To change a number to an array in MATLAB, you can simply use the `ones` function to create an array with the desired size, initialized to the number's value. Here’s a code snippet for clarity:
number = 5; % Example number
array = number * ones(1, 10); % Change number to an array of size 1x10
Understanding Arrays and Scalars in MATLAB
What is a Scalar?
In MATLAB, a scalar is defined as a single numeric value. It can be an integer, a floating-point number, or any other single numeric entity. For instance, if we assign the value 5 to a variable, we have created a scalar:
scalarValue = 5; % This is a scalar.
Scalars are fundamental to calculations in MATLAB but understanding how to manipulate them in array form can significantly enhance data handling.
What is an Array?
In contrast to a scalar, an array in MATLAB consists of multiple elements. These can be arranged in various dimensions:
- 1D arrays or row/column vectors,
- 2D arrays, which form matrices, and
- multi-dimensional arrays for more complex data structures.
For example, a simple array can be created as follows:
arrayValue = [1, 2, 3, 4, 5]; % This is a 1D array.
Each element in the array can be accessed and manipulated individually, making arrays essential for complex calculations and data analysis.

Why Change a Number to an Array?
Learning how to change a number to an array in MATLAB is crucial because:
- It allows for vectorized operations, simplifying the coding process.
- Many MATLAB functions require inputs in the form of arrays.
- It facilitates mathematical operations that involve multiple data points, enabling tasks such as graphing and simulations.
By converting numbers into arrays, users can leverage MATLAB's powerful array operations and functions for efficient data processing.
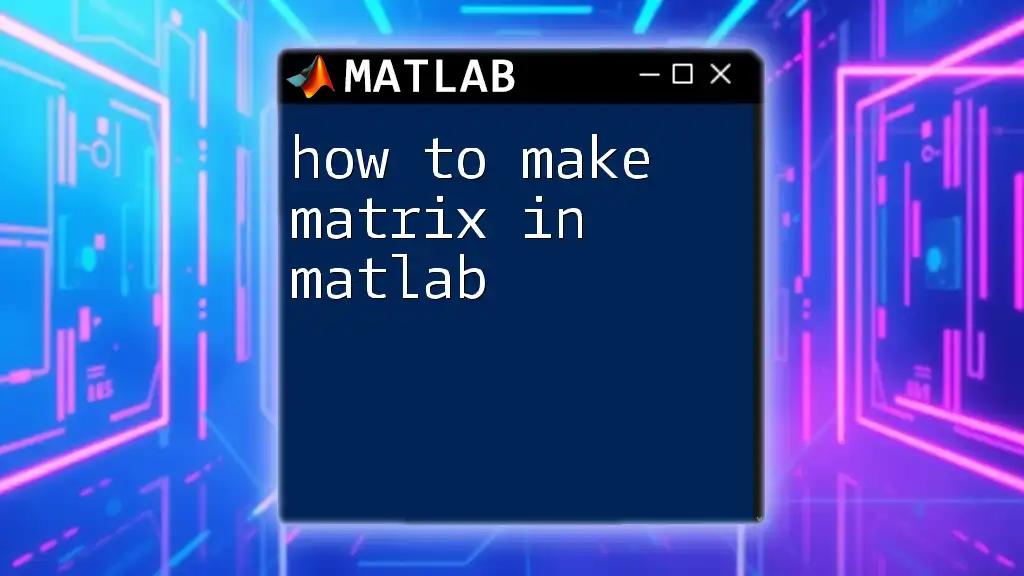
Method 1: Simple Array Conversion
Using Square Brackets
One of the simplest ways to convert a number to an array in MATLAB is by using square brackets. This method creates an array from a single scalar value. For example:
singleNumber = 10;
numberArray = [singleNumber]; % Convert to an array
In this case, `numberArray` now holds a 1D array containing the scalar value. This is particularly useful when you want to treat a scalar as an array for mathematical computations.
Example Scenario
Consider a situation where we want to double the value of a number:
singleNumber = 20;
numberArray = [singleNumber];
doubledArray = numberArray * 2; % Array operation
In this snippet, by converting `singleNumber` into an array, we can easily apply the multiplication operation across all elements in the array, even if it consists of just one number.

Method 2: Using Functions to Create Arrays
Using `zeros`, `ones`, and `linspace`
There are built-in functions in MATLAB that help create arrays based on specific requirements:
- `zeros`: This function creates an array initialized to zero. For instance, you can create a 5x5 array filled with zeros:
num = 5;
zerosArray = zeros(num); % Create a 5x5 array of zeros
- `ones`: Similar to `zeros`, this creates an array initialized to one. For example:
num = 3;
onesArray = ones(num); % Create a 3x3 array of ones
- `linspace`: This creates an array with evenly spaced values, useful for generating ranges. For instance:
startValue = 1;
endValue = 10;
numPoints = 5;
linspaceArray = linspace(startValue, endValue, numPoints); % Creates [1 3.25 5.5 7.75 10]
Example Scenario
Suppose you want to generate an array of numbers that represent a time interval for a simulation. Using `linspace`, you can efficiently create an array that covers all the necessary values:
timeArray = linspace(0, 10, 100); % Creates 100 evenly spaced samples between 0 and 10

Method 3: Using Array Functions
Using `repmat` to Replicate a Number
The `repmat` function is an excellent tool for creating larger arrays from a single numeric scalar. By specifying the dimensions of the desired array, you can easily multiply the scalar into a larger array format. Here’s how you can do it:
singleNumber = 7;
replicatedArray = repmat(singleNumber, 2, 3); % 2 rows and 3 columns
This code snippet forms a 2x3 matrix, where the number 7 is replicated in each position.
Example Scenario
Replicating values often comes in handy in data analysis or simulations. For instance, if you need to initialize a parameter across multiple runs of an experiment, this method is efficient and straightforward.
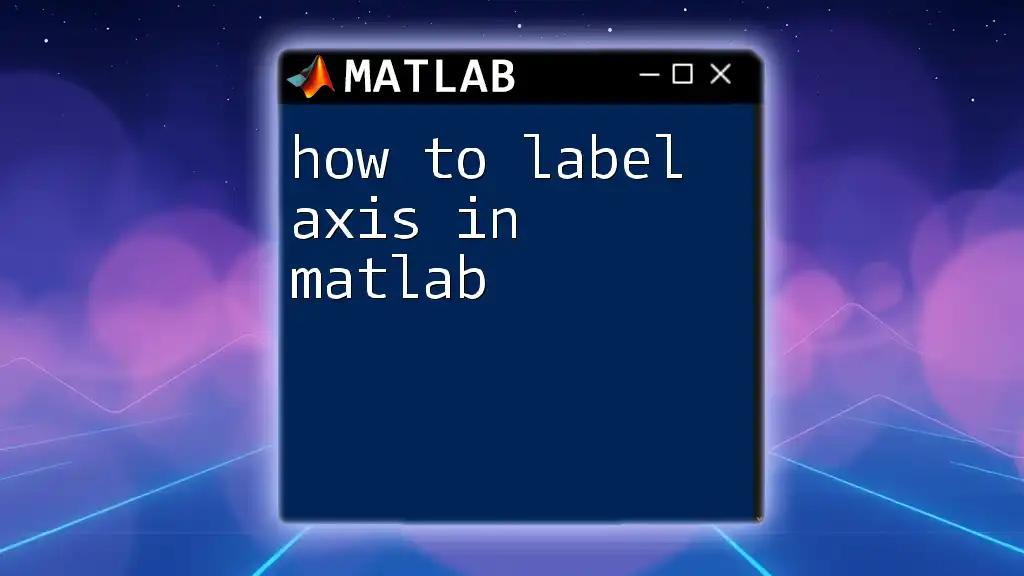
Method 4: Reshaping an Existing Array
Using `reshape`
Another powerful feature in MATLAB is the ability to reshape an existing array into new dimensions. This can enable better organization of data or prepare it for specific calculations. Here's an example:
existingArray = [1, 2, 3, 4, 5];
reshapedArray = reshape(existingArray, [5, 1]); % Convert a row array to column format
The above snippet converts a single row of elements into a column array, improving how the data can be processed in subsequent calculations.
Practical Application
Reshaping data is an essential process when preparing datasets, especially when formatting data for functions that expect certain arrangements of arrays, like linear regression models or image processing functions.
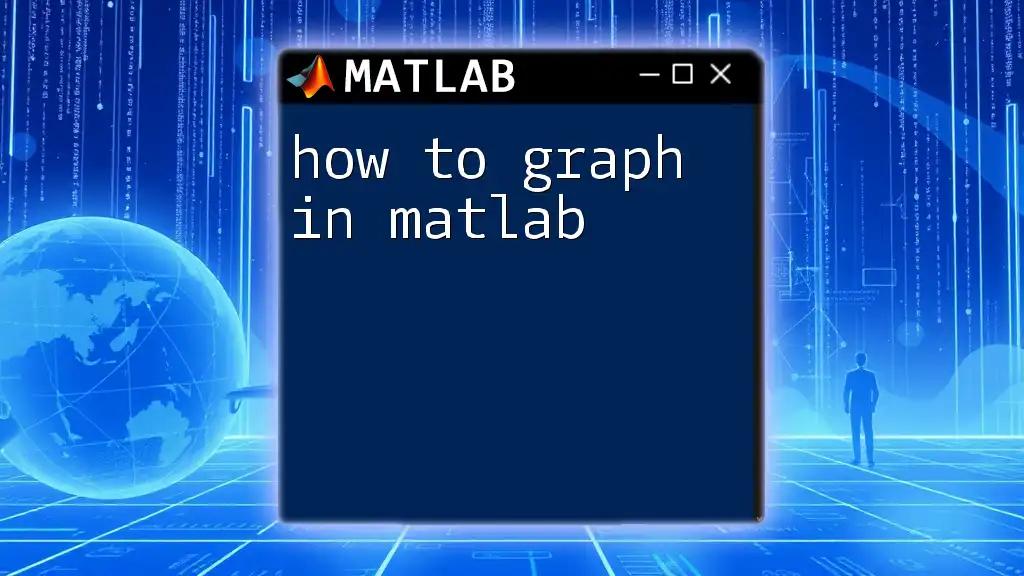
Conclusion
In this guide, we have explored how to change a number to an array in MATLAB, highlighting simple conversion techniques, useful functions, and practical applications. By mastering these methods, you can enhance your MATLAB programming skills and simplify your data manipulation tasks. Don't hesitate to try these techniques in your projects and leverage the flexibility of arrays for your computational needs!
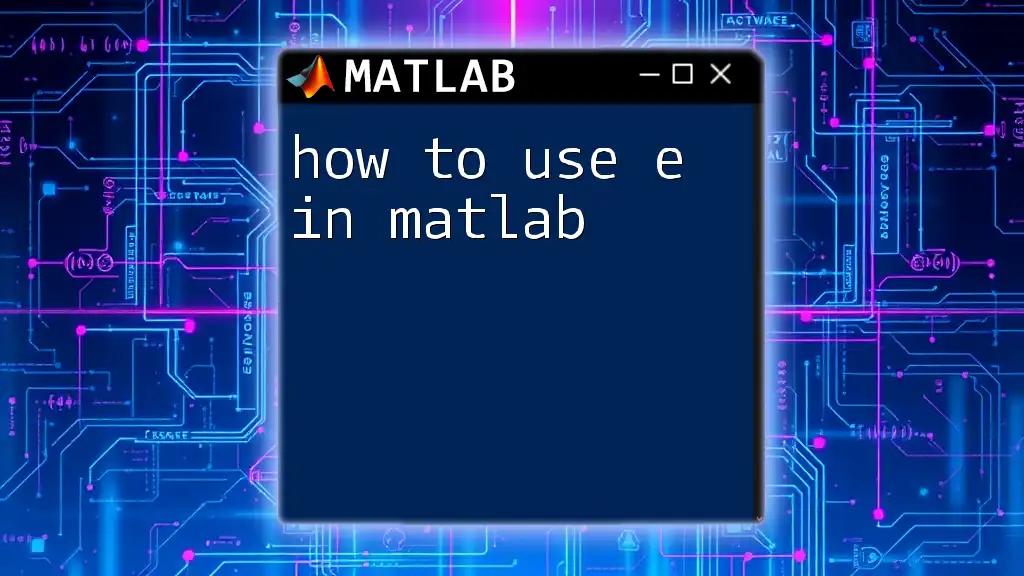
Additional Resources
For further exploration and detailed documentation, consider checking out the official MATLAB documentation and engaging with online communities dedicated to MATLAB enthusiasts.