The `fprintf` function in MATLAB is used to format and display text output to the command window or a file, allowing you to specify how the data should be presented.
Here's a simple example using `fprintf`:
x = 5;
fprintf('The value of x is: %d\n', x);
Understanding the Basics of `fprintf`
Syntax of `fprintf`
The basic structure of the `fprintf` command in MATLAB is as follows:
fprintf(formatSpec, A, ...)
Here, `formatSpec` is a string that specifies the output format, and `A` represents the data to print. It’s essential to understand this syntax as it allows you to control precisely how the output is formatted.
Format Specifiers
What are Format Specifiers?
Format specifiers in `fprintf` play a crucial role in determining how data appears when printed. They tell MATLAB whether you want a number displayed as an integer, decimal, string, etc. This control over output appearance is one of the key benefits of using `fprintf`.
Common Format Specifiers
- Integer Options:
- `%d` for signed decimal integers.
- `%i` for integers (signed, similar to %d).
- Floating-Point Options:
- `%f` for fixed-point notation.
- `%e` for scientific notation.
- `%g` automatically chooses between fixed and scientific notation based on the value.
- String Formats:
- `%s` for strings.
Example of Format Specifiers
Here’s a simple demonstration of using multiple format specifiers:
fprintf('Integer: %d, Float: %.2f, String: %s\n', 42, 3.14159, 'Hello World')
This command would output each element formatted according to the specified types.
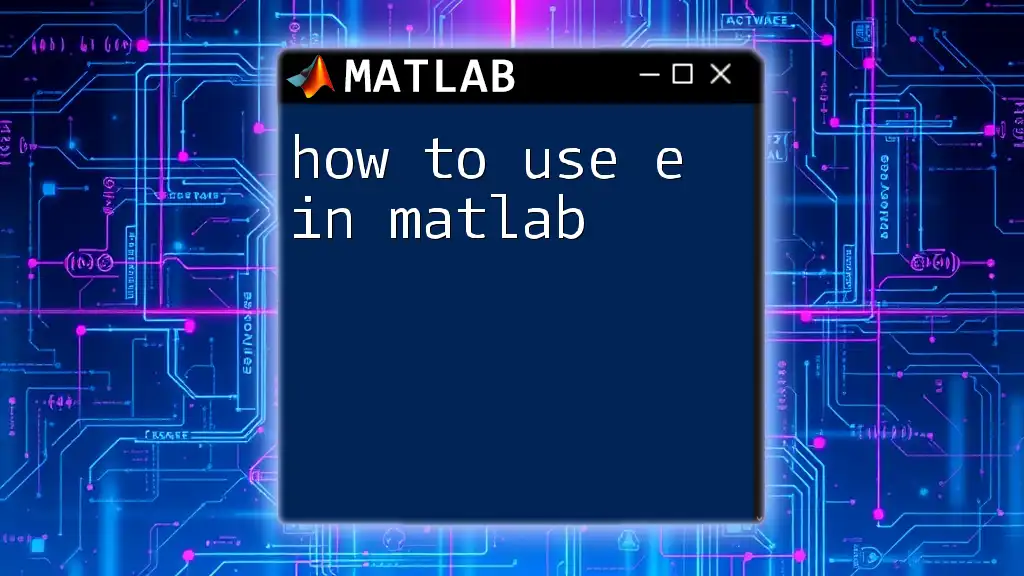
Using `fprintf` in Practice
Printing to the Command Window
Basic Output Example
Using `fprintf` is straightforward for basic text and variable printing. Here's how you can state your name and age positioned naturally in a sentence:
name = 'Alice';
age = 30;
fprintf('My name is %s and I am %d years old.\n', name, age);
The output will read: My name is Alice and I am 30 years old.
Printing Arrays and Matrices
Formatting Arrays
When printing matrices or arrays, `fprintf` can help display the data in an organized manner. Aligning numbers and controlling their precision enhances readability.
A = [1.234, 5.678; 9.012, 3.456];
fprintf('Matrix A:\n');
fprintf('%.2f %.2f\n', A.');
Notice we transpose the matrix with `A.'` to print it row-wise instead of column-wise. Each number will be printed with two decimal places assurance.
Using Escape Sequences
What are Escape Sequences?
Escape sequences are special characters that allow you to manipulate formatting further. They help create new lines, tabs, and other layout adjustments.
Common Escape Sequences
- New line: `\n`
- Tab: `\t`
- Backslash: `\\`
Example with Escapes
Consider this example that illustrates how using escape sequences can structure output:
fprintf('First Line\n\tSecond Line\n');
This output formats the second line under the first with a tab space, enhancing visibility.
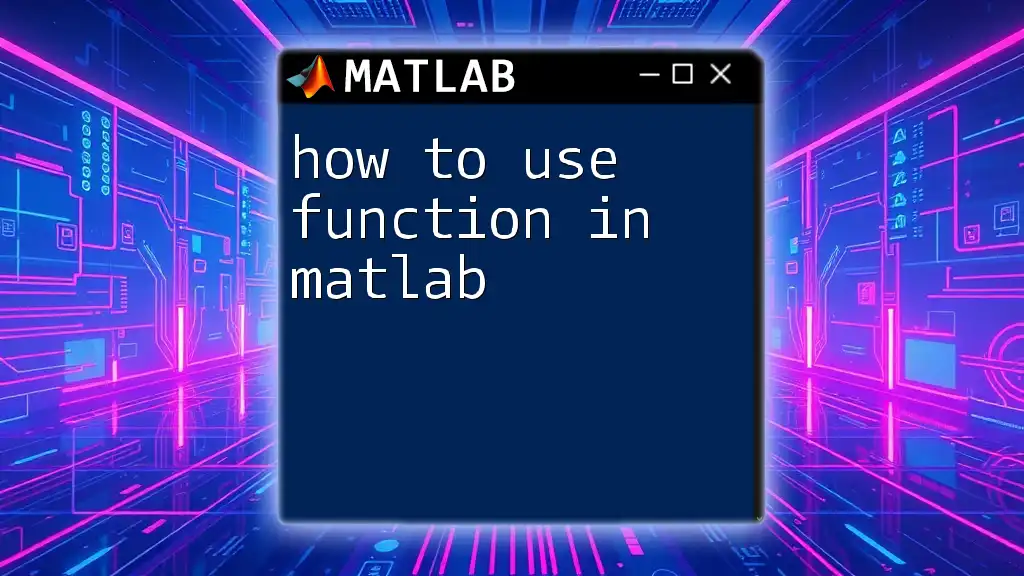
Advanced Formatting Techniques
Specifying Field Width and Precision
Field Width
Field width can be controlled by specifying the width in the format specifier. This allows for organized alignment of print outputs.
Precision Control
Precision allows you to define how many digits should be displayed for floating points.
Example of Field Width and Precision
Here’s a practical example showing both field width and precision:
x = pi;
fprintf('Value of Pi: |%10.4f|\n', x); % 10 characters wide, 4 decimals
This will output the value of Pi padded with spaces, ensuring it aligns correctly when printed in a table or list.
Using `fprintf` with Loops
Looping Through Data
In many scenarios, especially in data processing, you'll want to print data using a loop. This can be effectively done with `fprintf`.
Example with Loop
for i = 1:5
fprintf('Square of %d is %d\n', i, i^2);
end
This loop prints the squares of the numbers 1 through 5, demonstrating how to utilize `fprintf` for repeated outputs.
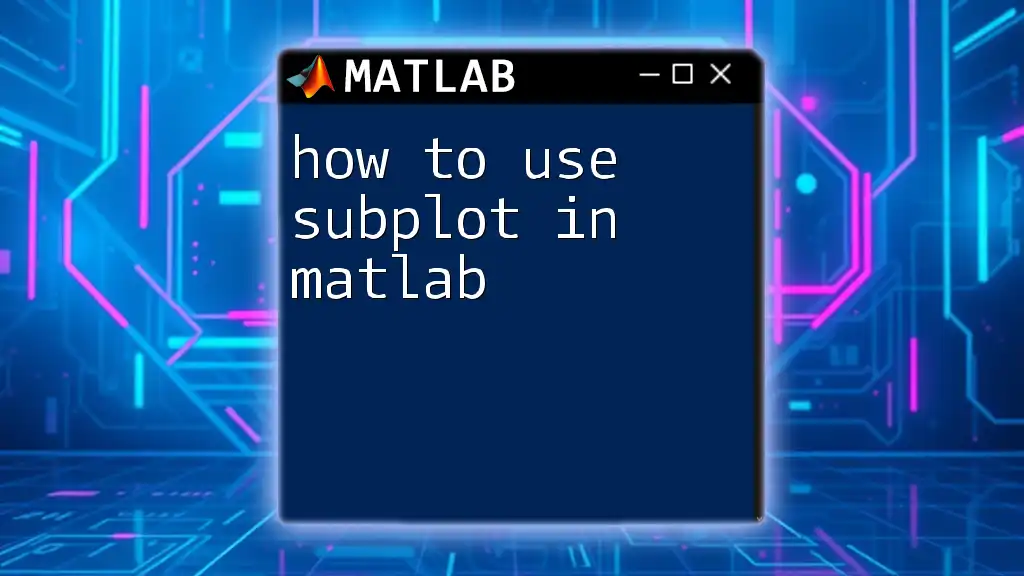
Common Use Cases for `fprintf`
Generating Reports
Creating Formatted Text Reports
One significant use of `fprintf` is to generate structured and well-formatted text reports. This makes it ideal for summarizing results in a readable format.
Example of a Simple Report
fprintf('Date: %s\n', datestr(now));
fprintf('Summary:\n');
fprintf('Average: %.2f\n', mean([1, 2, 3, 4, 5]));
This will generate a small report that outputs the current date and the average of a set of numbers, formatted for clarity.
Debugging with `fprintf`
Using `fprintf` for Debugging Purposes
Debugging often requires checking the value of variables at different stages. Using `fprintf` for this purpose is highly effective.
Example for Debugging
x = rand(1, 5);
for i = 1:length(x)
fprintf('Element %d: %f\n', i, x(i));
end
In this example, `fprintf` prints each element of the array, allowing you to track its value during execution and help in diagnosing errors.
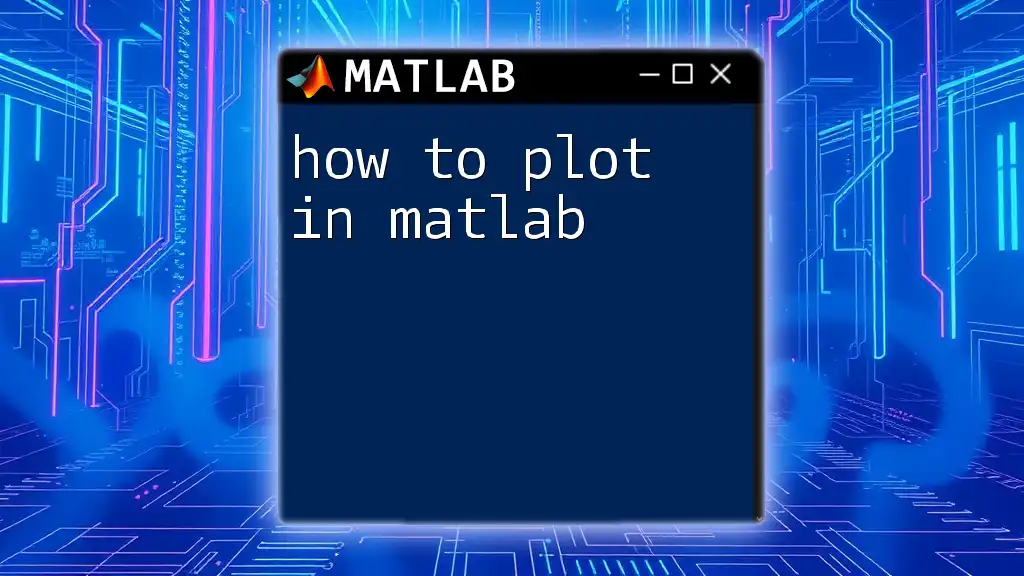
Conclusion
In summary, mastering how to use `fprintf` in MATLAB can significantly enhance your ability to format and present data effectively. Understanding syntax, format specifiers, and advanced techniques equips you with a powerful tool for outputting information in a clear and professional manner. By practicing these techniques, you will become more proficient in MATLAB and improve your coding style.
Do not hesitate to experiment and get creative with your output formatting! Encounter new challenges, and don’t forget to subscribe for more MATLAB tips and tutorials as you continue your journey.
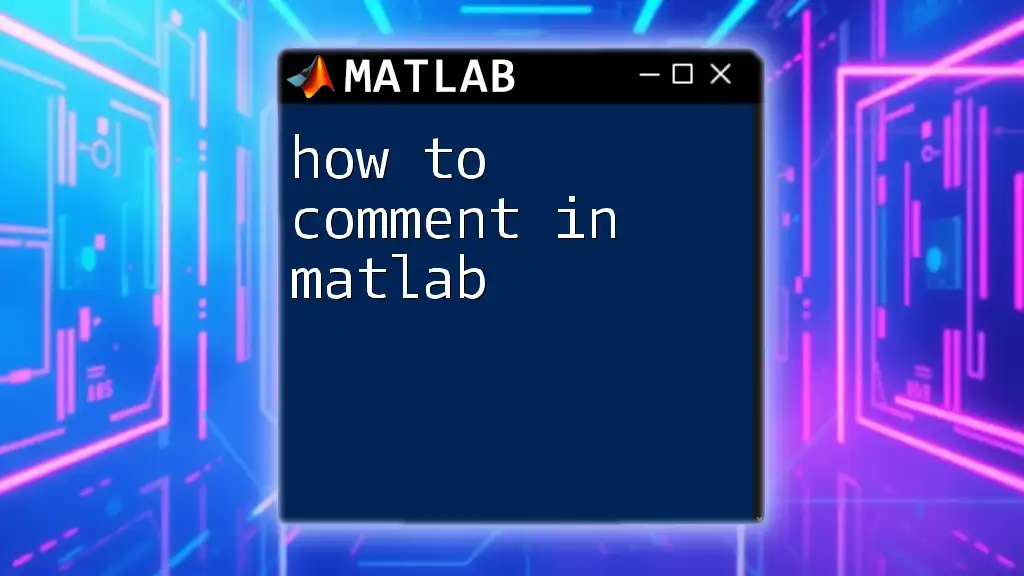
Additional Resources
For further learning, please explore the official MATLAB documentation and recommended tutorials for a deeper dive into `fprintf` and various other MATLAB functions.