The inverse cosine function in MATLAB can be calculated using the `acos` command, which returns the angle in radians whose cosine is the specified value.
Here's a code snippet demonstrating the usage:
% Calculate the inverse cosine of 0.5
angle_rad = acos(0.5);
disp(angle_rad); % Display the angle in radians
Understanding Inverse Cosine
What is Inverse Cosine?
The inverse cosine, commonly known as the arc cosine, is a mathematical function that returns the angle whose cosine is equal to a specified number. Simply put, if you have a known cosine value, the inverse cosine allows you to find the corresponding angle in a right triangle.
Importance of Inverse Cosine in Mathematics
The significance of the inverse cosine extends beyond simple trigonometry. Here are some key areas where it plays a vital role:
-
Applications in Trigonometry: It helps determine angles for a variety of geometrical problems and is foundational for solving triangles.
-
Real-World Applications: It is widely used in fields such as engineering, physics, and computer graphics, where angle calculations are crucial for design and analysis.
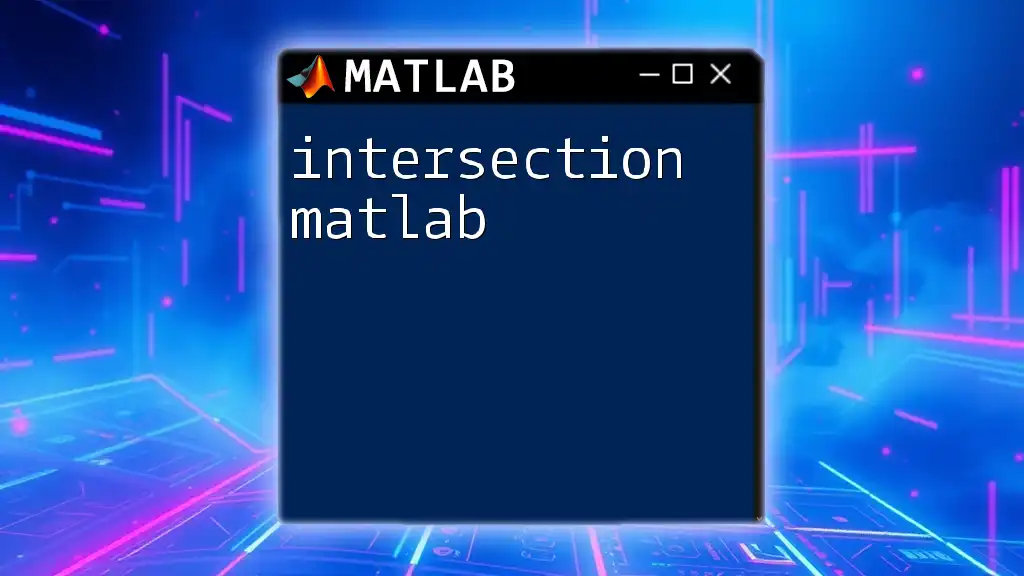
The `acos` Function in MATLAB
Syntax of the `acos` Function
In MATLAB, the inverse cosine function is implemented using the `acos` function, which follows this syntax:
Y = acos(X)
In this function:
- Y is the output variable that stores the angle in radians.
- X is the input value that must lie within the range of -1 to 1, as these are the only valid inputs for cosine.
Input Constraints
Valid Range for Input X
To ensure the function works correctly, it is essential to input values solely within the interval [-1, 1]. Any attempts to input values outside this range will result in either complex outputs or an error, indicating an invalid operation.
Output Format
Units of Output
The `acos` function by default returns angles in radians. To make the results more interpretable, especially in applications where degrees are more common, you may need to convert radians to degrees thereafter.
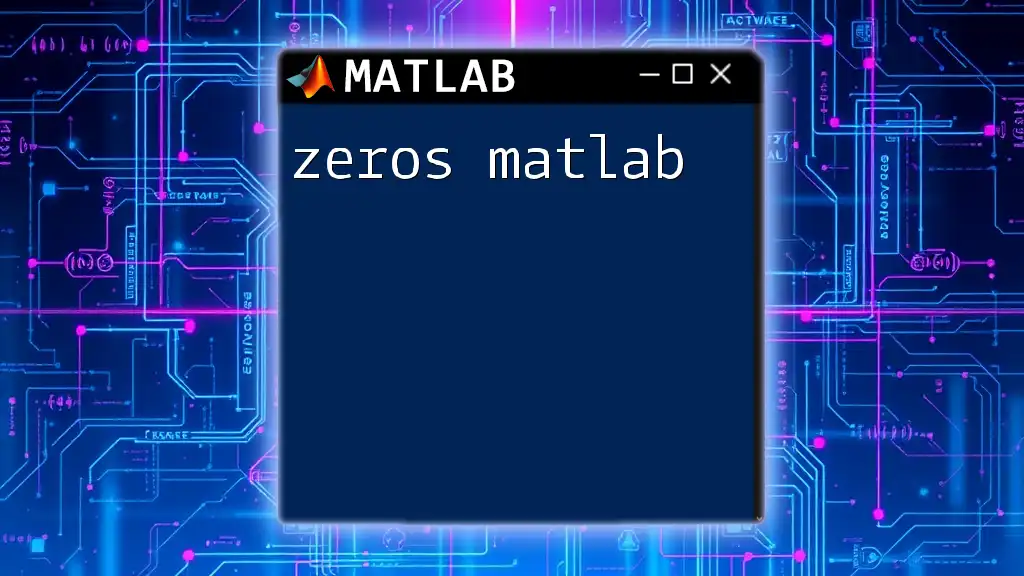
Examples of Using `acos` in MATLAB
Basic Example
To demonstrate the functionality of the `acos` function, consider a simple example:
angle = acos(0.5)
Here, the value of `angle` should return approximately 1.0472 radians, which represents π/3 radians (or 60 degrees).
Using `acos` with Vectors
You can also apply the `acos` function to arrays or vectors. For instance:
x = [-1, 0, 0.5, 1];
y = acos(x)
This example illustrates how the `acos` function can yield a vector of angles corresponding to the cosine values in array x. The output y will be an array of angles in radians.
Converting Radians to Degrees
Often, you may need the angle in degrees instead of radians. To convert radians to degrees, you can use MATLAB's built-in function `rad2deg`:
angle_degrees = rad2deg(angle)
This will provide a clearer understanding of the angle, particularly in contexts where degrees are the norm (e.g., navigation, map readings).
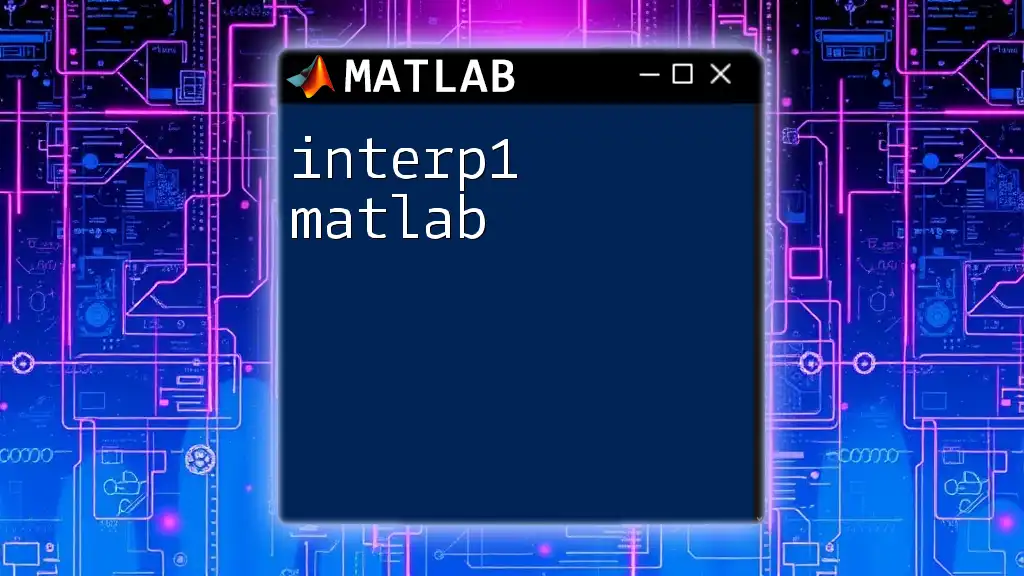
Practical Applications
Engineering
Inverse cosine plays a crucial role in engineering, especially in signal processing. Here, it can be utilized to interpret phase shifts in waveforms, helping engineers understand and manipulate signals for better performance.
Physics
In the field of physics, the inverse cosine function is instrumental in calculating angles of reflection and refraction. For example, using the inverse cosine can help you determine the angles in optics, particularly when applying Snell's Law to transition between different media.
Computer Graphics
When it comes to computer graphics, the `acos` function is essential for calculating the orientation and angle between objects. This becomes particularly relevant when transforming and rotating 3D objects or when determining the correct lighting angles in scenes.
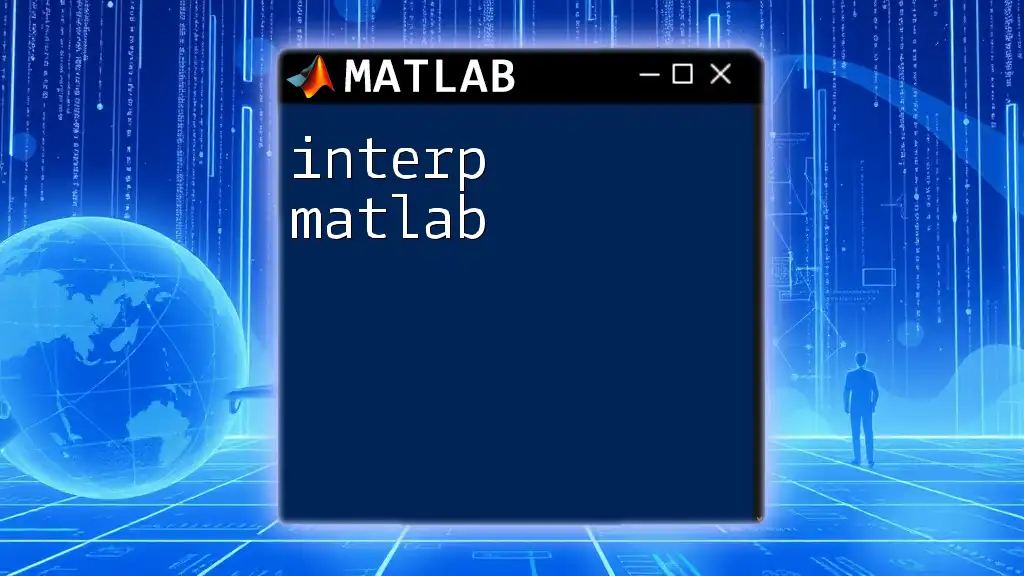
Common Errors and Troubleshooting
Error Handling
Understanding typical errors associated with the `acos` function can help ensure successful calculations.
Range Error
One common issue arises when input values exceed the allowable range of [-1, 1]. For instance, executing the following code:
angle = acos(2)
would lead to an error, as 2 is outside the range for cosine values.
Complex Numbers
When the input value goes out of the valid range, MATLAB may return complex results. For example:
angle = acos(1.5)
This command would typically generate a warning about obtaining a complex number, as 1.5 is not a valid cosine result.
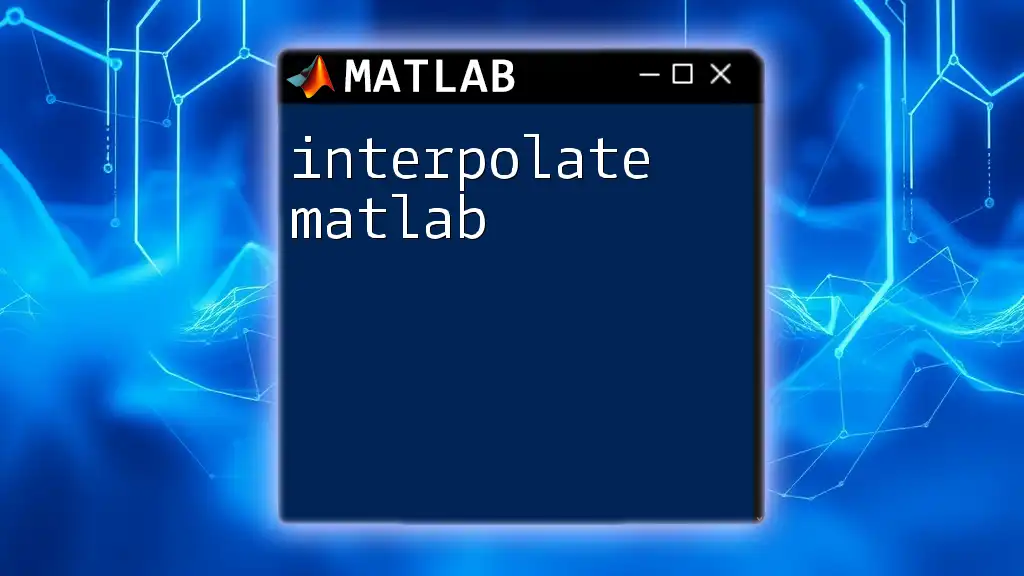
Conclusion
Through this comprehensive guide on using the inverse cosine function in MATLAB, we've delved into the syntax, practical examples, and applications while addressing common pitfalls. By mastering the `acos` function, you will enhance your programming capabilities, enabling you to apply it effectively in various scientific and engineering contexts.
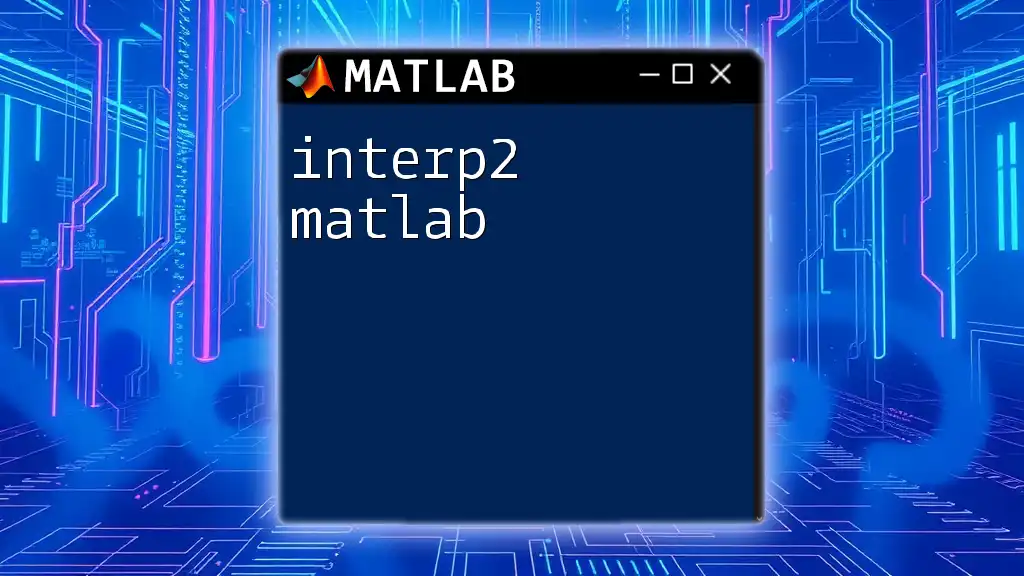
Additional Resources
Recommended Tutorials and References
Developing your understanding further can be achieved through exploring MATLAB's official documentation on trigonometric functions. Additionally, engaging with online coding forums may enrich your programming journey by connecting with a community of learners and experts.
Final Thoughts
Take the time to practice with different examples and actively integrate the `acos` function into your projects. The skills you build today will deepen your understanding of trigonometric functions and their applications in real-world scenarios. Happy coding!