To graph in MATLAB, you can use the `plot` function to create a simple 2D line graph of your data, as demonstrated in the following code snippet:
x = 0:0.1:10; % Define x data
y = sin(x); % Define y data as the sine of x
plot(x, y); % Create the graph
title('Sine Wave'); % Add a title
xlabel('X-axis'); % Label the x-axis
ylabel('Y-axis'); % Label the y-axis
grid on; % Turn on the grid
Understanding MATLAB Graphing Basics
What is a Graph in MATLAB?
Graphing in MATLAB is a fundamental tool for visual data representation and analysis. It allows users to create various types of graphical displays, such as line graphs, bar charts, scatter plots, and more, to interpret the underlying data more intuitively. Whether you are analyzing scientific data, trends over time, or statistical information, graphical representation can reveal insights that simple numerical listings cannot.
The Graphing Environment
MATLAB provides a robust plotting environment, comprising an intuitive interface and versatile commands that make graph creation straightforward. The MATLAB workspace integrates the capabilities of the command line and graphical user interface, allowing users to interact with datasets and create an array of visualizations seamlessly.
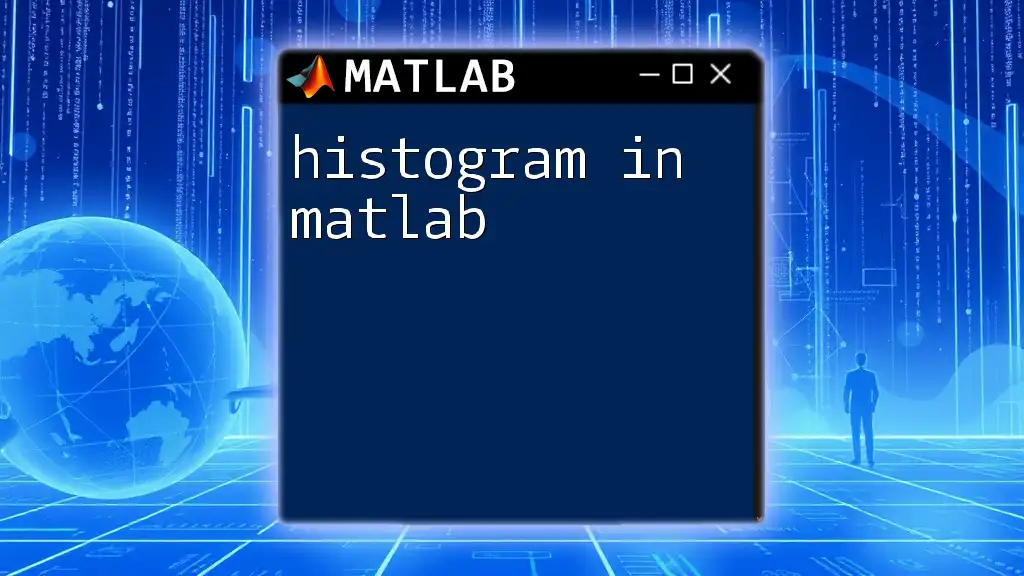
Core MATLAB Graphing Commands
The `plot` Function
The most basic yet powerful command for graphing in MATLAB is the `plot` function. It enables you to create simple 2D line graphs quickly.
To use the `plot` function effectively, you need two sets of data: x (independent variable) and y (dependent variable).
Here’s a basic example to illustrate its usage:
x = 0:0.1:10; % Generate x values from 0 to 10, with step of 0.1
y = sin(x); % Compute y values as sine of x
plot(x, y); % Create a plot of x vs y
xlabel('X-axis'); % Add a label for the x-axis
ylabel('Y-axis'); % Add a label for the y-axis
title('Sine Wave'); % Add a title for the graph
In this example, the command generates a sine wave and labels the axes and the graph for clarity.
Creating Multiple Graphs
Sometimes, you may want to overlay multiple functions on a single graph. The `hold on` and `hold off` commands are crucial here. By using these commands, you can plot multiple datasets without clearing the existing graph.
Here’s how you can overlay sine and cosine waves:
hold on; % Keep the current plot
plot(x, sin(x), 'r'); % Plot sine wave in red
plot(x, cos(x), 'b'); % Plot cosine wave in blue
hold off; % Release the hold on the plot
In this case, the sine wave appears in red, while the cosine wave appears in blue, making comparisons straightforward.
Customizing Graphs
Changing Color, Markers, and Line Styles
The default appearance of graphs in MATLAB can be modified to meet specific presentation needs. You can change colors, markers, and line styles to make your graphs more visually appealing and informative.
For example:
plot(x, sin(x), 'r--o', 'LineWidth', 2, 'MarkerSize', 5);
In this command, the sine wave is plotted with a red dashed line and circle markers. The line width and marker size are also customized, making the graph more readable.
Adding Legends and Text Annotations
Legends are essential for identifying each dataset in your plots. Adding text annotations can also help explain specific features of your graph to your audience.
Example:
legend('Sine Wave', 'Cosine Wave'); % Adds a legend to the plot
You could enhance clarity further by using `text` to place annotations directly on your graph, linking specific data points to explanations.
Adjusting Axes Limits
Setting axis limits allows you to focus on specific data ranges, enhancing the clarity of your graphs.
For instance:
xlim([0 10]); % Set x-axis limits from 0 to 10
ylim([-1 1]); % Set y-axis limits from -1 to 1
This not only helps to zoom in on relevant areas but also improves the overall readability of the graph.
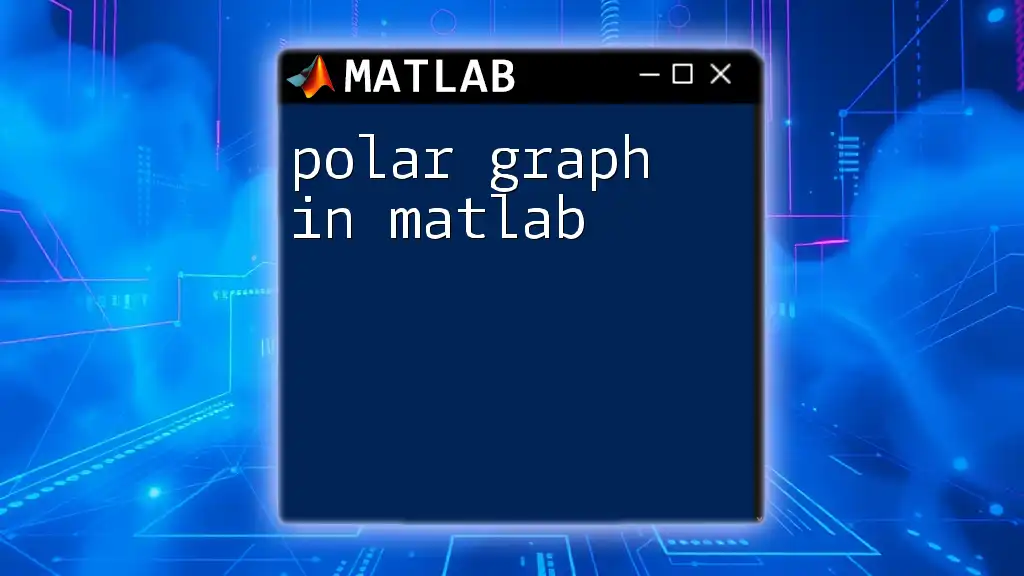
Specialized Graph Types
Bar Graphs
Bar graphs offer a visual representation of categorical data and serve to compare different groups. They stand out in cases where you need to emphasize differences between various categories.
To create a simple bar graph, you can use the following commands:
categories = {'A', 'B', 'C'}; % Define categories
values = [3, 5, 2]; % Corresponding values
bar(categories, values); % Generate a bar graph
This simple representation effectively highlights differences among the categories.
Scatter Plots
Scatter plots are great for examining the relationship between two continuous variables. They visualize data points in a two-dimensional space, providing insights into correlations or distributions.
Here’s how to create a scatter plot in MATLAB:
x = rand(1, 50); % Generate random x values
y = rand(1, 50); % Generate random y values
scatter(x, y, 'filled'); % Create a scatter plot with filled markers
This allows viewers to see how values of x relate to values of y in a compact and visually informative format.
Histograms
Histograms are commonly used to illustrate the distribution of numerical data. They break down large datasets into manageable intervals or "bins," showcasing frequency counts for each bin.
To create a histogram in MATLAB, use the following commands:
data = randn(1, 1000); % Generate normally distributed random data
histogram(data); % Create histogram for the data
This graphical representation helps in identifying the underlying frequency distribution of your dataset.
3D Graphs
For more complex data analysis, 3D graphs are incredibly insightful. Utilizing three-dimensional plots can help visualize relationships among three variables simultaneously.
A simple example of a 3D surface plot is as follows:
[x, y] = meshgrid(-5:0.5:5, -5:0.5:5); % Create a mesh grid for x and y values
z = sin(sqrt(x.^2 + y.^2)); % Compute z values based on x and y
surf(x, y, z); % Generate a 3D surface plot
This creates an appealing visual representation of a three-dimensional sine wave surface, allowing for sophisticated interpretations of multi-variable data.
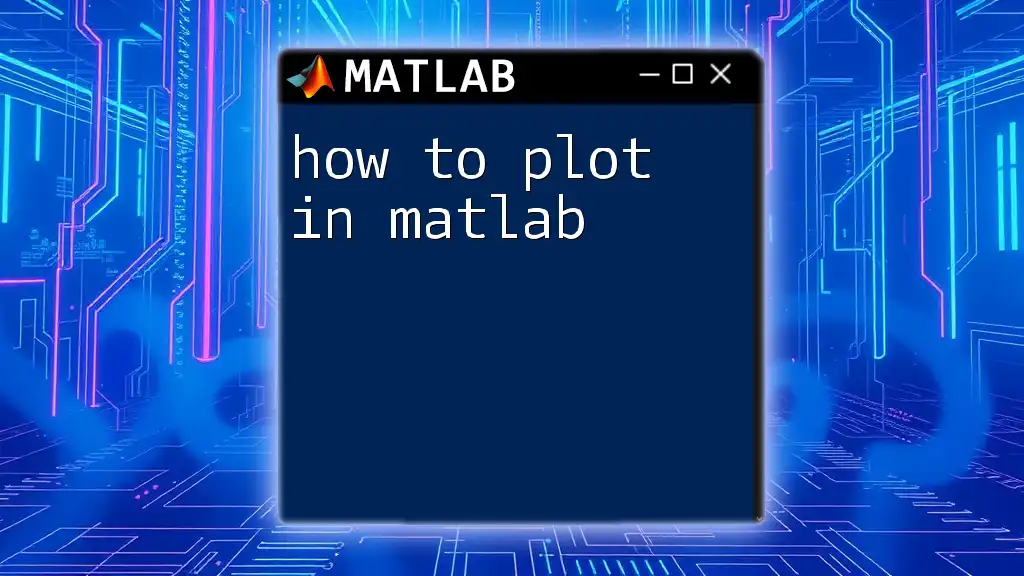
Advanced Graphing Techniques
Subplots
When analyzing different datasets or perspectives simultaneously, subplots can be incredibly useful. They allow you to draw multiple plots in a single figure for better comparisons.
The following example shows how to create subplots:
subplot(2, 1, 1); % Create a subplot grid, 2 rows, 1 column, first subplot
plot(x, sin(x)); % Create a sine wave in the first subplot
title('Sine Wave'); % Add a title to the first subplot
subplot(2, 1, 2); % Move to the second subplot
plot(x, cos(x)); % Create a cosine wave in the second subplot
title('Cosine Wave'); % Add a title to the second subplot
With this configuration, you can present two related functions in a compact and organized visual format.
Importing Data for Graphing
MATLAB provides straightforward commands for importing external data, allowing users to graph datasets collected in various formats, including CSV files.
For example:
data = readtable('data.csv'); % Import data from a CSV file
plot(data.Time, data.Value); % Create a plot using Time and Value columns
This command seamlessly integrates external datasets directly into your workspace, making your analysis more dynamic and relevant.
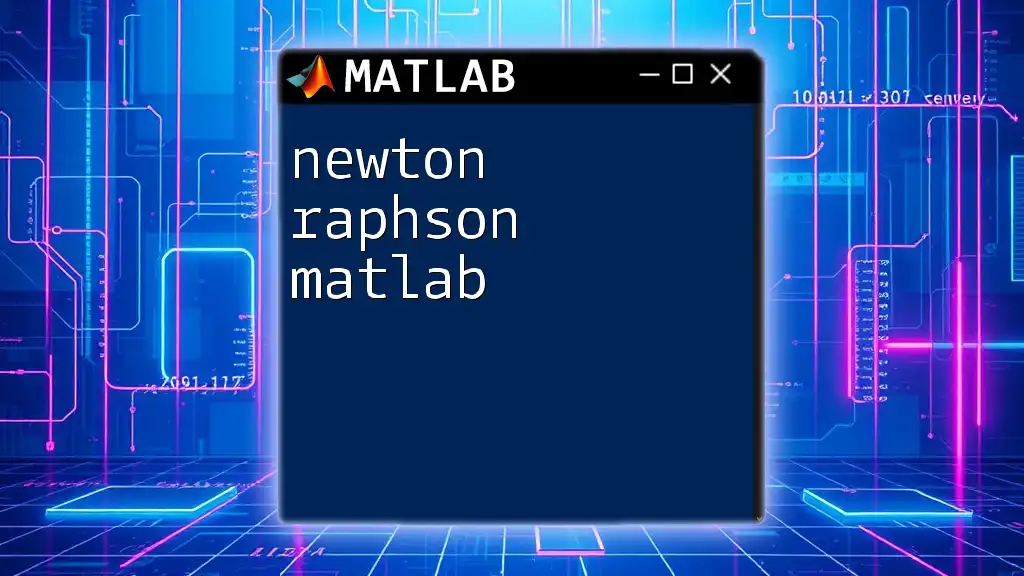
Common Pitfalls and Solutions
Troubleshooting Common Graphing Issues
While creating graphs in MATLAB, beginners might encounter several common issues, such as incorrect data formatting, inappropriate axis scaling, or missing legends. Ensuring that your data is in the correct format and verifying each command step-by-step can help resolve most problems.
Best Practices
Effective graphing in MATLAB revolves around clarity and simplicity. Use contrasting colors for different datasets, ensure labels are easy to read, and maintain consistency in styles across multi-graph setups. Always aim for your graphs to be informative at a glance, allowing viewers to understand complex data with minimal effort.
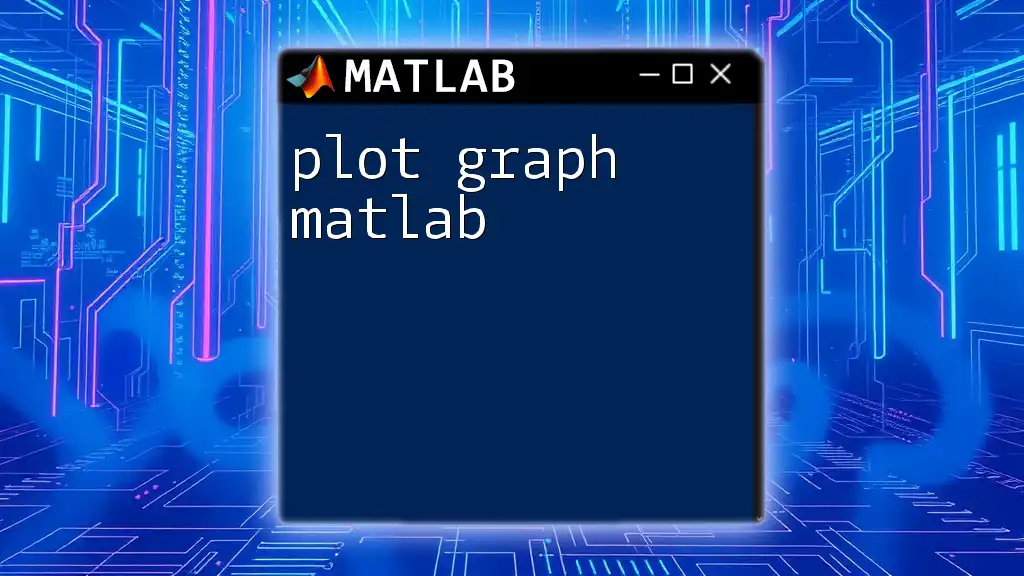
Conclusion
In conclusion, understanding how to graph in MATLAB is a valuable skill that enhances data analysis and presentation. From creating basic plots to advanced 3D representations, mastering MATLAB's graphing capabilities enables clearer insights and improved decision-making. Practice consistently with these commands to enhance your proficiency and confidence in graphing.
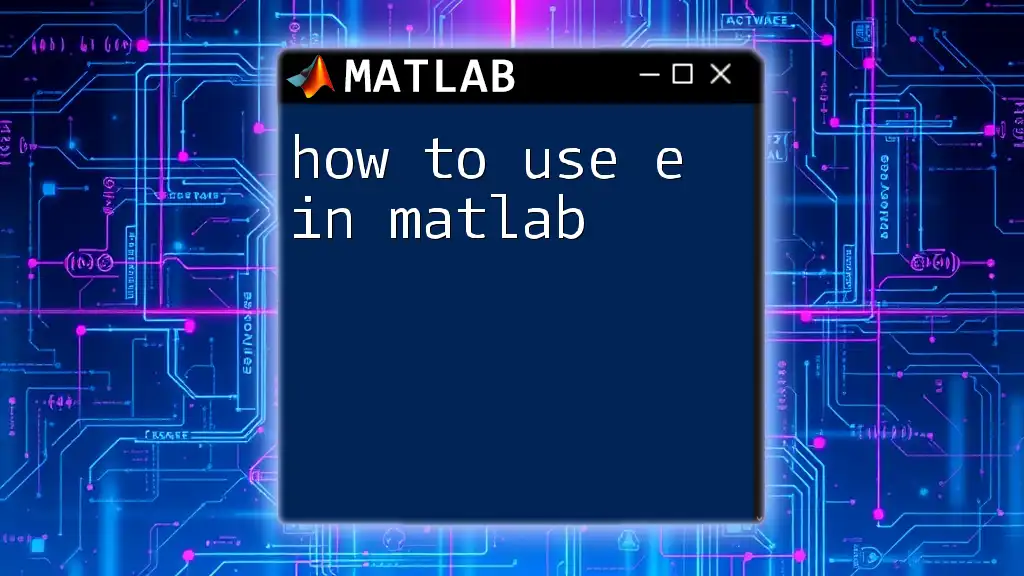
Additional Resources
Recommended Books and Online Courses
To further your learning journey, consider exploring various books and online courses that delve deeper into MATLAB’s functionalities and advanced graphing techniques. Engaging with structured materials will bolster your understanding and application of MATLAB in real-world scenarios.
Community and Support
Don’t hesitate to leverage the MATLAB community through forums, user groups, and online resources. Engaging with fellow users can provide valuable insights, solve problems, and enhance your learning experience as you continue to build your skills in MATLAB.