To transform instrument coordinates to planetary coordinates in MATLAB, you can use a matrix transformation that incorporates rotation and translation based on the specific alignment of your instrument with respect to the planetary reference frame.
Here's a simple code snippet to illustrate this transformation:
% Define instrument coordinates
instrument_coords = [x; y; z];
% Define the transformation matrix (rotation and translation)
R = [0 -1 0; 1 0 0; 0 0 1]; % Example rotation matrix
T = [dx; dy; dz]; % Translation vector
% Transform to planetary coordinates
planetary_coords = R * instrument_coords + T;
Understanding Coordinate Systems
What Are Instrument Coordinates?
Instrument coordinates are a specific coordinate system used to denote positions and orientations with respect to a particular instrument's reference frame. This system is crucial in various applications, including astronomical observations, where instruments like telescopes gather data about celestial objects. Each instrument may have its own unique set of axes or reference points, making it essential to clearly define the coordinate system used.
In practice, the instrument coordinates could refer to the physical measurements of the instrument itself or the raw data collected from observations. These coordinates are inherently linked to the instrument's orientation, meaning any tilt, rotation, or movement can significantly affect the recorded values.
What Are Planetary Coordinates?
Planetary coordinates, on the other hand, refer to a globally accepted coordinate system, typically based on celestial bodies. For astronomical applications, planetary coordinates can be defined in various formats such as equatorial coordinates, which include right ascension (RA) and declination (Dec), or ecliptic coordinates, which relate to the plane of the Earth's orbit.
Understanding these coordinates is crucial because they help scientists and astronomers locate and analyze celestial objects in a consistent manner. Whether studying planets, stars, or galaxies, transitioning between instrument coordinates and planetary coordinates is a common task that enhances our understanding of the universe.
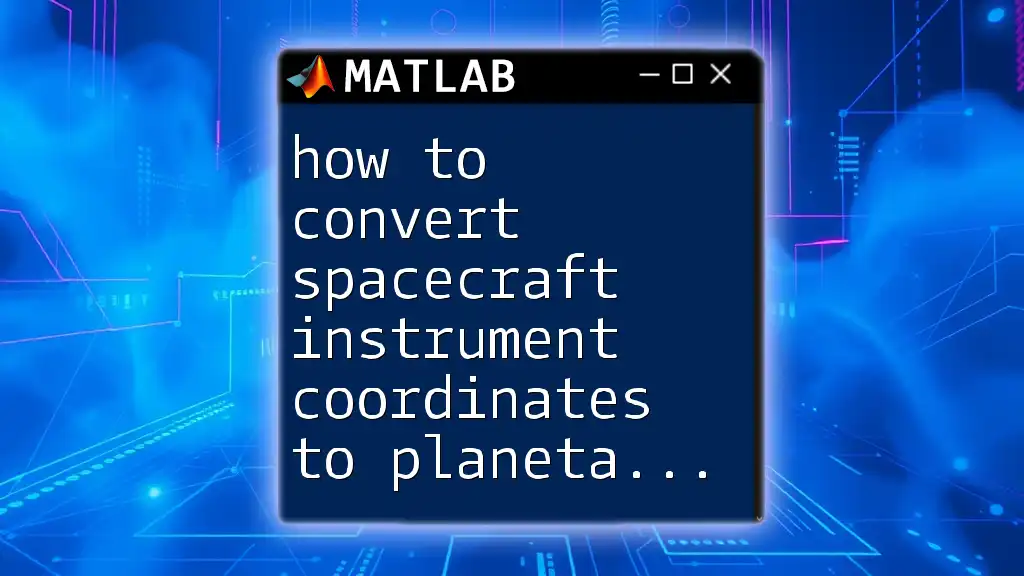
Concepts Behind Coordinate Transformation
Basics of Coordinate Transformation
Coordinate transformation involves expressing the coordinates of a point or a set of points from one system to another. Essentially, it acts as a bridge that allows data collected from an instrument to be interpreted within a broader context, such as astronomical databases or models.
There are mainly two types of transformations:
- Linear Transformations: These involve adding or multiplying coordinates by a constant or a matrix, maintaining parallelism and ratios of distances.
- Nonlinear Transformations: More complex transformations that can involve curves or other nonlinear relations between the coordinates.
Mathematical Foundations
To successfully transform instrument coordinates to planetary coordinates, one must employ a defined set of mathematical equations that describe the relationship between the two coordinate systems. This often revolves around creating a transformation matrix that considers any rotations, translations, or other geometric changes that have occurred.
A fundamental part of this is recognizing different reference frames that exist in the astronomical context, such as Earth-centered inertial or Earth-fixed frames, which will dictate how transformations are computed.
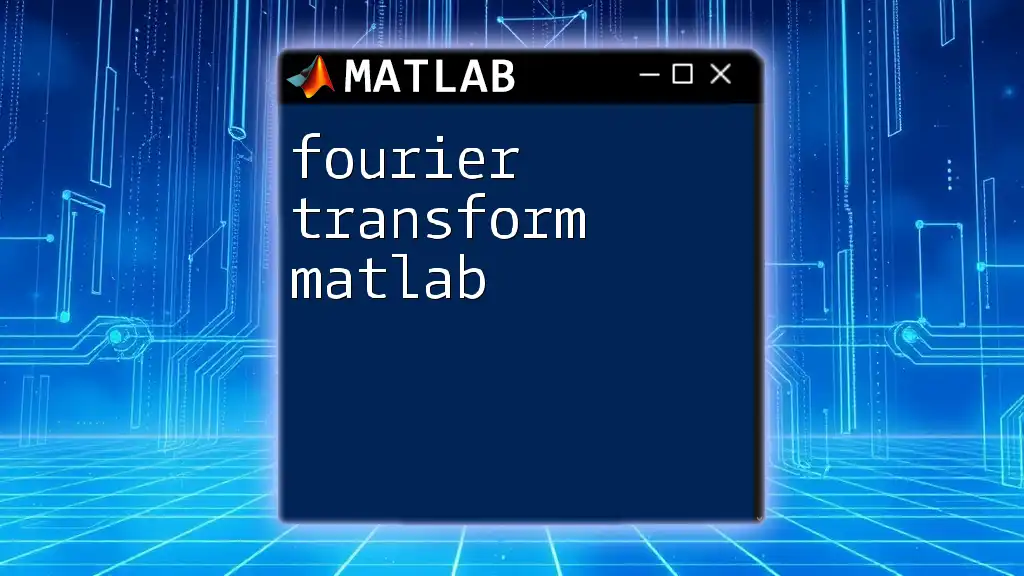
Implementing the Transformation in MATLAB
Setting Up Your MATLAB Environment
Before diving into the code, ensure that your MATLAB environment is set up appropriately. You may need specific toolboxes like the Aerospace Toolbox for more advanced functions related to coordinate transformation. Checking your installation for the necessary packages will save time and avoid errors later on.
Defining Instrument Coordinates
To begin, you need to define the instrument coordinates accurately within MATLAB. This could be a 3D vector representing the instrument's data in terms of its x, y, and z axes. Here's how to define these coordinates:
instrument_coords = [1; 2; 3]; % Example coordinates in a 3D space
In this snippet, `instrument_coords` is a 3D column vector representing some arbitrary point in the instrument's reference frame.
Determining Transformation Parameters
Reference Frame Selection
The first step in the transformation process is selecting the appropriate reference frame. This decision will depend on the specific astronomical context or objectives of the analysis. For instance, if you are transforming data from a ground-based telescope in a fixed position, you must consider the earth's rotation when selecting the reference frame to avoid discrepancies.
Transformation Matrix
The next crucial step is to construct a transformation matrix that encapsulates the rotation and translation necessary to map the instrument coordinates to planetary coordinates. This matrix will typically include angles of rotation about the axes, often denoted as theta. Here’s an example of how to define a simple transformation matrix:
theta = pi / 4; % Example angle in radians
R = [cos(theta) -sin(theta) 0;
sin(theta) cos(theta) 0;
0 0 1]; % 2D rotation in X-Y plane
In this transformation matrix `R`, the components correspond to a rotation in the X-Y plane. Each element in the matrix affects how the coordinates are transformed, with cosine and sine functions defining the rotation in relation to the specified angle.
Performing the Transformation
Now that you have both your instrument coordinates and the transformation matrix, performing the transformation is straightforward. The coordinates must first be transposed to facilitate matrix multiplication. Here’s how the transformation can be coded:
planetary_coords = R * instrument_coords;
Here, `planetary_coords` will yield the resulting coordinates in the planetary reference frame after applying the transformation matrix. It's essential to understand how the linear algebra properties work in this context, as they directly influence the outcome.
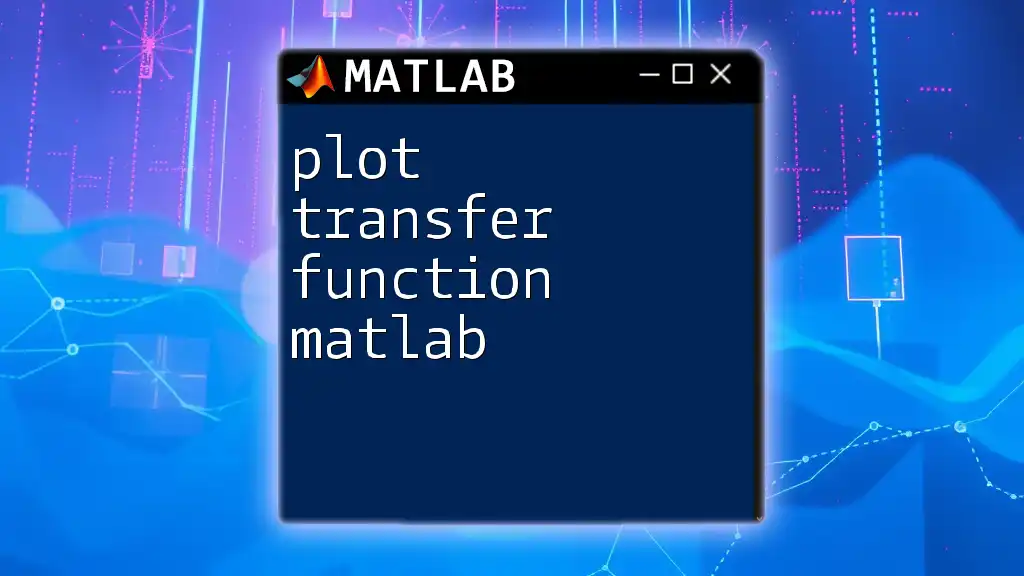
Practical Example
Scenario: Transforming Telescope Data
Let’s contextualize this transformation in a practical scenario involving data collected from a telescope. Say you have instrument coordinates collected from a telescope pointing at a celestial body, and you wish to convert these to planetary coordinates for analysis or comparison with celestial databases.
Assume the following setup:
% Define the angle of rotation
theta = pi / 4; % Example angle in radians
% Example instrument coordinates
instrument_coords = [1; 1; 0];
% Define the transformation matrix
R = [cos(theta) -sin(theta) 0;
sin(theta) cos(theta) 0;
0 0 1];
% Perform the transformation
planetary_coords = R * instrument_coords;
In this scenario, after performing the transformation, you would get outputs for `planetary_coords` that could be used in various databases or analytical tools, allowing for consistent analysis across different datasets.
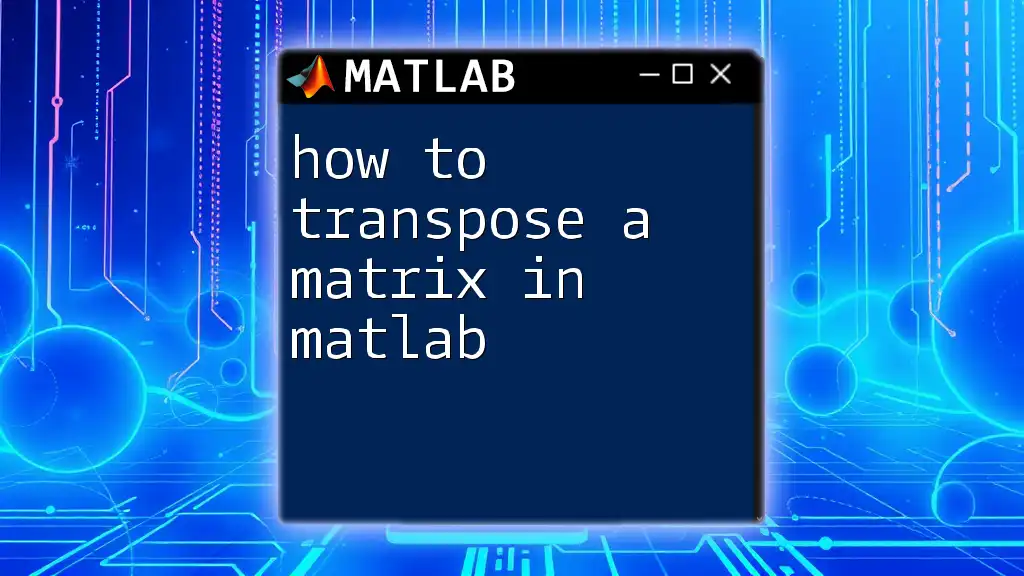
Troubleshooting Common Issues
Incorrect Outputs
There may be times when the transformation yields incorrect results. Common culprits include:
- Inaccurate angle definitions or unit discrepancies.
- Errors in the matrix setup, such as reversed signs or incorrect dimensions.
To debug these issues, verify each step meticulously, ensuring that the input coordinates and transformation matrix are defined and employed correctly.
Optimization Tips
To enhance efficiency in this transformation, consider the following best practices:
- Minimize data size where possible by pre-processing the input values.
- Use vectorized operations instead of loops when dealing with larger datasets to improve performance.
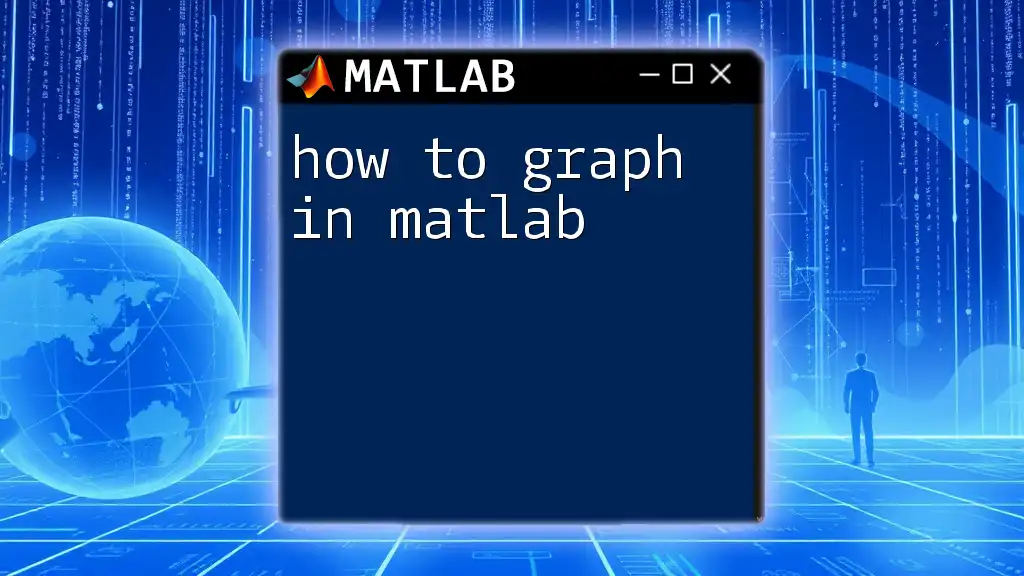
Conclusion
Transforming instrument coordinates to planetary coordinates in MATLAB is an essential skill for researchers and engineers operating in fields like astronomy and space exploration. By following the structured approach of defining coordinates, selecting reference frames, constructing transformation matrices, and performing transformations, you can navigate this complex yet rewarding aspect of data analysis.
Consistency in practice and a solid understanding of the underlying mathematics will empower you to tackle various coordinate transformation challenges with confidence.