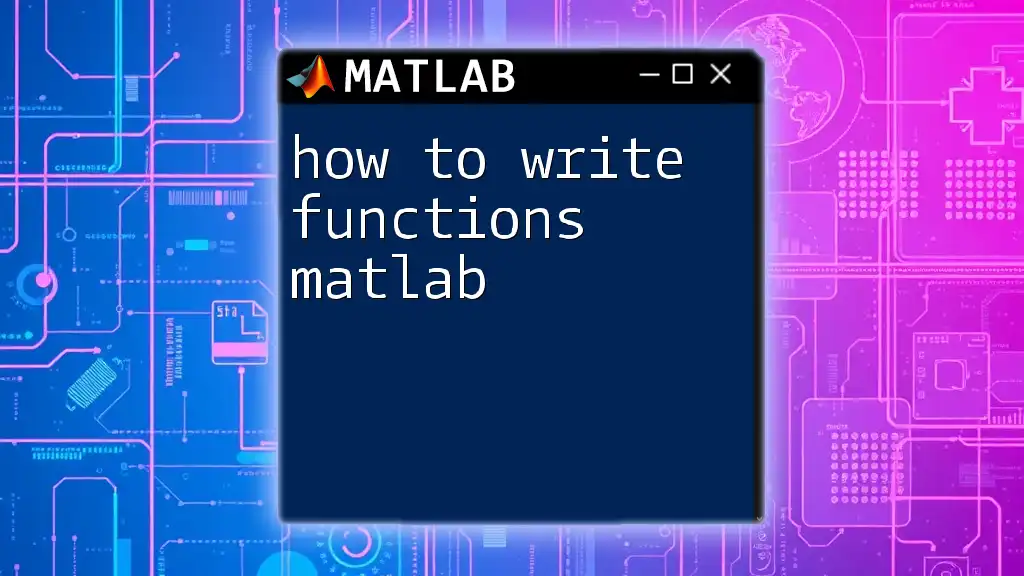
Function Handles
What is a Function Handle?
A function handle is a MATLAB data type that represents a function. It enables you to call functions indirectly, store them in variables, and pass them to other functions. To create a function handle, use the `@` symbol:
funcHandle = @simpleFunction;
result = funcHandle(20);
This creates a handle to `simpleFunction` and allows you to call it as though it were a variable.
Using Function Handles with `feval`
The `feval` function allows you to call functions dynamically using their handles:
result = feval(funcHandle, 15);
Using function handles becomes especially useful in scenarios where you need to pass functions as arguments to other higher-order functions or optimize computations.
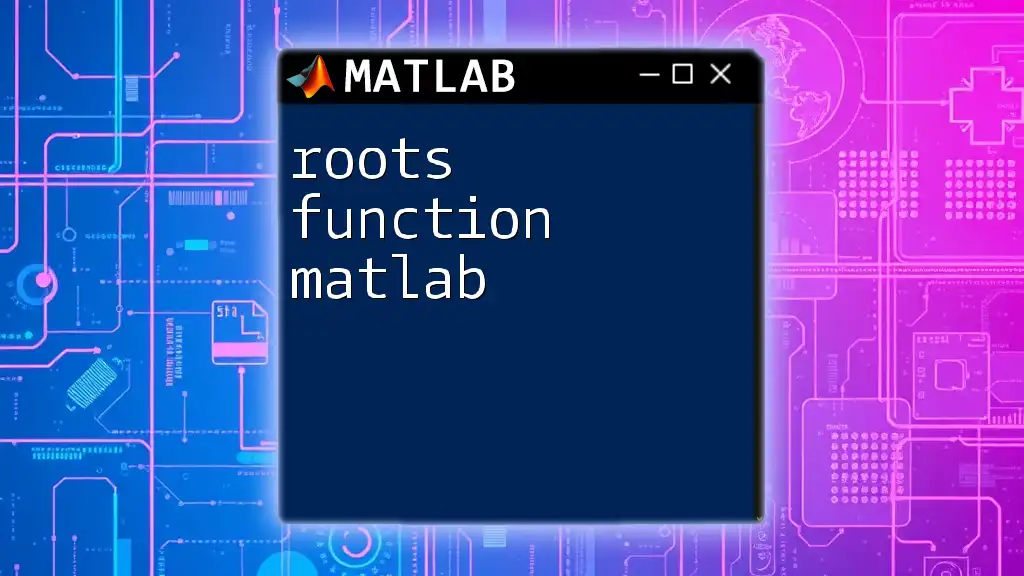
Conclusion
Functions are fundamental tools in MATLAB, allowing you to write clean, efficient, and reusable code. They help transform complex tasks into manageable sections of code, making programming not only easier but also more productive. Mastering how to use functions in MATLAB will significantly enhance your programming skills and efficiency.
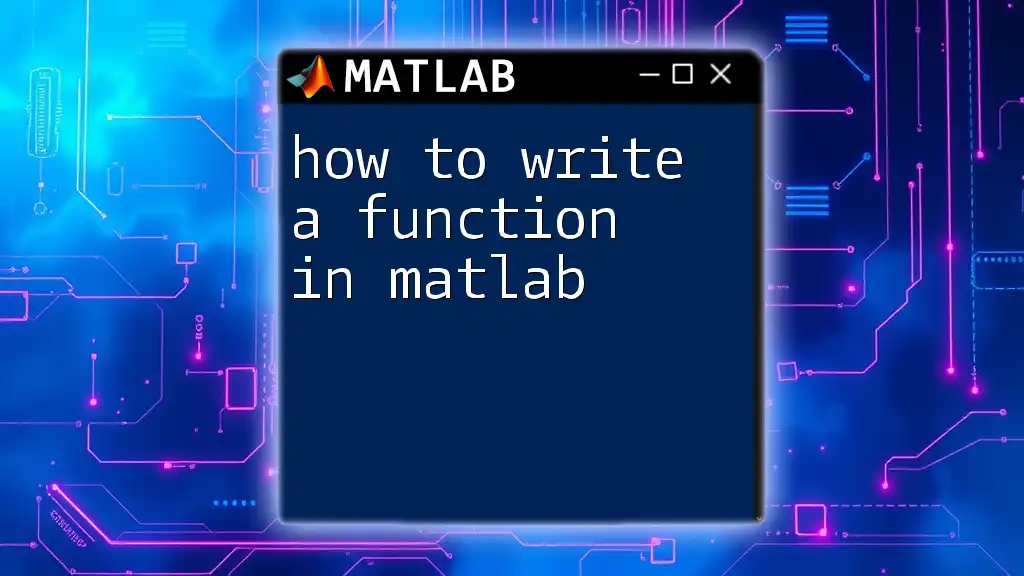
Additional Resources
To further deepen your understanding of MATLAB functions, explore the official MATLAB documentation or consider enrolling in a course that focuses on advanced MATLAB programming techniques. Engage with community forums to share knowledge and experience as you enhance your coding proficiency in MATLAB.