To create a simple 2D plot in MATLAB, you can use the `plot` function along with vectors representing the x and y coordinates of the points you want to visualize.
x = 0:0.1:10; % Define the x values
y = sin(x); % Calculate the corresponding y values using the sine function
plot(x, y); % Create the plot
xlabel('X-axis'); % Label for X-axis
ylabel('Y-axis'); % Label for Y-axis
title('Plot of Sin Function'); % Title of the plot
grid on; % Display grid on the plot
Getting Started with MATLAB
What is MATLAB?
MATLAB, short for Matrix Laboratory, is a powerful programming environment designed for numerical computing, data analysis, and visualization. It is extensively used by engineers, scientists, and researchers to perform complex calculations and to visualize data through various forms of plotting. Understanding how to plot in MATLAB is crucial because effective visualization can enhance data interpretation and facilitate communication of results.
Setting Up Your Environment
Before diving into plotting, the first step is to ensure that you have MATLAB installed on your computer. After installation, you'll become familiar with the MATLAB interface, which consists of the Command Window, Workspace, and Editor.
The Command Window is where you can type and execute commands directly. The Workspace shows variables you've created, and the Editor is where you can write and save scripts or functions. Mastering these tools will enhance your experience when learning how to plot in MATLAB.
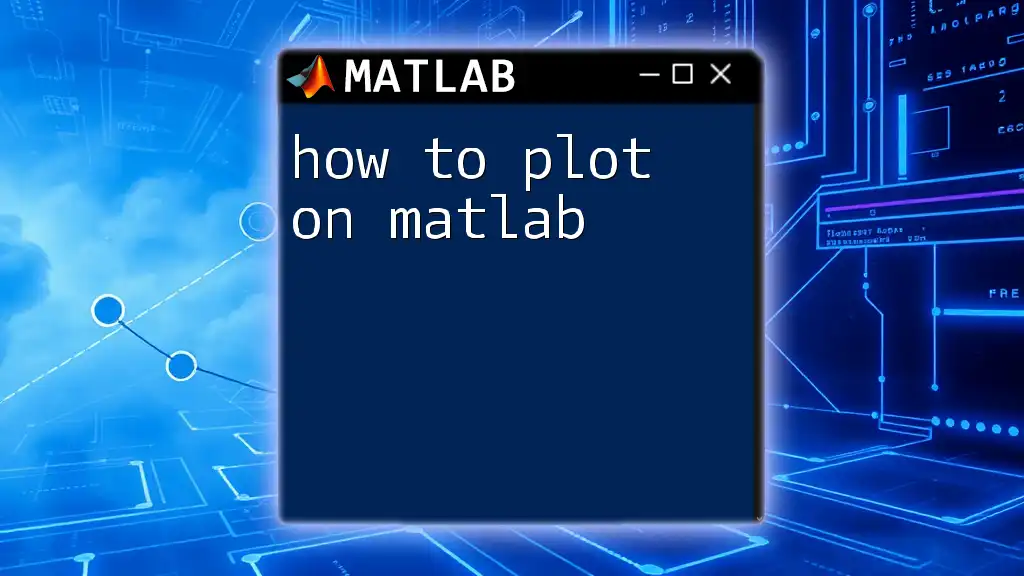
Basic Plotting in MATLAB
Plotting a Simple Line Graph
When learning how to plot in MATLAB, starting with a basic line graph is an excellent first step. The `plot` function creates 2D plots and allows you to visualize the relationship between two variables.
Here's a simple example:
x = 0:0.1:10; % Create a vector from 0 to 10 with a step of 0.1
y = sin(x); % Compute the sine of each element in x
plot(x, y); % Create a 2D line plot
title('Sine Wave'); % Adding a title to your plot
xlabel('X-axis'); % Labeling the X-axis
ylabel('Y-axis'); % Labeling the Y-axis
In this code snippet, we create a vector `x` ranging from 0 to 10 and compute its sine values in `y`. The `plot` function generates the line graph, while `title`, `xlabel`, and `ylabel` enhance readability.
Customizing Your Plots
Changing Line Styles and Colors
Customization is vital for clarity and aesthetics. You can specify line styles, colors, and markers using format specifiers. For instance, if you want to create a red dashed line, you can modify the `plot` function like this:
plot(x, y, 'r--'); % Red dashed line
In this case, `'r--'` specifies that the line should be red and dashed.
Adding Legends and Annotations
To make your plots more informative, adding legends and text annotations is essential. The `legend` function helps identify plotted data, while the `text` function places annotations. Here’s how to do that:
plot(x, y);
legend('Sine Function');
text(7, 0.5, 'Peak'); % Adding labeled text in the plot
In this code, the legend identifies the plotted data, and the `text` function places the word "Peak" at the coordinates (7, 0.5).
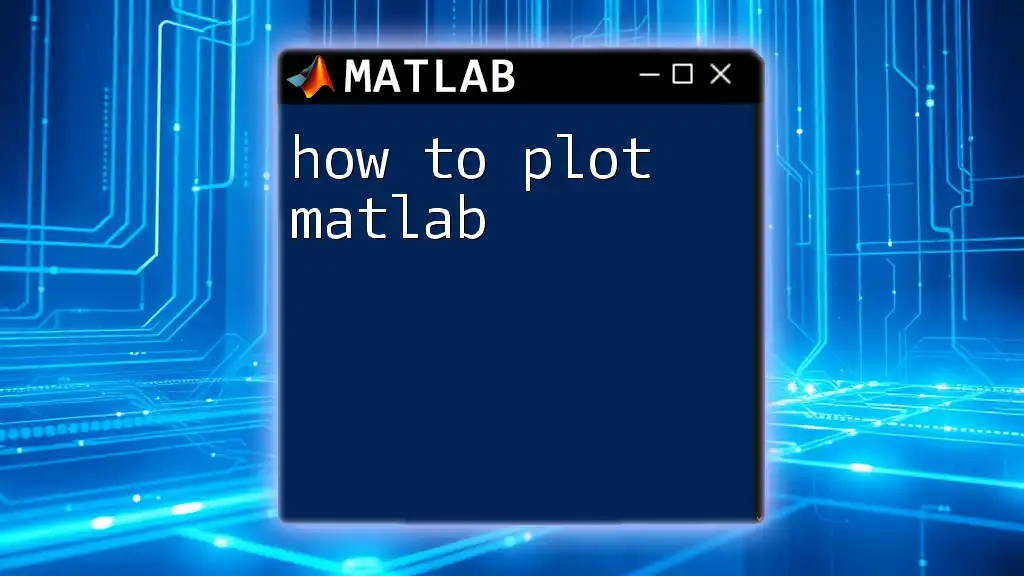
Types of Plots
Line Plots
Line plots are excellent for displaying continuous data, such as trends over time or changes in values across a range. They are fundamental in data visualization and frequently serve as a starting point in many analyses.
Scatter Plots
Scatter plots are used to examine relationships between two variables, showing how much one variable is affected by another. They are particularly useful for spotting correlations or potential outliers. Here’s how to create a scatter plot:
scatter(x, rand(size(x)), 'filled'); % Random y-values
title('Scatter Plot Example');
In this example, the `rand` function generates random y-values, highlighting the independence of the x and y data points.
Bar Graphs
Bar graphs are helpful for comparing categorical data—showing the differences between various groups or categories. Here’s an example of how to create a bar graph in MATLAB:
categories = {'A', 'B', 'C'};
values = [3, 7, 5];
bar(categorical(categories), values);
title('Bar Graph Example');
The `bar` function takes categorical data as the x-values, allowing for easy comparison of each category's value.
Histograms
Histograms play a vital role in visualizing the distribution of a dataset. They are especially useful for understanding the frequency of data points within specified ranges. See the following example:
data = randn(1000, 1); % Generating random data
histogram(data);
title('Histogram Example');
Here, `randn` generates random data from a normal distribution, and `histogram` displays the frequency of values across specified bins.
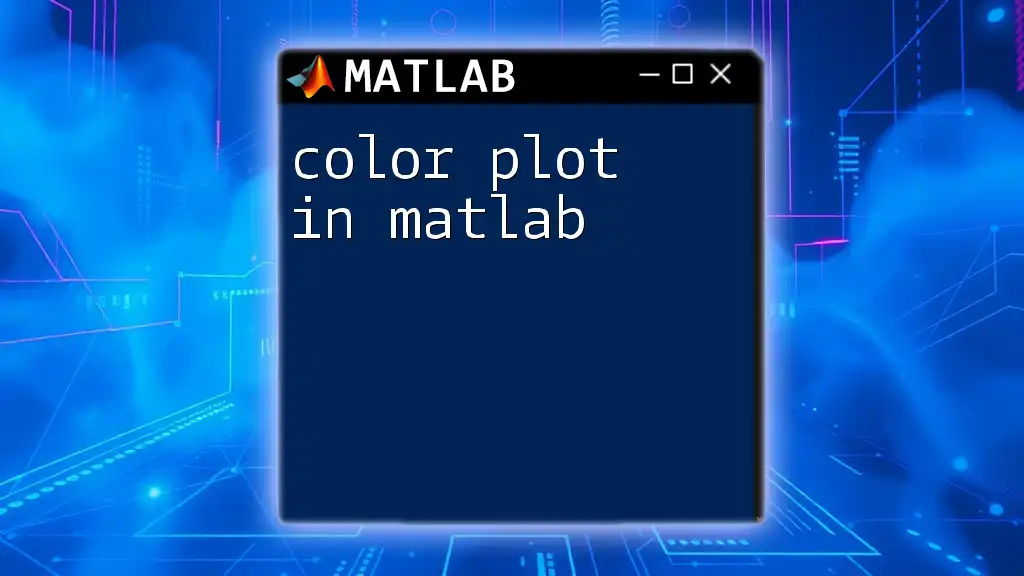
Advanced Plotting Techniques
Combining Multiple Plots
When visualizing more than one dataset, overlaying multiple plots can yield valuable insights. You can use the `hold on` command to keep the current plot active while adding new data. Here’s how:
plot(x, sin(x));
hold on;
plot(x, cos(x));
hold off;
legend('Sine', 'Cosine');
title('Overlap of Sine and Cosine Waves');
This approach demonstrates how different functions can co-exist in one visual, enhancing comparative analysis.
Subplots
Sometimes, presenting multiple plots within a single figure can be valuable. The `subplot` function allows you to arrange plots in a grid format. This is useful for displaying different datasets side by side. Here’s an example:
subplot(2, 1, 1); % Two rows, one column, first plot
plot(x, sin(x));
title('Sine');
subplot(2, 1, 2); % Second plot
plot(x, cos(x));
title('Cosine');
This code generates two vertically stacked plots, allowing easy comparison of the sine and cosine functions.
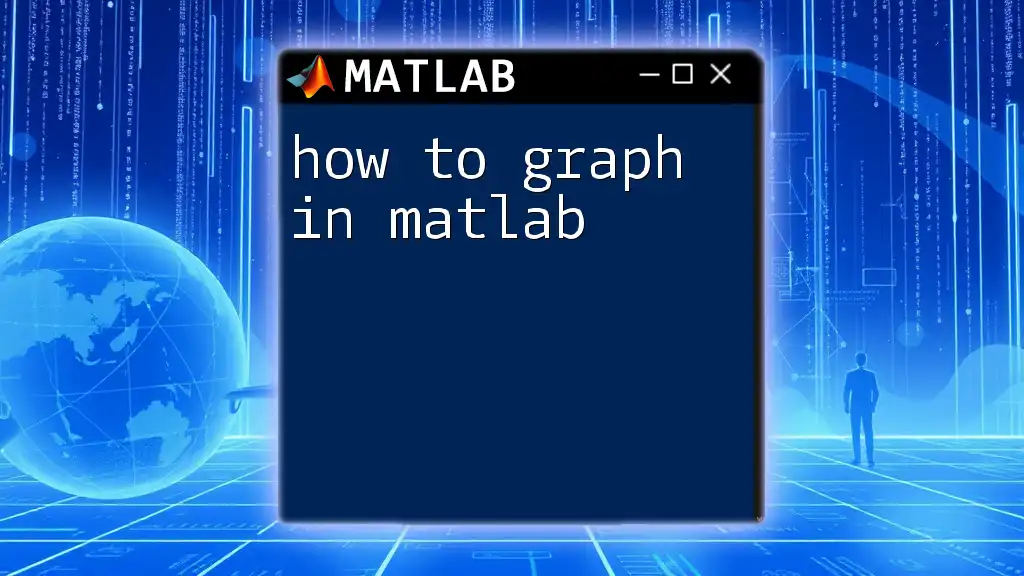
Saving and Exporting Your Plots
Saving as Image Files
Once you have created a visual representation of your data, you might want to save it for reports or presentations. You can easily save MATLAB plots as image files in various formats. Use the following command to save the current figure:
saveas(gcf, 'my_plot.png'); % Save current figure as PNG
This command allows you to specify the filename and format you'd like the plot saved in.
Exporting to Other Formats
Apart from images, MATLAB allows you to export plots in different formats, such as PDF or EPS, making it easy to include high-quality visuals in academic papers or presentations.
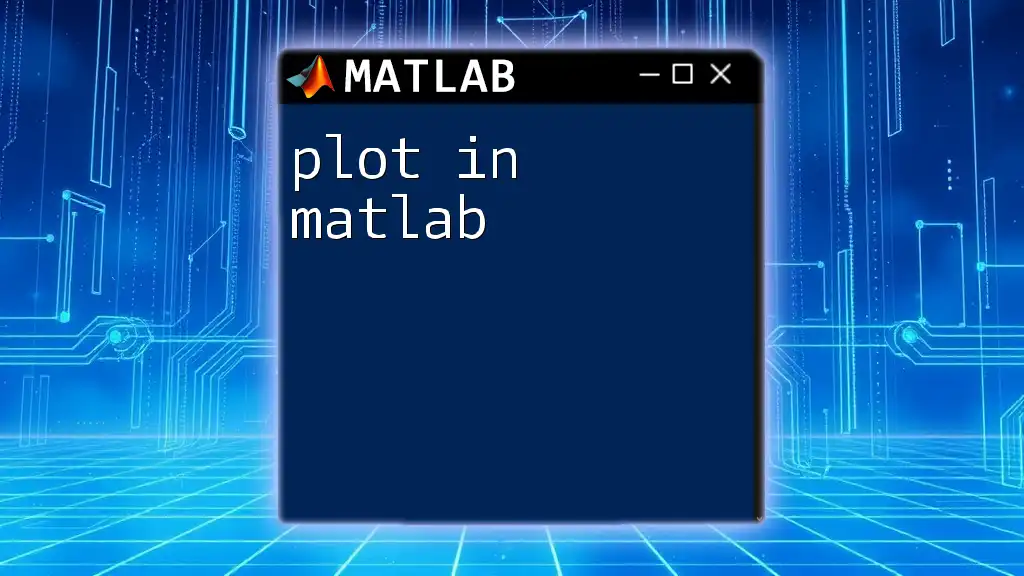
Conclusion
Mastering how to plot in MATLAB opens doors to better data visualization and interpretation. Through various plotting techniques—from simple line graphs to intricate subplots—you can effectively communicate complex data insights. By customizing your visuals with colors, legends, and annotations, you ensure your plots tell a clear story. Encouraging yourself to explore different types of plots and experimenting with the advanced features will make you a proficient MATLAB user, ready to tackle any data visualization challenge.