To convert spacecraft instrument coordinates to planetary coordinates in MATLAB, you can use transformation matrices based on the spacecraft's orientation and the planetary reference frame.
Here's a code snippet demonstrating this transformation:
% Define the spacecraft instrument coordinates
instrument_coords = [x_instrument; y_instrument; z_instrument]; % Replace with your coordinates
% Define the rotation matrix from instrument frame to planetary frame
% This example assumes a simple rotation; modify it as necessary
R = [cos(theta) -sin(theta) 0;
sin(theta) cos(theta) 0;
0 0 1]; % Replace theta with the relevant angle
% Convert to planetary coordinates
planetary_coords = R * instrument_coords;
Understanding Coordinate Systems
What are Spacecraft Instrument Coordinates?
Spacecraft instrument coordinates are the reference frames used by onboard instruments to capture and measure spatial data. These coordinates are typically expressed in a three-dimensional Cartesian format, denoted as \((x, y, z)\). Understanding these coordinates is crucial for tasks such as data collection, navigation, and scientific research in aerospace engineering.
What are Planetary Coordinates?
Planetary coordinates, on the other hand, find their application in capturing the position of points on a celestial body, usually defined in terms of latitude, longitude, and altitude. Latitude informs us how far north or south a point is from the equator, while longitude indicates how far east or west it is from the prime meridian. The altitude provides the height above or below the planetary surface. Converting spacecraft instrument coordinates to planetary coordinates enables scientists to position data accurately within a planetary context, which is invaluable for exploration and research.

Why Convert Coordinates?
The conversion from spacecraft instrument coordinates to planetary coordinates is essential for several reasons:
- Data Analysis: Accurate data interpretation relies on understanding the spatial relationships between measured points and the planet’s surface.
- Navigation and Positioning: Space missions involve navigating spacecraft accurately, which requires reference to planetary coordinates.
- Scientific Research: Many scientific studies, including geology and climatology, depend on correlating instrument data with known geographic locations.
For instance, when a satellite captures imagery over Mars, scientists need to convert these observations from spacecraft instrument coordinates to Martian coordinates to analyze environment changes, geology, or weather patterns effectively.
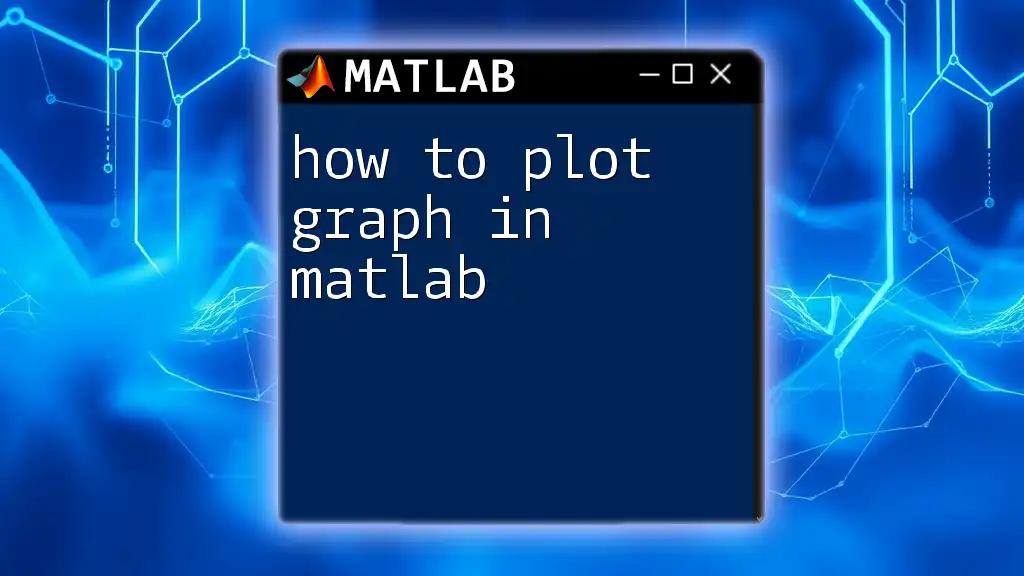
MATLAB Basics for Coordinate Conversion
Setting Up Your MATLAB Environment
Before diving into the code, ensure that your MATLAB environment is prepared for coordinate calculations. Make sure to install the necessary toolboxes, such as the Aerospace Toolbox, which provides essential functions for spatial transformations.
To start, open MATLAB and create a new script file where you will write your code. Getting familiar with MATLAB's editor and command window is crucial for executing commands and troubleshooting.
Common MATLAB Functions for Coordinates
Certain MATLAB functions are tailored for working with coordinate transformations. These include:
- `transformPointsForward`: This function transforms points from one coordinate space to another.
- `transformPointsInverse`: This function does the reverse, converting points back to their original coordinate system.
Here’s how you might use these functions in your code:
% Example of using transformPointsForward
% Define source and destination coordinates
srcCoords = [1, 2, 3; 4, 5, 6];
destCoords = transformPointsForward(srcCoords);
disp(destCoords);
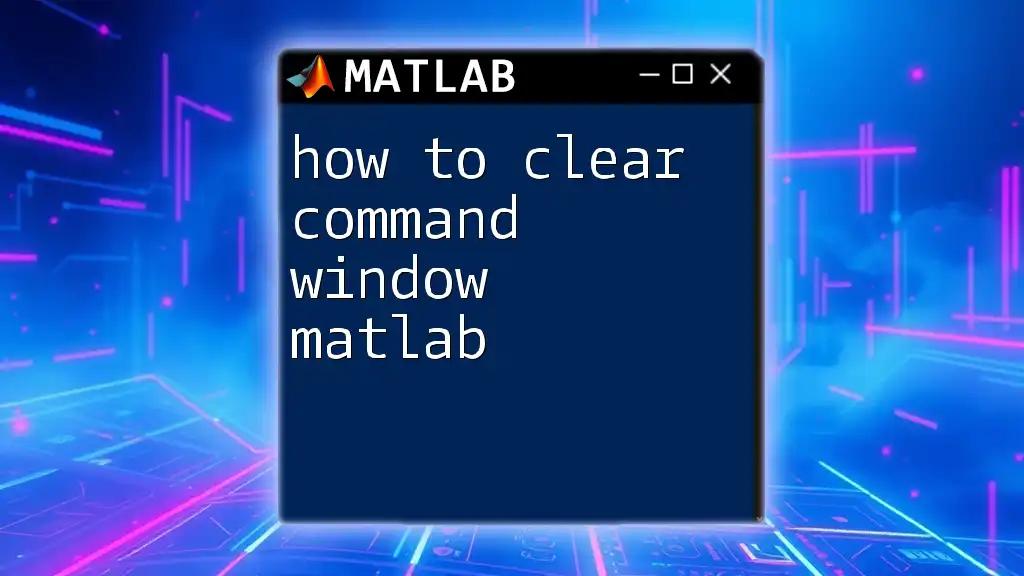
Converting Spacecraft Instrument Coordinates to Planetary Coordinates
Step 1: Representing Your Coordinates
The first essential step is to represent your spacecraft instrument coordinates in MATLAB. This is typically in a matrix or array format, where each row corresponds to a different point in space.
instrumentCoords = [500, 1000, 300]; % Example instrument coordinates
Step 2: Understanding the Transformation Process
To convert the coordinates, you need to apply a transformation that maps the spacecraft’s local coordinate frame to the planetary coordinate system. This involves converting Cartesian coordinates \((x, y, z)\) to spherical coordinates related to the planet’s surface.
You will need equations for spherical coordinates:
- Latitude: \(\phi = \arctan\left(\frac{z}{\sqrt{x^2 + y^2}}\right)\)
- Longitude: \(\lambda = \arctan\left(\frac{y}{x}\right)\)
- Altitude: This can often be calculated by the radial distance minus the planetary radius.
Step 3: Implementing the Transformation in MATLAB
Preparing the Transformation Algorithm
Next, prepare a MATLAB function that implements these transformations. Here’s a simple skeleton of that function:
function planetaryCoords = transformCoords(instrumentCoords)
% Extract XYZ coordinates
x = instrumentCoords(1);
y = instrumentCoords(2);
z = instrumentCoords(3);
% Calculate latitude, longitude, and altitude
latitude = atan2(z, sqrt(x^2 + y^2)); % lat in radians
longitude = atan2(y, x); % lon in radians
altitude = sqrt(x^2 + y^2 + z^2) - planetaryRadius; % Adjust for the planet's radius
% Return in a structured format
planetaryCoords = [latitude, longitude, altitude];
end
Example 1: Converting Simple Coordinates
Let’s consider an example where you need to convert a set of instrument coordinates that represent a point in space.
% Given instrument coordinates
instrumentCoords = [500, 1000, 300];
% Call the transformation function
planetaryCoords = transformCoords(instrumentCoords);
disp(planetaryCoords);
This code will display the transformed coordinates, thus allowing you to verify the conversion.
Step 4: Validating the Results
Once you have your planetary coordinates, it's vital to validate these results. One effective way to do this is through visualization. By plotting the converted coordinates, you can compare them against known values or a planetary map.
% Example of plotting the converted coordinates
figure;
plot3(planetaryCoords(1), planetaryCoords(2), planetaryCoords(3), 'o');
title('Converted Planetary Coordinates');
xlabel('Latitude');
ylabel('Longitude');
zlabel('Altitude');
This plot provides insight into the distribution of converted points and helps in confirming the accuracy of your transformations.
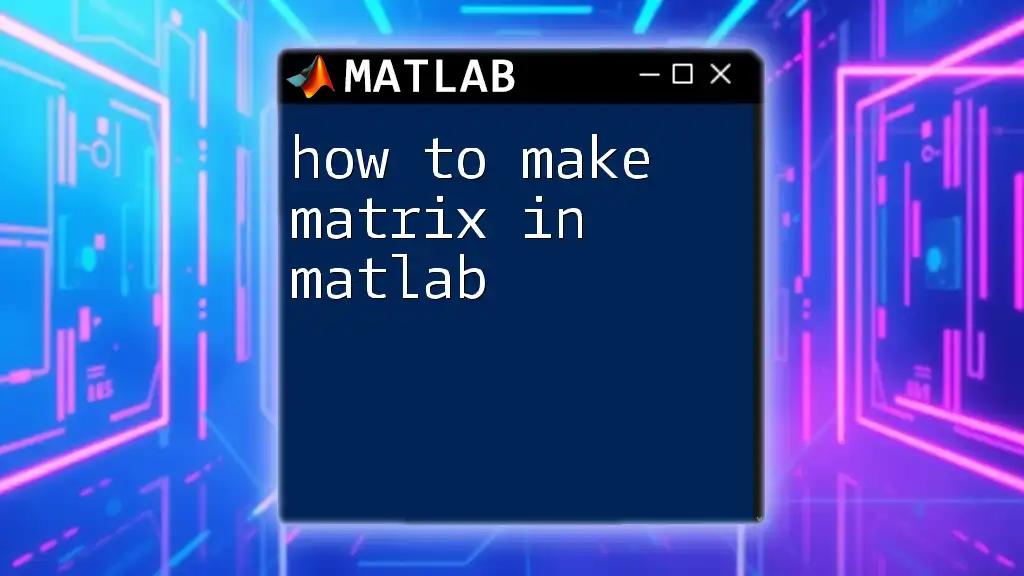
Advanced Techniques and Functions
Using Built-In MATLAB Functions
Leveraging MATLAB’s built-in functions can simplify complex conversions. After establishing your coordinate data, consider using `geodetic2ecef`, which translates geodetic coordinates directly into Earth-Centered, Earth-Fixed (ECEF) coordinates without manual calculations.
Handling Edge Cases
While working with coordinate transformations, be aware of potential issues such as:
- Singularity Points: Points very close to the poles may lead to inaccurate results in the coordinate conversion.
- Input Errors: Ensure that the input coordinates vary in their values; unexpected or extreme values can cause computational errors.
To troubleshoot issues efficiently, include error-checking mechanisms in your code to validate input ranges before attempting transformations.

Conclusion
Accurately converting spacecraft instrument coordinates to planetary coordinates in MATLAB is a fundamental skill in aerospace engineering and planetary science. By following the above steps, utilizing the provided code snippets, and understanding the underlying transformations, you can effectively interpret and analyze spatial data from various missions. Mastering this implementation not only highlights the significance of MATLAB commands but also strengthens your analytical capabilities in navigating the complexities of space data.

Further Resources
For those eager to deepen their understanding of MATLAB and coordinate systems, consider exploring MATLAB's official documentation and tutorials. They provide extensive resources on the capabilities of different MATLAB functions and tools specific to aerospace and geography.
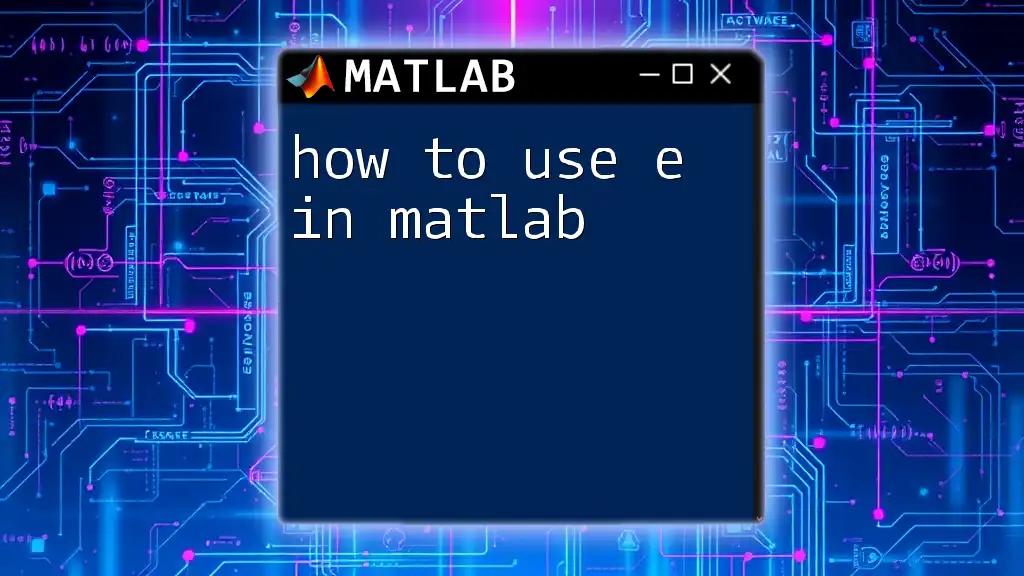
Call to Action
Try implementing the techniques discussed in this article, experiment with the code provided, and share your findings with your peers. For more tutorials on how to convert spacecraft instrument coordinates to planetary coordinates in MATLAB and similar topics, subscribe to our platform and stay updated!