In MATLAB, the `intersect` function returns the common elements between two arrays, allowing for easy identification of shared values.
A = [1, 2, 3, 4, 5];
B = [3, 4, 5, 6, 7];
common_elements = intersect(A, B);
Understanding Intersection Concepts
Definition of Intersection
In mathematical terms, an intersection refers to the common elements between two sets. When dealing with functions or geometric entities, it signifies the point(s) where they overlap. Understanding intersections is crucial in various fields, including computer graphics, optimization, and data analysis, as it allows for solving complex problems involving relationships between different quantities.
Types of Intersections
Intersection can take various forms:
- Set Intersection: This concerns the common elements in two or more sets. For instance, given sets A and B, their intersection includes all elements found in both A and B.
- Geometric Intersection: This refers to the points at which geometric figures, such as lines or shapes, meet or overlap.
- Line Intersection: In coordinate geometry, line intersection is the point where the graphs of two lines cross.
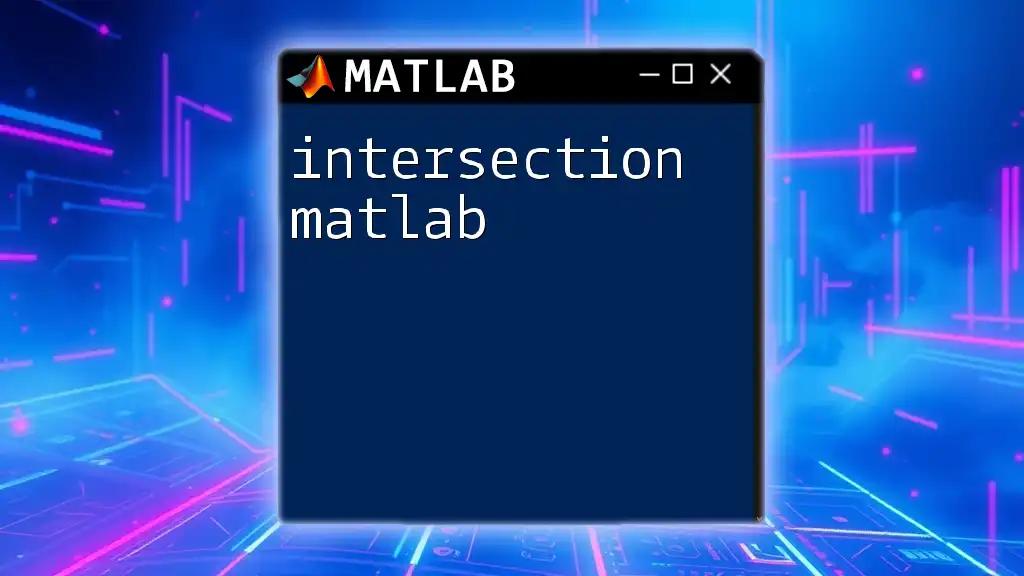
Using MATLAB for Set Intersections
Built-in Functions for Set Operations
MATLAB provides several built-in functions essential for executing set operations. The key functions include:
- `intersect()`: Finds common values in arrays.
- `union()`: Combines arrays while removing duplicates.
- `setdiff()`: Returns values in one array that are not present in another.
Syntax of the `intersect()` Function
The basic syntax for the `intersect()` function is:
[C, IA, IB] = intersect(A, B)
Here, A and B are the two arrays, C is the output array containing the common elements, IA contains the indices of the common elements from A, and IB contains the indices from B.
Examples of Set Intersection
Example 1: Basic Set Intersection
To illustrate how to use the `intersect()` function, consider the following example:
A = [1, 2, 3, 4];
B = [3, 4, 5, 6];
C = intersect(A, B);
disp(C); % Output: [3, 4]
In this case, the output shows the common elements 3 and 4, demonstrating how `intersect()` efficiently retrieves shared values between two arrays.
Example 2: Set Intersection with Duplicate Values
MATLAB handles duplicate values intuitively within sets. The following code snippet showcases this:
A = [1, 2, 2, 3, 4];
B = [2, 3, 3, 5, 6];
C = intersect(A, B);
disp(C); % Output: [2, 3]
Here, even though 2 and 3 appear multiple times, the function returns each unique common element only once, emphasizing MATLAB's ability to streamline operations on sets.
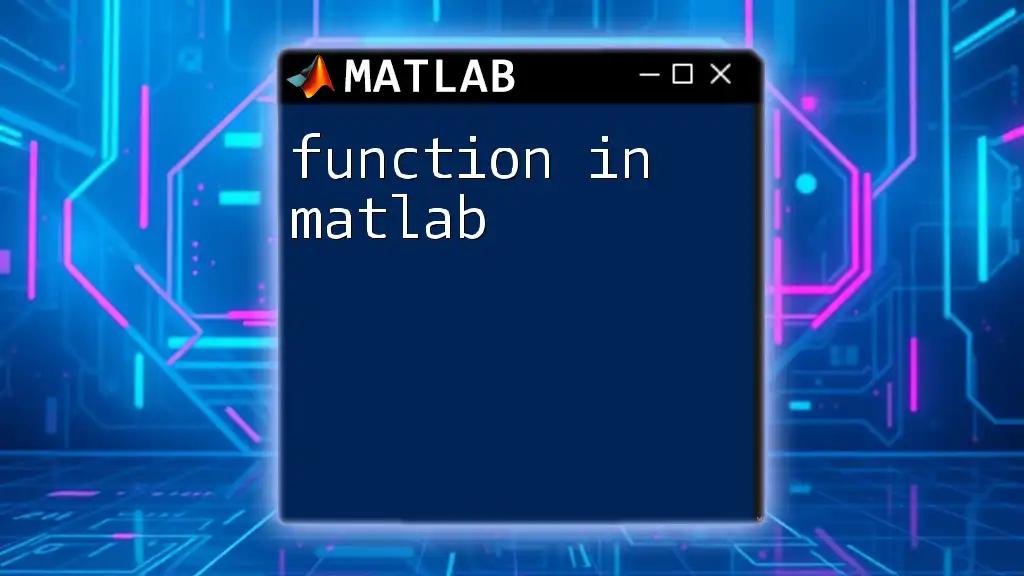
Intersection in Geometrical Contexts
Line Intersections
In geometry, the intersection of two lines can occur at one point, no points (parallel), or infinitely many points (overlapping lines). To find the intersection mathematically, one often solves the equations representing the lines.
MATLAB Techniques for Line Intersection
Example 1: Finding Intersection of Two Lines
Suppose we want to find the intersection point of the lines defined by the equations y = 2x + 1 and y = -1*x + 4. This can be executed as follows:
x = linspace(-10, 10, 100);
y1 = 2*x + 1;
y2 = -1*x + 4;
% Find intersection points
eq1 = @(x) 2*x + 1;
eq2 = @(x) -1*x + 4;
intersectionX = fsolve(@(x) eq1(x) - eq2(x), 0);
intersectionY = eq1(intersectionX);
plot(x, y1, x, y2);
hold on;
plot(intersectionX, intersectionY, 'ro');
title('Line Intersection');
This code plots the two lines and uses the `fsolve` function to numerically compute their intersection point, indicated on the plot with a red circle. Such graphical interpretation not only illustrates the outcome but reinforces the concept of intersection in a visual format.
Intersection of Geometric Shapes
In a broader sense, intersections can also involve geometric figures like circles or polygons. Methods for finding the intersection points among shapes typically involve solving polynomial equations.
Example: Circle Intersection
To demonstrate the intersection of two circles, we can represent the circles as follows:
theta = linspace(0, 2*pi, 100);
x1 = 1 + cos(theta); % Circle 1 centered at (1, 0)
y1 = sin(theta);
x2 = 3 + cos(theta); % Circle 2 centered at (3, 0)
y2 = sin(theta);
figure;
plot(x1, y1, 'r', x2, y2, 'b');
This code provides a framework to plot two circles. The next analytical step would involve calculating the intersection points, usually a more complex operation involving system equations or numerical approaches.
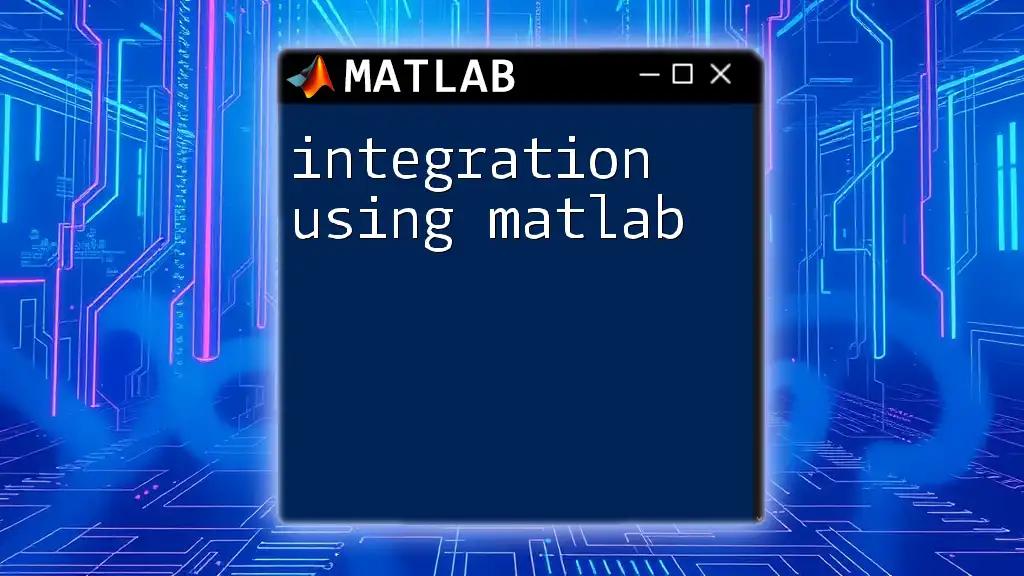
Advanced Intersection Techniques
Numerical Methods for Finding Intersections
MATLAB allows for advanced computations involving intersections through numerical methods. Techniques like Newton's method can be applied when analytical solutions are tedious or impractical. Using numerical approaches can significantly enhance the efficiency of finding intersection points, especially in complex datasets or higher dimensions.
Usage of Optimization Toolbox
MATLAB's Optimization Toolbox provides additional tools for tackling intersection problems more effectively. When dealing with non-linear equations or multiple variables, the optimization toolbox can help find the points of intersection through iterative algorithms, enhancing the user’s ability to solve complex mathematical challenges.
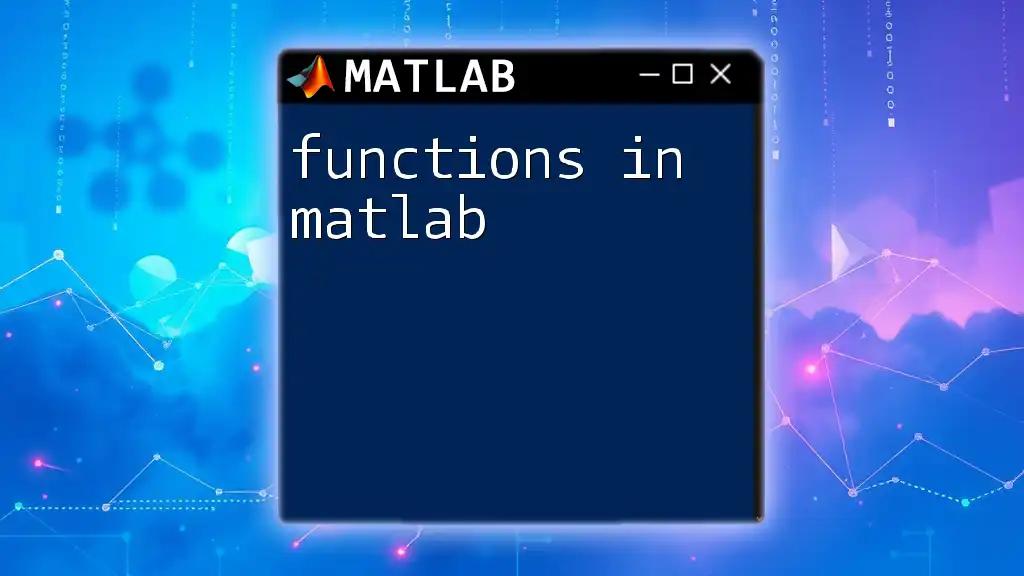
Conclusion
Understanding how to manipulate and calculate intersections in MATLAB is a vital skill for anyone looking to harness the full power of this programming environment. From basic set operations using the `intersect()` function to solving complex geometrical problems, MATLAB offers a robust framework for exploring intersection concepts.
By becoming proficient with these techniques, users can increase their programming capabilities and apply them to more advanced problems. Mastering intersections in MATLAB not only improves computational efficiency but also lays a foundation for tackling more intricate mathematical concepts and applications.
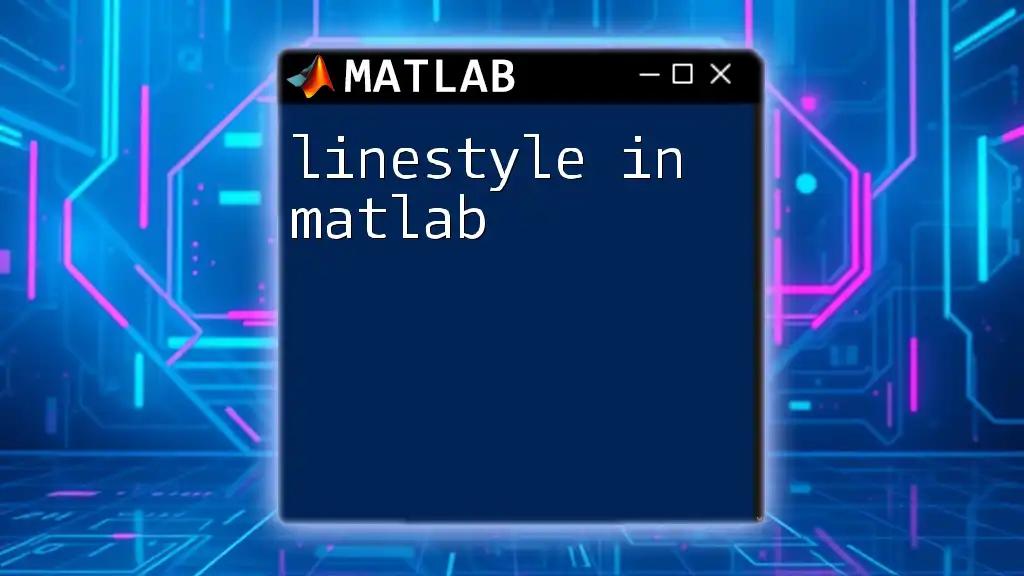
Additional Resources
To further your understanding, consider exploring MATLAB's extensive documentation, online courses, and community forums dedicated to problem-solving in MATLAB. This journey through the world of intersections in MATLAB is just the beginning of your exploration into the endless possibilities offered by this powerful tool.
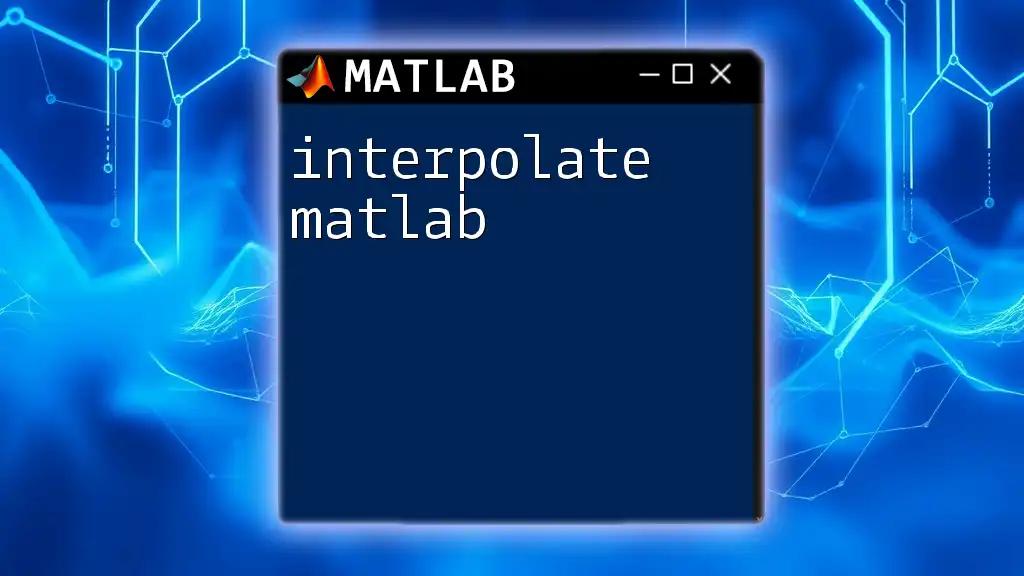
Call to Action
If you have specific intersection problems you'd like assistance with or if you want to join our upcoming workshops focusing on practical MATLAB techniques, feel free to reach out! Together, we can deepen your knowledge and proficiency in MATLAB commands and concepts.